Is Kotlin Really Better than Java for Android Development?
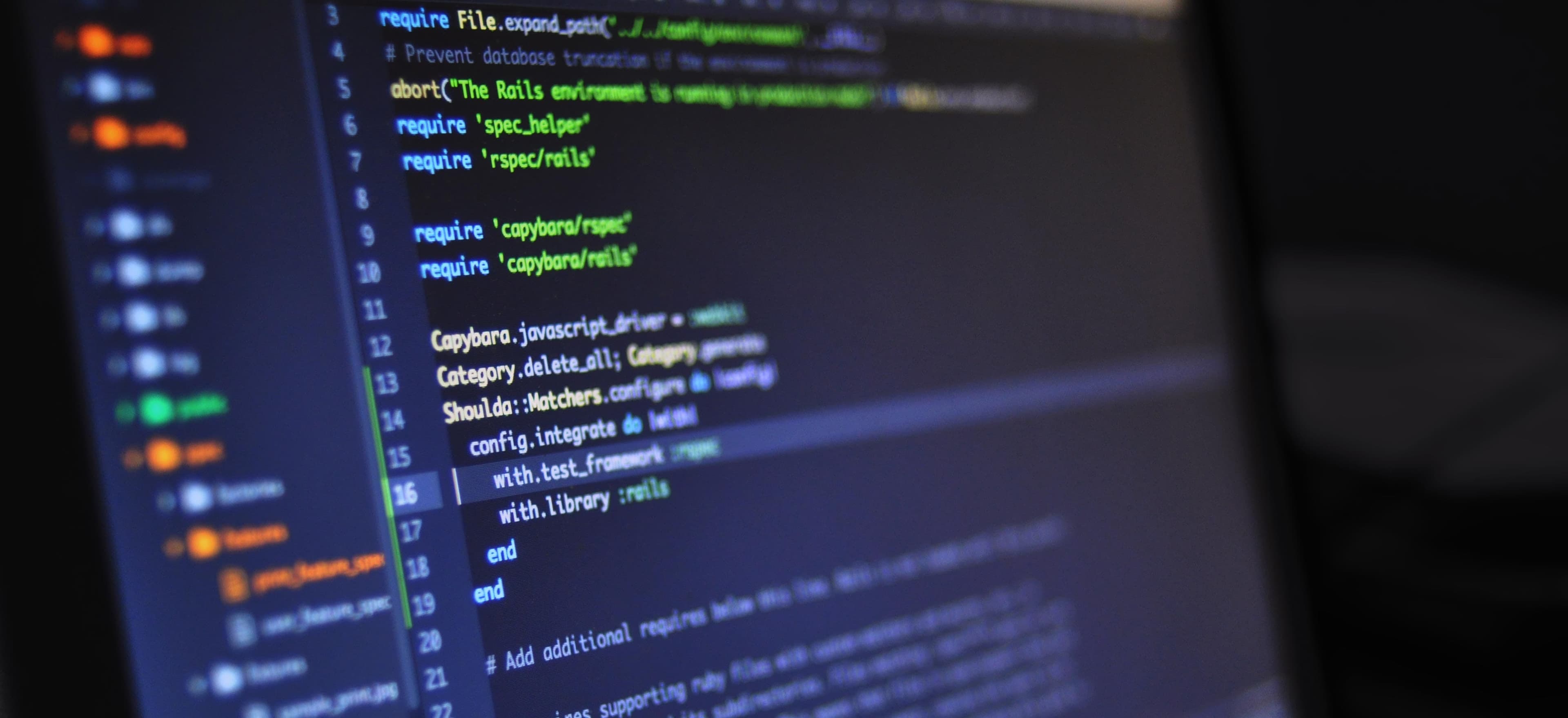
- Published on
Is Kotlin Really Better than Java for Android Development?
Android development has evolved significantly over the years, with various programming languages and frameworks gaining popularity. Among them, Kotlin has emerged as a prominent choice, often viewed as a substitute or improvement over Java. But is Kotlin really better than Java for Android development? Let's delve into the details by examining the features, syntax, and overall developer experience each language offers.
