Struggles in Auto-Formatting Large Codebases Explained
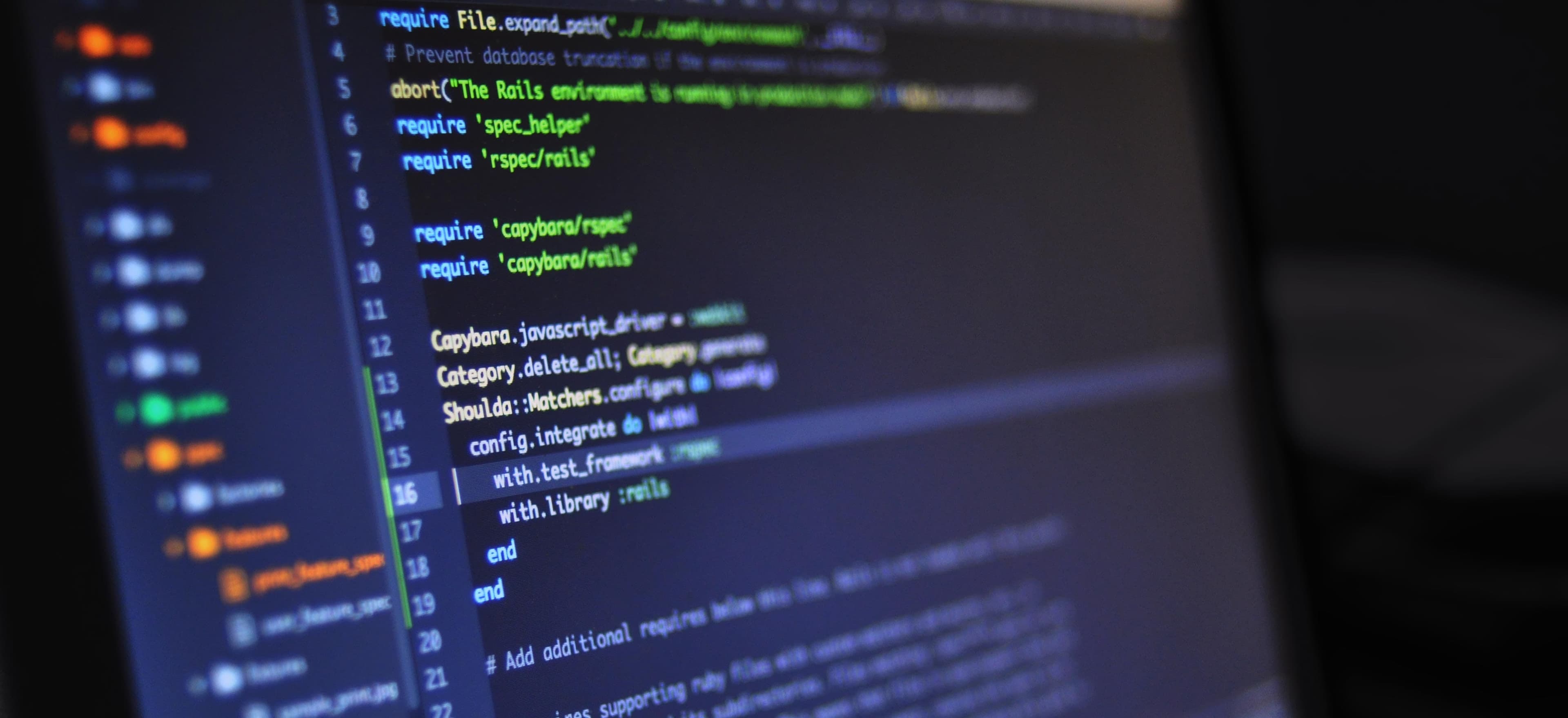
- Published on
Struggles in Auto-Formatting Large Codebases Explained
Programming is a craft that intricately weaves together logic, algorithms, and creativity. Developers often face challenges when it comes to maintaining clean, readable, and consistent code. One major aspect of this cleanliness comes from auto-formatting tools. While auto-formatting can significantly ease the pain of code style enforcement, it is not without its challenges—especially in large codebases.
In this article, we will explore the struggles associated with auto-formatting large codebases in Java. We'll discuss the common pitfalls, provide potential solutions, and illustrate key concepts with practical examples. By the end, you’ll better understand how to navigate the complexities of auto-formatting and why it is crucial in keeping your codebase healthy.
What is Auto-Formatting?
Auto-formatting refers to the process of automatically adjusting the layout and style of code according to predefined style guidelines. In Java development, this often involves adhering to popular standards such as Google Java Style or the Sun Code Conventions, which embrace whitespace management, naming conventions, and proper indentation.
