Overcoming Performance Issues in Java's Array Sorting
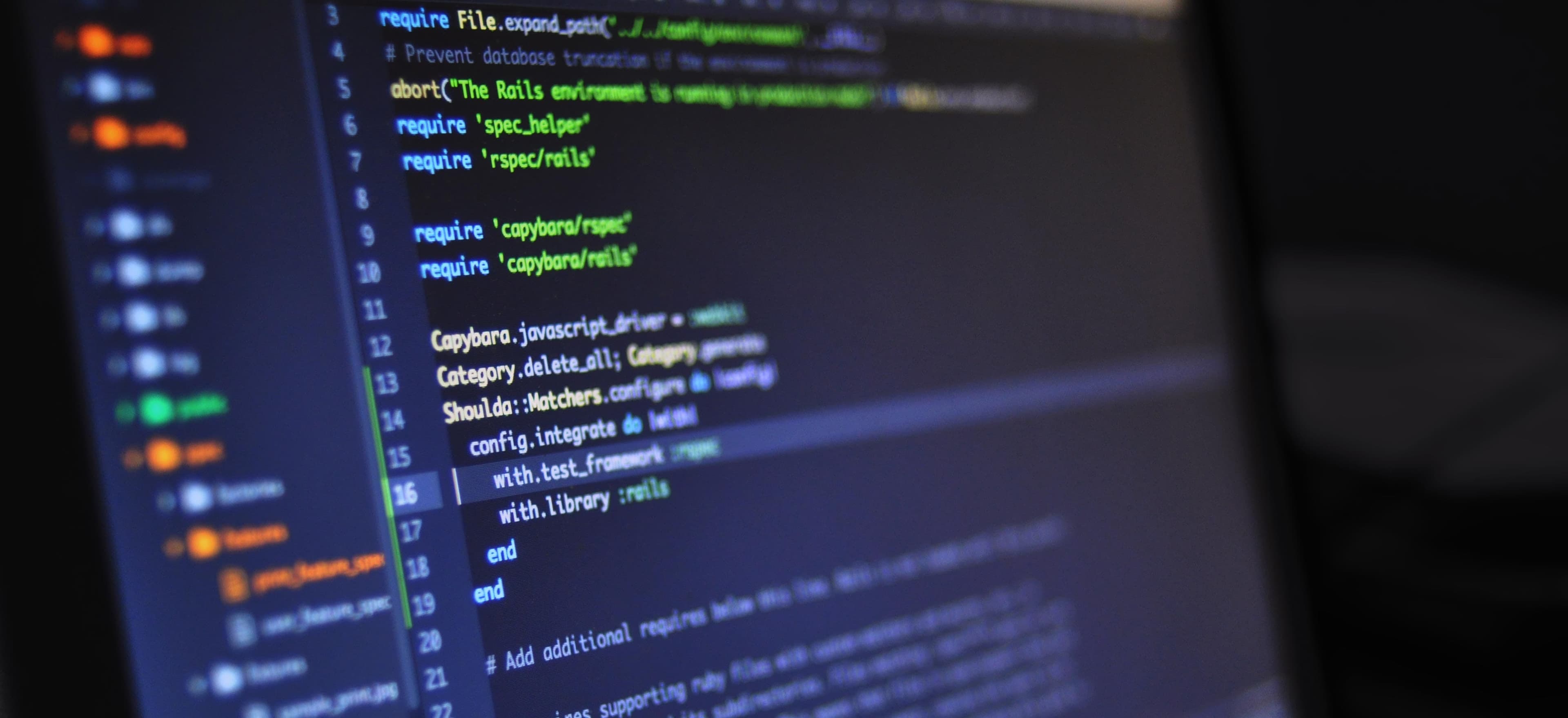
- Published on
Overcoming Performance Issues in Java's Array Sorting
Sorting arrays is a fundamental operation in computer science and programming. Efficient array sorting can significantly affect the performance of applications, particularly those that handle large datasets. Java provides several built-in mechanisms to sort arrays, yet performance issues can arise, especially with larger datasets. In this blog post, we’ll delve into the common performance bottlenecks associated with array sorting in Java and explore ways to optimize sorting.
Understanding the Basics of Array Sorting in Java
In Java, there are various ways to sort arrays. The most common methods include:
- Using Arrays.sort(): A built-in method that simplifies sorting.
- Implementing Custom Sorting Algorithms: Such as Quick Sort, Merge Sort, Bubble Sort, etc.
The built-in Arrays.sort()
method typically utilizes a dual-pivot quicksort algorithm for primitives, which is efficient on average but may suffer in specific cases.
