Unlocking the Power of Dynamic Features in JAX-RS APIs
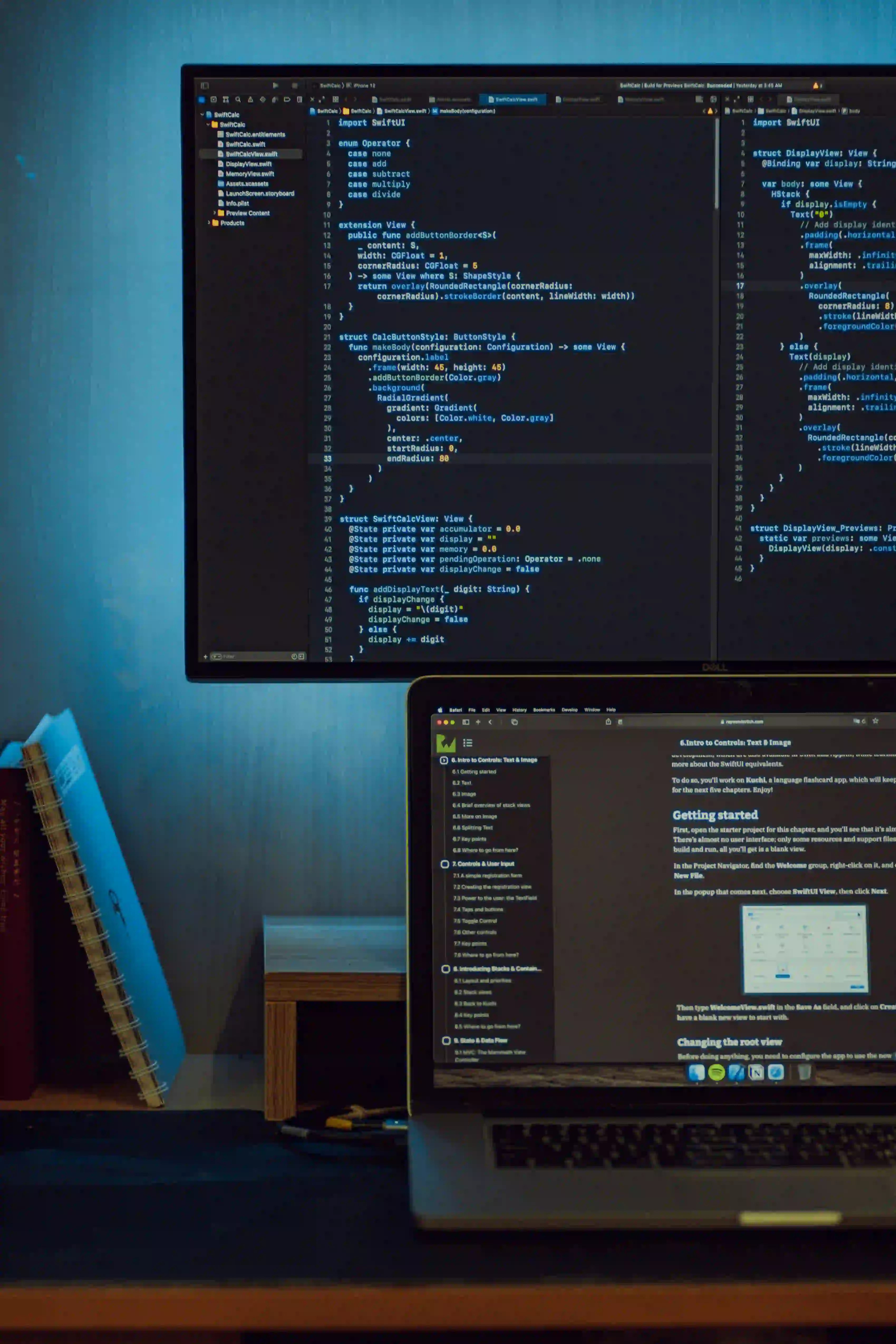
Unlocking the Power of Dynamic Features in JAX-RS APIs
When it comes to building RESTful web services in Java, JAX-RS has established itself as a standard for creating APIs with ease and efficiency. But did you know that JAX-RS allows you to customize and extend your API beyond static configurations? This is where Dynamic Features come into play.
Dynamic features in JAX-RS enable you to create flexible and modular applications that adapt to varying requirements at runtime. In this post, we will delve deep into dynamic features in JAX-RS, how they work, and when to use them.
What Are Dynamic Features?
Dynamic features are essentially components that can be registered with a JAX-RS application at runtime. This mechanism allows developers to apply modifications or enhancements to the API without needing to restart the application. It means that you can add, remove, or change the behavior of resource classes, filters, and interceptors on the fly.
Imagine a scenario where your API needs to adapt based on user roles or configurations that are loaded at runtime. Dynamic features make it possible to accommodate such changes seamlessly.
