Optimizing JPA Performance: Common Pitfalls to Avoid
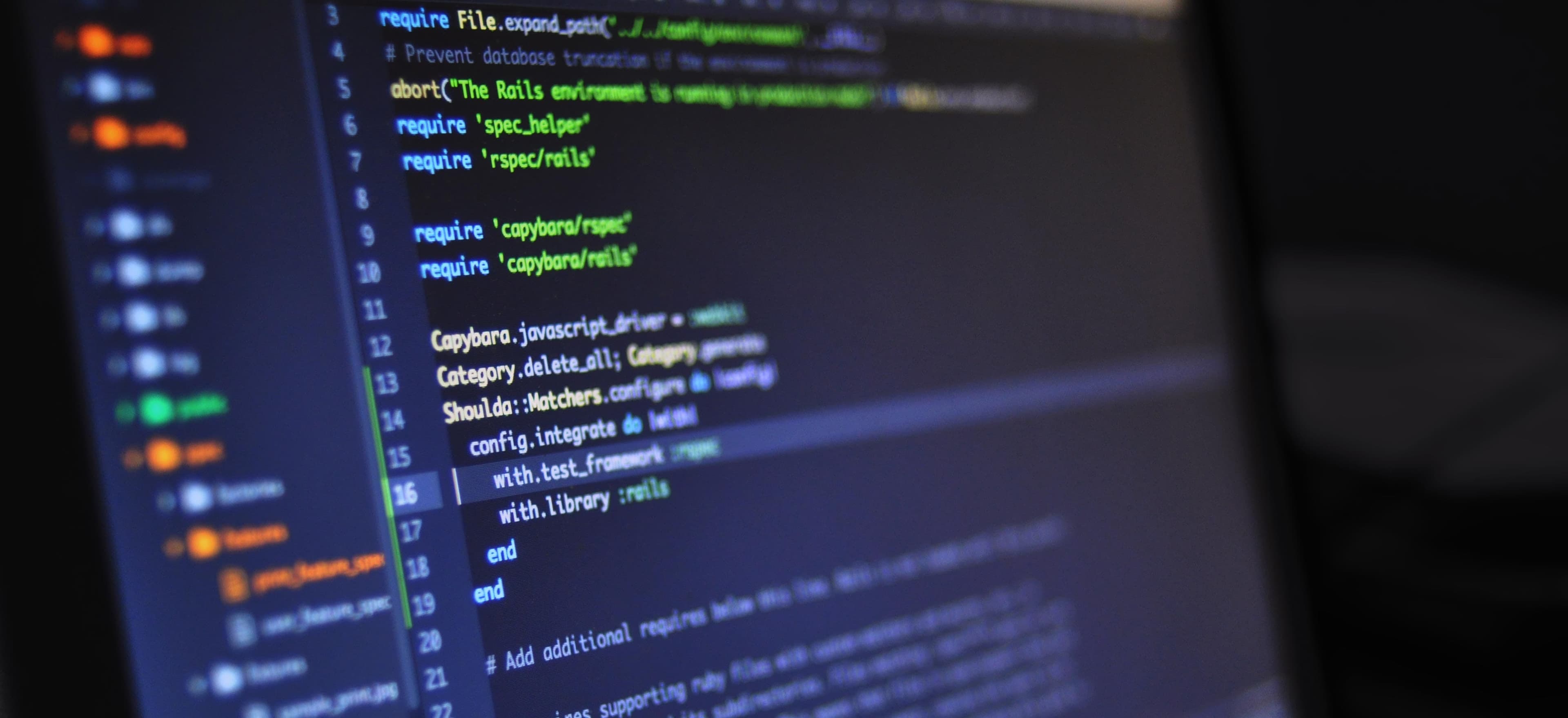
- Published on
Optimizing JPA Performance: Common Pitfalls to Avoid
Java Persistence API (JPA) is a powerful framework that simplifies the development of database-backed applications in Java. However, it can lead to performance issues if misconfigured. In this post, we will discuss common pitfalls to avoid when using JPA and how to optimize your application's performance. Whether you are a seasoned developer or just starting, the insights in this article will help you write efficient, scalable Java applications.
