Avoiding Common JPA Pitfalls: Top Mistakes to Ditch
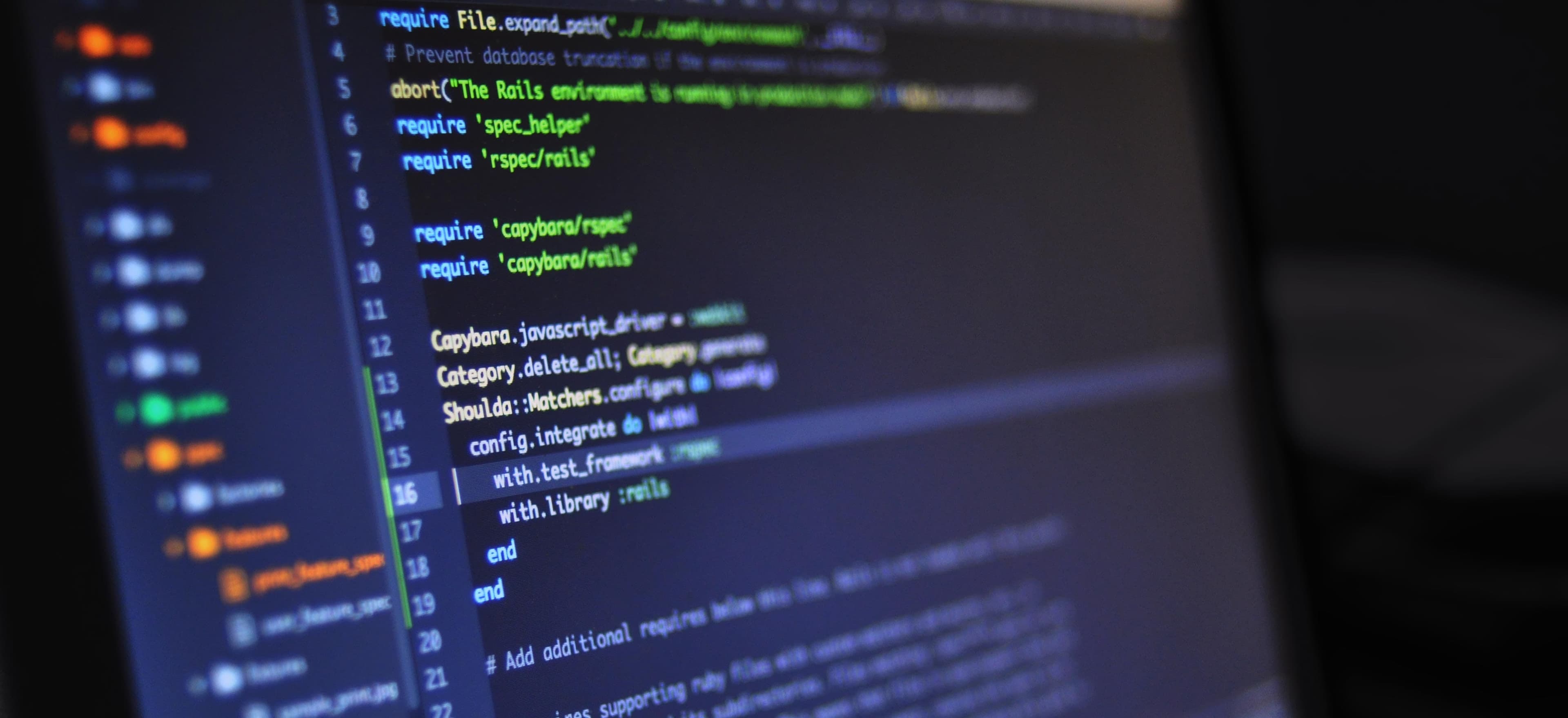
- Published on
Avoiding Common JPA Pitfalls: Top Mistakes to Ditch
Java Persistence API (JPA) is a powerful tool that simplifies the task of managing relational data in Java applications. However, it can be deceptively simple. Newcomers and even seasoned developers can encounter pitfalls that can lead to performance issues, data inconsistency, and other headaches. In this blog post, we will explore the most common JPA mistakes and provide practical solutions to avoid them.
What is JPA?
Before we dive into the mistakes, let's briefly cover what JPA is. JPA is a specification for managing relational data in Java. It provides a set of interfaces and annotations to work with Java objects and their interactions with the database. JPA is often implemented via frameworks like Hibernate or EclipseLink.
Pitfall 1: Ignoring Lazy Loading
What is Lazy Loading?
