Common Pitfalls in JPA 2.1 Enum Mapping and How to Avoid Them
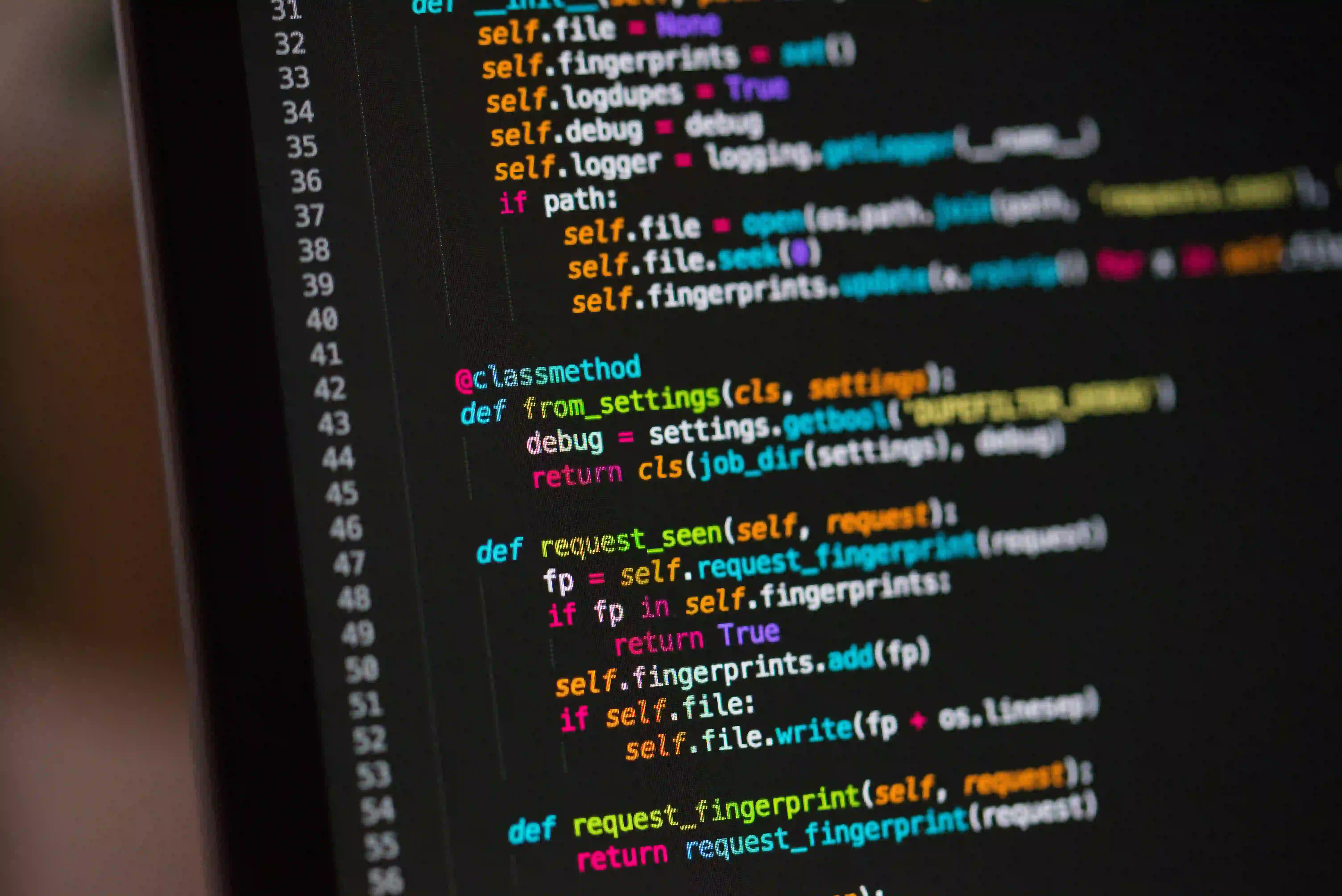
Common Pitfalls in JPA 2.1 Enum Mapping and How to Avoid Them
Java Persistence API (JPA) 2.1 introduced a standardized way of mapping Java Enums to database columns. Although the flexibility of enum mapping simplifies data interactions, developers often encounter pitfalls that can complicate their projects. In this blog post, we will explore common mistakes and how to avoid them for clean and effective JPA Enum mappings.
