Common CI/CD Pipeline Pitfalls and How to Overcome Them
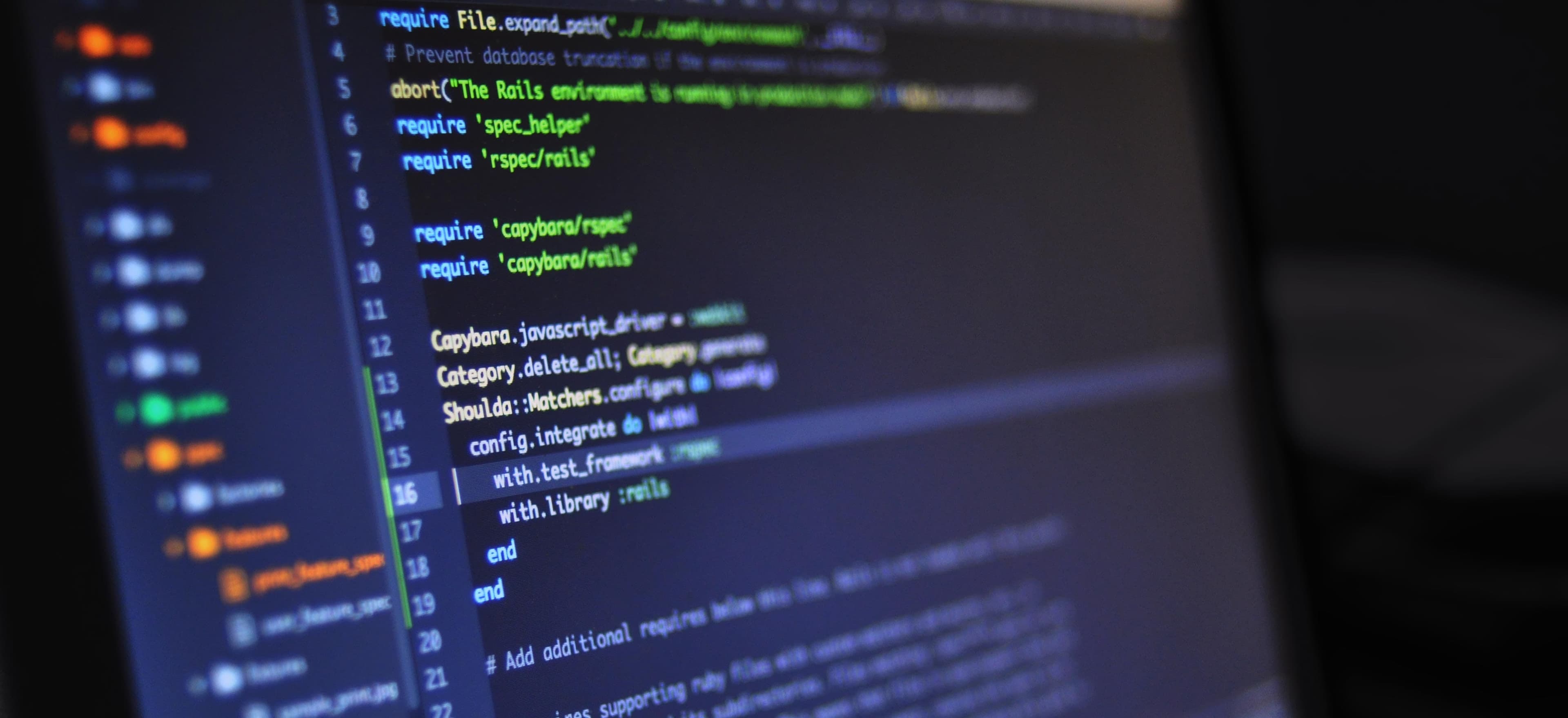
- Published on
Common CI/CD Pipeline Pitfalls and How to Overcome Them
Continuous Integration (CI) and Continuous Deployment (CD) are crucial components of modern software development. However, creating a CI/CD pipeline isn’t without its challenges. In this blog post, we’ll explore some of the most common pitfalls teams encounter when setting up their CI/CD pipelines and provide actionable strategies to overcome them.
Understanding CI/CD
Before diving into the pitfalls, it’s important to have a basic understanding of CI and CD.
