Dynamic Variables in PostgreSQL: A Complete Guide
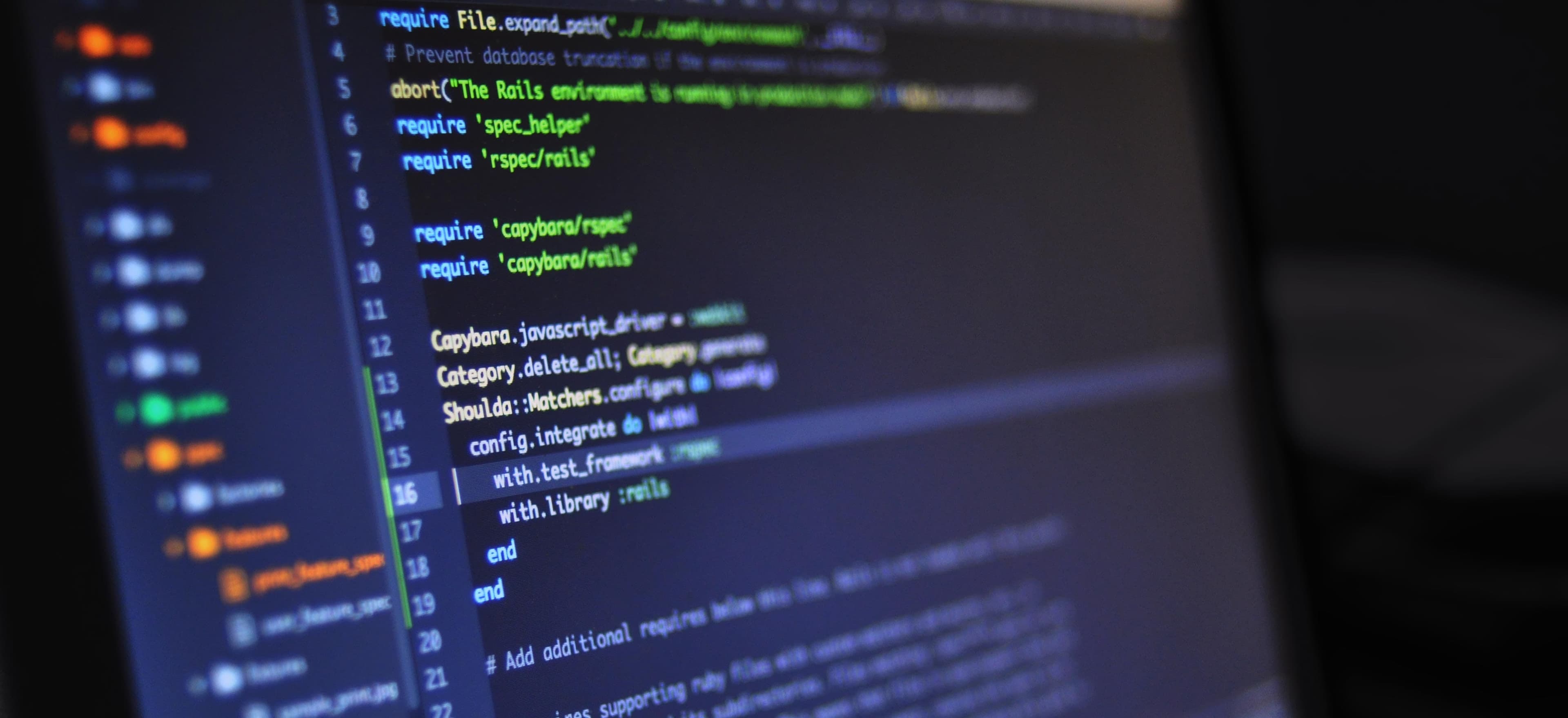
- Published on
Dynamic Variables in PostgreSQL: A Complete Guide
When working with databases, flexibility and adaptability are key. PostgreSQL, an advanced open-source relational database system, provides several features that enhance its usability, one of which is the concept of dynamic variables. In this comprehensive guide, we'll explore what dynamic variables are, how to use them in PostgreSQL, and why they matter in the context of database management and application development.
