Mastering Lambda Expressions: Common Pitfalls in Java 8
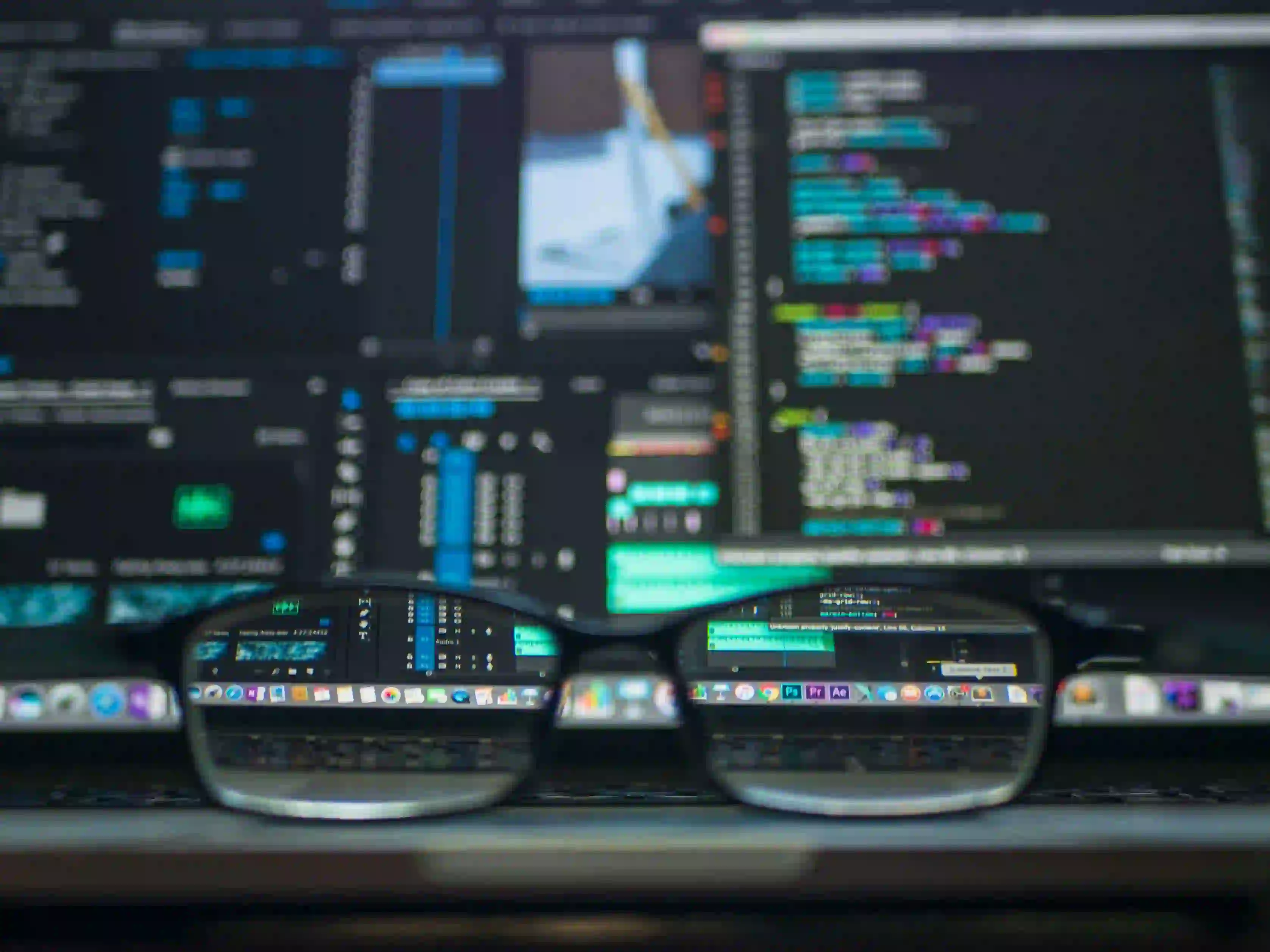
Mastering Lambda Expressions: Common Pitfalls in Java 8
Java 8 introduced a significant change in the way we program with the introduction of lambda expressions. This feature provides a clear and concise way to express instances of single-method interfaces (functional interfaces). As strong advocates of effective programming, we need to not only grasp the concept but also identify and avoid common pitfalls. Whether you are a seasoned developer or a complete novice, understanding these pitfalls will deepen your mastery of Java.
What are Lambda Expressions?
