Common Spring Boot and Apache Camel Integration Issues
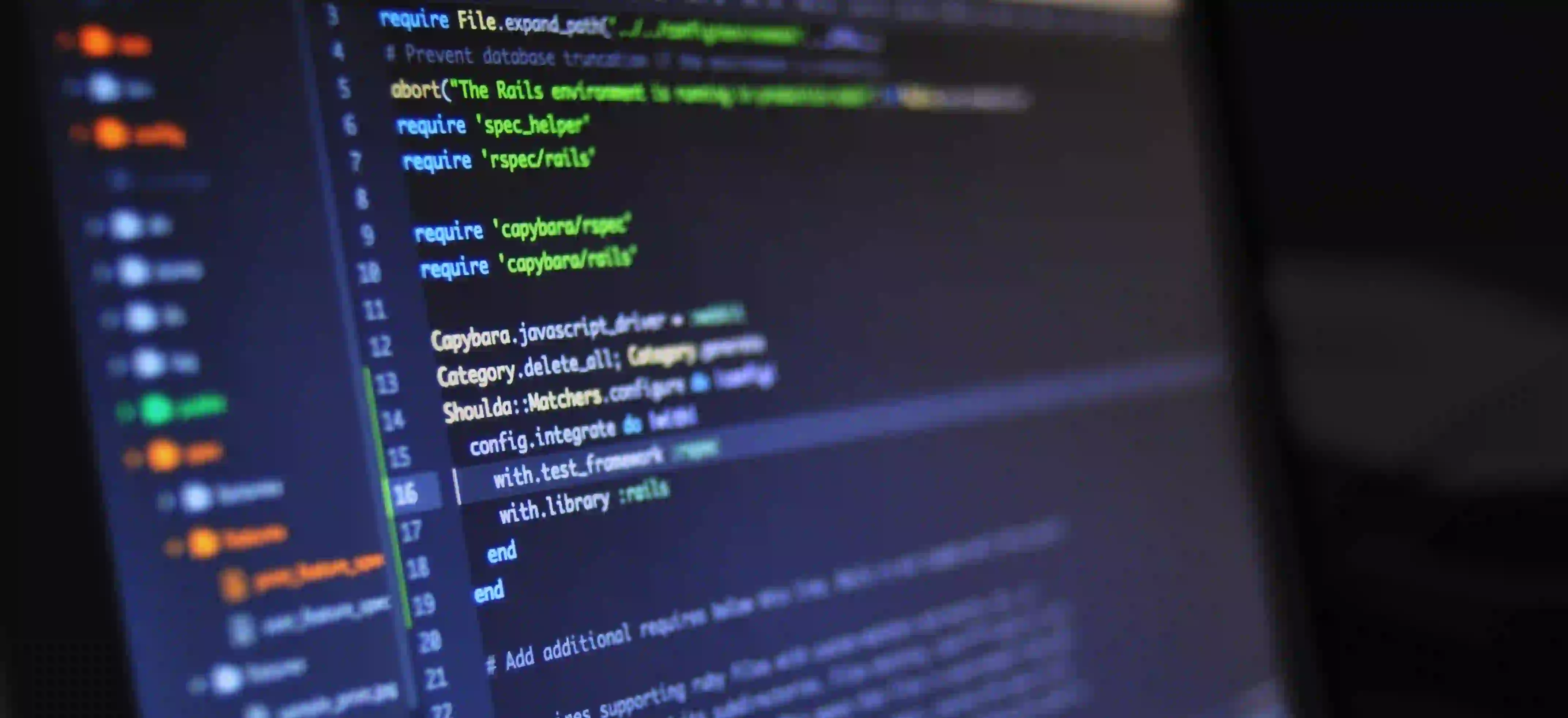
Common Spring Boot and Apache Camel Integration Issues
Integrating Spring Boot with Apache Camel has proven to be an efficient way to build robust microservices. However, as with any framework, developers may encounter issues during this integration. In this blog post, we will discuss some common challenges and their solutions, ensuring that your integration goes smoothly.
