Common Pitfalls of the Singleton Design Pattern
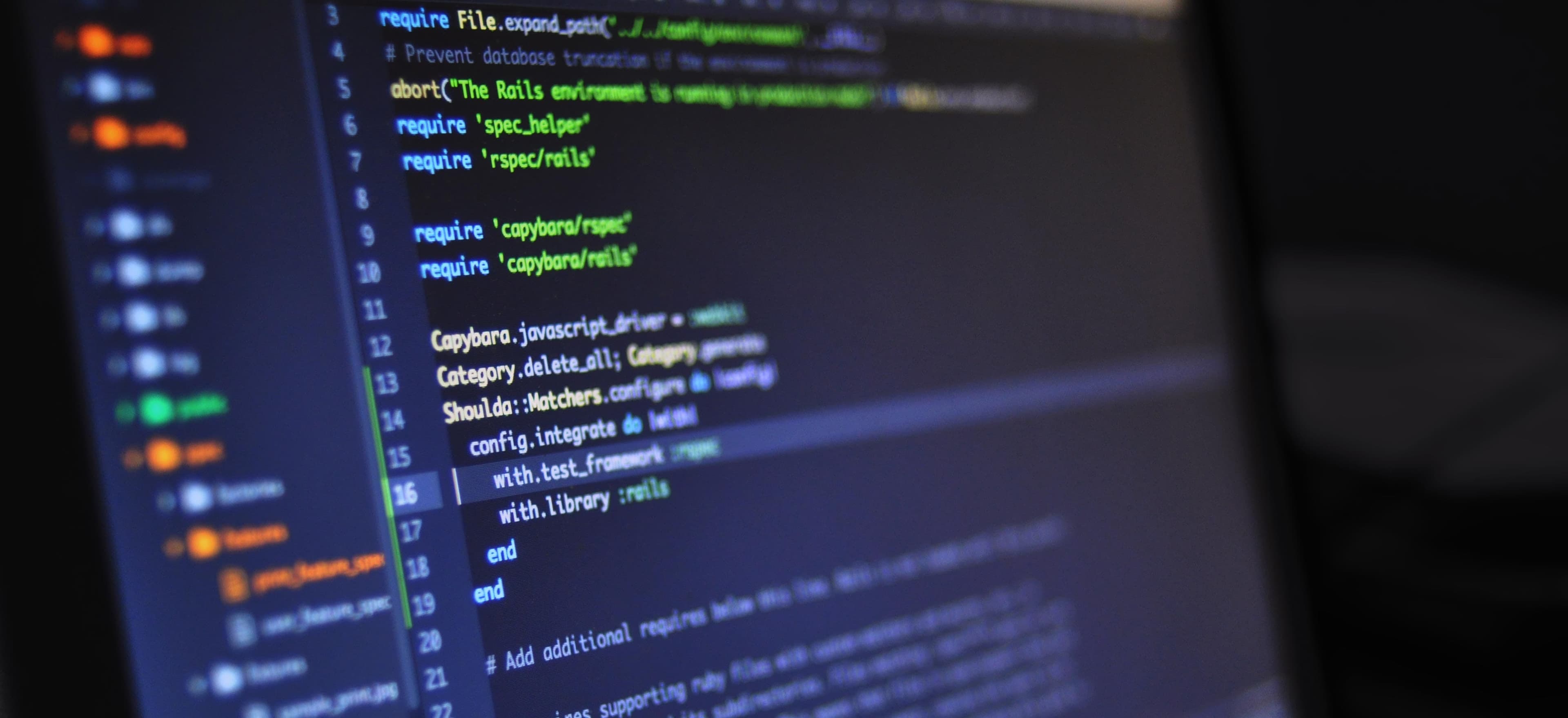
- Published on
Common Pitfalls of the Singleton Design Pattern
The Singleton design pattern is a familiar and often-used pattern in software engineering, particularly in Java. It restricts a class to a single instance and provides a global point of access to it. However, while writing a Singleton class, developers can easily fall into various pitfalls that can lead to inefficiencies, bugs, and less maintainable code. This blog post discusses common pitfalls associated with the Singleton pattern in Java, accompanied by code examples and suggestions for preventing these issues.
Not Handling Thread Safety
One of the primary pitfalls of the Singleton pattern is the lack of thread safety. In a multi-threaded environment, if two threads try to instantiate the Singleton simultaneously, two different instances could be created.
