Struggling with Timing Issues in Java EE RESTful Services?
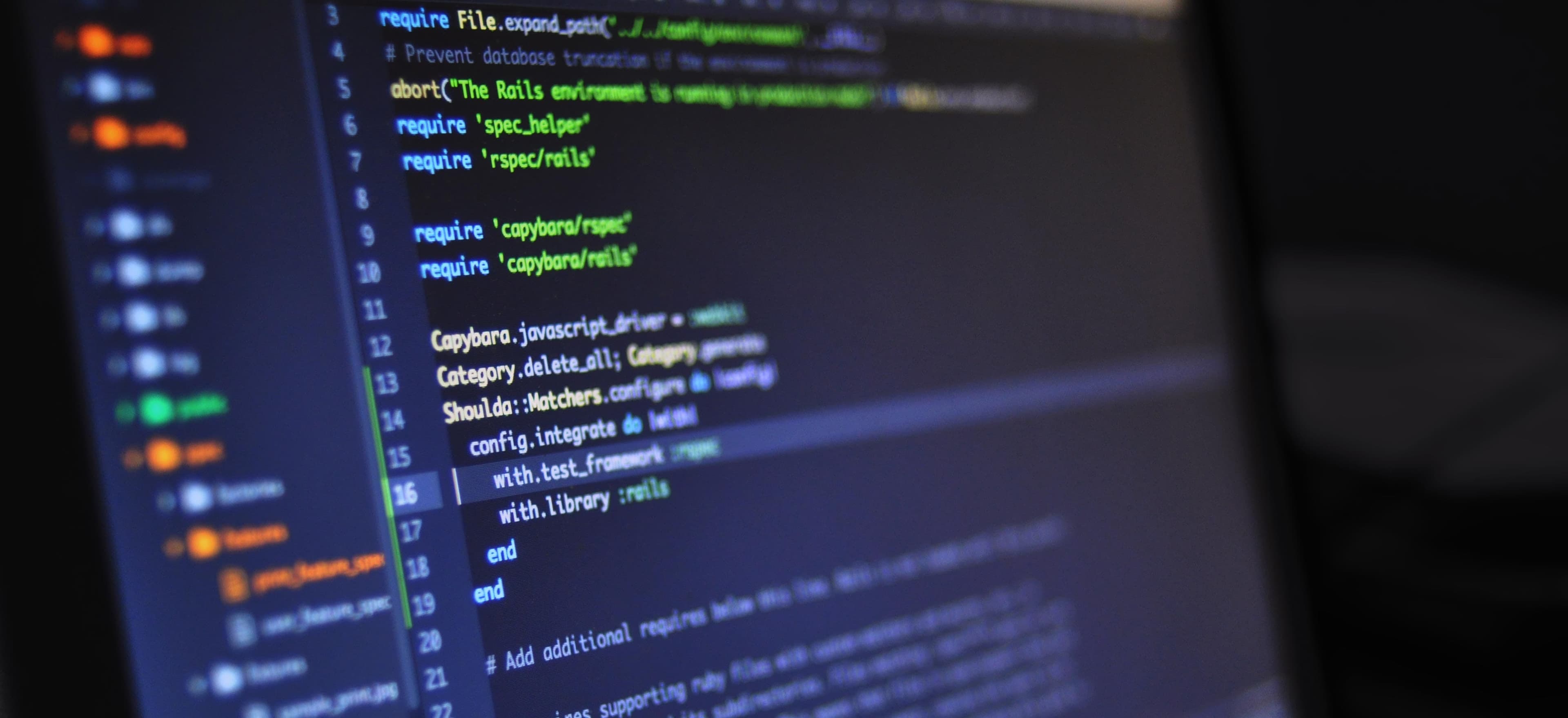
- Published on
Struggling with Timing Issues in Java EE RESTful Services?
When developing Java EE RESTful services, developers often encounter various challenges. One frequently overlooked aspect is the timing issues that can arise, impacting the response time, performance, and ultimately user experience. This blog post aims to shed light on timing issues in Java EE RESTful services, illustrates common pitfalls, and offers solutions.
Understanding RESTful Services Timing Issues
REST (Representational State Transfer) is an architectural style widely used for building web services. Java EE provides robust support for building RESTful services through JAX-RS (Java API for RESTful Web Services). However, several factors can lead to timing problems, including:
- Database Latency: Often, RESTful services interact with databases, and any delay in data retrieval can affect the overall timing.
- Network Latency: Depending on the distance between the client and server, network latency can introduce significant delays.
