Common Issues When Integrating Moxy with JAX-WS Services
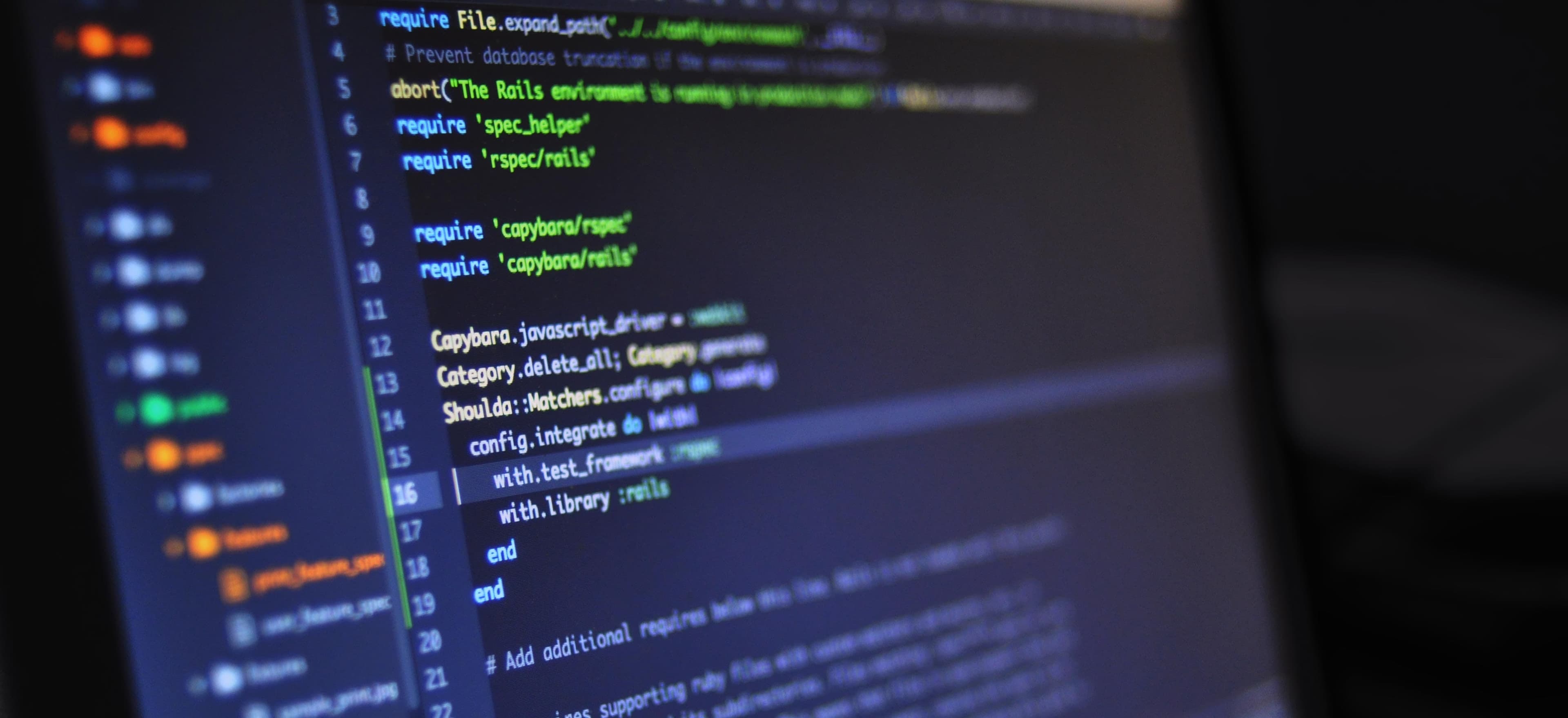
- Published on
Common Issues When Integrating Moxy with JAX-WS Services
Integration between Moxy and JAX-WS can provide an efficient means of handling XML data binding in Java applications. However, like any integration task, there are challenges and common issues that developers might encounter when trying to make these two frameworks work together seamlessly. This blog post will delve into those issues, offer insights into resolution strategies, and provide relevant context as needed.
Overview of Moxy and JAX-WS
Moxy is a JAXB (Java Architecture for XML Binding) implementation that primarily focuses on performance. It offers features like JSON binding, advanced type handling, and the ability to map XML content to Java objects. Meanwhile, JAX-WS (Java API for XML Web Services) simplifies the development of web services using XML messaging protocols.