Lazy loading is a design pattern that delays the initialization of an object until it is needed. In JPA, lazy loading is typically used for entity associations. This means related entities are not immediately loaded with the parent entity but are instead fetched on demand.
Why You Should Ditch This Mistake
Ignoring lazy loading can lead to performance issues. If you eagerly fetch all associations without necessity, you will end up retrieving too much data, which can slow down your application. This is particularly true for large object graphs where fetching everything upfront can overwhelm the network and memory resources.
Code Example:
@Entity
class Order {
@OneToMany(mappedBy = "order", fetch = FetchType.LAZY)
private List<OrderItem> orderItems;
}
In this code snippet, we define a one-to-many relationship between Order
and OrderItem
entities. By using fetch = FetchType.LAZY
, we ensure that order items are loaded only when needed, rather than all at once.
Pitfall 2: Forgetting to Manage Transactions Properly
Transaction Management in JPA
Transactions are crucial for ensuring the integrity of operations within the database. Failing to manage transactions properly can lead to inconsistencies and data corruption.
Why You Should Ditch This Mistake
Improper transaction management can leave your application vulnerable to issues such as dirty reads, lost updates, or phantom reads. Using transactions correctly also helps maintain a consistent state across your database operations.
Code Example:
EntityTransaction transaction = entityManager.getTransaction();
try {
transaction.begin();
// Perform operations.
transaction.commit();
} catch (RuntimeException e) {
if (transaction.isActive()) {
transaction.rollback();
}
throw e; // Re-throwing is often helpful for debugging.
}
In the above example, we begin by starting a transaction. If any exception occurs, we ensure that the transaction is rolled back, preserving the state of the database.
Pitfall 3: Overusing Entity Relationships
Understanding Entity Relationships
In JPA, it’s easy to create elaborate relationships between entities, such as One-to-Many, Many-to-Many, etc. However, overdoing it can lead to complications.
Why You Should Ditch This Mistake
Complex entity relationships can make your code harder to read and maintain. They can also lead to performance degradation, especially if you're performing unnecessary joins when querying your database.
Code Example:
@Entity
class Customer {
@OneToMany(mappedBy = "customer", fetch = FetchType.LAZY)
private Set<Order> orders;
}
While this snippet establishes a relationship between Customer
and Order
, it's essential to ensure that such relationships are necessary. If a simpler design is viable, it’s always better to opt for it.
Pitfall 4: Not Utilizing JPA Caching
The Importance of Caching
JPA provides a mechanism to cache the frequently accessed data. Caching reduces the number of database queries and improves the performance of your application significantly.
Why You Should Ditch This Mistake
Not utilizing caching in your JPA layer can lead to unnecessary database calls, putting additional load on your database server. JPA's second-level cache can store entities, collections, and even query results.
Enabling Second-Level Cache
To enable JPA caching, ensure that your persistence configuration has caching enabled. This can be done in the persistence.xml
:
<persistence-unit name="my-persistence-unit">
<properties>
<property name="hibernate.enable_second_level_cache" value="true"/>
<property name="hibernate.cache.region.factory_class" value="org.hibernate.cache.ehcache.EhCacheRegionFactory"/>
</properties>
</persistence-unit>
By enabling second-level caching, you can avoid unnecessary queries, significantly boosting the performance of your application.
Pitfall 5: Ignoring Fetch Strategies
Importance of Fetch Strategies
Fetch strategies dictate how related entities are retrieved from the database. In JPA, developers can choose between eager loading and lazy loading.
Why You Should Ditch This Mistake
Using the wrong fetch strategy can result in performance issues. Eager fetching is useful when you know you will need the related data, but if used indiscriminately, it can lead to loading unnecessary data.
Code Example:
@Entity
class Product {
@ManyToOne(fetch = FetchType.LAZY)
private Category category;
}
Using FetchType.LAZY
for the category
field here allows the Product
entity to load the Category
only when it’s explicitly called. This not only saves resources but also keeps your application responsive.
The Last Word
Working with JPA can be enjoyable and efficient when you avoid the common pitfalls outlined in this post. Understanding lazy loading, transaction management, entity relationships, caching, and fetch strategies are foundational to building high-performance Java applications.
If you're interested in deepening your knowledge of JPA best practices, I recommend reading on the Spring Persistence Documentation or exploring Hibernate’s documentation for additional insights.
By ditching these top mistakes, you can transform your JPA projects into models of performance and maintainability. Happy coding!