Resolving URL Parameter Issues in JBoss: A Practical Guide
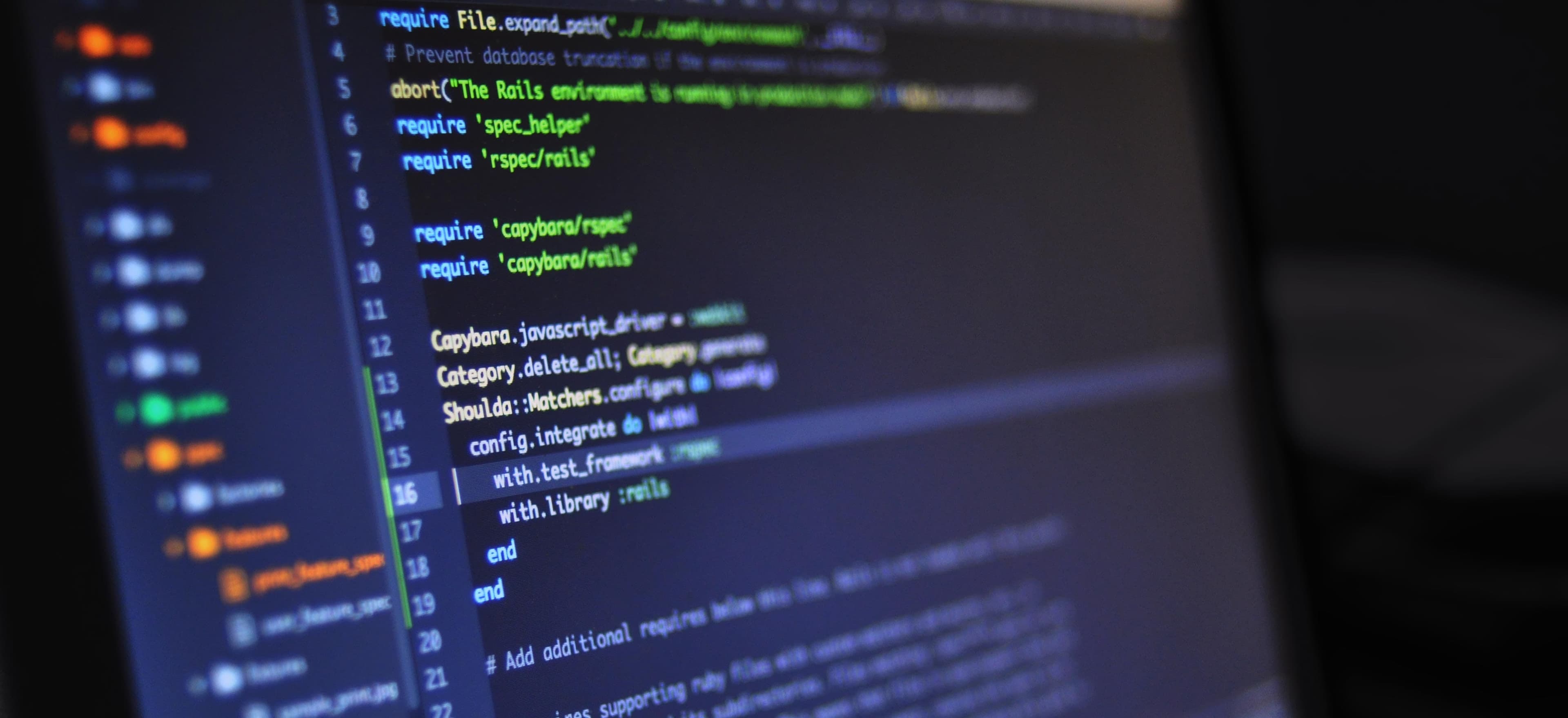
- Published on
Resolving URL Parameter Issues in JBoss: A Practical Guide
In the world of web applications, URL parameters often play crucial roles in data transmission and user navigation. However, using a Java server, such as JBoss, can sometimes complicate how these parameters are handled, leading to unexpected behavior. This blog post delves into resolving URL parameter issues in JBoss, providing practical examples and best practices for developers.
Understanding URL Parameters
URL parameters are key-value pairs appended to a URL following a question mark (?). They allow developers to communicate additional information to the server via GET requests. For example:
http://example.com/products?category=books&sort=asc
In this case, category
and sort
are URL parameters.
The Importance of URL Parameters
- User Experience: They help create dynamic and personalized experiences for users.