What Are Dynamic Variables?
Dynamic variables are variables whose values can change at runtime based on the logic of your application or queries. In PostgreSQL, these are often represented using table columns, user-defined variables in functions, or temporary tables that allow you to store values temporarily within a session.
Key Benefits of Dynamic Variables:
-
Flexibility: Dynamic variables allow you to store information that can change as your application processes data. This flexibility is crucial for applications that handle varied input and require real-time adjustments.
-
Performance: By leveraging dynamic variables, you can optimize queries and reduce processing time. Instead of hardcoding values, dynamic variables enable you to pass parameters, significantly enhancing performance.
-
State Management: In a database transaction, you can manage states effectively. For instance, using dynamic variables allows you to track changes and maintain session-specific data.
Types of Dynamic Variables in PostgreSQL
-
Session Variables: These are temporary variables that can store data for the duration of a database session.
-
Function Parameters: These variables allow you to pass values into functions, enabling dynamic behavior based on input.
-
Temporary Tables: When you need to store data temporarily, you can create temporary tables that last for the duration of a session or transaction.
Using Dynamic Variables: Examples and Techniques
1. Session Variables
PostgreSQL does not have true session variables as in some other databases, but you can emulate them using the SET
command.
SET myapp.current_user TO 'john_doe';
This command sets a custom variable myapp.current_user
for the session. You can retrieve it using:
SHOW myapp.current_user;
Why Use Session Variables?
Using session variables enables the storage of user-specific settings within a session. For example, if multiple users are querying the database, each can have their settings without interference.
2. Function Parameters
Dynamic behavior is often required in stored functions. You can create a function with parameters, allowing you to pass dynamic values during execution:
CREATE OR REPLACE FUNCTION get_user_details(username TEXT)
RETURNS TABLE(id INT, email TEXT) AS $$
BEGIN
RETURN QUERY
SELECT id, email FROM users WHERE username = username;
END;
$$ LANGUAGE plpgsql;
Invoking the Function
You can call the function using:
SELECT * FROM get_user_details('john_doe');
Importance of Function Parameters
Passing parameters into functions allows for reusable code. Instead of writing multiple queries for different users, a single function can handle various input.
3. Temporary Tables
Temporary tables are a powerful way to use dynamic variables in PostgreSQL:
CREATE TEMP TABLE temp_orders (
order_id SERIAL PRIMARY KEY,
user_id INT,
order_date DATE
);
You can insert data into this temporary table just like a regular table:
INSERT INTO temp_orders (user_id, order_date) VALUES (1, NOW());
And query it later:
SELECT * FROM temp_orders WHERE user_id = 1;
Temporary tables exist only within the session and are automatically dropped at the end of the session or transaction, ensuring there is no residual data left behind.
Why Use Temporary Tables?
Temporary tables allow for efficient handling of intermediate data within transactions or batch jobs without cluttering the main database tables.
Best Practices for Using Dynamic Variables
-
Keep Scope in Mind: Understand the scope of your variables. Session variables exist for the whole session; function parameters exist only during execution.
-
Use Meaningful Names: Naming conventions are vital. Use clear names for your dynamic variables to ensure your code is readable and maintainable.
-
Limit Temporary Table Usage: While temporary tables are handy, overusing them can affect performance. Use them when necessary and drop them when finished.
-
Handle Exceptions: Always include exception handling in your functions that utilize dynamic variables. This approach ensures that your application can handle unexpected cases gracefully.
The Closing Argument
Dynamic variables in PostgreSQL are essential for creating flexible and efficient database applications. Whether you use session variables, function parameters, or temporary tables, understanding how to implement and utilize these dynamic structures will significantly enhance your database management capabilities.
For more tips and advanced PostgreSQL features, you can refer to the PostgreSQL Official Documentation, which provides in-depth explanations and examples.
By exploring the full potential of dynamic variables, you can improve the functionality, performance, and user experience of your database environments. Whether you are developing sophisticated applications or performing data analysis, mastering dynamic variables is a skill worth acquiring. Happy querying!