- Synchronization Issues: If multiple instances of services compete for shared resources, it may lead to locks and delays.
- Unoptimized Code: Poorly structured code can introduce unexpected latency.
The Importance of Measurement
Before jumping into solutions, it's vital to understand how to measure timing issues. Numerous tools can help you analyze performance:
- Java Flight Recorder (JFR) - for data capture and analysis.
- VisualVM - to monitor the application's performance in real-time.
- New Relic or Dynatrace - for deep application monitoring.
It’s essential to establish metrics to quantify performance issues, enabling targeted improvements.
Common Timing Issues and Solutions
1. Database Latency
Database latency can significantly hinder the performance of a RESTful service. A typical example could be the inefficient reading of large datasets.
Example of Inefficient Query:
public List<User> getAllUsers() {
EntityManager em = entityManagerFactory.createEntityManager();
String query = "SELECT u FROM User u"; // Fetching all users
return em.createQuery(query, User.class).getResultList();
}
Why is it inefficient?
Fetching all users at once can become a performance bottleneck, particularly when the database contains hundreds of thousands of records.
Solution: Optimize with pagination.
public List<User> getUsers(int page, int size) {
EntityManager em = entityManagerFactory.createEntityManager();
String query = "SELECT u FROM User u";
return em.createQuery(query, User.class)
.setFirstResult(page * size)
.setMaxResults(size)
.getResultList();
}
The 'why' aspect: Pagination allows the retrieval of user data in manageable chunks, thus reducing memory consumption and improving response time.
2. Network Latency
When dealing with RESTful services, the travel time between client and server cannot be ignored. Network latency can become pronounced if the server handles multiple requests.
Example of a Blocking Request:
Suppose you’re making synchronous REST calls to external services:
public Response getUserDetails(String userId) {
Response response = externalServiceClient.callUserService(userId);
return response;
}
The problem: Synchronous calls can lead to high overall response times if external services take time to respond.
Solution: Use Asynchronous Calls.
Java EE provides an easy way to create non-blocking calls using javax.ws.rs.core.Response
:
@Asynchronous
public Future<Response> getUserDetailsAsync(String userId) {
// Simulating a network call with CompletableFuture
return CompletableFuture.supplyAsync(() -> {
Response response = externalServiceClient.callUserService(userId);
return response;
});
}
The 'why' aspect: This code allows the server to handle other requests while waiting for the external service to respond, improving overall responsiveness.
3. Synchronization Issues
When dealing with shared resources, it's crucial to manage synchronization properly. Improper locking mechanisms can lead to latency increases.
Example of Poorly Managed Synchronization:
synchronized public void updateConfiguration(Configuration config) {
// logic to update configuration
}
The issue: Too many threads waiting for a synchronized lock will slow down processing.
Solution: Use Concurrent Collections or Lock-Free Techniques.
Utilizing java.util.concurrent
collections can often eliminate the need for explicit locks.
private ConcurrentHashMap<String, Configuration> configCache = new ConcurrentHashMap<>();
public void updateConfiguration(Configuration config) {
configCache.put(config.getId(), config); // Thread-safe update
}
The 'why' aspect: This eliminates synchronization bottlenecks, allowing multiple threads to access the collection concurrently without waiting for locks.
4. Unoptimized Code
Lastly, unoptimized code can be a significant source of timing issues. Everything from inefficient algorithms to resource-heavy libraries can contribute to performance delays.
Example of Inefficient Looping:
public List<String> processData(List<String> data) {
List<String> results = new ArrayList<>();
for (int i = 0; i < data.size(); i++) {
if (data.get(i).contains("important")) {
results.add(data.get(i));
}
}
return results;
}
The issue: The loop checks each element; if the list is long, this can take a long time.
Solution: Use Java Streams for Optimization.
public List<String> processData(List<String> data) {
return data.stream()
.filter(s -> s.contains("important"))
.collect(Collectors.toList());
}
The 'why' aspect: Java Streams are optimized for performance and readability. The code becomes cleaner and often faster due to underlying implementation.
To Wrap Things Up
Timing issues in Java EE RESTful services can lead to considerable performance degradation. By focusing on database optimization, using asynchronous calls, managing synchronization effectively, and refining code, developers can significantly improve the performance of their services.
Additional Resources
- Interested in diving deeper? Explore more about improving REST API performance with Spring's extensive documentation.
- For further reading on Java Concurrency, check out Oracle's Java Concurrency tutorial.
By applying the practices outlined in this article, developers can craft RESTful services that are not just functional but also meet the performance standards expected in today’s applications.
Checkout our other articles