Is Pool Architecture the Key to Future Scalability?
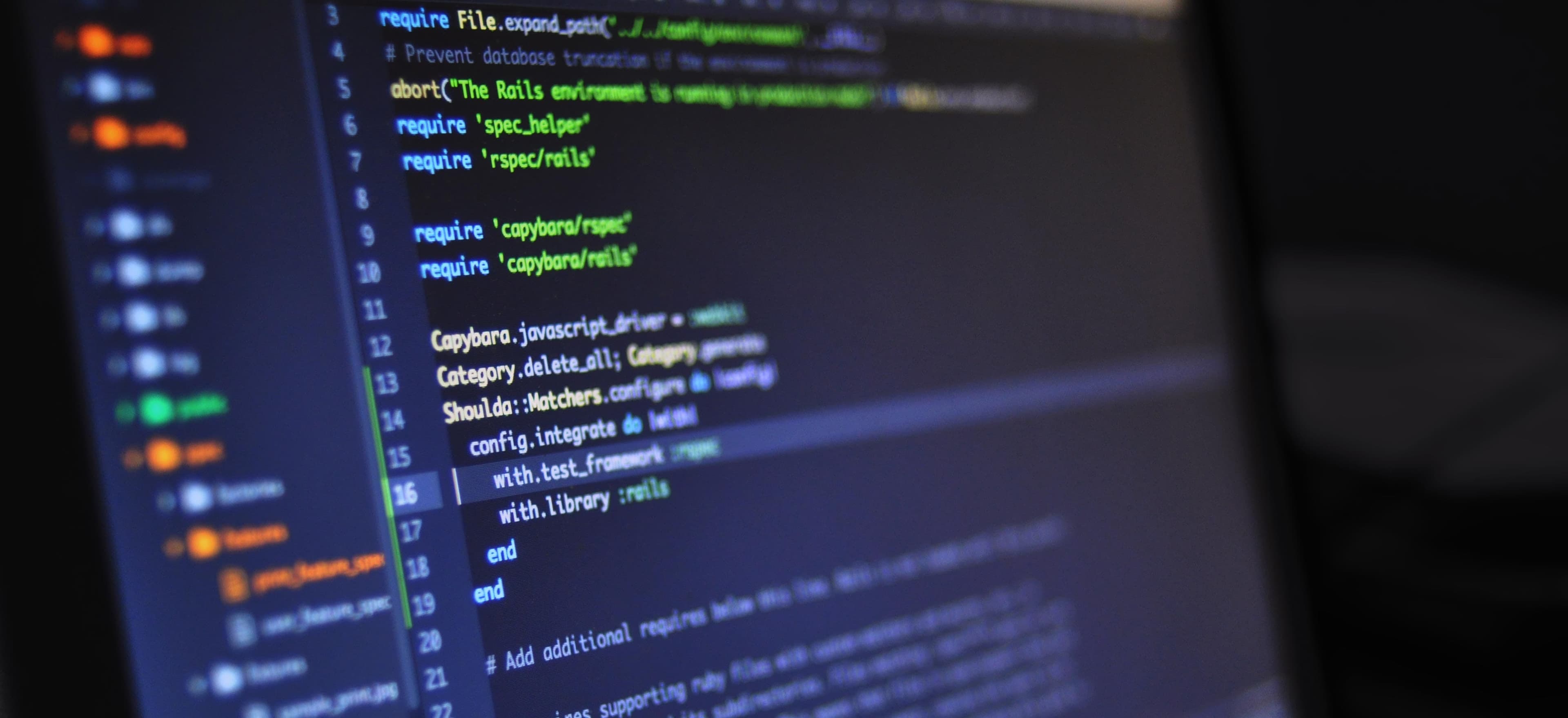
- Published on
Is Pool Architecture the Key to Future Scalability?
As software systems evolve, the quest for scalability remains at the forefront of technological advancements. Among the various architectural models, pool architecture is gaining traction as a potential solution to scalability challenges. In this blog post, we'll dive into what pool architecture is, its benefits, drawbacks, and how it can shape the future of scalable systems.
What is Pool Architecture?
Pool architecture is a design pattern that allows a group of resources, such as database connections or threads, to be created and managed efficiently as a "pool." This concept enables reusing existing resources instead of creating new ones for every request.
Key Components of Pool Architecture
- Resource Pool: A central container that holds a set of resources, ready to be utilized when needed.
- Allocator and Deallocator: Mechanisms to allocate resources from the pool when requested and to return them after usage.
- Configuration Settings: Parameters that dictate the size of the pool, timeout values, and resource limits.
