Common Mistakes When Using Spring Initializr for Boot Projects
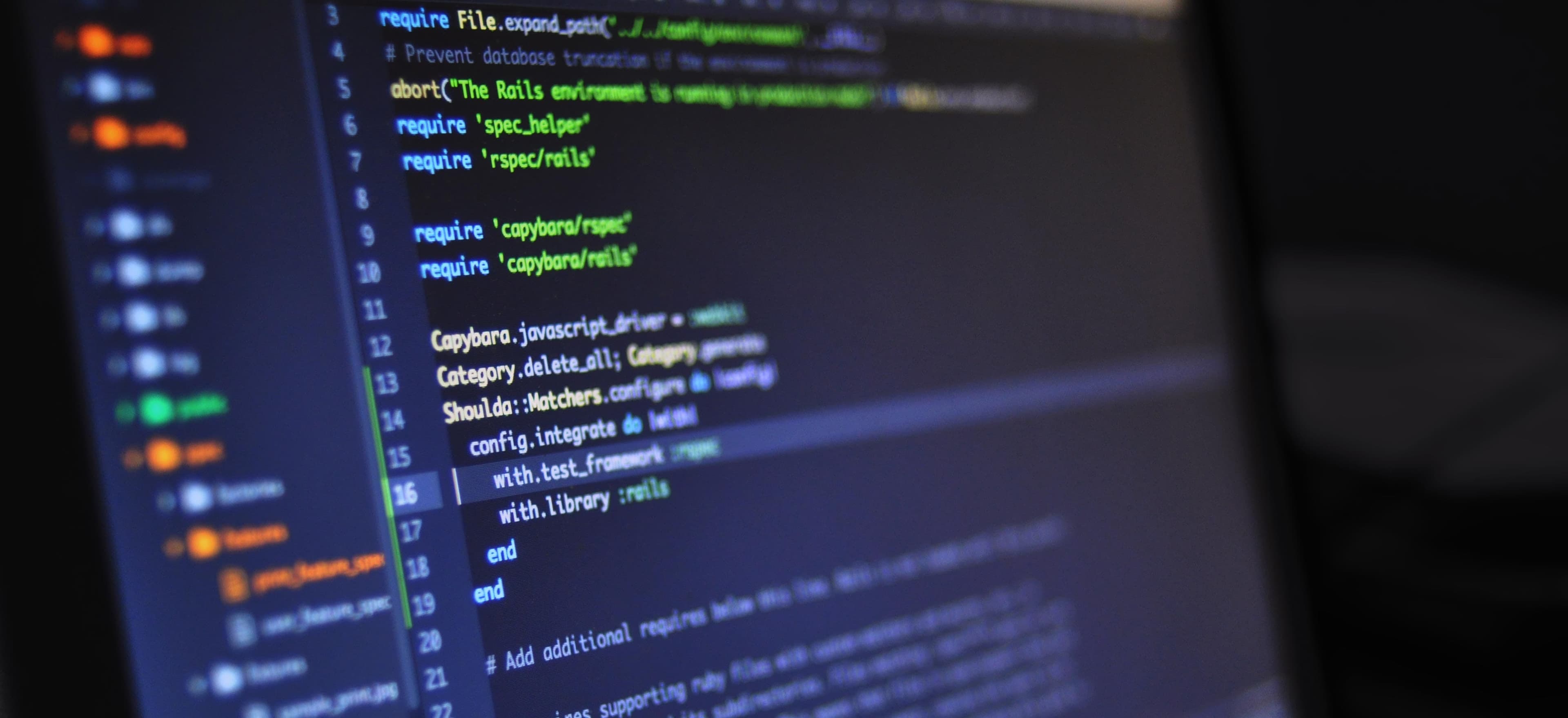
- Published on
Common Mistakes When Using Spring Initializr for Boot Projects
Spring Boot has revolutionized how Java developers build applications. The Spring Initializr is a powerful tool that simplifies the configuration of Spring Boot projects. However, despite its ease of use, developers can often stumble into common pitfalls. In this blog post, we'll explore these mistakes, how to avoid them, and provide best practices to ensure your Spring Boot applications are off to a great start.
What is Spring Initializr?
Before we dive into the common mistakes, let's first establish what Spring Initializr is. It is a web-based tool provided by Spring that allows developers to generate a Spring Boot project structure tailored to their needs. Users can select dependencies, project metadata, and more, which saves significant setup time.
