Common AJAX Issues in Spring MVC: How to Troubleshoot
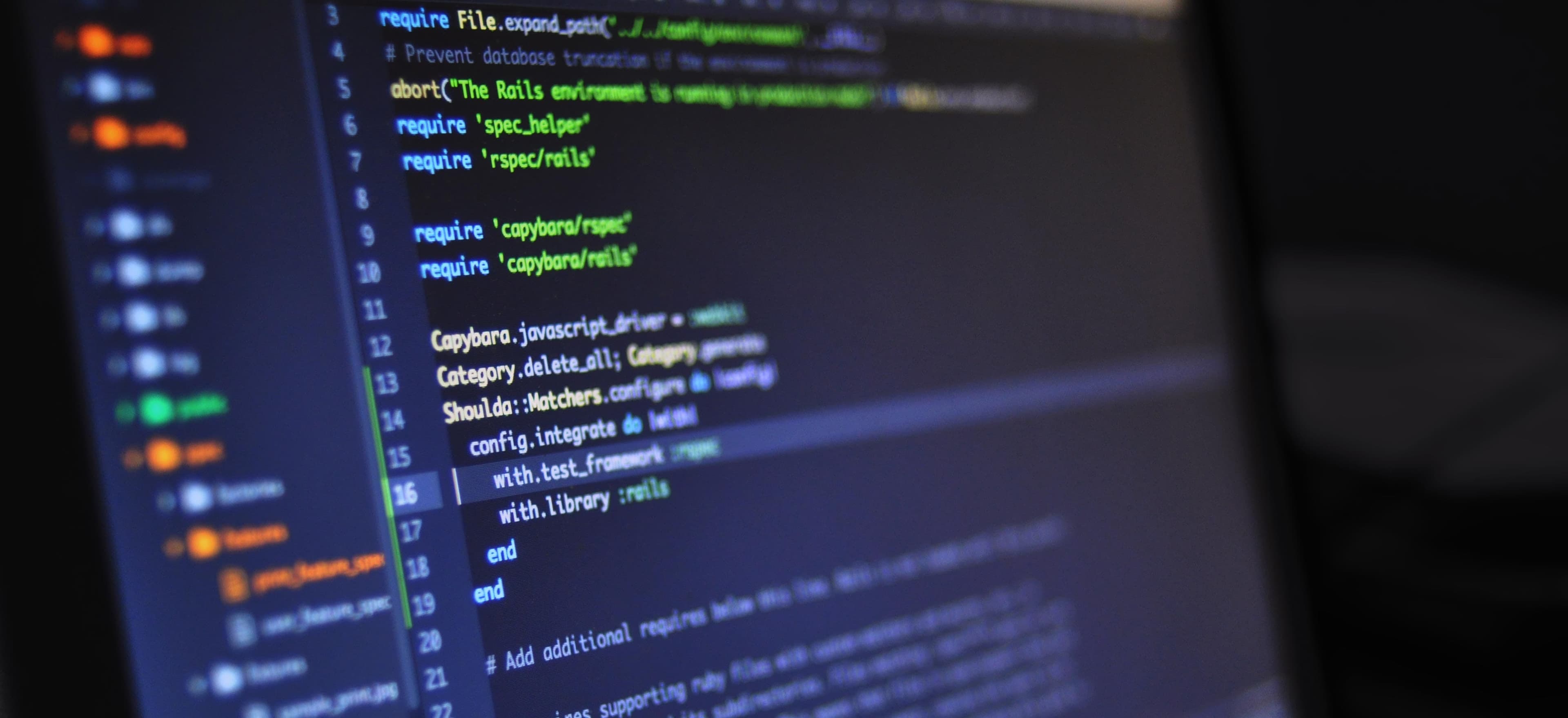
- Published on
Common AJAX Issues in Spring MVC: How to Troubleshoot
AJAX (Asynchronous JavaScript and XML) is a powerful technique used to enhance user experience by updating web pages asynchronously. In the context of Spring MVC, using AJAX can significantly improve the interaction between the front end and back end of your application. However, it's common to encounter several issues when implementing AJAX with Spring MVC. This blog post will explore these common problems and provide effective troubleshooting strategies.
Understanding AJAX in Spring MVC
Before delving into troubleshooting, it's essential to have a fundamental understanding of how AJAX operates in the context of Spring MVC.
