Overcoming PMD Customization Challenges in Eclipse
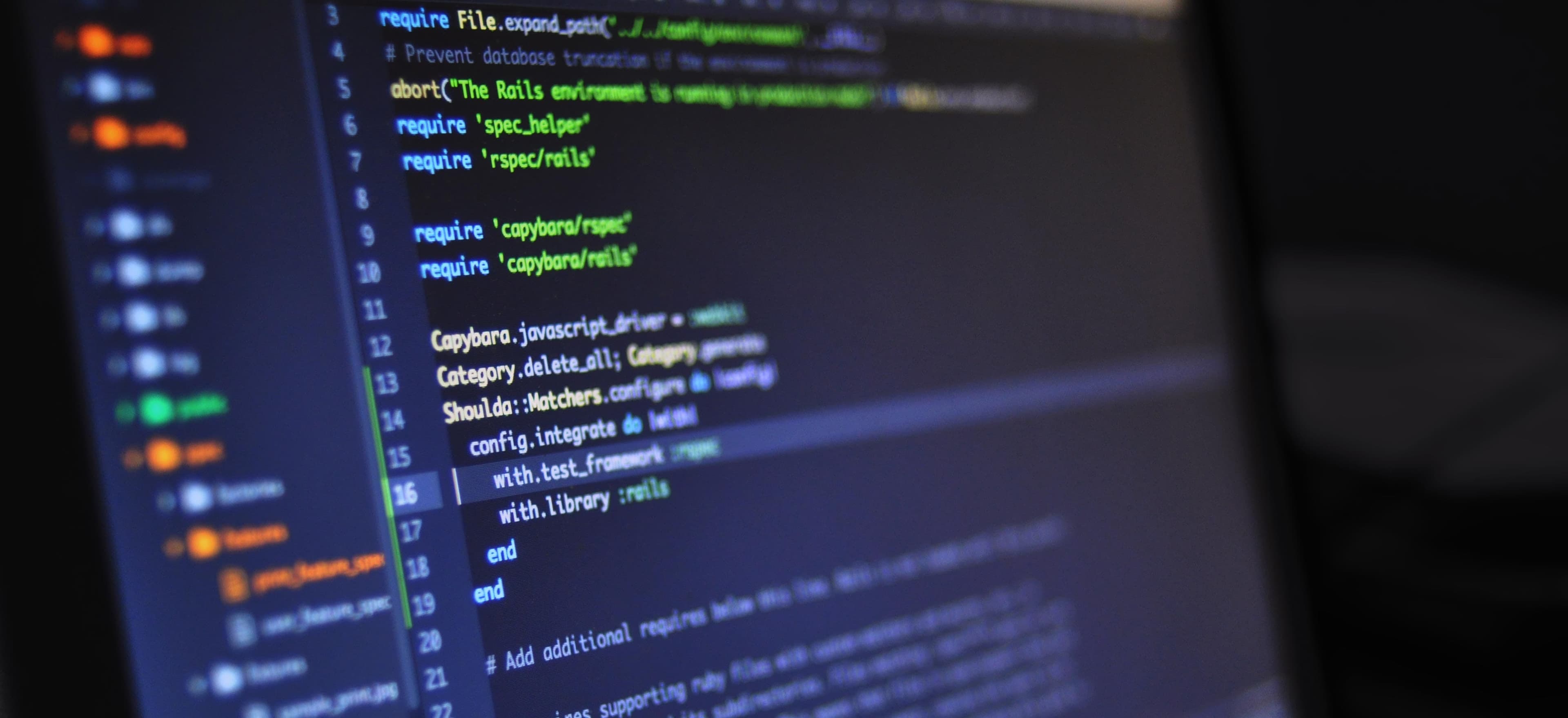
- Published on
Overcoming PMD Customization Challenges in Eclipse
The Starting Line
In the realm of software development, ensuring code quality is paramount. This is where PMD (Programming Mistake Detector) comes into play, helping developers identify and rectify issues in their Java code. However, customizing PMD to fit your project's specific needs can present a variety of challenges. This blog post will elucidate those challenges and provide practical solutions to overcome them, all while using the Eclipse IDE—a popular choice for Java developers.
What is PMD?
PMD is an open-source static code analysis tool that scans Java source files and identifies potential issues, such as code smells, bugs, and style violations. It uses a set of predefined rules to assess the code quality, making it essential for teams striving for high standards in software development.
