Mastering HashMap Sorting in Java: Common Pitfalls
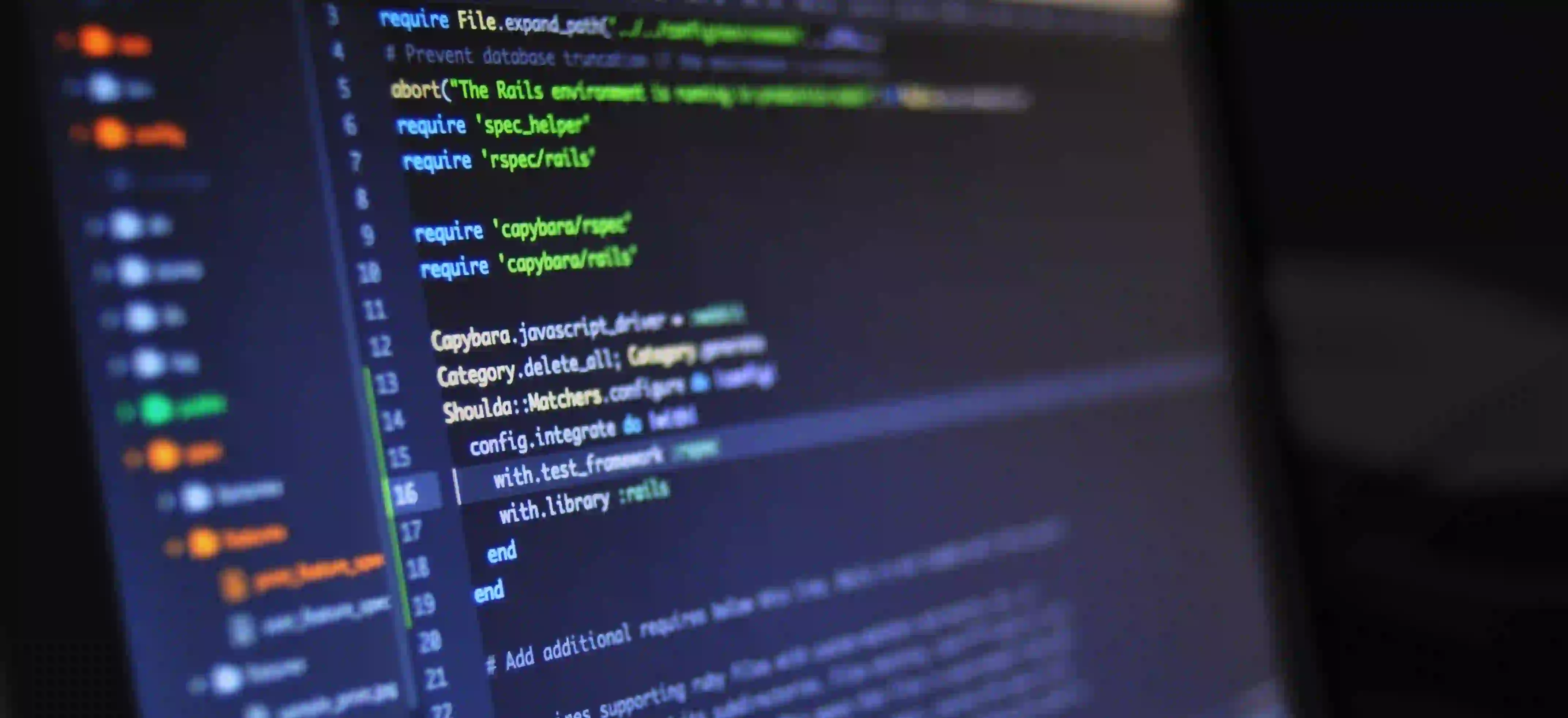
Mastering HashMap Sorting in Java: Common Pitfalls
Java offers a robust HashMap implementation for storing key-value pairs. However, sorting a HashMap is not as straightforward as one might expect. When developers attempt to sort HashMaps, they often run into common pitfalls. This guide will walk you through the intricacies of HashMap sorting, highlight frequent mistakes, and provide solutions with illustrative code snippets.
Understanding HashMap in Java
A HashMap stores data in key-value pairs, allowing for the efficient retrieval of values based on their corresponding keys. Despite its efficiency in data retrieval, a HashMap does not maintain any order. This is one of the primary reasons developers often find themselves needing to sort the data stored within a HashMap.
The core methods associated with HashMaps are:
put(key, value)
: Adds a key-value pair.get(key)
: Retrieves the value associated with a key.remove(key)
: Removes the key-value pair.size()
: Returns the number of key-value pairs.
Why Sorting a HashMap is Challenging
- Unordered Nature: HashMaps do not maintain the order of their elements. When you iterate over a HashMap, the order is entirely dependent on the hash of the keys.
- Sorting Mechanism: A sorting operation typically requires a data structure capable of maintaining order, which is not a feature of HashMap.
How to Sort a HashMap
To sort a HashMap, follow these steps:
- Convert the HashMap into a list of entries.
