Overcoming File Upload Challenges with GraphQL in Java
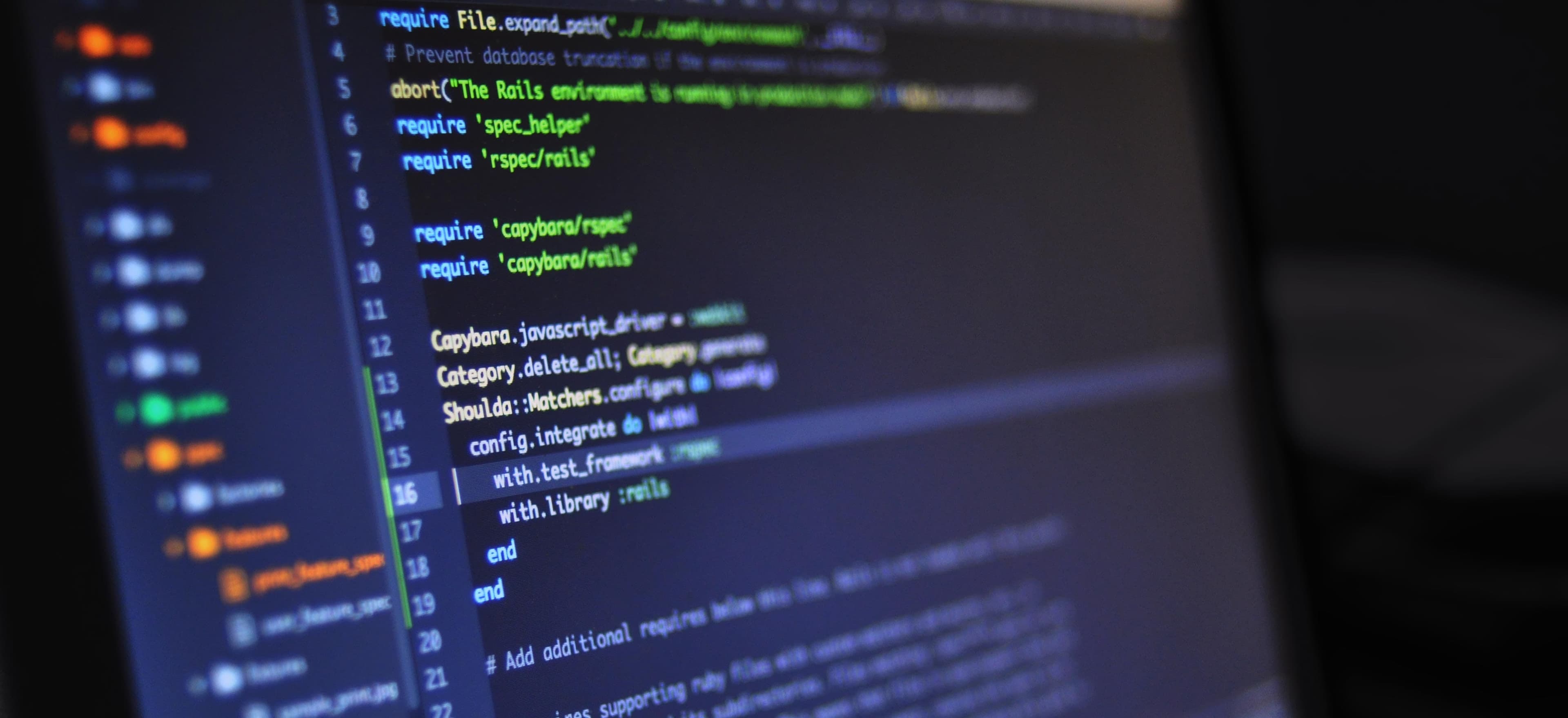
- Published on
Overcoming File Upload Challenges with GraphQL in Java
In the modern web landscape, file uploading is a common functionality, yet it remains a challenge for developers. Using GraphQL for file uploads in Java can enhance user experience while maintaining the flexibility that GraphQL offers. This blog post explores the nuances of handling file uploads via GraphQL in a Java environment and shares best practices along with code snippets.
Understanding GraphQL and File Uploads
GraphQL is a powerful query language for APIs, enabling clients to request exactly the data they need. However, file uploads are traditionally not part of the GraphQL specification. Unlike REST, where uploads are often handled via multipart requests, you need to implement a workaround in GraphQL. That said, using libraries like Apollo or graphql-upload can help streamline the process.
Key Points on File Uploads in GraphQL
-
Batch Requests: GraphQL supports batching requests, but file uploads typically require a separate handling mechanism.
-
Binary Data Handling: GraphQL is designed around text-based data exchange. Thus, uploading binary files involves encoding the data, usually in base64, when included in the request body.
