Overcoming Maven Dependency Conflicts: A Common Issue
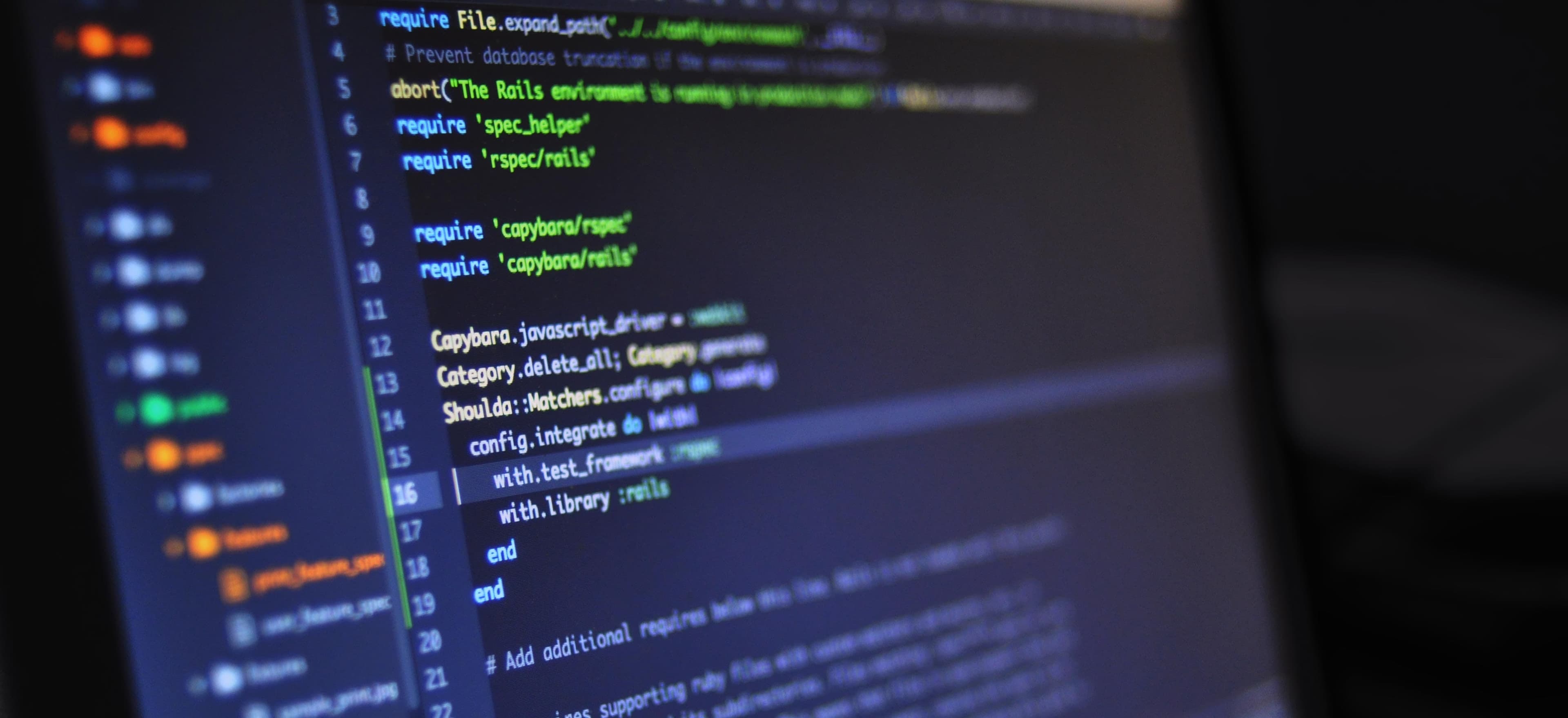
- Published on
Overcoming Maven Dependency Conflicts: A Common Issue
Working with Java projects often brings developers face-to-face with the complexities of dependency management. While Maven provides a robust system for handling libraries and their versions, it can lead to conflicts that disrupt project builds. This post aims to shed light on Maven dependency conflicts, how they arise, and effective strategies to overcome them.
Understanding Maven Dependency Management
Maven is a project management tool that simplifies Java project setups and builds. It relies on a pom.xml
file to manage project configurations, dependencies, and plugins. The real strength of Maven lies in its ability to manage libraries efficiently. However, this may sometimes backfire when two dependencies require conflicting versions of a given library.
