Securing User Sessions in EE Servlets: Common Pitfalls
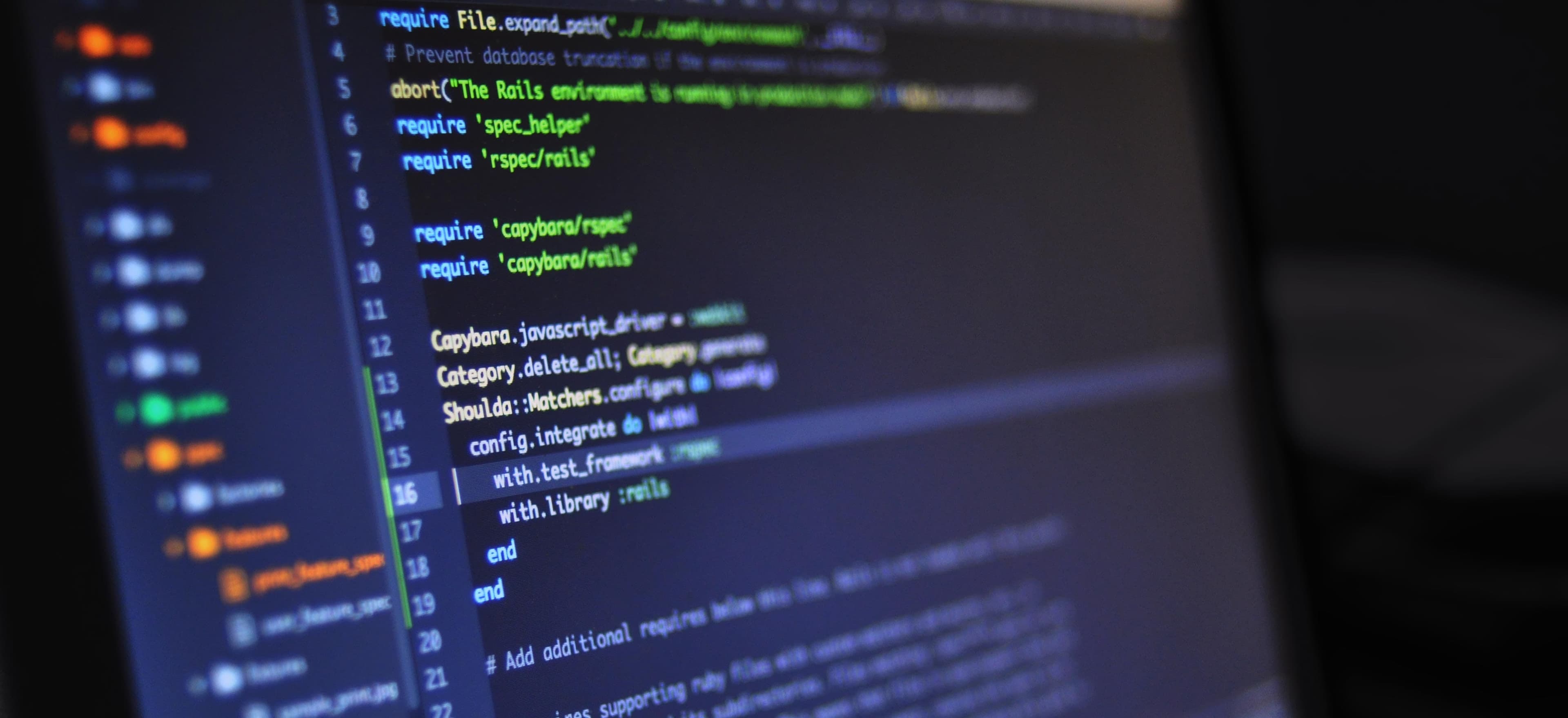
- Published on
Securing User Sessions in Enterprise Edition Servlets: Common Pitfalls
In today's digital landscape, ensuring the security of user sessions is paramount. For applications built using Java EE (Jakarta EE) Servlets, session management is a critical part of application security. Sessions maintain user identity through a sequence of interactions, and if compromised, can lead to unauthorized access, data breaches, and a host of other security issues.
This blog post will delve into common pitfalls associated with user session security in EE Servlets, providing actionable recommendations to avoid them. From improperly managing session timeouts to neglecting proper logout procedures, we'll cover essential best practices to bolster your application's defenses against common vulnerabilities.
Understanding the Basics of User Sessions
Before we dive into the pitfalls, it's essential to grasp what a user session entails in the context of Java EE Servlets. A session allows users to store information (attributes) associated with their actions on a server. This information is usually stored in a session object.