When an AJAX request is made, the browser sends a request to the server without reloading the page. The server processes the request and returns a response, typically in JSON format. Spring MVC facilitates this interaction via @ResponseBody
, which directly converts the returned object into the desired format (like JSON).
@Controller
public class MyController {
@GetMapping("/api/data")
@ResponseBody
public MyData getData() {
return new MyData("Sample Data");
}
}
In this code snippet, the getData
method returns an instance of MyData
, which Spring will automatically convert to JSON because of the @ResponseBody
annotation.
Common AJAX Issues
1. Incorrect URL Mapping
A frequent issue when working with AJAX in Spring MVC is incorrect URL mapping. If the URL specified in the AJAX request does not match the controller's endpoint, the server will respond with a 404 Not Found error.
Troubleshooting Steps:
- Double-check your AJAX call's URL.
- Ensure your Spring controller mappings are correct.
Example:
$.ajax({
url: '/api/data',
type: 'GET',
success: function(data) {
console.log(data);
},
error: function(jqXHR, textStatus, errorThrown) {
console.error("Error: " + textStatus + " - " + errorThrown);
}
});
Make sure the endpoint /api/data
matches the controller's mapping.
2. Content-Type Mismatches
AJAX requests can face issues related to Content-Type. For instance, sending JSON data but incorrectly setting the Content-Type can lead to server misinterpretation.
Troubleshooting Steps:
- Ensure the AJAX request sets the appropriate Content-Type header, such as
application/json
for JSON data:
$.ajax({
url: '/api/data',
type: 'POST',
contentType: 'application/json',
data: JSON.stringify({ name: 'Sample Data' }),
success: function(response) {
console.log(response);
}
});
And ensure your Spring controller can handle this format:
@PostMapping("/api/data")
@ResponseBody
public ResponseEntity<String> saveData(@RequestBody MyData data) {
// Handle the incoming data
return ResponseEntity.ok("Data saved successfully");
}
3. CSRF Protection Issues
For Spring MVC applications, Cross-Site Request Forgery (CSRF) protection is enabled by default. This can interfere with AJAX requests unless handled correctly.
Troubleshooting Steps:
- Include the CSRF token in your AJAX requests. For instance, in HTML:
<meta name="_csrf" content="${_csrf.token}"/>
<meta name="_csrf_header" content="${_csrf.headerName}"/>
Then, extract this token in your JavaScript to include in your AJAX headers:
let token = $('meta[name="_csrf"]').attr('content');
let header = $('meta[name="_csrf_header"]').attr('content');
$.ajaxSetup({
beforeSend: function(xhr) {
xhr.setRequestHeader(header, token);
}
});
Debugging Strategies
When faced with AJAX issues, debugging can be daunting. Here are some strategies to help you identify problems effectively:
1. Check Browser Developer Tools
The built-in developer tools in your browser (accessible by pressing F12) can greatly assist in diagnosing problems. Here's what you can do:
- Network Tab: Monitor the request and response. Check for status codes, response data, and header information.
- Console Tab: Look for any JavaScript errors that may hinder the execution of your AJAX call.
2. Server Logs
Examining the server logs can provide insight into what went wrong. If the response returned an error, the logs will often show stack traces or explain the issue that occurred.
3. Use Postman
Using tools like Postman can help verify if the API endpoints are working as intended. Simulating requests outside the JavaScript context ensures that the server behaves as expected.
Best Practices
To prevent issues from arising and enhance your AJAX experience in Spring MVC, consider these best practices:
- Consistent Naming Conventions: Use descriptive names for your API endpoints and adhere to RESTful principles.
- Graceful Error Handling: Always handle errors gracefully in your AJAX requests. Provide user-friendly messages and fallback strategies.
- Documentation: Document your AJAX API endpoints well, so that other developers can easily understand how to interact with your server-side logic.
Lessons Learned
While AJAX can greatly enhance user experience, it can also lead to a range of issues when not implemented correctly within a Spring MVC framework. By pinpointing common issues and applying the provided troubleshooting strategies, you can streamline your development process and ensure smooth communication between your front-end and back-end.
For further reading on AJAX and Spring MVC, consider exploring the official Spring Framework documentation and MDN Web Docs on AJAX.
By following these guidelines and continually testing your implementations, you will be better prepared to tackle any challenges that AJAX may present in your Spring MVC applications. Happy coding!
Checkout our other articles