Maximizing Efficiency: Tackling Non-Blocking Stream Latency
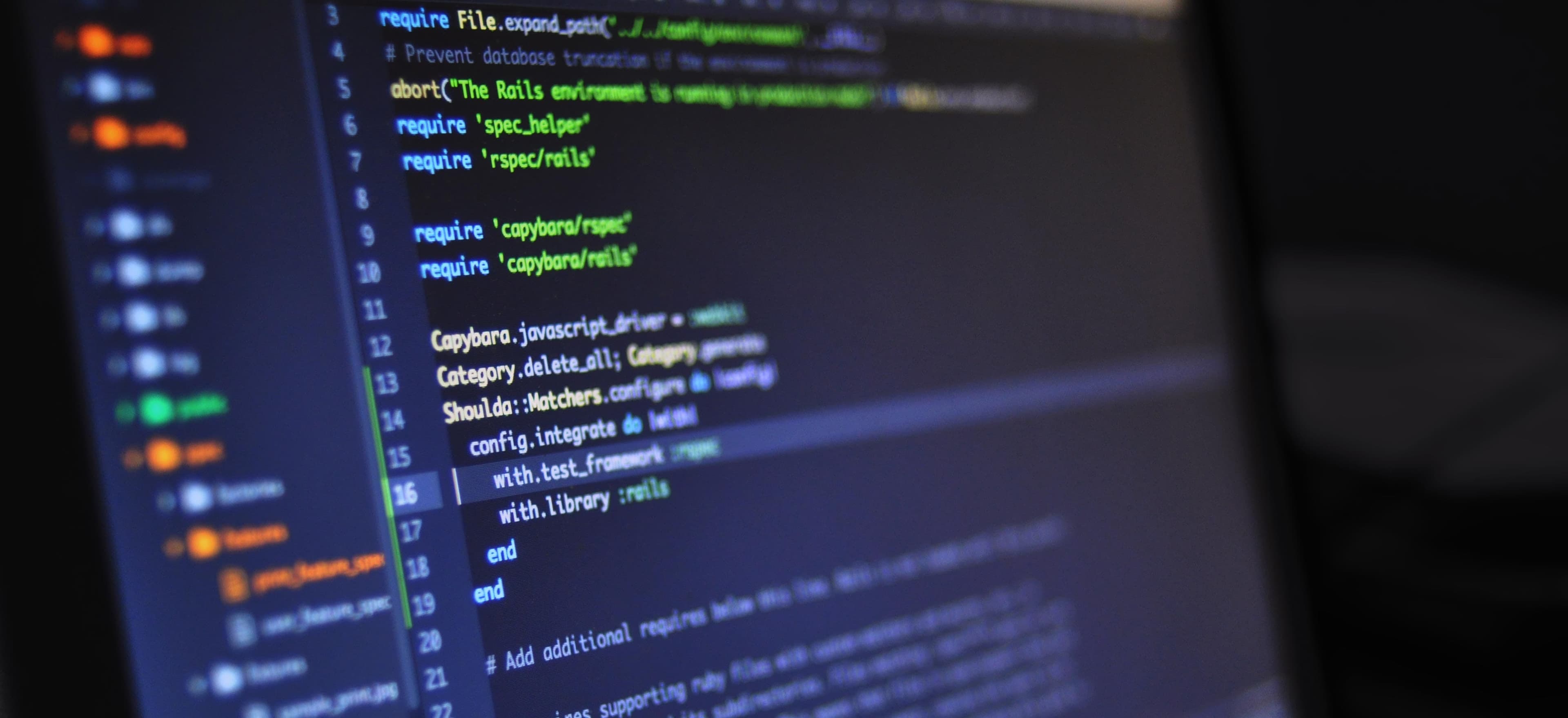
- Published on
Maximizing Efficiency: Tackling Non-Blocking Stream Latency in Java
In the modern landscape of software development, especially with Java, efficiency and performance are more important than ever. One area that poses a significant challenge is managing latency in non-blocking streams. This blog post will explore the intricacies of non-blocking streams in Java, how they operate, and strategies to minimize latency effectively.
What Are Non-Blocking Streams?
Non-blocking streams allow your application to perform I/O operations without halting the execution of your code. This means that while one stream is waiting for data to be read or written, your application can continue executing other tasks. This attribute makes them ideal for applications requiring high performance and responsiveness, such as web servers, data processing applications, and real-time systems.
Java’s java.nio
package contains powerful tools for working with non-blocking I/O, particularly through the use of channels and buffers.
Understanding Latency
Latency, in the context of stream processing, refers to the time delay between the initiation of an action and its completion. Low latency is crucial for real-time applications. High latency can lead to unresponsive behavior and a sub-par user experience.
Here are some factors that contribute to latency in non-blocking streams:
- Network Delays: The time taken for packets to traverse the network.
- I/O Contention: When multiple processes attempt to access the same resources concurrently.
