Unlocking Encapsulation: Common Misunderstandings Explained
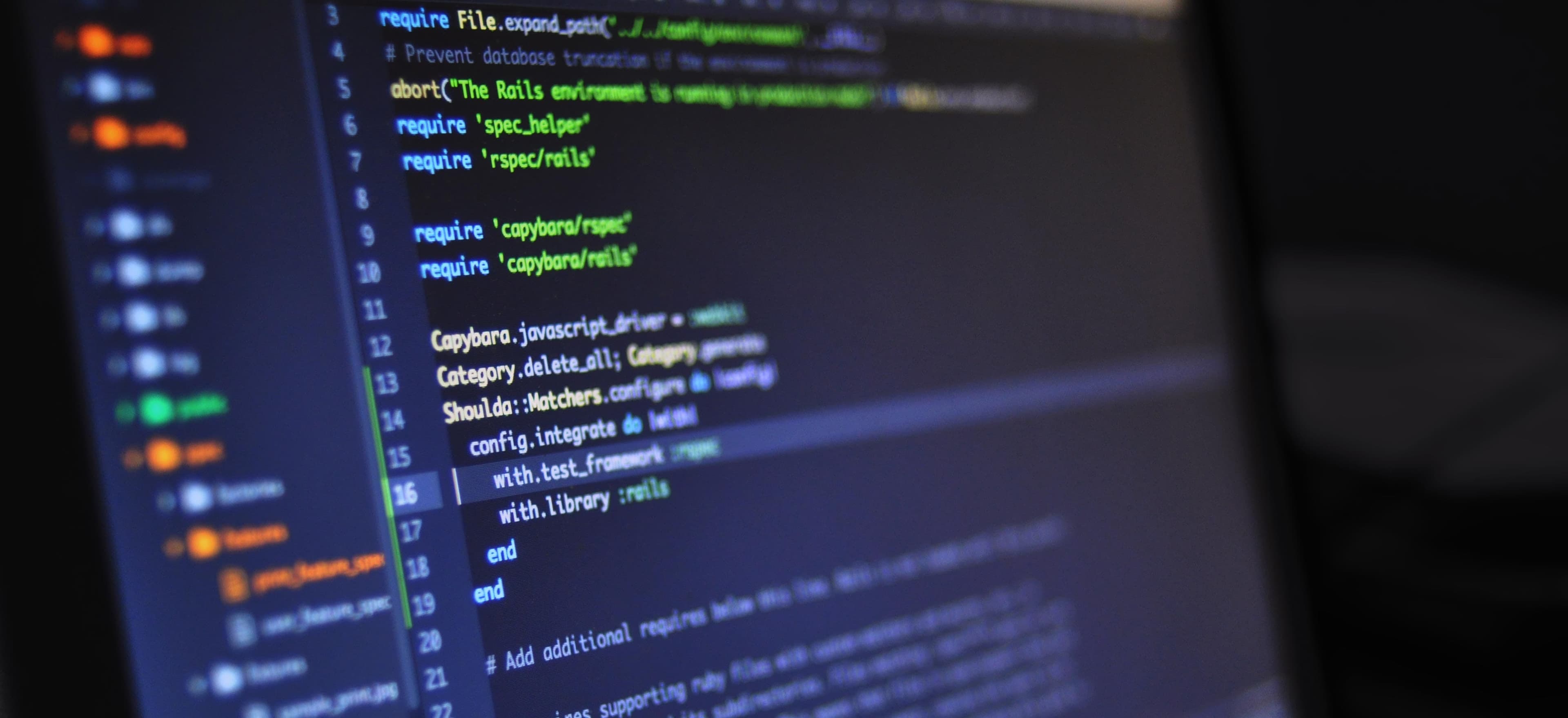
- Published on
Unlocking Encapsulation: Common Misunderstandings Explained
Encapsulation is one of the four fundamental Object-Oriented Programming (OOP) principles, alongside inheritance, polymorphism, and abstraction. It's a concept that ensures the internal representation of an object is hidden from the outside, allowing for a clean and manageable interface.
While many developers have heard of encapsulation, there are common misunderstandings that can lead to misimplemented code. This post aims to break down those misunderstandings and provide clear examples in Java to illustrate the correct applications of encapsulation.
What is Encapsulation?
