Overcoming Performance Issues in Cross-Platform Apps
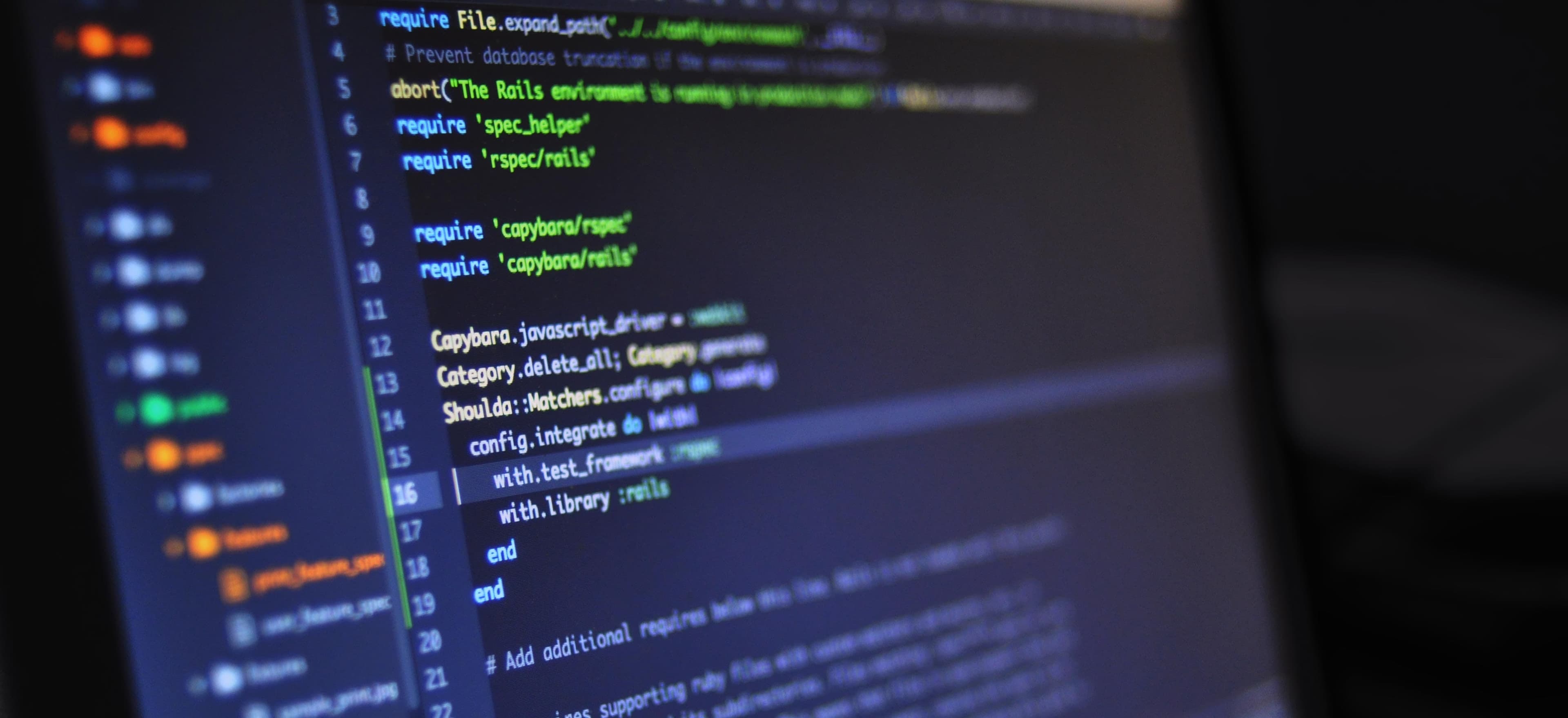
- Published on
Overcoming Performance Issues in Cross-Platform Apps
Cross-platform app development offers developers the flexibility to build applications that can operate on multiple platforms, such as Android and iOS, with a single codebase. However, along with this convenience, developers face a significant challenge: performance issues. Performance in cross-platform apps is critical, as it directly affects user experience, satisfaction, and ultimately, app success. In this blog post, we will explore common performance challenges that arise in cross-platform apps, along with effective strategies to overcome them.
