Troubleshooting Data Access Issues in Spring 3.1
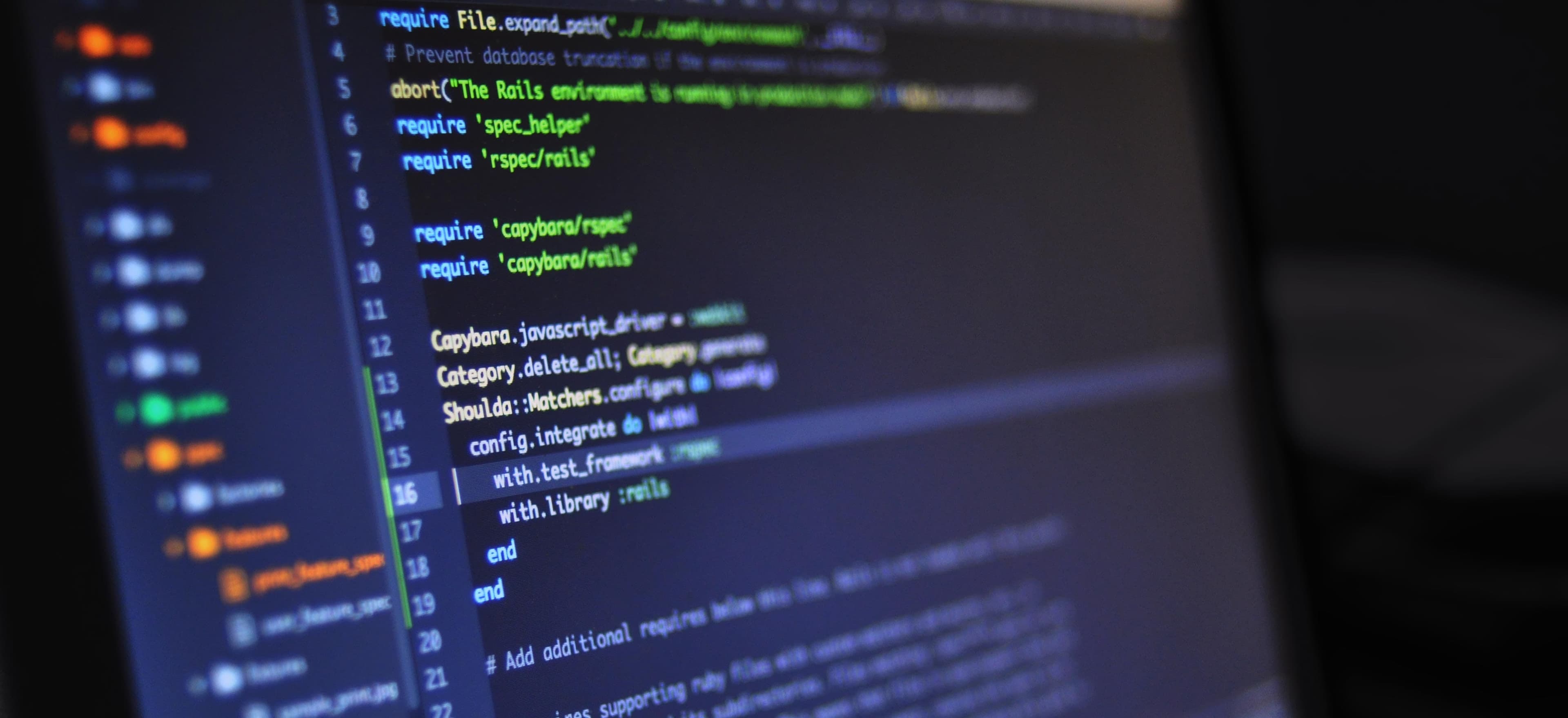
- Published on
Troubleshooting Data Access Issues in Spring 3.1
Data access is a fundamental part of any Java web application, and Spring Framework provides an excellent infrastructure to handle database interactions. However, developers often face challenges when working with data access technologies such as JDBC, Hibernate, or JPA. In this blog post, we'll dive deep into troubleshooting data access issues in Spring 3.1. We’ll explore typical problems, how to diagnose them, and solutions you can implement to resolve these issues effectively.
Understanding Spring 3.1 Data Access Mechanism
Spring 3.1 introduced several improvements, particularly in how it handles data access. The most notable feature is enhanced support for JPA and Hibernate, streamlining transaction management and simplifying the configuration process.
Key Components of Spring Data Access
Understanding the key components of Spring’s data access abstraction will help you diagnose issues:
- DataSource Configuration: Defines the database connection settings.
