Overcoming Latency Issues in Kafka Benchmarks on Chronicle Queue
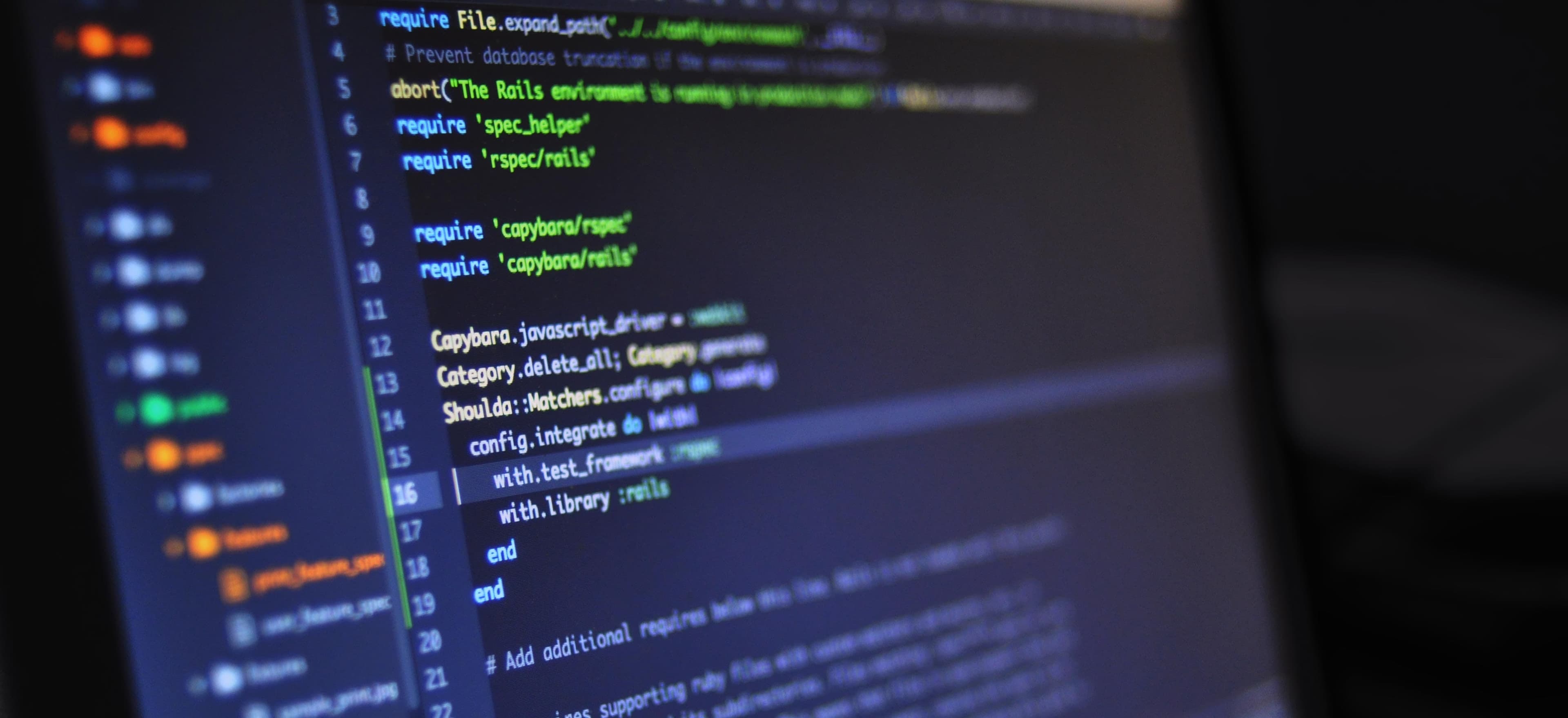
- Published on
Overcoming Latency Issues in Kafka Benchmarks on Chronicle Queue
In the world of distributed systems, achieving low latency while handling high throughput is the Holy Grail. Apache Kafka, an open-source stream-processing platform, has earned significant accolades for its speed and scalability. However, when benchmarking Kafka against other systems, such as Chronicle Queue, latency can sometimes fall short of expectations. In this blog post, we will explore ways to overcome latency issues while benchmarking Kafka against the Chronicle Queue, explaining the underlying principles and presenting code snippets for better understanding.
Understanding Kafka and Chronicle Queue
Kafka Overview
Kafka is designed to handle real-time data feeds with high throughput. It operates on a publish-subscribe model, allowing producers to publish data to topics, and consumers to subscribe and process that data. However, latency can be affected by several factors, such as the configuration of the brokers, network conditions, and client behavior.
