Simplifying Java Integration with PL/SQL: A jOOQ Guide
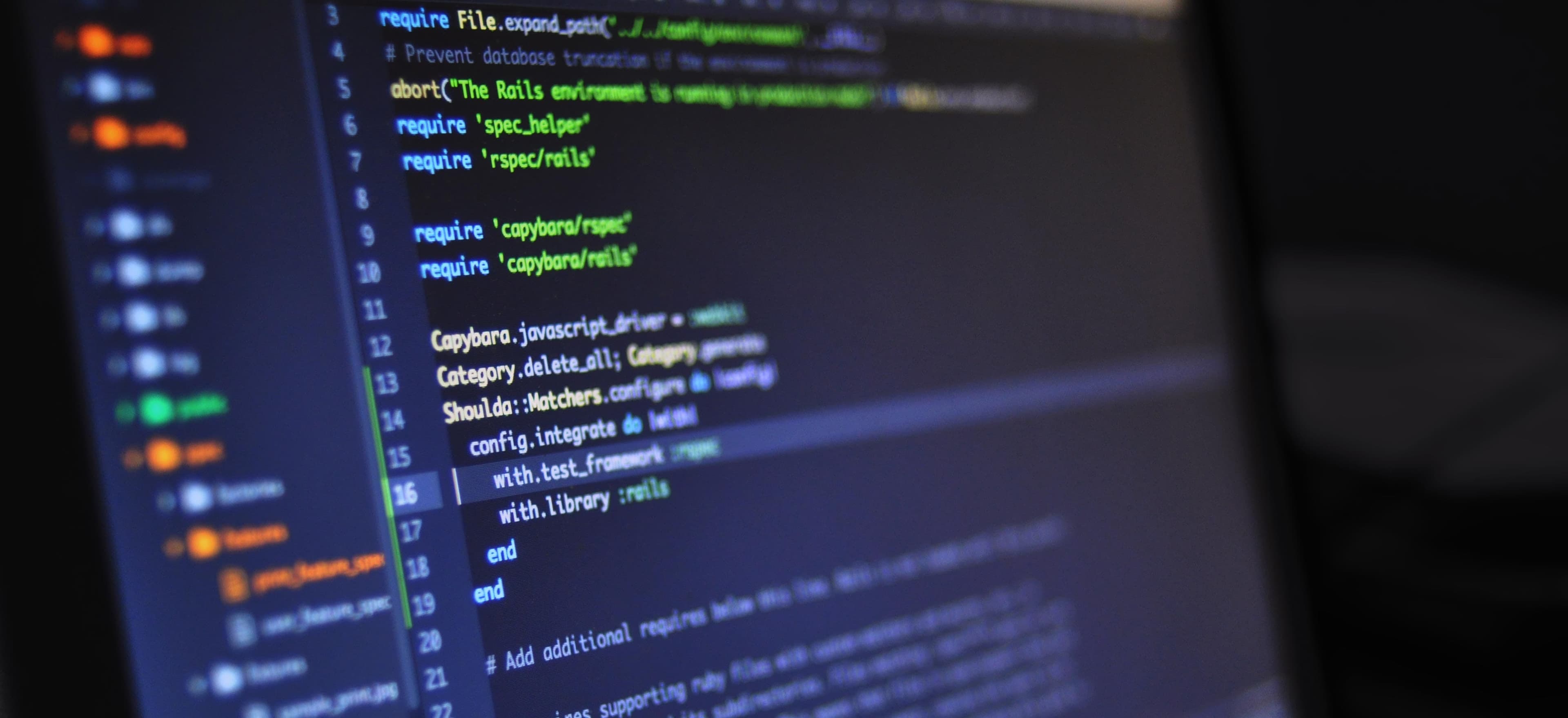
- Published on
Simplifying Java Integration with PL/SQL: A jOOQ Guide
In the dynamic world of software development, Java remains a stalwart language, particularly when interfacing with databases. One common scenario developers face is integrating Java with PL/SQL – the procedural extension of SQL found in Oracle databases. Traditionally, this task requires considerable boilerplate code. However, using libraries like jOOQ (Java Object Oriented Querying) can streamline the process considerably. This guide provides insight into how jOOQ simplifies Java integration with PL/SQL by elaborating on key concepts, code snippets, and best practices.