Benefits of Pool Architecture
1. Resource Efficiency
Resource pooling leads to significant reductions in resource overhead. Creating new connections or threads can be resource-intensive. With pooling, resources are reused, minimizing the overhead inadvertently tied to constant creation and destruction of these objects.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
class ConnectionPool {
private List<Connection> availableConnections;
public ConnectionPool(int poolSize) throws SQLException {
availableConnections = new ArrayList<>();
for (int i = 0; i < poolSize; i++) {
availableConnections.add(DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password"));
}
}
}
In this code snippet, we initialize a pool of database connections. This batch creation of connections reduces the overhead of individual connection setups during high-demand scenarios.
2. Improved Performance
By limiting the number of times resources are instantiated from scratch, pool architectures can enhance the overall performance of applications. When multiple threads or processes can share access to resources concurrently, we typically observe quicker response times.
3. Scalability
Pool architecture can improve scalability dramatically. As the demand for resources increases, applications can dynamically adjust the pool size based on real-time needs, provided that the underlying infrastructure can handle it.
4. Simplified Resource Management
By abstracting the complexity of resource management, pool architecture allows developers to focus on application logic while the pool manages the details of resource allocation and deallocation.
public Connection getConnection() throws SQLException {
if (availableConnections.isEmpty()) {
throw new SQLException("No available connections");
}
return availableConnections.remove(availableConnections.size() - 1);
}
This code snippet demonstrates how we obtain an available connection. If the pool is empty, it's crucial to handle this scenario gracefully, reflecting the management aspect of a pool architecture.
5. Improved Responsiveness During Load Spikes
With a pre-initialized pool, your application remains responsive even during load spikes, as resources can be allocated and deallocated in an instant.
Challenges of Pool Architecture
While pool architecture offers several advantages, it is essential to recognize that it also has its challenges:
1. Complexity in Implementations
Managing a pool of resources can add complexity to system design. Implementors must ensure efficient handling of resource contention, leaks, and timeouts.
2. High Resource Utilization Risks
Under-load situations can lead to under-utilization of the pooled resources. If not managed correctly, this can result in wasted resources.
3. Performance Bottlenecks
If a pool's configuration is not correctly calibrated, it can lead to performance bottlenecks. For example, too few threads or database connections in the pool can cause blocking and lagging.
Pool Architecture in Different Contexts
Database Connection Pooling
In web applications, database connection pooling is a common application of this architecture. Connection pools allow the simultaneous processing of multiple database requests with efficiency. For example, popular libraries such as HikariCP and Apache DBCP provide robust connection pooling mechanisms.
Thread Pooling
Thread pooling can also leverage pool architecture to manage threads effectively. A thread pool reuses a predefined number of threads to execute tasks, avoiding the overhead of thread creation for each job.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
class ThreadPoolExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(10);
for (int i = 0; i < 50; i++) {
executor.execute(new Task(i));
}
executor.shutdown();
}
}
class Task implements Runnable {
private final int taskNumber;
Task(int taskNumber) {
this.taskNumber = taskNumber;
}
@Override
public void run() {
System.out.println("Executing Task " + taskNumber);
}
}
In this example, the ExecutorService
creates a pool of threads to handle tasks concurrently. This utilization of pooling significantly boosts performance by minimizing the overhead tied to thread management.
Future Trends in Pool Architecture
- Dynamic Scaling: Future implementations will likely incorporate AI and ML to adjust pool sizes dynamically based on real-time usage metrics.
- Serverless Integrations: As serverless architectures gain popularity, pooling techniques will evolve to accommodate statelessness while still allowing efficient resource management.
- Containerization: With container orchestration platforms like Kubernetes, pooling strategies will adapt to the constraints and efficiencies inherent in containerized environments.
Closing the Chapter
Pool architecture certainly presents a strong case as a critical component for future scalability in software systems. Its benefits—including resource efficiency, improved performance, and simplified resource management—are compelling. However, one must also navigate the challenges it poses, such as complexity and potential performance bottlenecks.
As we move towards an increasingly interconnected digital landscape, embracing pool architecture could be key to building responsive, resilient, and scalable applications. It remains vital for developers to be judicious in its implementation, ensuring they maximize benefits while effectively managing challenges.
For further reading, consider exploring resources on HikariCP for efficient database connection pooling and checking out the Java Concurrent package for more in-depth thread management techniques.
With the right approach, pool architecture can indeed pave the way for the next generation of scalable systems. Would you consider adopting pool architecture in your projects?
Checkout our other articles