Common Pitfalls in Spring Boot Integration Testing with Selenium
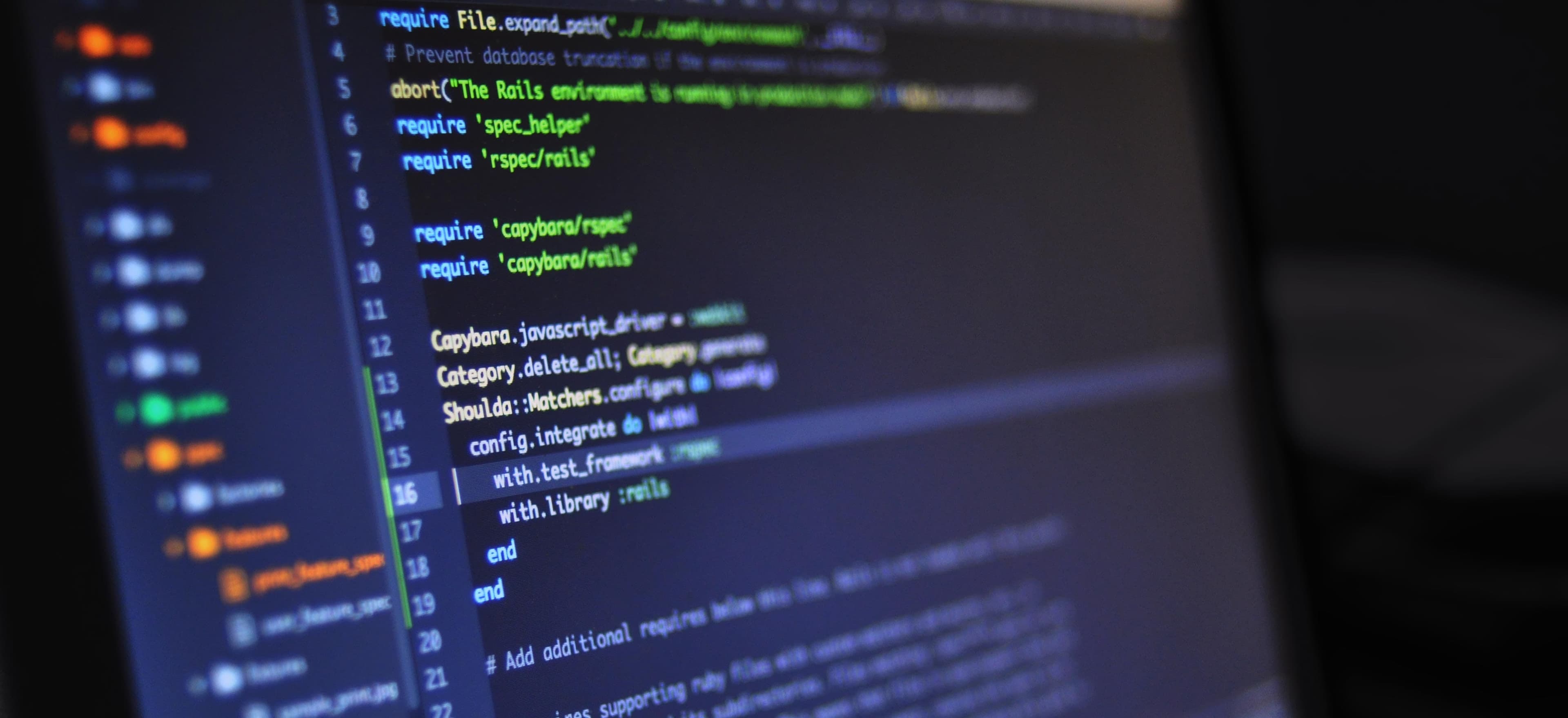
- Published on
Common Pitfalls in Spring Boot Integration Testing with Selenium
Integration testing is a critical aspect of the modern software development cycle. It helps ensure that various components of your application work together as expected. Spring Boot provides excellent tools for integration testing, and when combined with Selenium, you can test the entire web application stack, from the front end to the back end. However, there are common pitfalls that many developers encounter along the way. This blog post will explore these pitfalls while offering solutions to help you write effective integration tests.