How Dynamic Features Work
JAX-RS architecture is designed to leverage a variety of components. Dynamic features add to this modularity, allowing you to define new behavior and augment existing functionality. Here’s how you can start using dynamic features:
- Creating a Dynamic Feature: A dynamic feature implements the
javax.ws.rs.core.Feature
interface. - Registering the Dynamic Feature: Once created, the feature is automatically discovered by JAX-RS during the application bootstrap.
- Modifying the Application: The feature can modify the JAX-RS application by adding resources or configuring existing components.
Example: Creating a Dynamic Feature
Let’s dive into a code example to see how we can create a simple dynamic feature that adds a custom filter.
Step 1: Add Dependencies
First, ensure that you have the necessary dependencies in your pom.xml
file if you are using Maven:
<dependency>
<groupId>javax.ws.rs</groupId>
<artifactId>javax.ws.rs-api</artifactId>
<version>2.1</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.35</version>
</dependency>
Step 2: Define a Custom Filter
Here, we define a simple HTTP filter that checks for authentication:
import javax.ws.rs.container.ContainerRequestContext;
import javax.ws.rs.container.ContainerResponseContext;
import javax.ws.rs.container.ContainerRequestFilter;
import javax.ws.rs.container.ContainerResponseFilter;
import javax.ws.rs.ext.Provider;
import javax.ws.rs.core.Response;
import java.io.IOException;
@Provider
public class AuthFilter implements ContainerRequestFilter, ContainerResponseFilter {
@Override
public void filter(ContainerRequestContext requestContext) throws IOException {
String authToken = requestContext.getHeaderString("Authorization");
if (authToken == null || !isValidToken(authToken)) {
requestContext.abortWith(Response.status(Response.Status.UNAUTHORIZED).entity("Unauthorized").build());
}
}
private boolean isValidToken(String token) {
// Authentication logic here
return "valid-token".equals(token);
}
@Override
public void filter(ContainerRequestContext requestContext, ContainerResponseContext responseContext) throws IOException {
responseContext.getHeaders().add("X-Powered-By", "MyAPI");
}
}
- The
AuthFilter
class checks for an authorization token and dismisses unauthorized requests. - If authenticated, it adds a custom header to the response.
Step 3: Create the Dynamic Feature
Now, let’s define a dynamic feature that registers this filter at runtime.
import javax.ws.rs.core.Feature;
import javax.ws.rs.core.FeatureContext;
import javax.ws.rs.core.Application;
import javax.ws.rs.core.Application;
import java.util.HashSet;
import java.util.Set;
public class MyDynamicFeature implements Feature {
@Override
public boolean configure(FeatureContext context) {
context.register(AuthFilter.class);
return true;
}
}
- The
MyDynamicFeature
class registers theAuthFilter
, thus enabling authentication checks.
Registering the Dynamic Feature
You’ll also need a main application class to register this dynamic feature:
import javax.ws.rs.ApplicationPath;
import javax.ws.rs.core.Application;
@ApplicationPath("/api")
public class MyApplication extends Application {
@Override
public Set<Class<?>> getClasses() {
Set<Class<?>> classes = new HashSet<>();
classes.add(MyDynamicFeature.class);
return classes;
}
}
Running the Application
You can now deploy this application and see it in action. When you make requests, the authentication filter will ensure that only valid requests are processed, showcasing the flexibility and power of dynamic features.
When to Use Dynamic Features
Dynamic features are extremely useful in various scenarios:
- Feature Toggles: You may want to enable or disable features based on application configuration or user roles.
- Modular APIs: Dynamic features support modular design approaches, allowing you to add functionalities as required.
- Custom Error Handling: Adding custom filters for logging or error handling can be done dynamically, enhancing the API’s resilience.
However, it's essential to be cautious when using dynamic features. Overusing them can lead to more complicated architecture and a harder-to-maintain codebase.
Best Practices
- Simplicity: Keep your dynamic features simple. If a feature requires significant complexity, consider redesigning it into a more static feature.
- Documentation: Document your dynamic features clearly. This will aid in understanding their roles and when to activate them.
- Testing: Dynamic features should be thoroughly tested, especially considering the changing components of the API.
Real-world Applications of Dynamic Features
For real-world applications adopting JAX-RS dynamic features, Payara is a worthy mention. Their implementation showcases how dynamic features can enhance extensibility and modular design in enterprise applications.
Moreover, tools like Swagger can also integrate with JAX-RS to provide documentation for dynamically generated endpoints.
Key Takeaways
Dynamic features in JAX-RS empower developers to create modular and adaptable RESTful APIs. By understanding and effectively utilizing dynamic features, you can embrace flexibility in your applications, simplifying the development and maintenance process.
Remember, with great power comes great responsibility. Use dynamic features thoughtfully, align them in accordance with your project's architecture, and keep performance in check. Happy coding!
By implementing the principles in this post, you can start unlocking the true potential of your JAX-RS APIs. The dynamic nature of features can significantly enhance your API’s adaptability and make your development journey a whole lot smoother. Explore, experiment, and enjoy the benefits of dynamic features today!