Common Hibernate Pitfalls: List Retrieval Issues Explained
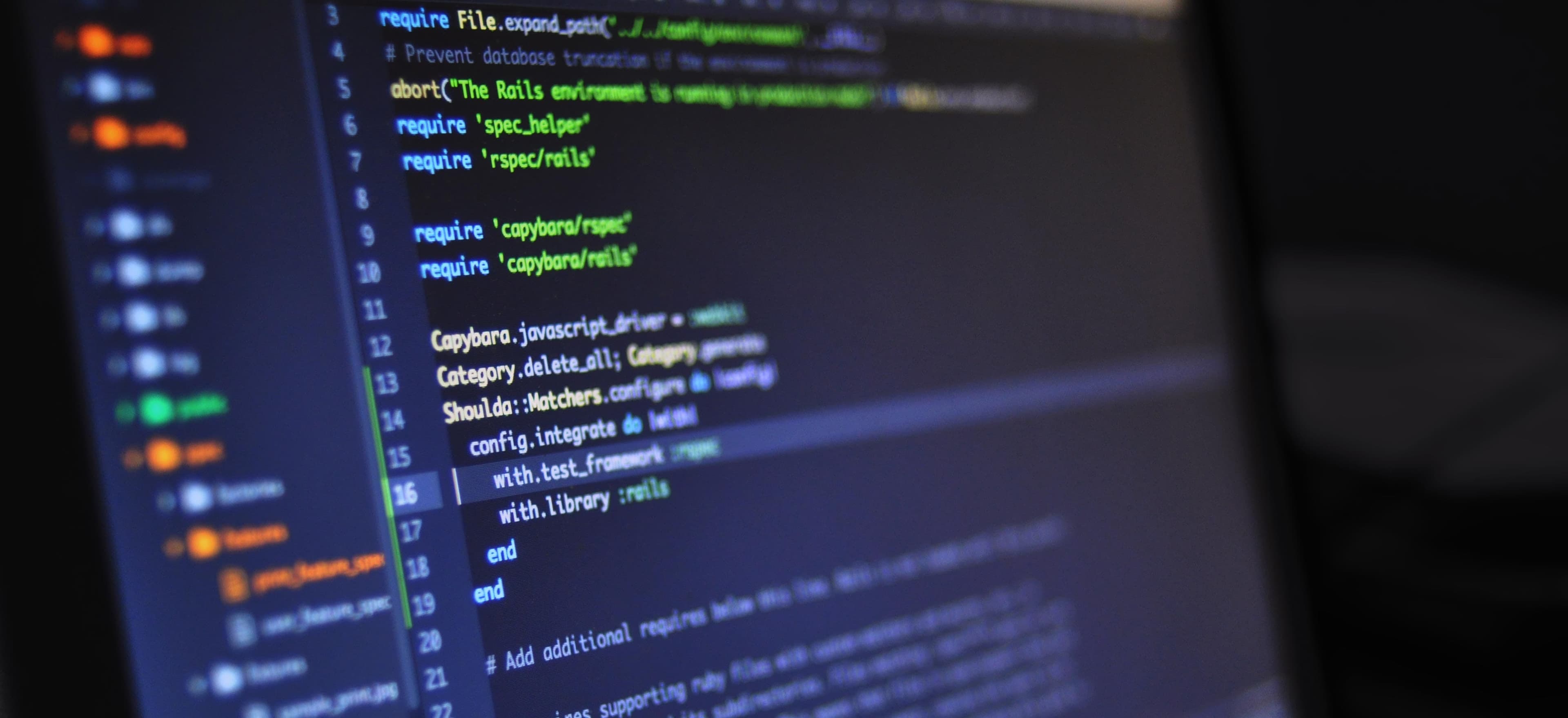
- Published on
Common Hibernate Pitfalls: List Retrieval Issues Explained
Hibernate is a powerful Object-Relational Mapping (ORM) tool for Java that simplifies database interactions. However, as developers harness its capabilities, they often encounter pitfalls, especially related to list retrieval. This blog post aims to address these issues, helping you to smoothly integrate Hibernate into your Java applications.
Understanding Hibernate's Session and Transactions
Before we dive deeper into the pitfalls, let’s have a quick refresh on Hibernate's architecture. Hibernate uses a Session which acts as a single-threaded context for interacting with the database. Each session corresponds to a single database connection and is bound to a transaction. Understanding these concepts is vital, as many list retrieval issues stem from improper management of sessions and transactions.
