Top 5 Microservices Questions That Need Clear Answers
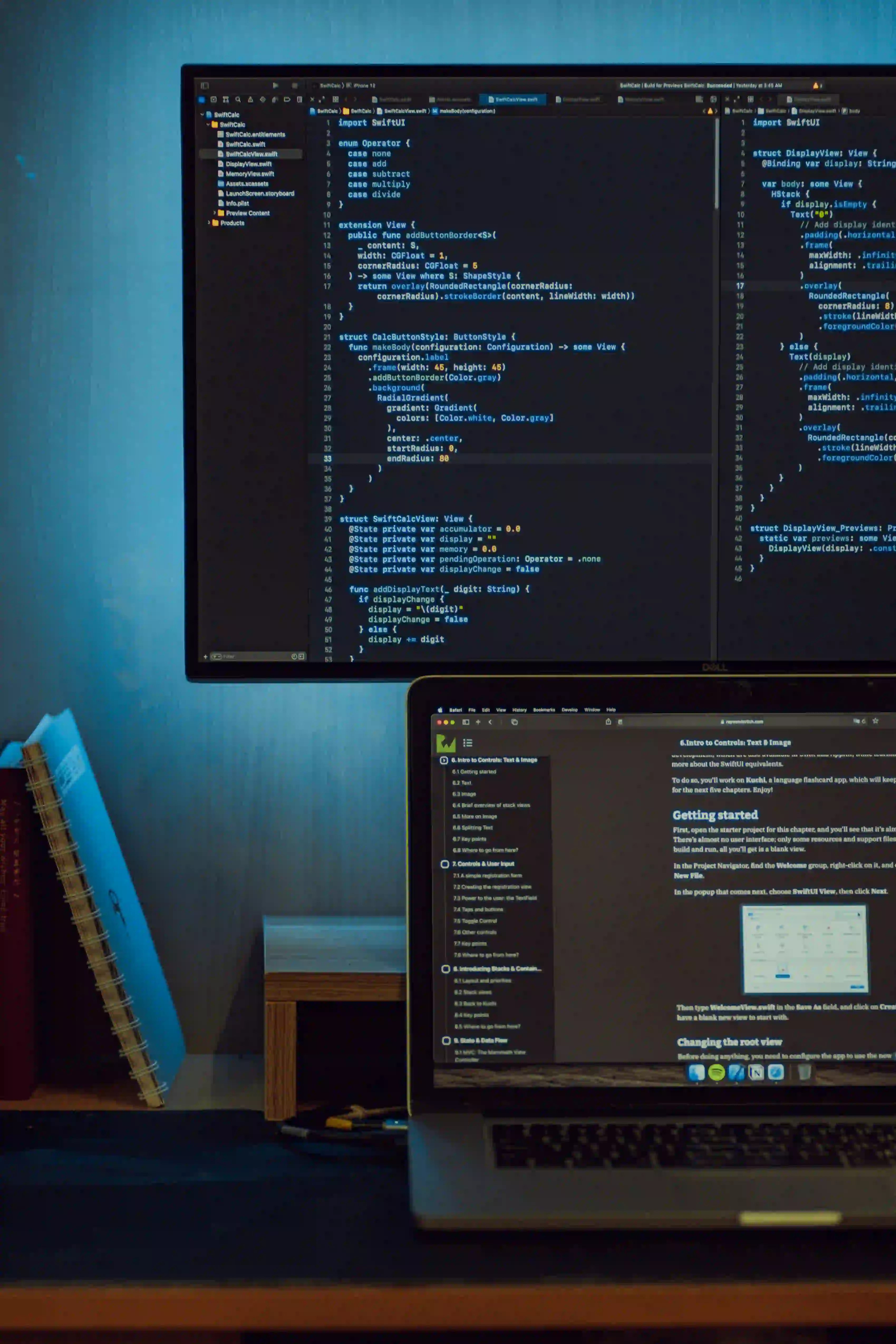
Top 5 Microservices Questions That Need Clear Answers
Microservices architecture has transformed the way we build and manage scalable applications. However, many still find it difficult to navigate this sophisticated paradigm. In this blog post, we will delve into five common questions about microservices, providing detailed explanations and code snippets to clarify the concepts at play. This will help developers, architects, and anyone interested in microservices gain a clearer understanding of this architecture style.
