Mastering Java Lambda Syntax: Overcoming Common Confusions
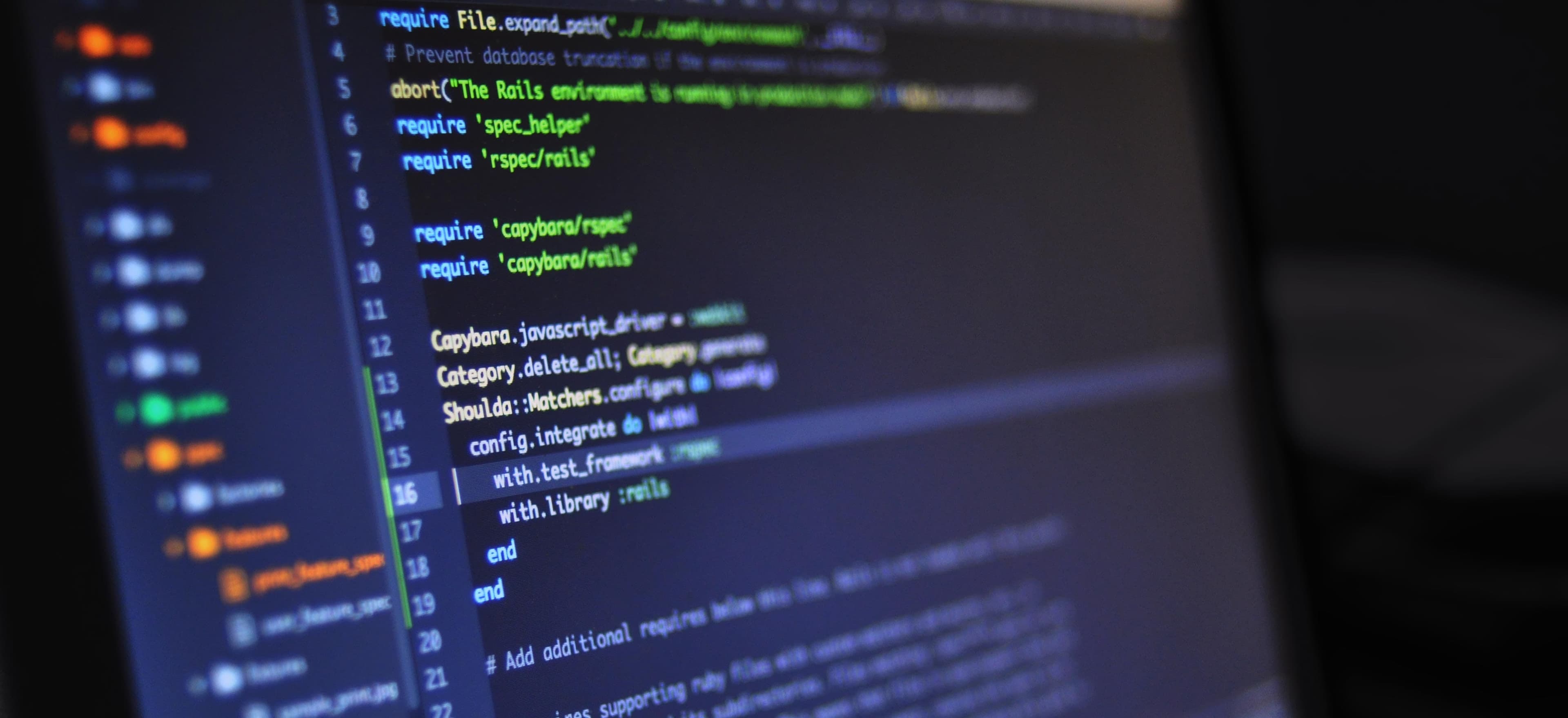
- Published on
Mastering Java Lambda Syntax: Overcoming Common Confusions
Java has undergone a significant transformation since the introduction of lambda expressions in Java 8. These powerful tools enable developers to write more concise and readable code. However, many programmers are still grappling with the nuances of this syntax. In this blog post, we'll demystify Java lambda expressions, address common confusions, and provide clear examples that underscore their practical applications.
What Are Lambda Expressions?
At its core, a lambda expression is a concise way to represent a function interface (an interface with only one abstract method). This allows you to treat functionality as a method argument, enabling you to pass behavior. The syntax is compact, making your code cleaner and more manageable.