1. Dependency Conflicts
One of the most common issues arises from dependency conflicts, specifically between Spring and Camel libraries. Often, this results in ClassNotFoundException
or NoSuchMethodError
.
Solution
Ensure that you are using compatible versions of Spring and Apache Camel. Here’s an example of how to define dependencies in pom.xml
to avoid conflicts:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-spring-boot-starter</artifactId>
<version>3.20.0</version>
</dependency>
Why: Keeping dependencies up-to-date and compatible prevents runtime errors, making your application more stable.
2. Configuration Issues
Using Apache Camel's configuration features within a Spring Boot application can lead to confusion, especially when using YAML or properties files.
Solution
Follow best practices when defining routes and configurations. Here’s how you can define a simple route in a configuration class:
import org.apache.camel.builder.RouteBuilder;
import org.springframework.stereotype.Component;
@Component
public class MyRouteBuilder extends RouteBuilder {
@Override
public void configure() throws Exception {
from("timer:foo?period=1000")
.setBody(constant("Hello World"))
.to("log:info");
}
}
Why: By structuring your routes within configuration classes and using Camel's DSL (Domain Specific Language), it becomes easier to manage routing logic.
3. Incorrect Bean Wiring
A misleading error that often surfaces is when Spring Boot fails to wire Camel's components correctly. This could be due to improper annotations or configuration.
Solution
Make sure you use the @Component
annotation correctly and import the necessary packages. Validate that all required beans are defined and accessible. Here's how to ensure correct wiring:
import org.apache.camel.CamelContext;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class MyRunner implements CommandLineRunner {
@Autowired
private CamelContext camelContext;
@Override
public void run(String... args) {
// your logic
}
}
Why: Getting the bean context right is vital for leveraging dependency injection correctly, which is fundamental to Spring's architecture.
4. Message Handling Issues
Sometimes, you may encounter problems with message processing. For instance, a message might not be processed as expected due to incorrect routes.
Solution
Use error handlers to manage messages effectively. Here’s a progressive message-handling setup:
@Override
public void configure() {
onException(Exception.class)
.handled(true)
.to("log:error");
from("direct:start")
.process(exchange -> {
// Your processing logic
})
.to("mock:result");
}
Why: Implementing error handlers allows a graceful recovery from unexpected events, enhancing the robustness of your application.
5. Performance Issues
Integrating multiple components using Apache Camel may lead to performance-related bottlenecks, especially under load.
Solution
Utilize Camel's asynchronous processing features and thread management. Here's an example:
from("direct:start")
.threads().executorServiceRef("myThreadPool")
.process(exchange -> {
// heavy processing
});
And define your thread pool bean in application.properties
:
camel.threadPool.myThreadPool.size=10
Why: Configuring thread pools properly can significantly improve throughput and responsiveness, critical for high-load applications.
6. Debugging Challenges
When issues arise, debugging can become a challenge due to the complexity of integrated systems.
Solution
Enable Camel logging to trace execution flow. Use the following configuration in application.properties
:
logging.level.org.apache.camel=DEBUG
Example of Logging in Camel
When designing a critical route, you can include logging:
from("direct:myRoute")
.log("Processing message: ${body}")
.to("mock:out");
Why: Effective logging aids in monitoring the application, helping to quickly identify and troubleshoot problems.
7. Lack of Documentation and Resources
Developers new to Apache Camel and Spring Boot may struggle with understanding the available features and best practices due to sparse documentation.
Solution
Refer to Apache Camel Documentation and Spring Boot's official guides for comprehensive information. Participating in community forums like Stack Overflow and Camel’s user mailing list can also provide additional support.
Why: Engaging with community resources can offer more practical examples and solutions that may not be encountered in the official documentation.
Key Takeaways
Integrating Spring Boot with Apache Camel offers remarkable capabilities for building efficient microservices. By proactively recognizing common issues and implementing best practices, you can enhance your application's performance and reliability. Remember that thorough testing and continuous learning play vital roles in mastering these technologies.
Happy coding! If you have more questions or particular integration issues, feel free to leave a comment below!