Example of a Non-Thread-Safe Singleton
public class NonThreadSafeSingleton {
private static NonThreadSafeSingleton instance;
private NonThreadSafeSingleton() {}
public static NonThreadSafeSingleton getInstance() {
if (instance == null) {
instance = new NonThreadSafeSingleton();
}
return instance;
}
}
Why This Is Problematic
If two threads execute getInstance
at the same time, they may both find instance
to be null and create separate instances of NonThreadSafeSingleton
. To ensure that only one instance of the class is created, we need to manage access using synchronization.
Solution: Thread-Safe Singleton
The simplest solution to ensure thread safety is to synchronize the method that returns the instance.
public class ThreadSafeSingleton {
private static ThreadSafeSingleton instance;
private ThreadSafeSingleton() {}
public static synchronized ThreadSafeSingleton getInstance() {
if (instance == null) {
instance = new ThreadSafeSingleton();
}
return instance;
}
}
Java's synchronized
keyword prevents multiple threads from executing the method at the same time, thus preventing the creation of multiple instances. It, however, comes at the cost of performance due to the overhead of managing synchronization.
Double-Checked Locking
For better performance, you can use the Double-Checked Locking pattern, which checks if the instance is null before entering a synchronized block.
Example of Double-Checked Locking
public class DoubleCheckedLockingSingleton {
private static volatile DoubleCheckedLockingSingleton instance;
private DoubleCheckedLockingSingleton() {}
public static DoubleCheckedLockingSingleton getInstance() {
if (instance == null) {
synchronized (DoubleCheckedLockingSingleton.class) {
if (instance == null) {
instance = new DoubleCheckedLockingSingleton();
}
}
}
return instance;
}
}
Why This Works
Here, the instance is checked both outside and inside the synchronized block. The volatile
keyword is crucial as it ensures visibility of changes to variables across threads. The performance is significantly improved since synchronization is only used when the instance is null.
Not Using Lazy Initialization
Another common pitfall is invoking the Singleton instance eagerly. This means creating the instance at the time of class loading, regardless of whether it's needed.
Example of Eager Initialization
public class EagerInitializedSingleton {
private static final EagerInitializedSingleton instance = new EagerInitializedSingleton();
private EagerInitializedSingleton() {}
public static EagerInitializedSingleton getInstance() {
return instance;
}
}
Problems with Eager Initialization
This approach consumes resources even when the instance may not be used, making it inefficient in scenarios where the Singleton’s functionality is costly to execute.
Suggested Approach: Lazy Initialization
As discussed earlier with thread-safe variants, lazy initialization is a more optimal way to allocate resources only when necessary.
Forgetting to Override Clone
and Serialize
Methods
A dangerously overlooked issue is the ability to create clones or serialize the Singleton instance. If cloning is allowed by a Singleton class, it can lead to multiple instances, violating the very characteristics of a Singleton.
Example of Cloneable Singleton
public class ClonableSingleton implements Cloneable {
private static final ClonableSingleton instance = new ClonableSingleton();
private ClonableSingleton() {}
public static ClonableSingleton getInstance() {
return instance;
}
@Override
protected Object clone() throws CloneNotSupportedException {
throw new CloneNotSupportedException("Singleton can't be cloned");
}
}
Best Practice
Override the clone
method in your Singleton class to prevent cloning it. Similarly, when your class implements Serializable
, ensure that the readResolve
method is implemented to maintain the Singleton property.
private Object readResolve() {
return instance;
}
Misuse in Testing
Another issue arises when Singleton classes are intertwined with testing frameworks, where dependencies on Singletons can introduce hidden states, making tests unreliable.
Solution: Dependency Injection
One way to mitigate this is to utilize Dependency Injection. By designing your application to accept dependencies instead of creating them internally, your tests can easily mock or replace Singleton instances.
Key Takeaways
The Singleton pattern can be a powerful tool in the Java programmer's toolkit. However, understanding the common pitfalls can help avoid the frustrations that arise from improper use. Leveraging proper thread safety, applying lazy initialization, managing cloning and serialization, and considering testing strategies can lead to more robust implementations of the Singleton design pattern.
Further Reading
To learn more about the Singleton pattern and take a deeper dive into design patterns, you can explore the following resources:
Remember that design patterns, including the Singleton, should be used judiciously. Their primary function is to help create well-structured and maintainable code, but over-reliance can contribute to unnecessary complexity. Happy coding!