Basic Syntax
The general syntax of a lambda expression is as follows:
(parameters) -> expression
Or, when there are multiple statements:
(parameters) -> { statements; }
Example
Here’s a simple example to kick things off:
(int a, int b) -> a + b
This expression represents a function that takes two integers and returns their sum.
Why Use Lambda Expressions?
Before we dive deeper, let’s explore why lambda expressions are worth mastering. Here are a few advantages:
- Reduced Boilerplate Code: Lambda expressions help eliminate the need for anonymous classes.
- Improved Readability: Code is often easier to read and understand.
- Enhanced Functionality: They allow you to use functional programming paradigms like
map
,filter
, andreduce
in collections.
Example with Collections
Consider a list of names from which you want to filter only those that start with 'J'.
Before Java 8:
Here you’d use an anonymous class:
List<String> names = Arrays.asList("John", "Jane", "Paul", "Rita");
List<String> jNames = new ArrayList<>();
for (String name : names) {
if (name.startsWith("J")) {
jNames.add(name);
}
}
With Java 8 Lambda:
The same operation can be accomplished in a more succinct manner:
List<String> jNames = names.stream()
.filter(name -> name.startsWith("J"))
.collect(Collectors.toList());
In this example, you can see how lambda expressions streamline the process.
Common Confusions
1. Parameter Type Inference
One common pitfall developers encounter is figuring out parameter types. While it's conventional to explicitly declare types, Java’s type inference can handle this for you.
Example with Type Inference:
List<String> names = Arrays.asList("John", "Jane", "Paul", "Rita");
names.forEach(name -> System.out.println(name));
In this case, you don’t need to specify the type of name
since it's inferred from the context.
2. Multiple Parameters
When working with multiple parameters, the parentheses around the parameters are obligatory only if you have more than one or if the parameters are of different types.
Single Parameter Example:
list.forEach(s -> System.out.println(s));
Multiple Parameters Example:
BiFunction<Integer, Integer, Integer> add = (a, b) -> a + b;
System.out.println(add.apply(5, 3)); // Outputs: 8
3. Implicit Return
Lambdas that consist of a single expression can omit the return
keyword entirely. This can lead to confusion, especially for newcomers.
Example:
Function<Integer, Integer> square = x -> x * x;
Contrast this with a block statement:
Function<Integer, Integer> square = x -> {
return x * x;
};
Both examples produce the same result, but the former is more concise.
4. Method References
Method references are a shorthand notation of a lambda expression to call a method. They are often advantageous when you have existing methods that can be used as is.
Example:
List<String> names = Arrays.asList("John", "Jane", "Paul", "Rita");
names.forEach(System.out::println);
Using System.out::println
is equivalent to using a lambda expression like name -> System.out.println(name)
, making your code cleaner.
Practical Use Cases of Lambda Expressions
1. Sorting with Custom Comparators
Lambda expressions can serve as powerful comparators, especially when sorting collections based on particular attributes.
Example:
List<String> names = Arrays.asList("John", "Jane", "Paul", "Rita");
Collections.sort(names, (a, b) -> a.length() - b.length());
System.out.println(names); // Outputs sorted by name length
2. Event Handling in GUI
In GUI programming, responses to events (like button clicks) can be defined using lambda expressions, making the implementation succinct.
Example:
Button button = new Button("Click me!");
button.setOnAction(event -> System.out.println("Button clicked!"));
3. Stream API for Complex Operations
The Java Stream API leverages lambda expressions for a plethora of data manipulation tasks ranging from filtering to grouping.
Example:
List<String> names = Arrays.asList("John", "Jane", "Paul", "Rita");
Map<Integer, List<String>> groupedByLength = names.stream()
.collect(Collectors.groupingBy(String::length));
Additional References
For further reading, check out these resources:
- Java Official Documentation on Lambda Expressions
- Functional Programming Concepts in Java
Closing the Chapter
Mastering Java lambda expressions is pivotal for modern Java programming. They not only simplify your code but also enhance its readability and maintainability. Despite some common confusions, by understanding the core principles and practicing examples, you can navigate these challenges with ease. As you familiarize yourself with this syntax, you'll unlock a new level of efficiency in your coding endeavors.
In summary, embrace the simplicity and power of lambda expressions in your Java applications. Happy coding!