A Brief Overview of Java and Kotlin
Java has been the backbone of Android development since its inception. Backed by a vast ecosystem and extensive libraries, Java has proven its reliability and robustness over the years. Despite its extensive use, Java can sometimes feel verbose, which leads to increased development time.
Kotlin, introduced by JetBrains and officially supported by Google in 2017, aims to address some of the shortfalls of Java. It's designed to be concise, expressive, and safe, making it an appealing alternative for Android developers. But what exactly are the features that make Kotlin stand out?
1. Syntax Sugar and Conciseness
One of the most noticeable differences between Kotlin and Java is the level of conciseness in coding. Here’s a simple comparison:
Java Implementation:
// Java
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button button = (Button) findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Code to be executed when button is clicked
}
});
}
}
Kotlin Implementation:
// Kotlin
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
button.setOnClickListener {
// Code to be executed when button is clicked
}
}
}
Commentary on Conciseness
Kotlin reduces boilerplate code significantly. Notice how in Kotlin, there's no need to use the findViewById
method and the lambda expression for the setOnClickListener
improves readability. This simplicity allows developers to focus more on functionality rather than syntax.
2. Null Safety
Java's null pointer exceptions (NPE) have plagued developers for years. Kotlin addresses this with a strong type system that enforces null safety. By distinguishing between nullable and non-nullable types, Kotlin reduces the risks associated with null references.
Kotlin Example:
var name: String? = null // This is explicitly nullable
Java Example:
String name = null; // Nullability is implicit and unguarded
Commentary on Null Safety
Kotlin’s null safety feature ensures that developers are mindful of potential null values right from the declaration. This reduces the likelihood of runtime crashes and enhances code stability.
3. Extension Functions
Kotlin introduces extension functions, which enable developers to extend existing classes without modifying their source code. This feature enhances code reusability and flexibility.
Kotlin Example:
fun String.addExclamation(): String {
return this + "!"
}
fun main() {
val excitedString = "Hello".addExclamation()
println(excitedString) // Output: Hello!
}
Commentary on Extension Functions
With extension functions, developers can create more expressive and versatile APIs, promoting a cleaner codebase. It allows Android developers to add utility methods to classes without resorting to static utility methods in Java.
4. Coroutines for Asynchronous Programming
Handling background tasks and asynchronous programming can be cumbersome in Java. Kotlin addresses this challenge through coroutines, which provide an efficient way to work with asynchronous code.
Kotlin Example:
import kotlinx.coroutines.*
fun main() = runBlocking {
launch {
delay(1000L)
println("World!")
}
println("Hello,")
}
Commentary on Coroutines
Kotlin’s coroutines simplify the management of complex asynchronous tasks, making code easy to understand and maintain. Developers can write sequential code that runs asynchronously without the convoluted callback methods common in Java.
5. Interoperability with Java
One of Kotlin's significant advantages lies in its interoperability with existing Java code. This means Android developers can gradually migrate their applications from Java to Kotlin without a complete rewrite.
// Using Java libraries in Kotlin
val list = ArrayList<String>()
list.add("Hello")
Commentary on Interoperability
This feature allows developers to adopt Kotlin incrementally, ensuring that no existing code is rendered obsolete, facilitating a smooth transition.
When to Choose Java?
While Kotlin has numerous advantages, Java is still a viable option for many scenarios. Here's why:
-
Legacy Code: If you are working on a project with a significant amount of existing Java code, sticking with Java can lead to fewer complications.
-
Learning Curve: Java may be more familiar to developers who have extensive experience in traditional programming paradigms.
-
Tooling: Some tools or libraries may still be tailored predominantly for Java, although this gap is steadily closing.
Closing Remarks: Kotlin vs. Java
The landscape of Android development is evolving, and selecting between Kotlin and Java ultimately depends on your project needs and personal/team familiarity with either language. Kotlin's advantages in terms of conciseness, null safety, extension functions, and coroutines make it an attractive choice for modern Android development.
For further reading, check out the official Kotlin documentation and consult the extensive Android developer guide for best practices and more insights.
In summary, while both languages offer their unique strengths, Kotlin is often seen as the "better" option due to its modern features and developer-friendly design. Should you make the switch? If you’re starting a new project or looking to integrate modern features into your Android apps, Kotlin may well be the best choice for your development toolkit.
Checkout our other articles