The Benefits of Auto-Formatting
- Consistency: Auto-formatting ensures uniformity across your codebase, making it easier for developers to read and understand the code.
- Time-Saving: Developers spend less time manually adjusting code style, allowing more focus on functionality and optimization.
- Error Reduction: Proper formatting reduces the likelihood of bugs caused by misalignment or improper structure.
Common Struggles in Auto-Formatting Large Codebases
Despite the advantages, using auto-formatting tools in large codebases can present various struggles, as outlined below.
1. Conflicting Formatting Rules
One of the most common issues is conflicting formatting rules amongst team members. If developers have different local settings for formatting, auto-formatting can lead to unexpected results.
// Example of conflicting formatting
public class Example {
public void exampleMethod() {
int x=0;System.out.println(x);
}
}
In the scenario above, different developers might format the code differently based on their IDE settings. This inconsistency creates noise during code reviews and increases misunderstandings among team members.
Solution: Agree on a unified style guide and configure your IDEs to adhere to these standards. Tools like Checkstyle can help ensure compliance with your defined style guide.
2. Merge Conflicts Due to Formatting Changes
In a large team environment, auto-formatting can lead to increased merge conflicts in version control systems. When one developer formats a file differently from another and both changes are pushed to the same branch, a merge conflict occurs.
# Typical merge conflict output in Git
CONFLICT (content): Merge conflict in Example.java
This issue becomes increasingly cumbersome as the codebase grows.
Solution: To mitigate this problem, enforce auto-formatting before committing changes. Encouraging developers to run auto-formatting locally or during Continuous Integration (CI) could help reduce conflicts.
3. Performance Issues with Large Files
Auto-formatting tools may struggle with performance when applied to large files. This struggle can result in long wait times, hampering productivity.
// Large Java file example
public class LargeClass {
// Imagine hundreds of lines of code here
public void largeMethod() {
// ...
// The auto-formatting process could hang here if not optimized
// ...
}
}
Solution: Break down large files into smaller, more manageable components. This practice not only helps with formatting but also adheres to the Single Responsibility Principle, enhancing overall design.
4. Loss of Contextual Formatting
Auto-formatting tools can sometimes strip away comments, whitespace, or meaningful formatting. This loss can make the code harder to read and understand.
// Before auto-formatting
public class Example {
// This method adds two numbers
public int addNumbers(int a, int b) {
return a + b; // returns result
}
}
// After auto-formatting
public class Example {
public int addNumbers(int a,int b){return a+b;}
}
In the above example, auto-formatting leads to loss of context, especially if not configured well.
Solution: Choose intelligent formatting tools that can preserve contextual comments and whitespace. A well-configured formatter can significantly ease this issue.
Practical Steps to Implement Effective Auto-Formatting
To reap the benefits of auto-formatting while sidestepping its struggles, consider the following approaches:
1. Use IDE Built-in Functionality
Most Java IDEs like IntelliJ IDEA and Eclipse come with built-in formatting options. These tools allow developers to set a common formatting style across all team members, ensuring consistency.
Example in IntelliJ IDEA
To configure formatting in IntelliJ, follow these steps:
- Navigate to Preferences > Editor > Code Style > Java.
- Set your desired parameters for indentation, braces, and other stylistic conventions.
- Share the configuration file with your team.
2. Employ Build Tools with Formatting Capabilities
Integrate tools such as Maven or Gradle with plugins for auto-formatting checks. For instance, using the Maven Formatter Plugin will help automate the formatting during the build process.
Example Maven Configuration
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>formatter-maven-plugin</artifactId>
<version>2.16.0</version>
<executions>
<execution>
<goals>
<goal>format</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Incorporating such configurations ensures that code gets shaped according to predefined rules before deployment.
3. Automated Code Reviews
Automate your code review process using tools like SonarQube or Codacy. These tools can enforce coding standards and alert developers about formatting issues before code merges.
The Closing Argument
Auto-formatting is an efficient and practical way to maintain a clean codebase, particularly in large projects. By being aware of the common struggle areas, teams can employ proactive strategies to mitigate these challenges. From setting uniform formatting rules to leveraging automated tools, it's essential to establish a culture that emphasizes code quality.
In an environment where developers are constantly shipping code, auto-formatting should serve as an enabler of productivity, not a bottleneck. Through proper implementation, we can appreciate the elegance of well-structured code while minimizing potential struggles.
By following the guidelines discussed, developers can enhance collaboration and maintain a cohesive codebase easier. Remember that coding is not just about writing; it is also about reading and understanding the thoughts and intentions behind every line of code. Happy coding!