Example of Using Arrays.sort()
import java.util.Arrays;
public class SortExample {
public static void main(String[] args) {
int[] numbers = {5, 3, 8, 1, 4, 7};
Arrays.sort(numbers);
System.out.println("Sorted Array: " + Arrays.toString(numbers));
}
}
Why Use Arrays.sort()
?
- Simplicity: The method is straightforward and easy to use.
- Optimized Performance: For most practical purposes, it offers optimal performance without requiring the user to implement complex algorithms.
- Built-in Handling: It handles edge cases such as null references and empty arrays.
However, as datasets grow larger and the sorting needs become more complex, drawbacks may emerge.
Common Performance Issues with Array Sorting
1. Time Complexity
The average time complexity for the built-in Arrays.sort()
is O(n log n). However, in worst-case scenarios, especially with poorly arranged data, the complexity can degrade to O(n^2). Custom implementations can introduce their own complexities.
2. Memory Overhead
Some sorting algorithms, like Merge Sort, require additional memory proportional to the size of the array. This can be particularly concerning in environments with limited memory resources.
3. In-place vs. Out-of-place Sorting
Sorting algorithms can be categorized as in-place (which does not require extra space) or out-of-place (which does). Using out-of-place sorting can lead to significant allocations that can degrade performance.
4. Handling of Specific Data Types
Sorting may vary in performance depending on the data types being handled. For example, sorting large arrays of objects may involve overhead due to comparative operations.
Performance Optimization Techniques
1. Choose the Right Sorting Algorithm
Understanding the data and its characteristics can facilitate the selection of the most appropriate sorting algorithm. For instance, if you know that the dataset is nearly sorted, an algorithm like Insertion Sort, which has a time complexity of O(n) in best-case scenarios, could be the optimal choice.
Custom Implementation Example
Here’s an example of implementing an optimized insertion sort:
public class InsertionSort {
public static void optimizedInsertionSort(int[] arr) {
for (int i = 1; i < arr.length; i++) {
int key = arr[i];
int j = i - 1;
// Move elements of arr[0..i-1], that are greater than key,
// to one position ahead of their current position
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j = j - 1;
}
arr[j + 1] = key;
}
}
}
Commentary on Insertion Sort
- Efficiency: Insertion Sort is efficient for small or nearly sorted data.
- In-place: It requires no additional memory, thus combating memory overhead issues.
2. Leveraging Parallel Sorting
For large datasets, leveraging parallel processing can result in significant performance improvements. Java 8 introduced the Arrays.parallelSort()
method, which uses multiple threads to carry out the sorting operation.
Parallel Sort Example
import java.util.Arrays;
public class ParallelSortExample {
public static void main(String[] args) {
int[] numbers = {5, 3, 8, 1, 4, 7, 9, 2, 6, 0};
Arrays.parallelSort(numbers);
System.out.println("Sorted Array (Parallel): " + Arrays.toString(numbers));
}
}
Why Use Parallel Sorting?
- Efficiency in Multithreading: It divides the array and sorts chunks in parallel, reducing overall sorting time.
- Utilization of Modern Hardware: Modern machines have multiple cores that can be utilized for simultaneous operations.
3. Custom Comparators for Stability
When sorting objects, using custom comparators can maintain order and improve performance by managing comparisons more effectively.
Custom Comparator Example
import java.util.Arrays;
import java.util.Comparator;
class Employee {
String name;
int age;
Employee(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Employee{name='" + name + "', age=" + age + '}';
}
}
public class ComparatorExample {
public static void main(String[] args) {
Employee[] employees = {
new Employee("Alice", 30),
new Employee("Bob", 24),
new Employee("Charlie", 28)
};
Arrays.sort(employees, Comparator.comparingInt(emp -> emp.age));
System.out.println("Sorted Employees by Age: " + Arrays.toString(employees));
}
}
Commentary on Custom Comparators
- Flexibility: Custom comparators permit sorting based on multiple attributes.
- Stability: They help keep the initial order intact for equal elements.
A Final Look
Sorting arrays efficiently in Java necessitates careful consideration of the algorithms and techniques used. Understanding the underlying mechanics allows developers to address performance issues like time complexity, memory overhead, and the specifics of data types effectively.
By leveraging built-in methods where appropriate, utilizing parallel processing capabilities, and selecting tailored algorithms based on contextual needs, you can optimize sorting performance considerably.
For further reading on Java sorting algorithms and their performance characteristics, check out the official Java Documentation for Arrays and explore detailed explanations of various Sorting Algorithms.
Optimized array sorting can make your Java applications more responsive and capable of handling large datasets efficiently. Happy coding!
Checkout our other articles