- Data Retrieval: They enable the retrieval of specific resources without the need for multiple endpoints.
- SEO Benefits: Well-structured URLs can enhance a site’s visibility in search engines.
Common URL Parameter Issues in JBoss
Before diving into solutions, let's look at some common issues developers face:
- Improper Encoding: Special characters in parameters may not be processed correctly.
- Parameter Loss: Occasionally, parameters may be missing entirely when passed to servlets.
- Data Type Mismatch: Using the wrong data type in URL parameters can lead to runtime errors.
Setting Up Your JBoss Server
Before we investigate specific cases, we'll ensure our JBoss server is well-configured. Follow these steps to set up your environment:
-
Download and Install JBoss: Visit the JBoss website to download the latest release.
-
Configure Your Application: Create a simple web application using Maven or Gradle.
-
Deploy to JBoss: Package and deploy your application to the JBoss server.
Example Application
Let's create a simple example that showcases how to correctly handle URL parameters in a JBoss application.
Step 1: Set Up a Servlet
In your web application, create a servlet that will handle incoming requests containing URL parameters. Here's a basic implementation:
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet("/products")
public class ProductServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String category = request.getParameter("category");
String sort = request.getParameter("sort");
if (category == null || category.isEmpty()) {
response.getWriter().write("Category parameter is missing");
return;
}
// Business logic to retrieve products based on category and sort
response.getWriter().write("Products in category: " + category + ", sorted by: " + sort);
}
}
Commentary on the Servlet Code
- Request Handling: The
doGet()
method captures incoming GET requests. - Parameter Retrieval: The
getParameter()
method fetches the parameters from the request. If the desired parameter is missing, we handle the issue gracefully by returning an informative message.
Step 2: Proper URL Encoding
URL encoding ensures that special characters are correctly transmitted. For instance, if your parameter could contain spaces or symbols:
String encodedCategory = URLEncoder.encode(category, "UTF-8");
This helps avoid issues often encountered with spaces (replaced by %20
) or other special characters.
Step 3: Handling Data Types
Suppose we want to retrieve a numeric parameter, like a product ID. Here’s how to safely manage data types:
String idParam = request.getParameter("id");
try {
int productId = Integer.parseInt(idParam);
// Use productId in business logic...
} catch (NumberFormatException e) {
response.getWriter().write("Invalid product ID format.");
}
In this code, we wrap our parsing logic in a try-catch block to gracefully handle format errors.
Debugging URL Parameter Issues
When dealing with URL parameters, you may encounter several frustrating scenarios. Let's go over some debugging strategies.
1. Logging
Make extensive use of Java’s logging facilities to trace parameters:
import java.util.logging.Logger;
private static final Logger logger = Logger.getLogger(ProductServlet.class.getName());
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String category = request.getParameter("category");
logger.info("Received category: " + category);
// Further processing...
}
This enables you to monitor incoming parameters in the server logs.
2. Testing Endpoints
For efficient debugging, consider utilizing tools like Postman or cURL to simulate requests with various URL parameters:
curl "http://localhost:8080/yourapp/products?category=books&sort=asc"
3. Cross-Origin Requests (CORS)
If your application is making requests across different origins, troubleshoot CORS issues. Ensure your server allows cross-origin requests, especially for AJAX calls. To enable CORS, you can set headers in your servlet:
response.setHeader("Access-Control-Allow-Origin", "*");
Best Practices for Managing URL Parameters
Adhering to best practices can mitigate many common issues associated with URL parameters in JBoss.
1. Validate Parameters
Always validate incoming parameters to ensure they're in the expected format.
2. Use URL Encoding
Implement URL encoding whenever special characters are involved; this prevents misinterpretation.
3. Implement Error Handling
Enhance user experience by providing informative error messages rather than generic ones.
4. Unit Testing
Write comprehensive unit tests for your servlets to ensure they handle parameters correctly. Use frameworks like JUnit along with Mockito to stub requests and responses.
@Test
public void testMissingCategory() throws Exception {
// Mock HttpServletRequest and HttpServletResponse
// Validate response output
}
Final Considerations
Handling URL parameters in a JBoss environment can be straightforward if you adhere to best practices and utilize proper debugging techniques. By implementing the concepts outlined in this guide, you can effectively manage URL parameters, resulting in improved functionality and user experience.
For further reading on JBoss and servlets, consider visiting:
By following this guide, you will not only resolve parameter issues but also enhance the robustness of your JBoss applications. Happy coding!