What are Dependency Conflicts?
A dependency conflict occurs when two or more dependencies within a project refer to different versions of the same library. This ambiguity can lead to issues such as ClassNotFoundExceptions or NoSuchMethodError.
For example, consider the following scenario:
- Dependent A relies on
Library X version 1.0
- Dependent B relies on
Library X version 2.0
When both dependencies are included in your project, Maven must decide which version of Library X
is the "winner." The resolution process, known as the nearest definition, can lead to unintended consequences that might break your application.
Identifying Dependency Conflicts
The first step in resolving dependency conflicts is to identify them. Maven provides a command that helps you visualize your project's entire dependency tree:
mvn dependency:tree
This command outputs a structured representation of all dependencies, helping you identify where conflicts lie. You may see output like this:
[INFO] +- com.example:dependent-a:jar:1.0:compile
[INFO] | \- org.example:library-x:jar:1.0:compile
[INFO] +- com.example:dependent-b:jar:2.0:compile
[INFO] | \- org.example:library-x:jar:2.0:compile
The above output clearly shows two versions of library-x
, indicating a conflict.
Strategies to Resolve Dependency Conflicts
Once you've identified a conflict, consider the following strategies:
1. Exclude Conflicting Dependencies
If a certain library version is unnecessary for your project, you can exclude it. For instance, if you want to use version 2.0
of Library X
, you can exclude version 1.0
in the pom.xml
like so:
<dependency>
<groupId>com.example</groupId>
<artifactId>dependent-a</artifactId>
<version>1.0</version>
<exclusions>
<exclusion>
<groupId>org.example</groupId>
<artifactId>library-x</artifactId>
</exclusion>
</exclusions>
</dependency>
This code snippet helps you avoid pulling in an unwanted version of the library, reducing the chance of conflict.
2. Use Dependency Management
Maven offers a <dependencyManagement>
section to centralize control over library versions. By declaring the versions you want to use here, you ensure your dependencies reflect consistent versions.
Here is an example:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.example</groupId>
<artifactId>library-x</artifactId>
<version>2.0</version>
</dependency>
</dependencies>
</dependencyManagement>
Adding this to your pom.xml
enforces that all dependencies using library-x
will use version 2.0.
3. Version Resolution
Maven will select the "nearest definition" based on dependency hierarchy. If dependent-a
is higher in the hierarchy than dependent-b
, Maven will use the version declared in dependent-a
by default. By manipulating the order of dependencies in your pom.xml
, you can influence which version is selected.
4. Utilize the dependency:analyze
Goal
Running the following command can give you insight into how dependencies in your project are used:
mvn dependency:analyze
This command will help you track which dependencies are declared but not used and which are used but not declared. Fine-tuning your dependencies can prevent conflicts from arising altogether.
A Real World Example
Let's walkthrough a practical example. Suppose you are working on a microservices project that has two services, Service A and Service B.
Service A depends on spring-boot-starter-web
version 2.4.0
, while Service B relies on spring-boot-starter-web
version 2.5.0
. If both services are packaged into a single application, this could result in a dependency conflict.
Step 1: Identify Conflicts
Run the dependency tree as shown earlier:
mvn dependency:tree
Step 2: Exclude the Older Version
To resolve this issue, you can exclude the older version:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.0</version>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</exclusion>
</exclusions>
</dependency>
Step 3: Use Dependency Management
Or you can enforce the version via dependency management:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.0</version>
</dependency>
</dependencies>
</dependencyManagement>
By following these steps, you can resolve the dependency conflict efficiently, ensuring that you're using a consistent and compatible version of spring-boot-starter-web
.
Final Thoughts
Maven dependency conflicts can be a substantial challenge for Java developers, especially as projects scale. By understanding how these conflicts arise and employing strategies to resolve them—such as excluding conflicting dependencies, leveraging dependency management, and using effective resolution practices—you can improve project stability and maintainability.
For further reading on Maven dependency management and resolving conflicts, refer to the Maven Documentation and Spring Documentation.
By mastering the art of dependency management with Maven, you equip yourself with essential skills that contribute to cleaner, more robust Java projects.