What Are Microservices?
Microservices represent an architectural approach to software development where applications are structured as a collection of loosely coupled services. Each service implements a specific business capability and can be developed, deployed, and scaled independently. This is in stark contrast to the traditional monolithic approach, where the entire application is tightly intertwined.
Why Microservices?
The adoption of microservices provides several advantages:
- Scalability: Individual services can be scaled independently based on demand.
- Technology Diversity: Different services can use different technologies suited to their needs.
- Faster Time to Market: Services can be developed concurrently, enabling quicker releases.
- Resilience: The failure of one service does not necessarily impact the others.
1. How Do Microservices Communicate?
Communication is crucial in a microservices architecture. Services often interact with each other using several communication protocols. The most common ones include HTTP/REST and messaging queues.
Example Using REST
Here’s a simple example illustrating how one service can communicate with another using REST.
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.client.RestTemplate;
@RestController
public class UserService {
@GetMapping("/user-details")
public ResponseEntity<String> getUserDetails() {
// Call to another microservice (Order Service)
String orderDetails = new RestTemplate().getForObject("http://order-service/orders/123", String.class);
return ResponseEntity.ok(orderDetails);
}
}
Why This Approach?
Using RESTful APIs allows for stateless interactions between microservices. It simplifies integration and provides flexibility in terms of technology and language choices.
2. How Do You Handle Data Management?
In a microservices architecture, managing data can become complex due to the decentralized nature of the services. Each service is designed to manage its data independently. Thus, a consistent strategy for data management is crucial.
Example of a Database per Service
Each microservice can have its own database schema to avoid data coupling. Here’s a simplistic representation:
| User Service Database | Order Service Database |
|-------------------------|------------------------|
| user_id | order_id |
| user_name | user_id |
| ... | ... |
Why This Strategy?
This separation allows each microservice to evolve independently. Services can optimize their storage solutions without impacting each other. However, it also enforces strong API boundaries, necessitating careful data exchange and consistency strategies.
3. What Are the Challenges of Microservices?
While microservices offer numerous benefits, several challenges must be addressed:
- Service Coordination: Managing multiple services can become complex.
- Data Consistency: Ensuring data integrity across services can be challenging.
- Deployment Overhead: More services mean more deployment and maintenance overhead.
How to Tackle These Challenges?
Utilizing containerization technologies like Docker can help streamline deployment processes. Furthermore, implementing observability tools such as Prometheus or Grafana can assist in monitoring and managing microservices more effectively.
For more on deployment strategies, check out this resource on microservices deployment.
4. What Tools and Frameworks Should You Use?
Choosing the right tools and frameworks can significantly impact your success in implementing a microservices architecture.
Popular Choices Include:
- Spring Boot: Simplifies building microservices with production-ready features.
- Docker: Provides containerization for easy deployment.
- Kubernetes: Manages clusters of containers, automating deployment, scaling, and operations.
Example: Setting Up a Simple Microservice with Spring Boot
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Why These Choices?
Spring Boot is widely embraced for its convention over configuration, making it ideal for quickly developing microservices. Docker and Kubernetes, on the other hand, enhance deployment efficiency and scalability, allowing teams to focus on development rather than operational concerns.
5. How Do You Ensure Security in Microservices?
Security remains one of the top priorities in any software development process. In a microservices architecture, it is essential to consider multiple security aspects, including:
- Authentication and Authorization: Use token-based authentication (like JWT) to secure API endpoints.
- Data Protection: Implement encryption for sensitive data in transit and at rest.
- Network Security: Secure communication between services using encrypted channels like HTTPS or TLS.
Example: Using Spring Security with JWT
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated();
}
}
Why Security Matters?
Microservices often communicate over networks, making them vulnerable to various attacks. By establishing strong security practices, developers can ensure that services are safeguarded against unauthorized access and data breaches.
Key Takeaways
Navigating the complexities of microservices doesn't have to be daunting. By understanding communication patterns, data management strategies, challenges, tools, and security requirements, you can establish a well-structured microservices architecture that meets your application's needs.
If you want to dive deeper into microservices, you may want to explore Microservices Patterns for comprehensive insights and best practices.
In the rapidly evolving landscape of software development, staying updated on microservices is essential in making informed decisions. Embrace this architectural style and leverage its advantages to enhance your applications. Happy coding!