Before we dive into the common pitfalls, let's clarify what lambda expressions are. They are essentially anonymous functions that represent a block of code, which you can pass around as an argument.
Syntax
The basic syntax of a lambda expression is as follows:
(parameters) -> expression
Or, if it consists of multiple statements, use curly brackets:
(parameters) -> {
// statements
}
For example, here's a simple lambda expression that takes two integers and returns their sum:
(int a, int b) -> a + b
Common Pitfalls to Avoid
Even though lambda expressions simplify code, there are several pitfalls that developers commonly encounter:
1. Scope and Effectively Final Variables
One of the most common issues with lambda expressions is understanding variable scope and the concept of effectively final variables. Unlike inner classes, lambda expressions can only use variables from their enclosing scope if those variables are either final or effectively final.
Example
int number = 10;
Runnable r = () -> System.out.println(number); // This works
number = 20; // Compilation error: local variables referenced from a lambda must be final or effectively final.
Why it matters: Preventing modification within a lambda expression prevents unintended side effects.
2. Using the Wrong Functional Interface
Using a functional interface that does not match the method signature of your lambda expression can lead to verbose compile-time errors. For every lambda you write, there needs to be a corresponding functional interface.
Example
Function<Integer, String> intToString = (Integer i) -> String.valueOf(i); // Correct
BiFunction<Integer, Integer, Integer> addition = (a, b) -> a + b; // Wrong! BiFunction expects two parameters.
Why it matters: Picking the wrong functional interface leads to compatibility issues, which could have been avoided by careful selection.
3. Overusing Lambda Expressions
In some cases, programmers might blindly use lambda expressions for every situation, which can lead to code that is less readable. Rarely is it necessary to convert everything into a lambda expression.
Example
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name)); // Obvious, but here a simple forEach can be clearer
Instead, consider:
for (String name : names) {
System.out.println(name);
}
Why it matters: Readable code is just as important as concise code, driving maintainability.
4. Not Handling Exceptions Properly
Lambda expressions ignore checked exceptions unless handled explicitly. Ignoring exception handling within a lambda can lead to unhandled exceptions during runtime.
Example
Let’s say we are reading a file. If you use a lambda within a stream, you need to handle potential IO exceptions.
import java.util.List;
import java.nio.file.Files;
import java.nio.file.Paths;
List<String> lines = Files.readAllLines(Paths.get("file.txt")); // Throws an IOException
lines.forEach(line -> {
// Experience an IOException if not handled
System.out.println(line);
});
Why it matters: Always ensure exceptions are appropriately managed to prevent application crashes.
5. Performance Considerations
Though lambda expressions are elegant, they sometimes introduce extra overhead. Each lambda expression can implement the functional interface through invocation, which could lead to performance degradation in performance-critical applications.
Example
List<String> list = Arrays.asList("one", "two", "three", "four");
list.stream()
.filter(s -> s.length() > 3)
.forEach(System.out::println); // Might have slight performance overhead in certain contexts
Why it matters: Performance implications should be considered in systems where every millisecond counts.
Best Practices for Using Lambda Expressions
Now that we've covered some of the pitfalls, let's look at a few best practices to follow when using lambda expressions.
1. Use Descriptive Parameter Names
Make sure your lambda arguments are easy to understand. This helps in maintaining readability and clarity.
List<String> items = Arrays.asList("apple", "banana", "orange");
items.forEach(item -> System.out.println(item)); // Clear and descriptive
2. Leverage Method References
Where applicable, consider using method references for cleaner code.
Example
Instead of:
items.forEach(item -> print(item));
You can instead refactor it to:
items.forEach(this::print);
3. Keep It Simple
If the logic inside your lambda expression starts to grow complex, consider using a named method instead.
Example
items.forEach(item -> {
if (item.startsWith("a")) {
System.out.println(item);
}
});
Refactor to:
items.forEach(this::printIfStartsWithA);
4. Use Streams Wisely
Java Streams allow you to easily manipulate collections. However, use the power of streams but understand when it makes sense to leverage them effectively.
A Final Look
Mastering lambda expressions in Java 8 opens up a world of possibilities for cleaner and more efficient code. By being aware of common pitfalls such as scope issues, improper interface usage, overutilization, exception handling, and performance considerations, developers can significantly enhance their coding practices.
For further reading, I encourage checking out:
- Oracle's Official Java Lambda Documentation - A comprehensive guide to understanding lambdas.
- Baeldung's Guide to Java Streams - A deep dive into the usage of streams in Java.
With these insights, grasp the power of lambda expressions and continue to improve your Java programming skills. Happy coding!