Mastering OSGi: Overcoming Microservices Integration Challenges
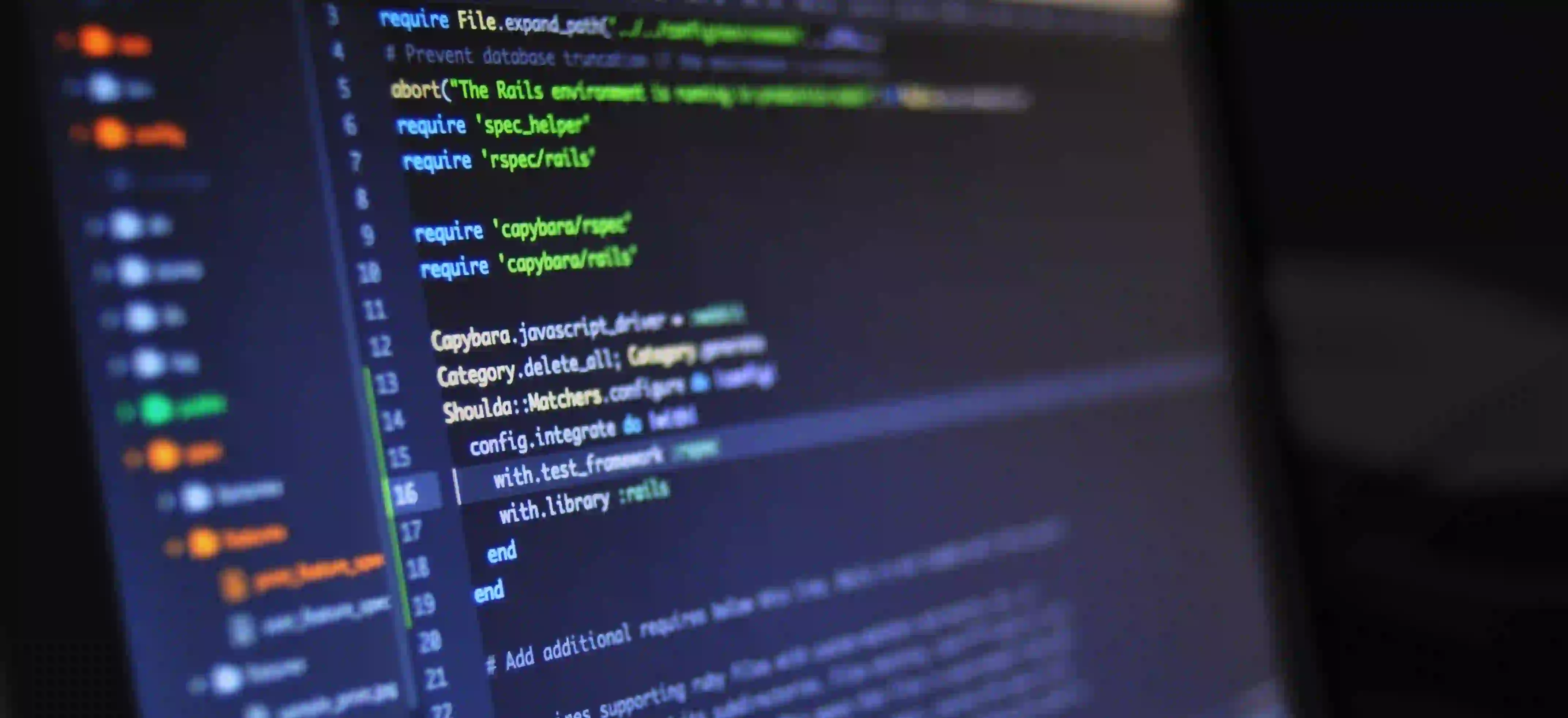
Mastering OSGi: Overcoming Microservices Integration Challenges
In today's fast-paced software landscape, microservices architecture has emerged as a cornerstone for building scalable and resilient applications. However, with its benefits come significant challenges, especially in terms of integrating various components. Enter OSGi (Open Service Gateway Initiative), a powerful framework designed to address these integration pitfalls. This blog post explores how OSGi can help overcome microservices integration challenges and delves into its best practices, using practical Java code snippets to illustrate the concepts.
Understanding Microservices Integration Challenges
Microservices architecture is defined by small, independent services that communicate with each other to form a complete application. Although this approach allows for flexibility and ease of deployment, it also introduces complexity in:
- Service Communication: With many services communicating over a network, ensuring reliable and efficient communication becomes difficult.
- Dependency Management: Services often have dependencies on shared resources, leading to version conflicts and increased maintenance overhead.
- Dynamic Discovery: Services must be able to discover each other dynamically, adding another layer of complexity.
OSGi provides an effective solution to these challenges by enabling dynamic modularity, making it easier to manage dependencies, and allowing for effective service management.
What is OSGi?