Chronicle Queue Overview
Chronicle Queue, on the other hand, is an open-source messaging library aimed at delivering low-latency data storage and retrieval. It employs a memory-mapped file approach, minimizing system calls and using caching effectively. This design yields remarkable performance, especially where microsecond latencies are paramount.
The Importance of Benchmarking
Benchmarking offers essential insights into the performance characteristics of different systems. When comparing Kafka to Chronicle Queue, understanding the latency factors can help you tune your Kafka setup or adapt your messaging strategy.
Key Latency Factors in Kafka
-
Network Configuration: Network conditions significantly affect Kafka's latency. Consistent, high-speed networks typically yield better performance.
-
Broker and Topic Configuration: Each Kafka topic has configurations such as replication factor, number of partitions, and log retention settings, all of which can impact latencies.
-
Client Configuration: The performance of Kafka's client libraries can directly influence latencies. Using
acks=all
means the producer will wait for all replicas to acknowledge receipt, affecting performance. -
Message Size: Larger messages can lead to increased latencies due to more time needed for serialization and transmission.
Chronicle Queue Considerations
Chronicle Queue can deliver lower latency due to its design, but it's worth considering factors such as:
-
Threading: Chronicle Queue employs a lock-free data structure that works well with multi-threading. Optimal thread-per-CPU ratios are important.
-
Configuration Settings: Tuning settings such as buffer size, file size, and cache configuration can lead to better performance.
-
Serialization Format: By employing efficient serialization formats, you can minimize the data overhead and improve response times.
Overcoming Latency Issues in Kafka
Let's present some strategies to optimize Kafka's performance to minimize latency:
1. Adjusting Broker Configurations
You can optimize configurations in server.properties
:
# Set a lower acknowledgment level
acks=1
This means that the leader will acknowledge when it has written the message. It significantly reduces latency but may increase the chances of data loss in case of a failure.
2. Configuring Producer Settings
You can customize producer settings to enhance responsiveness:
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("linger.ms", 5); // Wait for 5ms for more messages
props.put("buffer.memory", 33554432); // 32 MB buffer size
KafkaProducer<String, String> producer = new KafkaProducer<>(props);
Setting linger.ms
allows messages to accumulate in the buffer, improving throughput at the expense of increased latency for the first few messages.
3. Selecting the Right Client Library
The Java client library generally provides the best performance, but various configurations and optimization can also be done. Check out Confluent's Kafka Client Docs for tailored configurations.
4. Multi-Partitioning Strategy
Increasing the number of partitions can accelerate parallelism, leading to reduced latencies:
AdminClient adminClient = AdminClient.create(props);
NewPartitions newPartitions = NewPartitions.increaseTo(numPartitions);
adminClient.createPartitions(Collections.singletonMap(topicName, newPartitions));
By allowing more partitions, messages can be processed across various brokers, reducing latency by improving parallel processing.
Kafka and Chronicle Queue Benchmarking
Both Kafka and Chronicle Queue have unique strengths. When assessing which to use, it's essential to consider application requirements, desired latency, message volume, and throughput demands.
To benchmark, you can use the following steps:
-
Setup Environment: Ensure both systems are deployed in similar environments with similar data loads.
-
Use a Benchmarking Tool: Tools like Apache JMeter or custom scripts can help measure throughput and latency.
-
Perform Load Testing: Generate and consume data against both systems under identical load scenarios.
-
Analyze Metrics: Collect metrics such as throughput, latency, and error rates to understand which system is more efficient.
Sample Benchmark Code
Here is an example of how you can measure latency using a simple producer-consumer setup in Kafka:
long startTime = System.nanoTime();
producer.send(new ProducerRecord<>(topicName, key, value));
long endTime = System.nanoTime();
long latency = endTime - startTime; // Latency in nanoseconds
System.out.println("Latency: " + latency + " nanoseconds.");
This code snippet captures the time taken to send a message to Kafka, allowing you to measure performance changes when tweaking configurations.
Key Takeaways
In conclusion, overcoming latency issues in Kafka while benchmarking against Chronicle Queue involves a meticulous evaluation of multiple factors such as configurations, message sizes, networking, and client behaviors. While both systems serve different use cases, it's essential to tailor their configurations to suit your application needs.
In the fast-paced arena of distributed systems, monitoring and adapting continually can make a significant difference. Whether you choose Kafka, Chronicle Queue, or another messaging framework, careful benchmarking will guide your architecture choices.
Additional Resources
For further reading, consider exploring:
Stay tuned for future posts where we will delve deeper into specific strategies for optimizing Kafka configurations and advanced techniques for achieving lower latencies in distributed messaging systems!
Checkout our other articles