What is jOOQ?
jOOQ is a powerful library for building type-safe SQL query builders in Java applications. It allows you to write database interactions in a fluent style that closely resembles SQL while providing compile-time type safety. The mixing of Object-Oriented Programming with SQL brings forth a robust solution for application developers.
Why Use jOOQ for PL/SQL?
When thinking about integration with Oracle's PL/SQL, the benefits of using jOOQ become evident:
-
Type Safety: jOOQ generates Java code based on the database schema. This means you can catch any errors at compile time, preventing runtime SQL errors.
-
Fluency and Readability: jOOQ allows developers to write SQL queries in a fluent style, making the code more readable and maintainable.
-
Support for PL/SQL: jOOQ offers extensive support for stored procedures and functions written in PL/SQL, which is vital for many enterprise applications.
-
Database Independence: While jOOQ is tailored for specific SQL dialects, it also supports different database systems.
-
Integrated Code Generation: It can generate Java classes from your database schema, streamlining the process of interacting with your data.
Setting Up jOOQ
To get started with jOOQ, ensure you have the following prerequisites installed:
- Java Development Kit (JDK)
- Maven or Gradle for managing dependencies
- Oracle Database
Once the prerequisites are in place, include the jOOQ dependency in your Maven pom.xml
:
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq</artifactId>
<version>3.16.0</version>
</dependency>
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq-meta</artifactId>
<version>3.16.0</version>
</dependency>
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq-codegen</artifactId>
<version>3.16.0</version>
</dependency>
<dependency>
<groupId>org.oracle.jdbc</groupId>
<artifactId>ojdbc8</artifactId>
<version>19.8.0.0</version>
</dependency>
If you are using Gradle, your dependency block would look like this:
implementation 'org.jooq:jooq:3.16.0'
implementation 'org.jooq:jooq-meta:3.16.0'
implementation 'org.jooq:jooq-codegen:3.16.0'
implementation 'org.oracle.jdbc:ojdbc8:19.8.0.0'
Code Generation and Configuration
Before you can use jOOQ, you'll need to generate Java classes from your database schema. This can be done using the jOOQ code generation tool. Here’s a sample configuration that might be included in your Maven setup:
<build>
<plugins>
<plugin>
<groupId>org.jooq</groupId>
<artifactId>jooq-codegen-maven</artifactId>
<version>3.16.0</version>
<executions>
<execution>
<goals>
<goal>generate</goal>
</goals>
</execution>
</executions>
<configuration>
<jdbc>
<driver>oracle.jdbc.OracleDriver</driver>
<url>jdbc:oracle:thin:@localhost:1521:xe</url>
<user>your_username</user>
<password>your_password</password>
</jdbc>
<generator>
<database>
<name>org.jooq.meta.oracle.OracleDatabase</name>
<inputSchema>YOUR_SCHEMA</inputSchema>
</database>
<target>
<packageName>com.example.jooq.generated</packageName>
<directory>src/main/java</directory>
</target>
</generator>
</configuration>
</plugin>
</plugins>
</build>
Why Generate Code?
Generating Java classes from your database schema automates the tedious task of mapping between your database tables and your application models. It ensures that you work with up-to-date code, reducing discrepancies and potential human error.
Example jOOQ Queries with PL/SQL
Now let's examine how to execute PL/SQL stored procedures and functions using jOOQ. Here’s a simple example demonstrating how to call a PL/SQL stored procedure.
