Common RegEx Pitfalls in Java: Avoid These Mistakes!
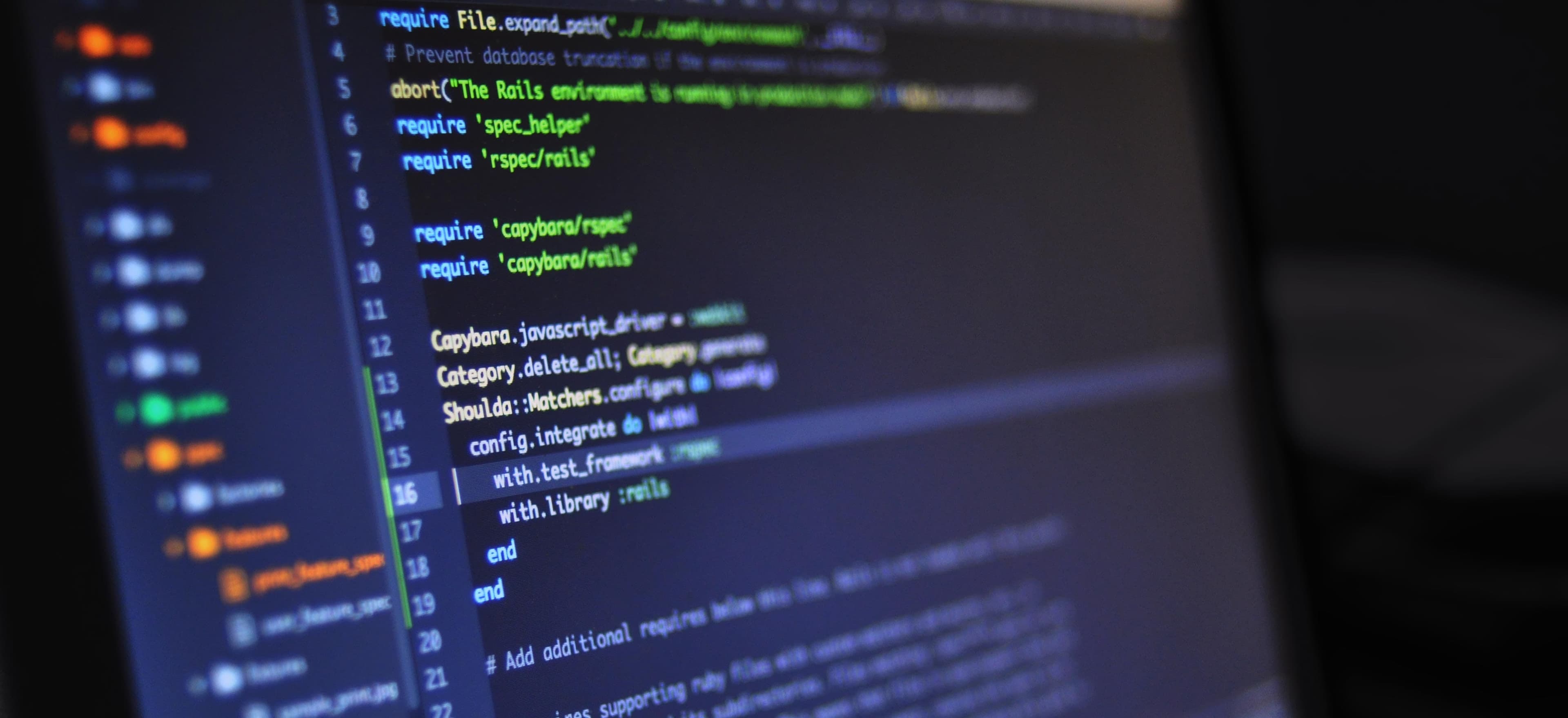
- Published on
Common RegEx Pitfalls in Java: Avoid These Mistakes!
Regular Expressions (RegEx) in Java are powerful tools for pattern matching and text manipulation. They allow developers to validate input, search through strings, and even split data efficiently. However, despite their power, many developers stumble into common pitfalls when using RegEx. This post will explore these mistakes, provide code snippets for better understanding, and offer solutions to help you navigate Java's RegEx world effectively.
What is Regular Expression?
A Regular Expression (RegEx) is a sequence of characters that forms a search pattern. This pattern is often used for string searching algorithms, data validation, and parsing. In Java, RegEx is integrated into the language through the java.util.regex
package.