Encapsulation is the bundling of data (attributes) and methods (functions) that operate on that data within a single unit or class. It restricts direct access to some of the object's components, which can help prevent unintended interference and misuse.
The primary goal of encapsulation is to protect the object's integrity by controlling how data is accessed or mutated.
Why Use Encapsulation?
- Control: You have control over what is exposed to the outside world.
- Flexibility: You can change the internal implementation without affecting external classes.
- Maintenance: Easier to maintain due to a defined and limited interface.
Core Concepts
Let’s explore encapsulation in Java with some code snippets to clarify its implementation.
1. Access Modifiers and Their Uses
Java provides several access modifiers to control access levels. Here are the main ones:
private
: The member is accessible only within its own class.protected
: The member can be accessed within its own package and by subclasses.public
: The member is accessible from anywhere.
Here is a basic example of these modifiers in a Java class:
public class Employee {
private String name; // Only accessible within Employee class
protected int id; // Accessible within the same package and subclasses
public String department; // Accessible everywhere
public Employee(String name, int id, String department) {
this.name = name;
this.id = id;
this.department = department;
}
// Getter for name
public String getName() {
return name;
}
// Setter for name
public void setName(String name) {
this.name = name;
}
}
Commentary on Access Modifiers
In the Employee
class:
- The
name
field is private to ensure that it can only be modified through its setter method, preserving control over what values can be set (e.g., you could add validation in the setter). - The
id
field is protected so that subclasses can directly access it, which is beneficial in inheritance scenarios. - The
department
field is public and directly accessible, which could be a design choice if the department doesn't require strict encapsulation.
2. Getters and Setters
Getters and setters are methods that provide controlled access to the attributes of the class. They ensure that the internal representation remains encapsulated while still allowing external interaction.
Here’s how the Employee
class leverages getters and setters:
public String getName() {
return name; // Provides controlled access to the private variable
}
public void setName(String name) {
if (name != null && !name.isEmpty()) { // Validates name before setting it
this.name = name;
}
}
Why Getters and Setters?
- They provide a way to enforce encapsulation by controlling access.
- They allow for validation and error-checking before setting or getting values, aiding in maintaining the integrity of the data.
3. Common Misunderstandings
Myth: Private Members are Completely Hidden
Many believe that declaring a member as private completely hides it. While this is true from an outside class perspective, subclasses can access protected or package-private members.
Reality Check
Consider the following:
public class Manager extends Employee {
public Manager(String name, int id, String department) {
super(name, id, department);
}
public void display() {
System.out.println("Manager ID: " + id); // Accessible since 'id' is protected
// System.out.println("Employee Name: " + name); // Error! name is private.
}
}
This demonstrates that encapsulation applies differently across inheritance hierarchies.
4. Ignoring Encapsulation Effects on Maintenance
Some developers often prioritize immediate coding needs over solid encapsulation. When a class exposes too much information via public members, it creates dependencies that can lead to maintenance nightmares.
Example of What Not to Do
public class Project {
public ArrayList<Employee> employees; // Poor encapsulation
public Project() {
employees = new ArrayList<>();
}
}
This exposes the internal list structure, leading to potential misuse. An external class could directly modify the list, breaking encapsulation.
Preferred Approach
Instead, an encapsulated version would look like this:
public class Project {
private ArrayList<Employee> employees; // Protected by encapsulation
public Project() {
employees = new ArrayList<>();
}
public void addEmployee(Employee employee) {
employees.add(employee); // Controlled access
}
public List<Employee> getEmployees() {
return new ArrayList<>(employees); // Returns a copy for safe access
}
}
5. Conclusion: Why Encapsulation Matters
Encapsulation is more than just a coding practice; it's a vital principle that enhances code quality and maintainability. It allows developers to protect and manage code effectively.
By effectively utilizing access modifiers and providing thoughtful getters and setters, you encourage software that is robust and less prone to unintentional bugs.
As you move forward in your Java journey, keep in mind the true power of encapsulation. It not only protects your data but also ensures your code is adaptable and easier to maintain.
For more advanced insights on Object-Oriented Programming principles, check out this article from Oracle for deeper understanding.
Further Reading
To deepen your understanding of design principles and practices in Java, consider reading "Effective Java" by Joshua Bloch.
By paying attention to encapsulation and understanding its principles, you’ll be on your way to becoming a more proficient Java developer, apt at creating clean, manageable, and reliable code.