Mastering Java Time Zone Issues: A Beginner's Dilemma
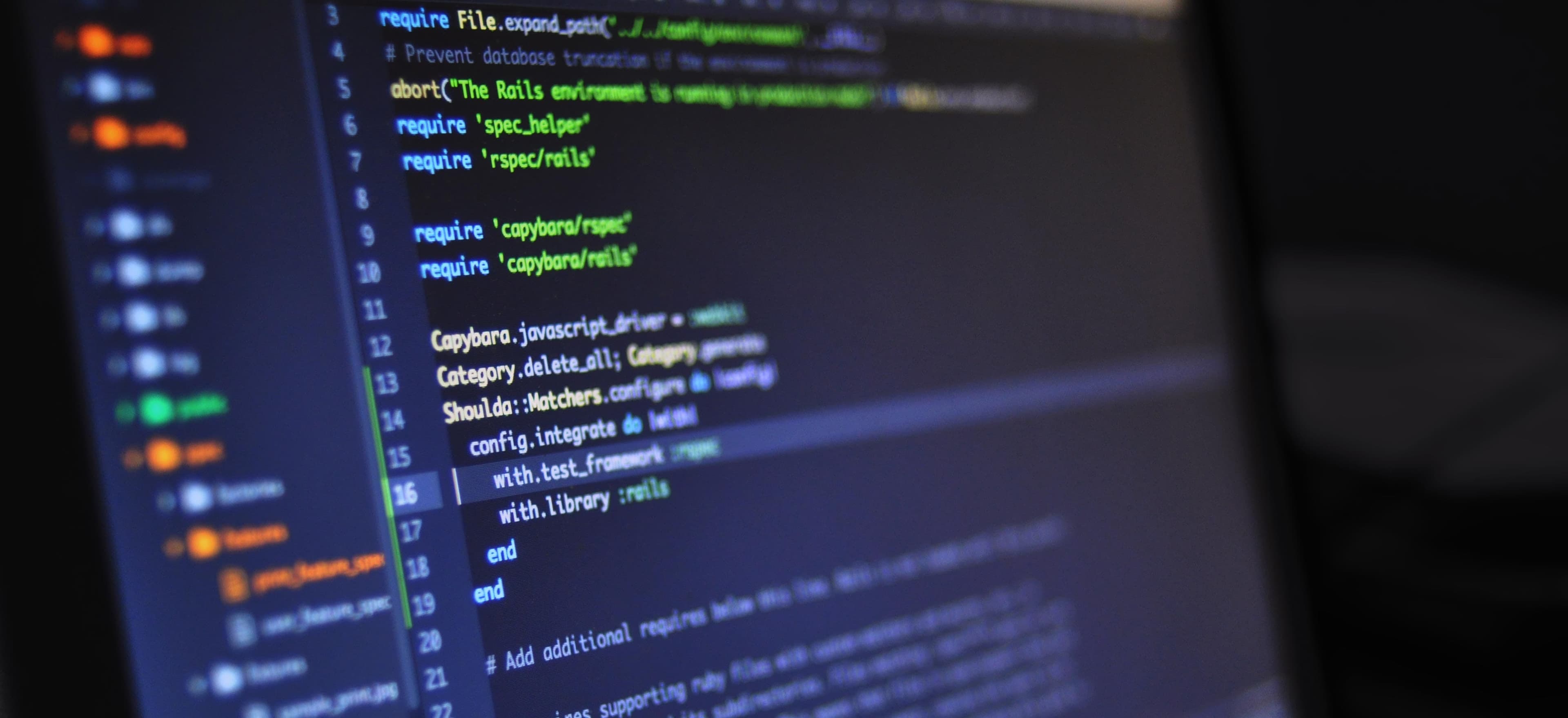
- Published on
Mastering Java Time Zone Issues: A Beginner's Dilemma
When working with applications that manage date and time data, understanding how to handle time zones can be a daunting task for many developers, especially beginners. In Java, dealing with time zones is not only crucial but can also be one of the trickiest areas to navigate.
This blog post aims to unravel the complexity surrounding Java time zones, providing clear explanations, code examples, and practical advice. By the end of this post, you'll have a better grasp of how to manage time zones effectively in your Java applications.
Understanding Time Zones
What is a Time Zone?
A time zone is a region of the Earth that has the same standard time. For example, when it's noon in New York (EST), it's 9 AM in Los Angeles (PST). There are many factors that play into when and how these time zones are established and adjusted, including the Earth's rotation and societal timerules.
Why Time Zones Matter
Time zones are critical in programming because they help display the correct time for users from different locations. Mismanaging time zones can lead to incorrect timestamps, problematic event scheduling, and general confusion in applications.
Java's Date and Time Library
Java has had multiple libraries for handling dates and times. Historically, java.util.Date
and java.util.Calendar
were widely used, but they are often criticized for being difficult to work with and not thread-safe. Fortunately, Java 8 introduced the java.time
package, which aligns better with the ISO-8601 calendar system, making time manipulation clearer and more robust.