Basic Components of RegEx
- Literals: Simple characters that match themselves, like
abc
matches the string "abc". - Metacharacters: Special characters that represent classes of characters or positions, such as
.
(any character),^
(start of a string), and$
(end of a string). - Quantifiers: Define how many instances of a character or group can occur, like
*
(zero or more) or+
(one or more). - Character Classes: Defined using brackets, such as
[abc]
which matches either 'a', 'b', or 'c'.
Mistake 1: Ignoring Escape Characters
One of the most common pitfalls is not escaping special characters correctly. In Java regular expressions, you'll often use backslashes, which also serve as escape characters in Java strings.
Example
String regex = "\\d+"; // Matches one or more digits
String input = "There are 123 apples.";
if (input.matches(".*" + regex + ".*")) {
System.out.println("Found digits in the input.");
}
Why:
If you wrote it as "\d+"
, it would fail; Java interprets \
as an escape character, causing an error.
Mistake 2: Overusing Greedy Matching
Greedy quantifiers, such as *
and +
, match as much text as possible, which can lead to unexpected results.
Example
String regex = "a.*b"; // Greedy matching
String input = "a1b2a3b";
System.out.println(input.replaceAll(regex, "X")); // Outputs "X2a3b"
Why:
The regex matched the first 'a' to the last 'b'. If you want to match the closest 'b', you need to use non-greedy (lazy) quantifiers like .*?
.
Fixing Greedy Matching
String regex = "a.*?b"; // Non-greedy matching
System.out.println(input.replaceAll(regex, "X")); // Outputs "X3b"
Mistake 3: String Presumption
A common mistake is assuming that string methods will behave similarly across contexts, especially with input types.
String input = "123abc456";
String regex = "[0-9]+"; // Matches one or more digits
System.out.println(input.replaceAll(regex, "X")); // Outputs "XabcX"
For the above use case, the output may seem intuitive but can lead to confusion if you do not clearly understand the RegEx.
Advanced Example
If you want to replace just the first sequence of digits:
String input = "123abc456";
String regex = "[0-9]+";
System.out.println(input.replaceFirst(regex, "X")); // Outputs "Xabc456"
Mistake 4: Confusing Character Sets and Caret
Character classes are defined using brackets [ ]
, but many forget that ^
inside them negates the class.
Example
String regex = "[^a-z]"; // Matches anything that is not a lowercase letter
String input = "Hello123!";
System.out.println(input.replaceAll(regex, "X")); // Outputs "XXXXXX"
Why:
While [a-z]
matches lowercase letters only, [^a-z]
will match everything that is not a lowercase letter. Be cautious with this distinction.
Mistake 5: Misusing Flags
Java RegEx supports flags which change pattern behavior, such as ignoring case with Pattern.CASE_INSENSITIVE
.
Example
String input = "hello";
String regex = "HELLO";
if (input.matches("(?i)" + regex)) {
System.out.println("Match Found!"); // Outputs "Match Found!"
}
Why:
Using the (?i)
flag allows for case-insensitivity in the matching process.
Alternative Flag Usage
You can also compile a pattern with flags:
Pattern pattern = Pattern.compile(regex, Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(input);
if (matcher.matches()) {
System.out.println("Match Found!"); // Outputs "Match Found!"
}
Mistake 6: Not Testing Regular Expressions
Finally, testing your RegEx can save you from many common pitfalls. Many developers jump straight into coding without verifying their expressions.
Recommended Tool
Use regex101.com to build and test your regular expressions before integrating them into your Java code. This tool provides immediate feedback and shows detailed explanations for each component of your RegEx.
Bringing It All Together
Regular expressions in Java are incredibly powerful for string manipulation and validation. However, developers frequently encounter pitfalls that can confuse the correct pattern-making process. By avoiding escaping issues, greedy matching, misused character sets, and overlooking flags, you can write more robust and efficient RegEx.
Make sure to take the time to test your regular expressions effectively and consider using communities such as Stack Overflow for additional input and tips. This blog post is just a starting point; continue exploring the vast capabilities of RegEx to enhance your Java programming skills!
For more extensive reading, feel free to check out the following resources:
- Java Regular Expressions Official Documentation
- Comprehensive Guide to Java Regex
By keeping these pitfalls in mind and learning from each mistake, you'll be on your way to mastering RegEx in Java!