A session is initiated when a user accesses a web application, acquiring a unique session ID, which is generally stored in a cookie. The server uses this ID to retrieve stored session data.
To understand user session management better, let's examine a simplified session snippet.
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
public void createUserSession(HttpServletRequest request) {
HttpSession session = request.getSession(); // Create or get existing session
session.setAttribute("user", userObject); // Storing user's information
session.setMaxInactiveInterval(30 * 60); // Set session timeout for 30 minutes
}
Why is This Important?
- Session Object: The session object allows you to track user state.
- User Info: Storing user information in the session helps personalize the user experience.
- Timeout: Setting a timeout prevents unauthorized access when a user leaves their session idle.
Common Pitfalls in User Session Management
1. Inadequate Session Timeout Configuration
One of the most common errors is setting the session timeout too long or, conversely, not at all. A longer session timeout increases the risks of session hijacking.
Best Practice: Always set a reasonable session timeout period.
session.setMaxInactiveInterval(15 * 60); // Setting a timeout of 15 minutes
This practice helps mitigate the risks when a session is left active unintentionally.
2. Poor Session ID Management
By not regenerating session IDs after significant actions (like logging in), your application could be vulnerable to session fixation attacks. This occurs when an attacker sets a user’s session ID to one of their choosing.
Best Practice: Regenerate the session ID when a user logs in, using the HttpSession
API as shown below.
session.invalidate(); // invalidate the previous session
HttpSession newSession = request.getSession(true); // create new session
Regenerating the session ID not only creates a new session but also eliminates the older one, thus improving security.
3. Not Using Secure Cookies
Cookies are essential for session management, but they can be hijacked if not configured correctly. Cookies must be marked as secure and HTTPOnly to avoid being accessed through unsecured channels or via JavaScript.
Best Practice: Configure your cookies correctly.
Cookie userSessionCookie = new Cookie("JSESSIONID", session.getId());
userSessionCookie.setHttpOnly(true); // Prevents JavaScript from accessing cookie
userSessionCookie.setSecure(true); // Ensures cookie is always sent over HTTPS
response.addCookie(userSessionCookie);
Why is this important: Enforcing these security flags guards against cross-site scripting (XSS) attacks and man-in-the-middle attacks.
4. Failure to Implement Proper Logout Procedures
One frequently overlooked aspect is the implementation of a proper logout mechanism. Users may leave sessions open unintentionally, leading to unauthorized access.
Best Practice: Always provide users with a clear logout option.
public void logout(HttpServletRequest request, HttpServletResponse response) {
HttpSession session = request.getSession(false);
if (session != null) {
session.invalidate(); // End the session
}
// Redirect to login page or homepage
response.sendRedirect("login.jsp");
}
Ensure that all sensitive actions require authentication, enhancing security by preventing unauthorized data access.
5. Ignoring Cross-Site Request Forgery (CSRF) Protection
Neglecting CSRF protection can jeopardize session security. Attackers can exploit an authenticated session to perform unwanted actions on behalf of the user.
Best Practice: Implement CSRF tokens in your forms.
<form action="performAction" method="post">
<input type="hidden" name="csrfToken" value="<%= session.getAttribute("csrfToken") %>">
<!-- Other form fields -->
<button type="submit">Submit</button>
</form>
On server-side validation, ensure this token is checked against the session token to guarantee security.
6. Lack of HTTPS
Using HTTP instead of HTTPS exposes session cookies to a variety of spoofing attacks. Unencrypted data can be intercepted easily.
Best Practice: Always enforce HTTPS throughout your application.
Here’s a snippet to enforce HTTPS on all pages:
<security-constraint>
<web-resource-collection>
<web-resource-name>Protected Area</web-resource-name>
<url-pattern>/*</url-pattern>
</web-resource-collection>
<auth-constraint>
<role-name>USER</role-name>
</auth-constraint>
<transport-guarantee>CONFIDENTIAL</transport-guarantee>
</security-constraint>
Using HTTPS ensures that all communication between the server and client is encrypted, significantly bolstering session security.
Final Thoughts
In summary, session management is a fundamental component of user authentication and authorization within Java EE Servlets. By being aware of common pitfalls—such as improper timeout configurations, insecure cookie handling, and lack of proper logout procedures—you can enhance the security of your web application.
For developers keen on learning more about security measures in Java EE, additional readings such as OWASP's Java Security can provide deeper insights.
Remember, in an age where security breaches are increasingly common, investing time in proper session handling is not merely beneficial but essential. Keep your applications secure, and ensure user trust through diligent session management practices.
Stay tuned for more posts on Java EE best practices, and happy coding!
Checkout our other articles