-
Client Complexity: The client's responsibility increases as it must manage multipart requests and file metadata.
Dependency Setup
Before we dive into the code, make sure you have the necessary dependencies in your pom.xml
if you're using Maven:
<dependency>
<groupId>com.graphql-java-kickstart</groupId>
<artifactId>graphql-spring-boot-starter</artifactId>
<version>11.1.0</version>
</dependency>
<dependency>
<groupId>com.graphql-java-kickstart</groupId>
<artifactId>graphql-upload-spring-boot-starter</artifactId>
<version>13.0.0</version>
</dependency>
The above dependencies include support for integrating GraphQL with Spring Boot along with file upload capabilities.
Creating the GraphQL Schema
The first step is to define the GraphQL schema. Create a schema.graphqls
file in your resource folder:
scalar Upload
type Mutation {
uploadFile(file: Upload!): String!
}
Explanation
- scalar Upload: Defines a custom scalar for handling file uploads.
- uploadFile Mutation: A mutation that accepts a file of type
Upload
and returns a success message as a string.
Implementing the Upload Logic
Create a service to handle file uploads. This service will process incoming files and store them appropriately. Here is a simple implementation:
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
@Service
public class FileUploadService {
private final String uploadDir = "uploads/";
public String saveFile(MultipartFile file) throws IOException {
// Create directory if it doesn't exist
File dir = new File(uploadDir);
if (!dir.exists()) {
dir.mkdirs();
}
// Define the path for saving the file
Path path = Paths.get(uploadDir + file.getOriginalFilename());
// Save the file
Files.write(path, file.getBytes());
// Return a success message with the filename
return "File uploaded successfully: " + file.getOriginalFilename();
}
}
Code Explanation
-
File Directory Creation: The service checks if the upload directory exists and creates it if not. This step helps prevent
FileNotFoundExceptions
. -
Path Handling: The Path class is used for simplicity and safety in file operations, which is preferable over string manipulation.
-
File Writing: The
Files.write
method directly handles byte data, ensuring that your application can handle binary file types.
Setting Up the GraphQL Resolver
Create a GraphQL resolver to use the upload service you just created:
import graphql.servlet.GraphQLServlet;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.graphql.data.GraphQlSourceBuilder;
import org.springframework.graphql.execution.GraphQlSource;
import org.springframework.stereotype.Component;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.bind.annotation.RequestParam;
import graphql.execution.instrumentation.InstrumentationContext;
import graphql.servlet.GraphQLUpload;
@Component
public class FileUploadResolver {
@Autowired
private FileUploadService fileUploadService;
public String uploadFile(@RequestParam("file") GraphQLUpload upload) {
try {
// Convert GraphQLUpload to MultipartFile
MultipartFile file = upload.getFile();
return fileUploadService.saveFile(file);
} catch (IOException e) {
e.printStackTrace();
return "File upload failed: " + e.getMessage();
}
}
}
Why Use Separate Resolvers?
Separating your logic into resolvers:
- Encapsulates Business Logic: Resolvers are cleanly organized with respective services, making your codebase easier to maintain.
- Increases Readability: Logical separation aids in understanding the flow of data in your application.
Testing the File Upload Functionality
At this point, your backend can handle file uploads. You can test it using GraphQL Playground or through tools like Postman supporting GraphQL.
Example Upload Mutation
mutation {
uploadFile(file: <FileInput>) {
status
}
}
Explanation
Replace <FileInput>
with the actual input file, enabling you to test the upload functionality.
Handling Errors and Edge Cases
While your initial implementation should work for basic usages, various scenarios can introduce challenges.
- File Size Limit: You may want to limit the size of files uploaded to prevent DDoS attacks or overconsumption of storage. Configuring this in Spring Boot will help.
spring.servlet.multipart.max-file-size=10MB
spring.servlet.multipart.max-request-size=10MB
- File Type Validation: Always validate the file type based on your application's needs. You can check for accepted file extensions by expanding the
saveFile
method.
public String saveFile(MultipartFile file) throws IOException {
String contentType = file.getContentType();
if (!"image/jpeg".equalsIgnoreCase(contentType) && !"image/png".equalsIgnoreCase(contentType)) {
throw new IllegalArgumentException("Unsupported file type: " + contentType);
}
// Remaining implementation...
}
To Wrap Things Up
Using GraphQL for file uploads in Java can simplify your API design while providing robust functionality. From understanding the GraphQL schema to implementing the service layer, this guide has outlined the steps needed for a streamlined file upload process.
Embrace the unique benefits of GraphQL while adhering to best practices around file management. Always consider security, validation, and performance to enhance your application's robustness.
For further reading on GraphQL and its many facets, check out the official GraphQL documentation. Happy coding!
Checkout our other articles