Table of Contents
- Understanding JPA
- Common Performance Pitfalls
- 2.1 N+1 Select Problem
- 2.2 Inefficient Fetch Strategies
- 2.3 Improper Use of Entity Relationships
- 2.4 Ignoring Transaction Management
- Performance Optimization Techniques
- 3.1 Use of Fetch Joins
- 3.2 Batch Processing
- 3.3 Caching Strategies
- 3.4 Profiling and Monitoring
- Conclusion
1. Understanding JPA
Before diving into optimization, it is crucial to understand what JPA is and how it operates. JPA is a specification that allows developers to manage relational data in their Java applications. It provides an object-relational mapping (ORM) framework, allowing you to work with entities and their relationships without dealing directly with SQL.
Get Started with JPA
If you're just starting, check out the official documentation for a comprehensive guide on setting up JPA.
2. Common Performance Pitfalls
2.1 N+1 Select Problem
One of the most frequent performance issues when using JPA is the N+1 select problem. This occurs when a query requires fetching an entity and then lazily loading its relationships in separate queries.
Example:
List<Product> products = entityManager.createQuery("SELECT p FROM Product p", Product.class).getResultList();
for (Product product : products) {
System.out.println(product.getCategory().getName());
}
Why it's a Pitfall: The above example results in one query to get the products and N additional queries to fetch categories (one for each product). This leads to a significant number of database round trips, which can degrade performance.
2.2 Inefficient Fetch Strategies
Fetching strategies (EAGER vs. LAZY) can significantly impact performance. While EAGER loading fetches related entities in one query, it may retrieve unnecessary data. Conversely, LAZY loading might lead to the N+1 select problem mentioned above.
Solution:
Choose the fetching strategy based on your use case. If you frequently access a relationship and its dataset is not overly large, EAGER might be suitable. However, for many-to-many relationships or large datasets, LAZY loading is typically better.
2.3 Improper Use of Entity Relationships
Another common issue is the misuse of relationships. For instance, using cascade=ALL
indiscriminately can lead to unintended consequences during data persistence, which can result in performance overhead.
Solution:
@Entity
public class Order {
@OneToMany(mappedBy = "order", cascade = CascadeType.PERSIST)
private List<OrderItem> items;
}
Here, we only cascade PERSIST
, avoiding overhead during remove operations. Be judicious when using cascade types to maintain performance.
2.4 Ignoring Transaction Management
Not managing transactions properly can also lead to performance issues. If entities are not managed correctly within transactions, it can lead to longer database locks or memory leaks.
Best Practices:
- Always open and close sessions properly.
- Use
@Transactional
to mark service-layer methods to manage transactions effectively.
3. Performance Optimization Techniques
3.1 Use of Fetch Joins
Fetching related entities can improve performance, especially when working with large datasets.
Example:
List<Product> products = entityManager.createQuery(
"SELECT p FROM Product p JOIN FETCH p.category", Product.class
).getResultList();
Why it's Effective: This query retrieves products and their associated categories in a single query, preventing the N+1 select problem.
3.2 Batch Processing
For large operations, such as inserting multiple entities, using batch processing can drastically improve performance.
Example:
EntityTransaction transaction = entityManager.getTransaction();
transaction.begin();
for (int i = 0; i < 1000; i++) {
entityManager.persist(new Product("Product " + i));
if (i % 50 == 0) { // 50, same as the JDBC batch size
entityManager.flush();
entityManager.clear();
}
}
transaction.commit();
Why it Works: This reduces the number of round-trips to the database by grouping multiple inserts/updates within a transaction.
3.3 Caching Strategies
Implementing caching can also lead to significant performance enhancements. JPA providers (like Hibernate) offer multiple caching layers (first-level cache, second-level cache, and query cache).
Example:
@Entity
@Cacheable
public class Product {
@Id
private Long id;
private String name;
// Getters and setters
}
Why Caching is Important: Caching can minimize database queries and improve response times considerably. Be mindful of cache invalidation to ensure data integrity.
3.4 Profiling and Monitoring
Monitor your application's performance regularly. Use profiling tools to identify bottlenecks in queries and transaction processing.
- Tools: Consider using tools like JVisualVM or YourKit to monitor JPA operations.
- Database Logs: Look for slow queries and adjust them as necessary.
4. Conclusion
Optimizing JPA performance involves understanding both the framework and how it interacts with your database. Avoid common pitfalls like N+1 select problems, improper use of fetching strategies, and mismanaged transactions. Implement optimization techniques like fetch joins, batch processing, and caching to enhance performance.
For further reading and deeper insights, consider visiting resources such as Hibernate Performance Tuning and JPA Best Practices for more advanced strategies.
By following these guidelines, you'll set a strong foundation for building high-performance Java applications utilizing JPA. Happy coding!
Checkout our other articles