Understanding the Performance Challenges
Cross-platform development typically involves using frameworks like React Native, Flutter, or Xamarin. These frameworks allow developers to write code once and deploy it across various platforms, but they introduce performance challenges due to differences in rendering engines, platform-specific behaviors, and device differences.
1. Overhead from Abstraction
One of the main issues with cross-platform development is the overhead incurred when using an abstraction layer. This added layer can significantly slow down the app, especially for graphics-intensive applications such as games or multimedia editors.
- Solution: It’s crucial to minimize unnecessary abstraction where possible. Focus on using native components for critical performance sections of the app. For instance, utilize native UI components instead of relying on custom or generic ones.
2. Inefficient Rendering
Rendering issues often arise from the complex structure of UI elements being used in cross-platform frameworks. The frequent redraws and re-renders can lead to sluggish performance.
- Solution: Use profiling tools provided by the frameworks to identify performance bottlenecks in rendering. Tweaking the rendering logic could involve strategies such as debouncing input or leveraging shouldComponentUpdate in React to prevent unnecessary renders.
3. Network Latency
Network calls in cross-platform apps can lead to lag if not managed correctly. Service calls can block UI interactions, creating a sluggish experience.
- Solution: Implement asynchronous calls and utilize techniques like caching APIs to reduce the number of requests your app makes. Popular libraries like Axios help manage requests efficiently in JavaScript frameworks.
Example using Async/Await in JavaScript:
async function fetchData(url) {
try {
const response = await fetch(url);
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching data:', error);
}
}
This code helps in fetching data asynchronously without blocking the main thread, enhancing user experience.
Best Practices for Cross-Platform Performance
To ensure your cross-platform apps run smoothly, consider implementing the following best practices:
1. Optimize Images and Assets
Images and other assets can significantly affect load times and performance. Large assets can slow down rendering and increase memory consumption.
Optimization Techniques:
- Use image formats like WebP or SVG that are optimized for web.
- Implement lazy loading for images that are off-screen.
2. Use Code Splitting
Code splitting is a technique that helps load only the essential code initially, reducing the burden on the application's startup time.
Example in React:
const About = React.lazy(() => import('./About'));
function App() {
return (
<React.Suspense fallback={<div>Loading...</div>}>
<About />
</React.Suspense>
);
}
This example demonstrates how to split code by lazy-loading the About component. It can significantly enhance performance by reducing the initial load time.
3. Profiling and Monitoring
Profiling tools are an integral part of the performance optimization process. Regularly monitoring your app can help identify and alleviate potential issues.
- Use tools like Google Lighthouse or React Native Performance Monitor to track performance metrics and address them proactively.
4. Minimize Stateful Components
In many frameworks, stateful components can lead to unnecessary re-renders, especially if they hold large amounts of data.
- Solution: Use local state as much as possible and lift the state only when necessary. Consider using React’s context for shared state management to reduce re-renders on unrelated components.
5. Leverage Native Modules
For complex functionalities that require high performance, use native modules when possible. While cross-platform frameworks allow for extensive reusable code, integrating native components can vastly improve performance.
Example with React Native:
If you require high-performance computations, you might want to write a native component in Swift for iOS or Kotlin for Android. This allows you to take advantage of native performance without sacrificing cross-platform functionality.
Additional Resources
To dive deeper into optimizing your cross-platform apps, consider these two resources:
The Last Word
Performance issues in cross-platform applications can be challenging, but they are not insurmountable. By understanding the underlying challenges, employing best practices, and utilizing available tools, you can significantly enhance the performance of your app. Your users will appreciate the difference, resulting in higher engagement and satisfaction.
Remember to regularly profile your application, stay updated on tools and techniques, and keep user experience at the forefront of your development process. Addressing performance proactively will ensure your cross-platform app stands out in an increasingly competitive market.
By closely following these strategies, you can build a performant, user-friendly cross-platform app that meets the needs of users across various devices.
Checkout our other articles