Understanding JPA Enum Mapping
Enums are a special Java type used to represent a fixed set of constants. They are particularly useful in database column mapping, where you might want to represent a finite set of values, such as order statuses (e.g., PENDING, COMPLETED, CANCELLED).
In JPA 2.1, you can map enums using two primary strategies:
- ORDINAL: Maps the enum to its position in the declared enum constants (starting with 0).
- STRING: Maps the enum to its name as a string.
Enum Declaration Example
public enum OrderStatus {
PENDING,
COMPLETED,
CANCELLED;
}
This declaration of an enum OrderStatus
will be our example throughout this discussion.
Common Pitfalls in JPA 2.1 Enum Mapping
1. Using ORDINAL Without Caution
Using ORDINAL
mapping is tempting for its simplicity. However, it presents significant risks. If the order of enum values changes, existing database records can effectively become corrupted.
Example of ORDINAL Mapping
@Entity
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Enumerated(EnumType.ORDINAL)
private OrderStatus status;
}
Why Avoid This?
- Breaking Changes: If you ever reorder the enum constants, it will lead to inconsistencies.
- Hard to Read: The database entry becomes harder to interpret without checking the code.
Avoid It By Using STRING Mapping
Opt for STRING
mapping, which improves readability and prevents the pitfalls associated with ORDINAL. Here’s how you can do this:
@Entity
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Enumerated(EnumType.STRING)
private OrderStatus status;
}
This mapping stores the actual enum name as a string, allowing for clearer relationships with backend logic and a safeguard against reordering issues.
2. Misunderstanding Enum Persistence During Object Merging
When working with entity merging, ensure your enum values are persisted correctly. Failing to do so can cause mismatches during updates or when retrieving data.
Common Mistake
Let’s say you have an existing order with an OrderStatus.PENDING
in the database. When updating, if you set the order status with a string value or an "unknown" enum, it might not hit the database as expected.
Proper Handling of Enum Updates
public void updateOrderStatus(Order order, String newStatus) {
order.setStatus(OrderStatus.valueOf(newStatus));
entityManager.merge(order);
}
3. Neglecting to Handle Enum in DTOs (Data Transfer Objects)
When you are using DTOs for transferring data between layers, be mindful of how enums are handled. If not appropriately defined, you may find yourself with inconsistencies.
Example of a DTO without Enum Handling
public class OrderDTO {
private Long id;
private String status; // This should be an enum for better type safety - "OrderStatus" instead.
// Getters and Setters
}
Instead, redefine this DTO to include the enum:
public class OrderDTO {
private Long id;
private OrderStatus status;
// Getters and Setters
}
By using the enum type, you enforce type safety and reduce the risk of errors down the line.
4. Overlooking Enum Customization for Specific Use-Cases
Sometimes, default enum behavior may not fulfill specific needs. For instance, you may want to store a custom string representation instead of the default enum name.
Custom Enum Representation Example
public enum OrderStatus {
PENDING("Pending"),
COMPLETED("Completed"),
CANCELLED("Cancelled");
private String displayValue;
OrderStatus(String displayValue) {
this.displayValue = displayValue;
}
public String getDisplayValue() {
return displayValue;
}
}
With the above structure, you can easily access a user-friendly representation of your Enum:
public String toString() {
return displayValue;
}
5. Ignoring Enum Value Validations
Always validate enum values particularly when relying on external inputs such as REST APIs. Directly mapping user inputs to enum values without validation can throw exceptions or lead to data integrity issues.
Example of Value Validation
public void setStatus(String statusString) {
try {
this.status = OrderStatus.valueOf(statusString);
} catch (IllegalArgumentException e) {
throw new IllegalArgumentException("Invalid status: " + statusString);
}
}
Wrapping Up
JPA 2.1 Enum Mapping can significantly simplify your data persistence layer, but it also comes with its share of pitfalls. By understanding the nuances of enum mapping and keeping best practices in mind, you can save yourself from common issues.
For further reading on JPA and Hibernate, consider checking out the official JPA documentation and Hibernate Enums Tutorial for more in-depth discussion and advanced topics.
By making the right choices with enum mapping, you ensure that your applications remain robust, maintainable, and easy to understand. Happy coding!