To access Spring Initializr, you can visit start.spring.io. Here, you can choose the desired project settings and download a zipped project file.
Common Mistakes When Using Spring Initializr
1. Ignoring Dependency Management
Mistake: One of the most common mistakes developers make is ignoring the importance of selecting the right dependencies.
Solution: Carefully evaluate your application needs before choosing dependencies. Initially, it may seem tempting to choose all available dependencies. However, this can lead to bloated applications, unnecessary complexity, and longer startup times.
Example of Dependency Selection:
dependencies {
// Choose only what you need
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
implementation 'mysql:mysql-connector-java'
}
In this example, only essential dependencies such as web and data access are included. This keeps the project lightweight and more manageable.
2. Not Setting Java Version
Mistake: Another oversight is neglecting to specify the correct Java version while generating the project in Spring Initializr.
Solution: Always set the Java version that your project requires. This impacts the language features and libraries available to your application.
Example of Setting Java Version:
In Spring Initializr, look for the "Java version" dropdown and select the appropriate version, such as Java 11 or Java 17, which are among the currently popular choices for Spring Boot applications.
3. Overlooking Project Metadata
Mistake: Developers often gloss over the project's metadata fields such as Group, Artifact, and Name.
Solution: These fields provide vital information about your project. Take the time to fill them out accurately. Having a clear structure aids in identifying your project later, especially in larger environments.
Example of Project Metadata:
- Group: com.example.myapp
- Artifact: myapp
- Name: My Application
4. Skipping the Packaging Format
Mistake: Another error is neglecting to specify the packaging format, either JAR or WAR.
Solution: Select JAR for most microservices and standalone applications. Choose WAR if you intend to deploy on a servlet container.
Code Snippet Example:
// In build.gradle
plugins {
id 'org.springframework.boot' version '3.0.0'
id 'io.spring.dependency-management' version '1.0.12.RELEASE'
id 'java'
}
group = 'com.example.myapp'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '17'
jar {
enabled = true
}
This snippet specifies that your project will be packaged as a JAR file, enabling smooth execution as a Spring Boot application.
5. Insufficient Understanding of Autoconfiguration
Mistake: New developers often neglect understanding how Spring Boot's autoconfiguration feature works.
Solution: Familiarize yourself with how Spring Boot autoconfigures beans and settings based on the included dependencies. This knowledge is crucial for troubleshooting issues and effectively customizing your application.
Read more in the official Spring documentation: Spring Boot Autoconfiguration.
6. Using Default Values
Mistake: Many developers fall into the trap of relying solely on default values without adjusting the properties file to their needs.
Solution: Customize the application.properties
or application.yml
file as soon as your project is generated.
Example of application.properties:
# Server configuration
server.port=8081
# Database configuration
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=password
7. Failing to Include Testing Frameworks
Mistake: Often, testing dependencies are ignored during the initial setup, leading to difficulties in later stages of the project.
Solution: Include testing frameworks such as JUnit or Mockito from the outset. Incorporating testing early on paves the way for TDD (Test-Driven Development) or at least better coverage later.
Example of Testing Dependencies:
dependencies {
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
8. Not Familiarizing with the Directory Structure
Mistake: Once the project is generated, ignoring the directory structure can lead to chaos.
Solution: Understand the hierarchy of the generated project. Spring Boot projects typically use the following structure:
src/
└── main/
├── java/
│ └── com/
│ └── example/
│ └── yourapp/
└── resources/
└── application.properties
Each layer has a purpose; for example, resources/
is usually where your configuration files reside. Understanding this structure helps you maintain your project as it grows.
9. Not Using the Spring Boot Actuator
Mistake: Skipping the Spring Boot Actuator dependency.
Solution: Always consider adding Spring Boot Actuator, which provides vital insights into the application's metrics, health checks, and more.
Code Snippet:
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-actuator'
}
The Actuator will help you monitor your service and take necessary actions based on health metrics.
Final Thoughts
The Spring Initializr is an excellent tool that lays a solid foundation for your Spring Boot projects but it requires careful consideration of various elements to optimize your work. By avoiding these common mistakes and implementing best practices, you can create an efficient and maintainable application from the get-go.
For further exploration of Spring Boot features, consider visiting the official Spring Boot documentation to deepen your understanding.
By making informed choices in the setup stage, each of your Spring Boot projects can flourish into robust applications ready to tackle real-world requirements effectively. Happy coding!