OSGi is a specification that defines a dynamic component model for Java. Components, or bundles, can be deployed, updated, and removed independently, facilitating a modular design. OSGiβs dynamic nature makes it particularly suitable for microservices integration, allowing more flexibility in dealing with components that must be updated frequently.
Key Features of OSGi
- Modularity: OSGi bundles are modular, encapsulating their classes and resources.
- Dynamic Loading: Bundles can be installed, started, stopped, and updated at runtime without requiring a restart.
- Service Registry: OSGi provides a service registry that allows bundles to search for and bind to services dynamically.
Getting Started with OSGi
To illustrate how to implement OSGi in Java, letβs walk through a simple example of creating an OSGi bundle.
Step 1: Setting Up the Environment
To start using OSGi, you'll need to set up an OSGi runtime. Apache Felix is a widely used implementation. You can download it from Apache Felix.
Step 2: Creating Your First OSGi Bundle
- Project Structure: Create a new Maven project with the following structure:
my-osgi-bundle
βββ pom.xml
βββ src
β βββ main
β βββ java
β βββ com
β βββ example
β βββ HelloWorldService.java
- POM Configuration: Your
pom.xml
should have the following dependencies for OSGi:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-osgi-bundle</artifactId>
<version>1.0.0</version>
<packaging>bundle</packaging>
<dependencies>
<!-- OSGi API Dependency -->
<dependency>
<groupId>org.osgi</groupId>
<artifactId>org.osgi.core</artifactId>
<version>6.0.0</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.felix</groupId>
<artifactId>maven-bundle-plugin</artifactId>
<version>5.1.2</version>
<extensions>true</extensions>
</plugin>
</plugins>
</build>
</project>
Step 3: Implementing a Service
Now, let's create a simple service interface and its implementation. Here's the service interface (HelloWorldService.java
):
package com.example;
public interface HelloWorldService {
String sayHello();
}
And here is the implementation:
package com.example;
import org.osgi.service.component.annotations.Component;
@Component(service = HelloWorldService.class)
public class HelloWorldServiceImpl implements HelloWorldService {
@Override
public String sayHello() {
return "Hello, OSGi!";
}
}
Why OSGi? By using the @Component
annotation, we ensure that our service can be registered and discovered at runtime. This encapsulation promotes reusable and modular designs.
Step 4: Registering the Service
In OSGi, it's imperative to manage lifecycle events of the registered services. The service can be registered like so:
import org.osgi.service.component.annotations.Component;
import org.osgi.service.component.annotations.Reference;
@Component
public class HelloWorldClient {
private HelloWorldService helloWorldService;
@Reference
public void setHelloWorldService(HelloWorldService helloWorldService) {
this.helloWorldService = helloWorldService;
System.out.println(helloWorldService.sayHello());
}
}
Dynamic Changes with OSGi: When you deploy this bundle to an OSGi container like Apache Felix, the HelloWorldClient
automatically discovers the HelloWorldService
and invokes its sayHello
method.
Step 5: Running the OSGi Application
To run your project, ensure Apache Felix is up and running. You can do this by placing your bundle JAR file into the bundle
directory of Apache Felix.
java -jar bin/felix.jar
Once you're in the Felix console, use the command:
install bundle:<path-to-your-jar-file>
start <bundle-id>
Benefits of Using OSGi for Microservices
-
Dynamic Updates: OSGi allows for seamless updates to parts of your application without downtime. This is crucial when you need to deploy fixes quickly.
-
Seamless Dependency Management: With OSGiβs service registry, services can have their dependencies dynamically resolved at runtime, decreasing version conflict scenarios.
-
Modularity & Reusability: OSGi promotes a modular approach, allowing teams to build and maintain services independently. This supports scaling efforts by enabling diverse teams to work concurrently on different services.
Best Practices for OSGi in Microservices
-
Define Clear Service Interfaces: Always define clear and concise service interfaces. This encourages a clean contract between the producer and consumer.
-
Version Your Services: Maintain different versions of your services in OSGi to prevent breaking changes from impacting existing consumers.
-
Regularly Audit Bundles: Keep track of the bundles, dependencies, and services you have in your OSGi container. This promotes easy maintenance and troubleshooting.
-
Logging and Monitoring: Implement robust logging and monitoring within your OSGi bundles. Knowing how services are interacting can help debug complex issues that arise during microservices communication.
Final Thoughts
OSGi presents a viable solution to the common microservices integration challenges faced today. By leveraging its modular architecture, dynamic loading capabilities, and service registry, developers can build flexible and resilient applications with ease.
The dynamic nature of OSGi empowers teams to quickly respond to changes, reduce deployment hassles, and improve maintainability and scalability.
For further reading, you can dive into the official OSGi specifications to deepen your understanding. Additionally, exploring Apache Felix documentation will provide insights into real-world applications of OSGi.
Happy coding!