-
Continuous Integration (CI): This practice involves automatically integrating code changes from multiple contributors into a shared repository. The primary goal is to minimize integration issues, ensuring that changes are tested and merged frequently.
-
Continuous Deployment (CD): This extends CI by automatically deploying all code changes to production after the build stage. The key here is to release software in a way that is seamless and without human intervention.
For more in-depth knowledge, consult resources such as Atlassian’s guide on CI/CD.
Pitfall #1: Not Automating Enough
The Challenge
A CI/CD pipeline isn’t just about integrating code; it’s also about automating the build, test, and deployment processes. Failure to automate these processes can lead to human error, longer release cycles, and inconsistent deployments.
The Solution
Automation is key. Leverage tools such as Jenkins, GitLab CI, or CircleCI. These platforms allow you to define how your code should be built and tested.
Here's an example of a simple Jenkins Pipeline for a Java project:
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
sh 'mvn clean package'
}
}
}
stage('Test') {
steps {
script {
sh 'mvn test'
}
}
}
stage('Deploy') {
steps {
script {
sh 'java -jar target/my-app.jar'
}
}
}
}
}
Why This Matters: Automating builds and tests reduces human error, ensures consistency, and speeds up the release process.
Pitfall #2: Insufficient Testing
The Challenge
Rushing through testing is a common mistake teams make. Without comprehensive testing in place, you may end up deploying flawed code to production.
The Solution
Incorporate various types of testing into your CI pipeline:
- Unit Testing: Validate the smallest parts of the application to ensure correctness.
- Integration Testing: Check if different modules work together.
- End-to-End Testing: Simulate user scenarios to validate the entire application flow.
Here's an example of a basic JUnit test in a Java application:
import static org.junit.Assert.*;
import org.junit.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3));
}
}
Why This Matters: Properly implemented testing ensures that defects are caught early, preventing bug-ridden deployments. For more on testing strategies, take a look at Martin Fowler's Testing Pyramid.
Pitfall #3: Ignoring Security
The Challenge
Security often takes a backseat in CI/CD implementations, which can introduce vulnerabilities into your application.
The Solution
Integrate security tools into your CI/CD pipeline through a method known as DevSecOps. Tools such as Snyk and SonarQube can help identify vulnerabilities early in the development cycle.
A simple script to run SonarQube analysis in your Jenkins pipeline would look something like this:
stage('SonarQube Analysis') {
steps {
script {
sh 'mvn sonar:sonar -Dsonar.projectKey=my-project -Dsonar.host.url=http://sonarqube:9000'
}
}
}
Why This Matters: Addressing security early lowers the risk of vulnerabilities impacting production environments.
Pitfall #4: Lack of Version Control for Infrastructure
The Challenge
Many teams overlook the importance of versioning their infrastructure. This can lead to “Configuration Drift,” where environments become inconsistent over time.
The Solution
Infrastructure as Code (IaC) is a solution here. Using tools like Terraform or AWS CloudFormation allows teams to manage and provision their infrastructure programmatically.
Example of a simple Terraform script:
provider "aws" {
region = "us-east-1"
}
resource "aws_instance" "web" {
ami = "ami-0c55b159cbfafe01e"
instance_type = "t2.micro"
}
Why This Matters: Version controlling your infrastructure ensures consistent environment setups, making deployments predictable and reliable.
Pitfall #5: Overcomplicating the Pipeline
The Challenge
Sometimes, teams try to implement overly complex workflows in their CI/CD pipeline. This can lead to confusion and errors, negating the benefits of automation.
The Solution
Keep it simple. Focus on build, test, and deploy; only introduce complexities if they add real value. Strive to adhere to the 12-factor app methodology which emphasizes simplicity and best practices.
Why This Matters: A streamlined pipeline is easier to maintain and troubleshoot, leading to faster release cycles and happier development teams.
Closing the Chapter
While setting up a CI/CD pipeline, it’s critical to be aware of common pitfalls. By focusing on automation, robust testing, security, infrastructure version control, and simplicity, you can create a resilient CI/CD pipeline that enhances your development process.
For further reading on CI/CD best practices, consider checking out DevOps.com’s guide to building CI/CD pipelines.
By approaching your CI/CD strategy strategically, you not only improve workflow efficiency but also deliver high-quality software that meets modern user expectations. Stay committed to continuous improvement and keep iterating on your pipeline. Happy coding!