- Buffer Management: Inefficient management of input/output buffers can lead to increased wait times.
To build a robust application, we must address these latency issues proactively.
Key Strategies for Reducing Latency
1. Optimize Buffer Size
The choice of buffer size can significantly influence latency. If a buffer is too small, it will lead to more frequent I/O operations, causing overhead. Conversely, excessively large buffers can cause delays in processing data.
Example:
import java.nio.ByteBuffer;
public class BufferOptimization {
public static void main(String[] args) {
// Optimal buffer size
int bufferSize = 1024; // 1KB
ByteBuffer buffer = ByteBuffer.allocate(bufferSize);
// Comment: A balance between small and large buffers promotes efficiency.
}
}
In this example, we allocate a buffer of an optimal size, aiming for a balance that minimizes the frequency of I/O operations while ensuring timely data processing.
2. Use Asynchronous I/O
Java provides asynchronous I/O operations through the AsynchronousChannel
interface, enabling your application to continue its execution while waiting for I/O operations to complete. Using this can drastically reduce the perceived latency in applications.
Example:
import java.nio.channels.AsynchronousFileChannel;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
import java.nio.ByteBuffer;
import java.nio.channels.CompletionHandler;
public class AsyncIOExample {
public static void main(String[] args) throws Exception {
AsynchronousFileChannel channel = AsynchronousFileChannel.open(
Paths.get("example.txt"), StandardOpenOption.READ
);
ByteBuffer buffer = ByteBuffer.allocate(1024);
channel.read(buffer, 0, null, new CompletionHandler<Integer, Void>() {
@Override
public void completed(Integer result, Void attachment) {
System.out.println("Read " + result + " bytes from the file.");
}
@Override
public void failed(Throwable exc, Void attachment) {
System.err.println("Failed to read the file: " + exc.getMessage());
}
});
// Comment: This call returns immediately, and we handle the result asynchronously.
}
}
In this code, the read
operation does not block the main thread. Instead, it continues execution and processes the read completion asynchronously. This enhances responsiveness and minimizes latency.
3. Minimize Context Switching
Non-blocking I/O operations may lead to numerous context switches, particularly in multithreaded applications. To mitigate this, try to minimize the number of threads that compete for resources and ensure efficient management of thread pools.
Example:
import java.nio.channels.AsynchronousChannelGroup;
import java.util.concurrent.Executors;
public class ThreadPoolingExample {
public static void main(String[] args) throws Exception {
AsynchronousChannelGroup group = AsynchronousChannelGroup.withCachedThreadPool(
Executors.newFixedThreadPool(4), 0
);
// Now, use this group for your asynchronous operations.
// Comment: A fixed thread pool can reduce context switching overhead.
}
}
Using a dedicated thread pool for asynchronous operations allows for more predictable performance and reduced context switching, leading to lower latency overall.
4. Use the Right Data Structures
Choosing the appropriate data structures can significantly influence the efficiency of data processing. For instance, using concurrent collections can help avoid bottlenecks when multiple threads operate on shared data.
Example:
import java.util.concurrent.ConcurrentLinkedQueue;
public class DataStructureExample {
public static void main(String[] args) {
ConcurrentLinkedQueue<String> queue = new ConcurrentLinkedQueue<>();
// Adding elements to the queue
queue.add("Data1");
queue.add("Data2");
// Comment: Using concurrent collections allows safe concurrent access.
}
}
The ConcurrentLinkedQueue
offers a thread-safe implementation that allows multiple threads to enqueue and dequeue items without explicit locking. This can lead to diminished access latency when processing streams of data.
5. Implement Back-Pressure Mechanisms
In high-throughput systems, data can come in faster than it can be processed. Implementing back-pressure mechanisms can help manage the flow of data, allowing systems to signal when they are overloaded. This can prevent bottlenecks that contribute to latency.
Example:
import java.util.concurrent.ArrayBlockingQueue;
public class BackPressureExample {
public static void main(String[] args) throws InterruptedException {
ArrayBlockingQueue<String> queue = new ArrayBlockingQueue<>(5);
// Simulate data producer and consumer
// Comment: Use bounded queues to manage back-pressure effectively.
}
}
Using an ArrayBlockingQueue
helps maintain a limit on how many items can be queued. When the queue is full, producers are blocked, which mitigates the risk of overwhelming the consumer and ultimately enhances responsiveness.
Final Thoughts
Tackling non-blocking stream latency in Java requires a careful approach that includes optimizing buffer sizes, embracing asynchronous I/O, minimizing context switching, using appropriate data structures, and implementing back-pressure mechanisms.
For more in-depth understanding, consider exploring Java's NIO (New Input/Output) package documentation and how asynchronous channels can be integrated into your projects for superior performance and lower latency.
By maintaining focus on efficiency and performance, you can ensure that your Java applications are not only responsive but also robust and reliable in handling real-time data. This approach will ultimately contribute to an exceptional user experience and a more effective application.