Key Classes to Know
- ZonedDateTime: Represents a date-time with a time zone.
- ZoneId: Represents a time zone identifier, such as "America/New_York".
- LocalDateTime: Represents a date-time without a time zone.
- Instant: A moment on the timeline in UTC (Coordinated Universal Time).
The Importance of Time Zone in Java
Consider an application that schedules meetings across different time zones. Without proper handling, a meeting scheduled for 3 PM in New York could be misunderstood as 3 PM in Los Angeles, causing attendees to miss their meetings. Thus, a clear understanding of Java’s time handling methods is key to providing accurate scheduling tools.
Handling Time Zones in Java
Creating a ZonedDateTime
Let's delve into how to create an instance of ZonedDateTime
and convert it to a different time zone.
import java.time.ZonedDateTime;
import java.time.ZoneId;
public class TimeZoneExample {
public static void main(String[] args) {
// Create a ZonedDateTime instance for New York
ZonedDateTime newYorkTime = ZonedDateTime.now(ZoneId.of("America/New_York"));
System.out.println("New York time: " + newYorkTime);
// Convert to Los Angeles time
ZonedDateTime laTime = newYorkTime.withZoneSameInstant(ZoneId.of("America/Los_Angeles"));
System.out.println("Los Angeles time: " + laTime);
}
}
Explanation of the Code
- ZonedDateTime.now(ZoneId.of("America/New_York")): This line fetches the current date-time for New York (Eastern Time).
- withZoneSameInstant: Converts the time to another time zone (Los Angeles in this case), preserving the exact moment in time but adjusting it to the local time in Los Angeles.
Why Use ZonedDateTime?
The ZonedDateTime
class is ideal because it contains all the necessary components for time zone manipulation, including daylight saving time changes.
Working with LocalDateTime
There's a scenario where you want to store a date and time without referencing any time zone. Here's how to handle it effectively.
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
public class LocalDateTimeExample {
public static void main(String[] args) {
// Create a LocalDateTime instance
LocalDateTime localDateTime = LocalDateTime.now();
System.out.println("Local DateTime without time zone: " + localDateTime);
// Convert it to a ZonedDateTime in New York
ZonedDateTime zonedDateTime = localDateTime.atZone(ZoneId.of("America/New_York"));
System.out.println("Zoned DateTime in New York: " + zonedDateTime);
}
}
Key Takeaways
- LocalDateTime: Represents a date-time but is unaware of the time zone.
- atZone(): Converts a LocalDateTime to a ZonedDateTime based on the specified timezone.
Common Pitfalls and How to Avoid Them
-
Ignoring Time Zones: Always be mindful of the time zones when dealing with date and time. Use
ZonedDateTime
overLocalDateTime
when time zones matter. -
Daylight Saving Time: Be aware of regions that observe Daylight Saving Time. This alters the expected time offset in certain periods of the year.
-
UTC vs Local Time: When performing comparisons or storing dates, store them in UTC to minimize confusion, and convert them to local time only when displaying them to users.
Best Practices for Time Zone Management
-
Always Use ZonedDateTime: When dealing with times that should respect time zones, default to using
ZonedDateTime
instead ofLocalDateTime
. -
Store Dates as UTC: For databases or persistence layers, store timestamps in UTC and convert to local time when necessary.
-
Utilize Helper Methods: Create utility methods to handle common time zone conversions to reduce redundancy.
For deeper understanding, you can check Java Time Zone Documentation.
Testing Time Zone Logic
When writing unit tests involving time zones, ensure your tests account for various scenarios including:
- Different time zones.
- Daylight Saving Time changes.
- Leap seconds (if applicable).
Implement tests using libraries like JUnit to assert correct time zone calculations.
import java.time.ZonedDateTime;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class TimeZoneTest {
@Test
public void testTimeZoneConversion() {
ZonedDateTime newYorkTime = ZonedDateTime.parse("2023-04-01T15:00:00-04:00[America/New_York]");
ZonedDateTime laTime = newYorkTime.withZoneSameInstant(ZoneId.of("America/Los_Angeles"));
// Assert - Compare expected and actual values
assertEquals("2023-04-01T12:00:00-07:00[America/Los_Angeles]", laTime.toString());
}
}
Wrapping Up
Mastering Java time zone issues is essential for creating applications that operate accurately, especially in a global context. As you dive deeper into Java:
- Leverage the
java.time
package. - Create utility methods for common tasks.
- Always be mindful of the time zones involved.
By following the practices discussed in this post, you’ll reduce errors and enhance the user experience when dealing with dates and times across different regions.
For further reading and resources, you can explore Baeldung's Guide to Java Time.
Happy coding, and enjoy mastering the complexities of time zones in your Java applications!
Checkout our other articles