Why Customize PMD?
While PMD comes equipped with a robust set of rules, each project has unique requirements. Customization allows you to:
- Focus on project-specific coding standards.
- Flag issues relevant to your codebase.
- Enhance team productivity by automating tedious reviews.
Common PMD Customization Challenges
1. Understanding the Rule Sets
PMD utilizes XML rule definitions, which can be intimidating for new users. The default rules may not cater to all your project’s specific needs.
Solution: Create Custom Rule Sets
You can create your own rule sets based on your project's requirements. For instance, if you want to enforce a maximum line length of 80 characters, you can define a custom rule.
Here's an example of how to define a custom rule:
<ruleset name="My Custom Rules" xmlns="http://pmd.sourceforge.net/ruleset_2_0.dtd">
<description>
Custom rules for our project.
</description>
<rule ref="category/java/design.xml/ExcessiveMethodLength">
<properties>
<property name="minimum" value="1"/>
<property name="maximum" value="10"/>
</properties>
</rule>
</ruleset>
Why this approach? Customizing PMD allows your team to align on standards that are specific to your coding practices.
2. Integration into Eclipse
Integrating PMD into Eclipse can be a cumbersome process for those unfamiliar with the tool.
Solution: Use the PMD Plugin for Eclipse
To integrate PMD, download and install the PMD Eclipse Plugin:
- Eclipse Marketplace: Go to Help → Eclipse Marketplace.
- Search for PMD: Type "PMD" and follow the prompts to install the plugin.
With it installed, you'll be able to access PMD features directly within the IDE.
Code Example: Running PMD through Eclipse
Once you have the PMD plugin set up, running a scan can be easily accomplished:
- Right-click on your project in the Project Explorer.
- Hover over "PMD" and select "Check Code."
By doing this, you initiate a scan. The results will appear in the PMD view, providing insights into your code’s quality.
Why is this beneficial? Direct integration with Eclipse streamlines your workflow and ensures immediate feedback, making it easier to fix issues as they arise.
Advanced Customization of PMD
1. Creating Custom Rules
Creating custom rules is essential for specific patterns in your code that PMD’s default rules might overlook.
A custom rule can be developed in Java by extending AbstractJavaRule
. Here’s a simple example:
package com.your.package;
import net.sourceforge.pmd.lang.java.rule.AbstractJavaRule;
import net.sourceforge.pmd.lang.java.ast.ASTMethodDeclaration;
public class NoPublicMethodsRule extends AbstractJavaRule {
@Override
public Object visit(ASTMethodDeclaration node, Object data) {
if (node.isPublic()) {
addViolation(data, node);
}
return super.visit(node, data);
}
}
Why create custom rules? You are addressing specific needs and inconsistencies that aren't catered to by existing PMD rules.
2. Testing Your Custom Rules
Testing is crucial to ensure that your custom rules work as expected.
Use PMD’s built-in testing framework to write unit tests for your rules:
public class NoPublicMethodsRuleTest extends RuleTst {
public void testPublicMethod() {
Rule rule = new NoPublicMethodsRule();
// This method should trigger the rule violation if it's public
assertViolations(rule,
"public void shouldTriggerViolation() { }",
1);
}
}
Why test your custom rules? It guarantees that your rules accurately capture violations and maintain the integrity of your codebase.
Incorporating PMD into Your Build Process
1. Automate PMD with Maven
Integrate PMD into your build lifecycle to ensure code quality isn't overlooked in production. Use the PMD Maven Plugin:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-pmd-plugin</artifactId>
<version>3.14.0</version> <!-- Check for the latest version -->
<configuration>
<rulesets>
<ruleset>/path/to/your/custom-ruleset.xml</ruleset>
</rulesets>
</configuration>
</plugin>
Why automate? It ensures that PMD checks are consistently applied, reducing the chances of introducing problematic code.
Final Thoughts
Customizing PMD in Eclipse can undoubtedly be challenging, but by understanding its rule sets, properly integrating the plugin, creating custom rules, and automating the process, these challenges can be effectively overcome.
For further reading on static code analysis tools, check out SonarQube's Documentation and PMD's Official Documentation.
Apply the techniques presented here in your projects to not only enhance code quality but also to improve overall team productivity. Happy coding!
Checkout our other articles