Using these two frameworks together can yield a coherent and powerful web service architecture. However, certain complexities arise during integration.
Common Issues
1. Mismatched JAXB Annotations
One common issue occurs when JAXB annotations—the foundation upon which both Moxy and JAX-WS operate—are not aligned. For instance, if you use different annotations to indicate elements, the data may not bind correctly, leading to runtime exceptions.
Code Example:
Here’s a simple Java class demonstrating JAXB annotations:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "customer")
public class Customer {
private String name;
@XmlElement(name = "customerName")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In the above code, if the web service expects <customerName>
but you provide a different tag name, it could lead to issues.
Solution:
Ensure that the class-level and field-level annotations in your data model are consistent with the XML structure the JAX-WS service produces or consumes.
2. Unsupported Features
Moxy offers advanced capabilities like custom adapter support and JSON binding, but JAX-WS doesn’t completely utilize all these features. This inconsistency can lead to serialization issues.
Code Example:
Consider a scenario where you might want to serialize a Java enum using Moxy:
import javax.xml.bind.annotation.XmlEnum;
@XmlEnum
public enum OrderStatus {
NEW,
PROCESSING,
SHIPPED
}
Using this enum with JAX-WS might lead to serialization issues if the XML schema does not anticipate enumerated types correctly.
Solution:
Stick to the basic features that JAX-WS natively supports. Avoid using features exclusively provided by Moxy unless absolutely necessary, and ensure compatibility with the expected XML schema.
3. Namespace Misconfiguration
Moxy allows for careful handling of XML namespaces, but misconfiguration can lead to errors during parsing or serialization.
Code Example:
When defining namespaces, ensure that they are aligned correctly in the XML mappings:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement(name = "order")
public class Order {
@XmlElement(namespace = "http://www.example.com/orders")
private OrderStatus status;
// Getters and Setters
}
If the consuming or producing JAX-WS service expects a different namespace, mismatches can occur.
Solution:
Maintain a well-structured XML namespace. Document the namespaces expected by both Moxy and JAX-WS components, and reference them correctly in your annotations.
4. Proxy-Related Issues
When using JAX-WS, sometimes the proxies generated can lead to issues because they might not be compatible with the expected structure defined by Moxy.
Code Example:
Here’s how you can generate a proxy:
URL wsdlURL = new URL("http://localhost:8080/service?wsdl");
MyService service = new MyService(wsdlURL);
MyPortType port = service.getMyPort();
If MyPortType
is expected to be serialized/deserialized using Moxy conventions but isn't designed for it, runtime errors may arise.
Solution:
Manually handle the response and request mappings. Ensure that the data being sent/received matches Moxy expectations, potentially utilizing adapter classes to bridge the gap.
5. Antipatterns in Exception Handling
When integrating Moxy with JAX-WS, developers often overlook effective exception handling techniques, leading to poor fault tolerance.
Code Example:
Improve your handling of exceptions in a JAX-WS service like this:
@WebService
public class MyWebService {
@WebMethod
public String getOrder(String orderId) {
try {
// fetch order logic
} catch (Exception e) {
throw new WebServiceException("Error fetching order", e);
}
}
}
An unhandled exception during XML conversion could expose implementation details instead of user-friendly messages.
Solution:
Implement a global exception handler that captures all exceptions and returns well-structured SOAP faults with appropriate error messages. This approach is particularly beneficial in maintaining a clean API.
Debugging Tips
To effectively identify issues when integrating these frameworks, consider the following debugging strategies:
- Enable Verbose Logging: Both Moxy and JAX-WS support logging. Set your logging level to DEBUG for detailed output.
- Validate Against the XML Schema: Ensure your data model adheres to the expected XML schema. Use tools like JAXB's validation features.
- Use Unit Tests: Writing unit tests for each service endpoint can expose integration issues early in the development cycle.
My Closing Thoughts on the Matter
Integrating Moxy with JAX-WS services can open the door to efficient XML handling in Java applications. However, awareness of common issues such as mismatched annotations, unsupported features, namespace misconfigurations, proxy-related issues, and poor exception handling is crucial for a successful integration.
By following best practices and leveraging the provided solutions, you can streamline your integration process, making it less error-prone. For further reading, consider the Oracle Soa documentation or explore resources on Moxy features.
Stay informed, and optimize your Java service integrations today!