- Sort that list based on your criteria (e.g., by key or by value).
- Use a LinkedHashMap to maintain the sorted order.
Here's a simple example:
import java.util.*;
public class HashMapSortingExample {
public static void main(String[] args) {
// Create a HashMap
HashMap<String, Integer> scoreMap = new HashMap<>();
scoreMap.put("Alice", 30);
scoreMap.put("Bob", 20);
scoreMap.put("Charlie", 25);
// Sort the HashMap by keys
List<Map.Entry<String, Integer>> entryList = new ArrayList<>(scoreMap.entrySet());
Collections.sort(entryList, new Comparator<Map.Entry<String, Integer>>() {
public int compare(Map.Entry<String, Integer> o1, Map.Entry<String, Integer> o2) {
return o1.getKey().compareTo(o2.getKey());
}
});
// LinkedHashMap to maintain order
Map<String, Integer> sortedMap = new LinkedHashMap<>();
for (Map.Entry<String, Integer> entry : entryList) {
sortedMap.put(entry.getKey(), entry.getValue());
}
System.out.println("Sorted HashMap by key: " + sortedMap);
}
}
Commenting on the Code Example
- Sorting Mechanism: The
Collections.sort()
method sorts the list of entries. A custom comparator is used to sort based on keys. - LinkedHashMap Usage: We utilize a LinkedHashMap to preserve the order after sorting. This is key, as a regular HashMap would disrupt the order again.
Pitfall #1: Forgetting to Use LinkedHashMap
After sorting, some developers default back to using a plain HashMap, which causes them to lose the sorted order. Remember, the crucial aspect of sorting is maintaining the order, which is why using a LinkedHashMap is essential.
Solution
Always encapsulate your sorted data in a LinkedHashMap.
Sorting a HashMap by Values
You may also want to sort a HashMap based on its values. Here's how to accomplish this:
import java.util.*;
public class SortByValueExample {
public static void main(String[] args) {
// Create a HashMap
HashMap<String, Integer> scoreMap = new HashMap<>();
scoreMap.put("Alice", 30);
scoreMap.put("Bob", 20);
scoreMap.put("Charlie", 25);
// Sort the HashMap by values
List<Map.Entry<String, Integer>> entryList = new ArrayList<>(scoreMap.entrySet());
Collections.sort(entryList, new Comparator<Map.Entry<String, Integer>>() {
public int compare(Map.Entry<String, Integer> o1, Map.Entry<String, Integer> o2) {
return o1.getValue().compareTo(o2.getValue());
}
});
// Maintain order
Map<String, Integer> sortedMapByValue = new LinkedHashMap<>();
for (Map.Entry<String, Integer> entry : entryList) {
sortedMapByValue.put(entry.getKey(), entry.getValue());
}
System.out.println("Sorted HashMap by value: " + sortedMapByValue);
}
}
Insights on Sorting by Values
- Custom Comparator: A new comparator is used to compare the values, thus facilitating sorting based on value rather than key.
- Outcome: The final output is a LinkedHashMap maintaining the sorted order of values.
Pitfall #2: Sorting Large HashMaps
Sorting large HashMaps using the methods above can lead to performance issues. If efficiency is your concern, especially with larger datasets, consider the implications of time complexity and memory usage.
Solution
- Use Streams: Java 8 introduced Streams, which provide a cleaner and often more readable way to accomplish sorting. Here's how you can use Streams:
import java.util.*;
import java.util.stream.Collectors;
public class StreamSortingExample {
public static void main(String[] args) {
// Create a HashMap
HashMap<String, Integer> scoreMap = new HashMap<>();
scoreMap.put("Alice", 30);
scoreMap.put("Bob", 20);
scoreMap.put("Charlie", 25);
// Sort HashMap by value using Streams
Map<String, Integer> sortedMapByValueStream = scoreMap.entrySet()
.stream()
.sorted(Map.Entry.comparingByValue())
.collect(Collectors.toMap(
Map.Entry::getKey,
Map.Entry::getValue,
(e1, e2) -> e1,
LinkedHashMap::new
));
System.out.println("Sorted HashMap by value using Streams: " + sortedMapByValueStream);
}
}
Code Explanation
- Stream API: The
stream()
method allows for a functional style of processing data. - Comparison and Collection:
comparingByValue()
is used for sorting by values, and the results are collected back into a LinkedHashMap.
Common Pitfalls Recap
- Neglecting LinkedHashMap: It’s crucial to remember that a HashMap does not maintain order.
- Performance Issues: Be aware of the time complexity, especially with larger datasets. Stream API can sometimes avoid traditional sorting pitfalls.
A Final Look
Sorting a HashMap in Java presents unique challenges and common pitfalls that developers often face. By understanding the underlying principles, utilizing the power of the Stream API, and being vigilant about maintaining order with LinkedHashMap, you can efficiently and effectively sort your HashMap data.
For further reading, you can refer to the Java Documentation for more details on HashMaps and sorting methodologies.
By mastering HashMap sorting, you enhance your ability to manage collections and optimize your code for better performance. Happy coding!