Sample Code: Opening a Hibernate Session
SessionFactory sessionFactory = new Configuration().configure().buildSessionFactory();
Session session = sessionFactory.openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
// Your code goes here
transaction.commit();
} catch (HibernateException e) {
if (transaction != null) transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
In this snippet, we open a session and start a transaction. If an exception occurs, we ensure to roll back any changes, preserving data integrity. Knowing how to handle sessions correctly can mitigate many retrieval issues.
Common Pitfalls in List Retrieval
Below are some prevalent pitfalls developers face when retrieving lists in Hibernate, along with coding solutions and explanations.
1. Lazy Initialization Exception
Problem: When you try to access a collection or a proxy entity that was not initialized when the session was closed, Hibernate throws a LazyInitializationException
.
Solution: Always be aware of when your session is open and closed. If you need to access a lazy collection outside the session context, consider initializing it beforehand.
Example Code: Initializing a Lazy Collection
Transaction transaction = null;
try (Session session = sessionFactory.openSession()) {
transaction = session.beginTransaction();
// Eager fetching by using join fetch
List<Product> products = session.createQuery("FROM Product p JOIN FETCH p.reviews", Product.class).getResultList();
// Accessing reviews outside the session won't throw LazyInitializationException
for (Product product : products) {
System.out.println(product.getReviews().size());
}
transaction.commit();
} catch (HibernateException e) {
if (transaction != null) transaction.rollback();
e.printStackTrace();
}
In this example, using JOIN FETCH
allows us to retrieve Product
and its reviews
in one transaction, ensuring they remain initialized.
2. N+1 Select Problem
Problem: The N+1 problem occurs when you query a list of entities and, for each entity, a subsequent query is executed to retrieve its related entities. This results in excessive database calls, degrading performance.
Solution: Use fetch joins or DTO projections to reduce the number of database calls.
Example Code: Using Fetch Join
List<Category> categories = session.createQuery(
"SELECT c FROM Category c JOIN FETCH c.products", Category.class).getResultList();
for (Category category : categories) {
System.out.println(category.getProducts().size());
}
Here, fetching categories along with their products in a single query prevents the N+1 select issue and optimizes performance.
3. Incorrect HQL/JPQL Queries
Problem: A common pitfall is crafting incorrect HQL/JPQL queries that may not return the expected results or may even throw exceptions.
Solution: Always validate your HQL/JPQL syntax and ensure you're querying against the correct entity.
Example Code: Validating a Query
List<User> users = session.createQuery("FROM User u WHERE u.active = :isActive", User.class)
.setParameter("isActive", true)
.getResultList();
if(users.isEmpty()) {
System.out.println("No active users found.");
}
Here, using parameters helps avoid SQL injection and improves query readability.
4. Forgetting to Clear the Session
Problem: If you do not clear the Hibernate session, it may retain entities from previous transactions or queries, leading to memory issues or stale data.
Solution: Use session.clear()
to detach all objects from the session context.
Example Code: Clearing the Session
session.clear(); // Clear session to avoid stale data
List<Payment> payments = session.createQuery("FROM Payment", Payment.class).getResultList();
Clearing the session can alleviate memory bloat, especially in long-running processes.
5. Not Using Caching Properly
Problem: Failing to configure second-level caching can lead to performance bottlenecks, as Hibernate will hit the database every time it needs to fetch an entity.
Solution: Configure second-level caching and properly set cacheable queries.
Example Code: Enabling Second-Level Cache
<property name="hibernate.enable_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">
org.hibernate.cache.ehcache.EhCacheRegionFactory
</property>
By enabling the second-level cache in hibernate.cfg.xml
and annotating your entities with @Cache
, you can significantly boost performance.
Wrapping Up
Hibernate is an incredible tool, but it comes with its own set of challenges. By identifying and understanding these common pitfalls, you can improve your application's performance and data integrity.
For further reading, you can explore the Hibernate User Guide to deepen your understanding and best practices in Hibernate ORM.
Remember, mastering Hibernate not only enhances your Java programming skills but also aids in building robust and efficient applications. Keep learning, coding, and don’t let these pitfalls discourage you!
References
This guide should better equip you to handle list retrieval issues in Hibernate, fostering a successful development journey in your Java projects. Happy coding!