Table of Contents
- Understanding Integration Testing in Spring Boot
- The Power of Selenium
- Common Pitfalls
- 3.1 Not Setting Up the Test Environment Properly
- 3.2 Ignoring Test Data Management
- 3.3 Failure to Isolate Tests
- 3.4 Improper Use of Selenium’s Wait Mechanism
- 3.5 Not Cleaning Up After Tests
- Best Practices for Integration Testing in Spring Boot
- Conclusion
Understanding Integration Testing in Spring Boot
Integration testing in Spring Boot allows you to validate that your application components, such as services and controllers, work well together. Spring Boot simplifies this process by providing:
- Auto-configuration: Reduces boilerplate code.
- Annotations: Such as
@SpringBootTest
for easy test setup.
For further reading, you can check out the Spring Boot Testing Documentation.
Example of a Simple Integration Test
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
class MyApplicationTests {
@Autowired
private MyService myService;
@Test
void contextLoads() {
// This test will fail if the application context cannot be loaded
assertNotNull(myService);
}
}
Why It Matters: This test ensures that your Spring context loads correctly, verifying that your beans are set up properly.
The Power of Selenium
Selenium is a widely used framework for automating web applications for testing purposes. It allows you to simulate user interactions with your application, such as clicking buttons or filling out forms.
Setting Up Selenium
To get started with Selenium in a Spring Boot context, ensure you have the following dependencies in your pom.xml
.
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.141.59</version>
<scope>test</scope>
</dependency>
Common Pitfalls
3.1 Not Setting Up the Test Environment Properly
One common mistake is failing to configure the application’s test environment correctly. This can lead to tests that run in a different environment than expected, leading to unpredictable results.
Solution: Always ensure that you are using @SpringBootTest
to bootstrap your application context in a consistent manner. Use profiles effectively.
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
@ActiveProfiles("test") // Use a 'test' profile for configurations
3.2 Ignoring Test Data Management
When tests run, they often rely on a database filled with the required data. Forgetting to set up the correct test data can lead to failures that are difficult to diagnose.
Solution: Use @BeforeEach to populate your database with the necessary data:
import org.junit.jupiter.api.BeforeEach;
import org.springframework.beans.factory.annotation.Autowired;
class MyIntegrationTest {
@Autowired
private MyRepository myRepository;
@BeforeEach
void setUp() {
// Clear out the repository
myRepository.deleteAll();
// Insert test data
myRepository.save(new MyEntity("Test Data"));
}
}
Why It Matters: Providing a clean state for each test ensures they run independently and reliably.
3.3 Failure to Isolate Tests
Running integration tests that depend on each other can lead to cascading failures. This is a recipe for debugging nightmares.
Solution: Ensure tests are isolated. Each test should set up its environment independently.
@Test
void testFeatureOne() {
// Test logic specific to Feature One
}
@Test
void testFeatureTwo() {
// Test logic specific to Feature Two
}
3.4 Improper Use of Selenium’s Wait Mechanism
Selenium provides several ways to wait for elements, like implicit waits and explicit waits. Relying solely on implicit waits can lead to flaky tests.
Solution: Use explicit waits more effectively. Explicit waits wait for a specific condition to occur.
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
Why It Matters: This makes your tests more reliable, ensuring elements are present and ready for interaction.
3.5 Not Cleaning Up After Tests
Another common pitfall is failing to clean up test data after running tests. This can lead to unexpected consequences and inconsistencies.
Solution: Use @AfterEach or @Transactional annotations to rollback changes after each test.
@AfterEach
void tearDown() {
myRepository.deleteAll(); // Clean up test data
}
Best Practices for Integration Testing in Spring Boot
To avoid the aforementioned pitfalls, consider these best practices:
- Use Profiles Wisely: Set up different configuration profiles for testing environments.
- Utilize Test Containers: Libraries like Testcontainers can help simulate databases and services.
- Review Each Test: Ensure that each test is self-contained and does not depend on existing state.
- Log Wisely: Use logging to capture the execution flow during tests.
- Collaborate: Share knowledge about testing pitfalls within your team to avoid repeated mistakes.
The Closing Argument
Integration testing is an integral part of software development, and when combined with Selenium, it provides a comprehensive testing strategy to verify application functionality. However, by being aware of common pitfalls—such as misconfigured environments, data management issues, and improper use of waiting mechanisms—you can enhance the reliability of your tests significantly.
By applying best practices and avoiding these pitfalls, you will build a robust integration testing strategy that can help maintain the quality of your Spring Boot applications. For more information, explore the official Selenium Documentation.
Happy testing!