Understanding Java Collections: Common Quirks Explained
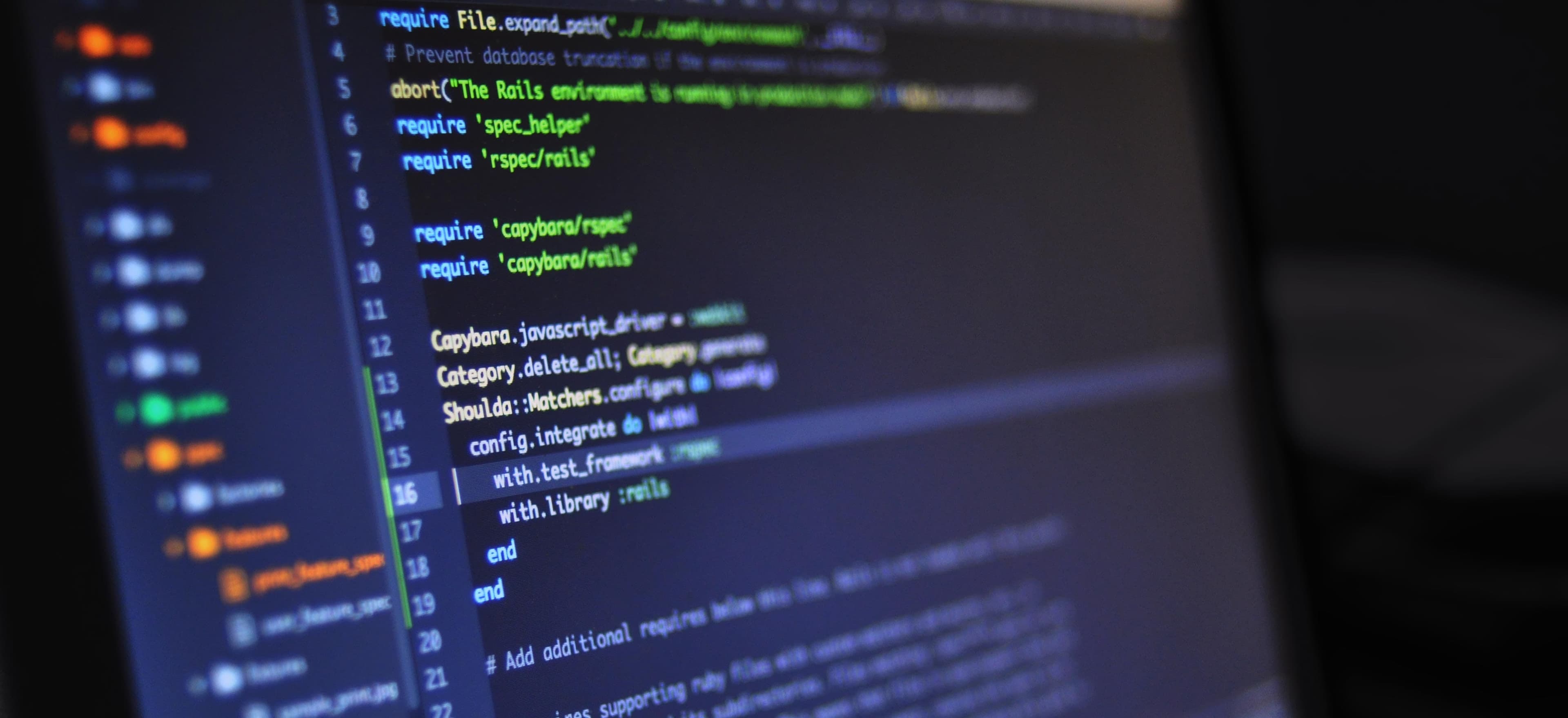
- Published on
Understanding Java Collections: Common Quirks Explained
Java Collections Framework (JCF) is an essential part of Java programming that helps store, manipulate, and manage data efficiently. It provides a set of classes and interfaces to handle groups of objects, and mastering these collections can make you a far more effective Java developer. However, new users often encounter quirks and unexpected behavior while working with Java Collections, which can be confusing. In this blog post, we'll cover common quirks in Java Collections, providing clear explanations and code examples to enhance your understanding.
What Are Java Collections?
Java Collections are a set of classes and interfaces that implement commonly reusable collection data structures. These include lists, sets, maps, and queues. The core interface of the Collections Framework resides in the java.util
package, providing a range of options suitable for different programming needs. Understanding the nuances of these collections can help you write more efficient, cleaner, and more robust code.