Defining a Stored Procedure
First, let’s say you have a simple PL/SQL procedure:
CREATE OR REPLACE PROCEDURE add_employee (
p_name IN VARCHAR2,
p_salary IN NUMBER,
o_id OUT NUMBER
) AS
BEGIN
INSERT INTO employees (name, salary) VALUES (p_name, p_salary)
RETURNING id INTO o_id;
END add_employee;
Calling the Stored Procedure with jOOQ
You can call this procedure in jOOQ as follows:
import org.jooq.*;
import org.jooq.impl.DSL;
import org.jooq.impl.DefaultDSLContext;
import static org.jooq.impl.DSL.*;
import static com.example.jooq.generated.tables.Employees.*;
public class JooqExample {
public static void main(String[] args) {
// Create a DSLContext using the connection (replace this with your actual connection)
DSLContext dsl = DSL.using(SQLDialect.ORACLE, connection);
// Prepare the output variable
Long employeeId = null;
// Calling the PL/SQL procedure
dsl.connection(connection -> {
CallableStatement cs = connection.prepareCall("{call add_employee(?, ?, ?)}");
cs.setString(1, "John Doe");
cs.setDouble(2, 75000.00);
cs.registerOutParameter(3, Types.BIGINT);
cs.execute();
employeeId = cs.getLong(3);
return null;
});
System.out.println("Inserted Employee ID: " + employeeId);
}
}
Commentary on the Code
-
DSLContext: This is the central entry point for jOOQ operations. It provides fluent and type-safe ways to build SQL queries.
-
CallableStatement: We are using a
CallableStatement
to call the PL/SQL procedure. It allows passing input and output parameters. -
Registering Out Parameters: This enables capturing output from the procedure.
-
Executing: The execution of the procedure is straightforward and reads like regular Java code.
Handling SQL Exceptions
Always ensure to handle SQL exceptions gracefully. jOOQ makes this easier with its robust exception handling:
try {
// ... code to call stored procedure
} catch (DataAccessException e) {
System.err.println("Error accessing the database: " + e.getMessage());
}
Working with PL/SQL Functions
The integration doesn't stop at procedures. You can also integrate PL/SQL functions seamlessly. Here's how:
Defining a PL/SQL function
CREATE OR REPLACE FUNCTION get_employee_name (
p_id IN NUMBER
) RETURN VARCHAR2 IS
v_name VARCHAR2(100);
BEGIN
SELECT name INTO v_name FROM employees WHERE id = p_id;
RETURN v_name;
END get_employee_name;
Calling the PL/SQL Function
You can call this function in jOOQ as follows:
String employeeName = dsl.select(inline(1))
.from(table(name("get_employee_name")))
.fetchOne().value1();
System.out.println("Employee Name: " + employeeName);
Key Takeaways
-
Simplicity: jOOQ simplifies calling complex PL/SQL code.
-
Flexibility: You can adapt to changing database schemas without heavy refactoring.
-
Type Safety: Enjoy peace of mind knowing your SQL queries are checked at compile time.
Best Practices
-
Keep It Modular: Maintain separate modules for database operations to enhance maintainability.
-
Reuse DSLContext: Create a singleton for
DSLContext
to avoid connection overheads. -
Code Generation: Regularly regenerate your jOOQ classes whenever changes to the database schema occur.
-
Test Thoroughly: PL/SQL procedures often interact with multiple tables. Ensure integration tests cover these interactions.
-
Error Handling: Implement comprehensive error handling to manage exceptions gracefully.
Closing the Chapter
Integrating Java with PL/SQL might seem daunting at first, but tools like jOOQ can significantly simplify the process. By enhancing type safety, readability, and maintainability, jOOQ empowers developers to focus on building robust applications rather than wrestling with SQL syntax.
With jOOQ, you can easily handle stored procedures, functions, and complex queries while benefiting from Java's strong type-checking capabilities. By following the guidelines and best practices outlined in this guide, you can effectively harness the power of jOOQ in your Java applications integrating with Oracle's PL/SQL.
Explore more about jOOQ and its features on the official jOOQ documentation. Happy coding!