- JdbcTemplate: Simplifies JDBC operations and handles resources automatically.
- Exception Translation: Converts database-specific exceptions into a Spring consistent hierarchy.
With these components in play, let's explore common errors developers encounter.
Common Data Access Issues
1. Bean Creation Exceptions
Issue: You may encounter NoSuchBeanDefinitionException
during the application context initialization. This can happen if the DataSource
or EntityManagerFactory
bean isn't defined correctly.
Solution: Ensure that your configuration is set up correctly in either XML or Java configuration. Here’s an example of a common XML configuration:
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/mydb"/>
<property name="username" value="user"/>
<property name="password" value="password"/>
</bean>
<bean id="sessionFactory" class="org.springframework.orm.hibernate5.LocalSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<property name="packagesToScan" value="com.example.model"/>
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">org.hibernate.dialect.MySQL5Dialect</prop>
<prop key="hibernate.show_sql">true</prop>
</props>
</property>
</bean>
2. Transaction Management Errors
Issue: Often you will experience issues with transaction management when the method annotated with @Transaction
fails but does not rollback the transaction.
Solution: Ensure your service class is annotated with @Transactional
and that your transaction manager bean is correctly configured.
@Service
public class UserService {
@Autowired
private UserDao userDao;
@Transactional
public void createUser(User user) {
userDao.save(user); // This should rollback on failure
}
}
Note that placing @Transactional
on a method in the same class won't affect transactions due to Spring's proxy mechanism. Ensure to call the method externally via a proxy.
3. SQLException Handling
Issue: Developers often mistakenly handle SQL exceptions without using Spring's exception translation mechanism, resulting in application crashes.
Solution: Leverage Spring’s DataAccessException
to ensure any SQL-related exceptions are translated properly.
public User getUserById(Long id) {
try {
return jdbcTemplate.queryForObject("SELECT * FROM users WHERE id = ?", new Object[]{id}, new UserRowMapper());
} catch (DataAccessException e) {
// Log the error with proper logging framework
throw new CustomDataAccessException("Error while accessing user data", e);
}
}
4. Lazy Loading Issues
Issue: When using Hibernate, developers might come across LazyInitializationException
when attempting to access a lazily loaded property outside of a session context.
Solution: To resolve this, you have several options:
- Use
FetchType.EAGER
to load all necessary data in one go. - Utilize the
@Transactional
annotation to keep the session open long enough to get the required data.
@Entity
public class User {
@OneToMany(mappedBy = "user", fetch = FetchType.LAZY)
private Set<Order> orders;
}
Tools for Diagnosing Issues
While troubleshooting, developers have access to various tools that can simplify the diagnosis process:
1. Log Frameworks
Developers should leverage logging frameworks (like SLF4J and Logback) to get a detailed view of what's going on with database queries.
2. SQL Profiler
Using a SQL profiler can help you see the actual queries being executed against the database, along with their execution time.
3. Debugging
Use debugging tools in your IDE, such as breakpoints and watch expressions, to trace the flow of your application, especially around data access logic.
Best Practices to Avoid Data Access Issues
-
Use Spring’s Template Classes: For simpler CRUD operations, use
JdbcTemplate
andNamedParameterJdbcTemplate
. They simplify error handling and resource management.NamedParameterJdbcTemplate namedParameterJdbcTemplate = new NamedParameterJdbcTemplate(dataSource);
-
Embrace Annotations: Utilize Spring’s annotations (
@Transactional
,@Repository
, etc.) to manage transaction boundaries and mark your persistence classes. -
Profile Your Queries: Routinely profile your SQL queries to ensure they are efficient. Avoid unnecessary data retrieval with appropriate
WHERE
clauses. -
Upgrade to Newer Versions: If possible, upgrade to a newer version of Spring that has fixed known bugs and offered improved features. Although Spring 3.1 was groundbreaking at the time, later versions significantly enhance the data access layer's capabilities. Visit Spring's official documentation for more insights.
To Wrap Things Up
Data access is a vital part of Java applications using Spring. While troubleshooting issues can feel overwhelming, understanding the common problems and having the right tools at your disposal can significantly reduce development time. By adhering to best practices and continually refining your approach, you can ensure a smooth integration with databases.
In conclusion, it’s crucial to stay informed about the latest enhancements in Spring and regular paradigms in Java data access. The above strategies and coding practices should empower you to tackle any data access issues in Spring 3.1 effectively. If you encounter challenges beyond the common issues outlined here, consulting more detailed resources such as Baeldung's Spring Data guide can offer deeper insights into specific scenarios. Happy coding!