Types of Collections
Before diving into common quirks, it's important to understand the major types of collections in Java:
-
List: An ordered collection (also known as a sequence) that can contain duplicate elements. The most commonly used implementations are
ArrayList
andLinkedList
. -
Set: A collection that cannot contain duplicate elements. The most commonly used implementations are
HashSet
andTreeSet
. -
Map: An object that maps keys to values, with unique keys. Common implementations include
HashMap
andTreeMap
. -
Queue: Typically used to hold elements prior to processing. Implementations include
LinkedList
,PriorityQueue
, andArrayDeque
.
Common Quirks in Java Collections
1. Differences in .equals() and .hashCode()
One of the most common quirks is related to how equality is evaluated for objects in collections, especially in Set
and Map
. When you store objects in collections that rely on hashing (like HashSet
or HashMap
), it is crucial to correctly implement the equals()
and hashCode()
methods in your object classes.
Why?
If two objects are considered equal according to the equals()
method but return different hash codes, they can behave unexpectedly in collections. For example, adding a duplicate entry in a HashSet
may lead to one of the entries being lost.
Example
class User {
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (!(obj instanceof User)) return false;
User user = (User) obj;
return username.equals(user.username);
}
@Override
public int hashCode() {
return username.hashCode();
}
}
In this code, the User
class overrides equals()
and hashCode()
. This ensures that two User
instances with the same username are treated as equal and are therefore not added twice in a HashSet
.
2. Iterating with Fail-Fast Behavior
Java collections are designed to be fail-fast, meaning they throw a ConcurrentModificationException
if they detect that the collection has been modified while iterating through it. This can often catch developers by surprise.
Why?
Fail-fast behavior is intended to prevent unpredictable behavior in your code due to concurrent modifications.
Example
List<String> names = new ArrayList<>(Arrays.asList("Alice", "Bob", "Charlie"));
for (String name : names) {
if (name.equals("Bob")) {
names.remove(name); // Causes ConcurrentModificationException
}
}
To avoid this exception, consider using an Iterator
to safely remove elements:
Iterator<String> iterator = names.iterator();
while (iterator.hasNext()) {
String name = iterator.next();
if (name.equals("Bob")) {
iterator.remove(); // Safe way to remove elements
}
}
3. The Pitfalls of Autoboxing
Java Collections can lead to unexpected behavior due to autoboxing and unboxing of primitives to their corresponding wrapper classes. This can impact performance and logic, especially with collections that store primitive types.
Why?
Collections like ArrayList<Integer>
actually store Integer
objects, not int
. If you mistakenly rely too much on boxing or fail to account for potential NullPointerExceptions
, bugs can arise.
Example
List<Integer> numbers = new ArrayList<>();
numbers.add(1); // Autoboxing: int 1 is converted to Integer 1
numbers.add(null); // Possible null entry
for (Integer number : numbers) {
System.out.println(number + 10); // Throws NullPointerException if number is null
}
Be cautious when processing collections that can include null
and ensure that your code can handle them gracefully.
4. Consideration of Ordering in Sets
Another common quirk is the order of elements in collections. For instance, HashSet
does not guarantee any specific order, while LinkedHashSet
maintains insertion order, and TreeSet
sorts elements according to their natural ordering or a specified comparator.
Why?
If you require a collection that maintains order, choosing the appropriate set implementation is crucial.
Example
Set<String> hashSet = new HashSet<>();
hashSet.add("Charlie");
hashSet.add("Alice");
hashSet.add("Bob");
System.out.println(hashSet); // No guarantee on order of output
Set<String> linkedHashSet = new LinkedHashSet<>(hashSet);
System.out.println(linkedHashSet); // Outputs in the order of insertion
If order matters in your application, choose LinkedHashSet
or TreeSet
appropriately.
A Final Look
Understanding Java Collections and their quirks can significantly improve your programming efficiency. By effectively utilizing the strengths and being mindful of the limitations of different collection types, you can write cleaner and more effective Java code. Enhancing your knowledge of Java Collections not only benefits your immediate projects but also builds a strong foundation for more complex aspects of Java programming.
For further reading, consider checking out Oracle's official Java Collections Framework documentation and explore advanced topics such as parallel streams and concurrent collections.
By mastering these common quirks and pitfalls, you will enhance your ability to manage data in your applications, leading to robust, efficient, and maintainable code. Happy coding!
Checkout our other articles