Solving Common jOOQ and Spring Configuration Challenges
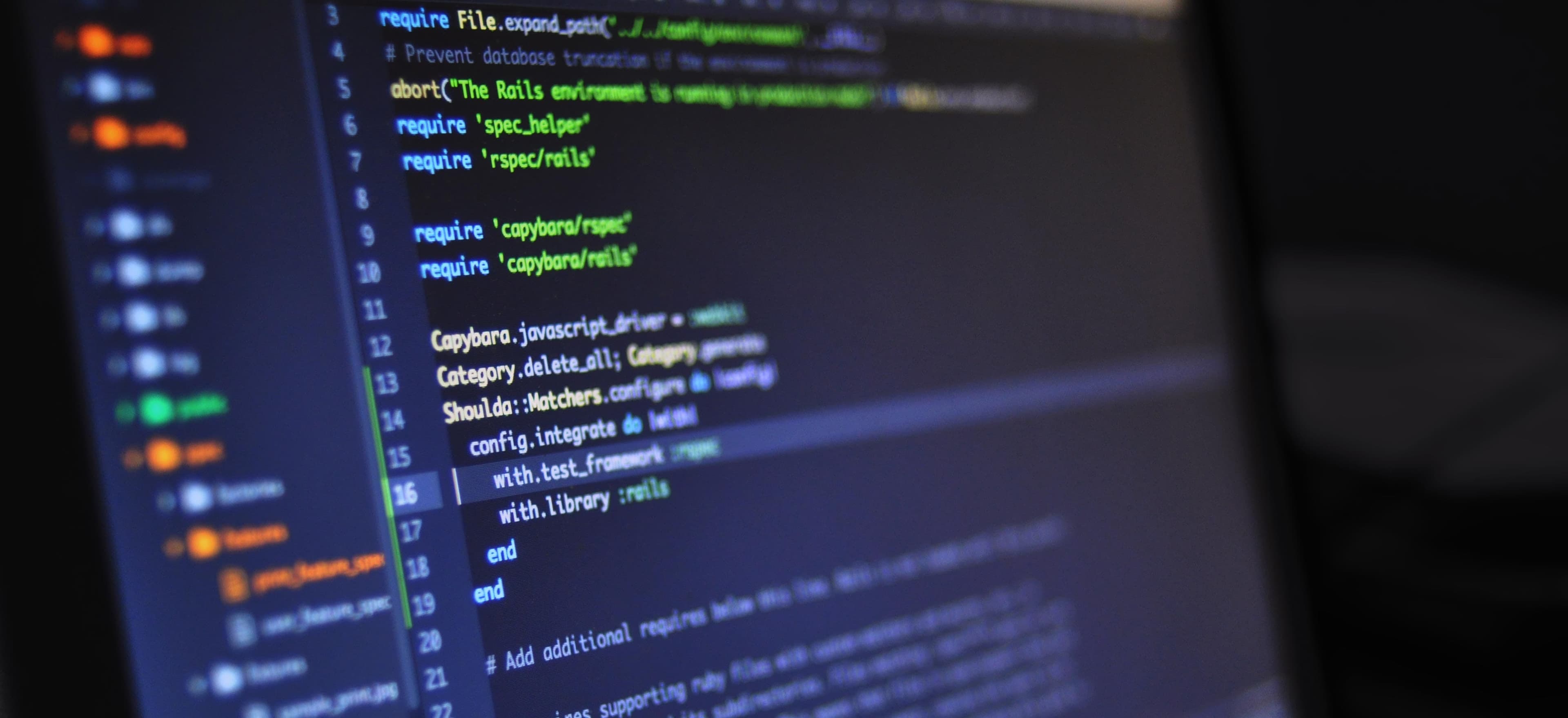
- Published on
Solving Common jOOQ and Spring Configuration Challenges
When it comes to modern Java applications, integrating jOOQ with Spring can be both a powerful and daunting task. While jOOQ offers type-safe SQL querying capabilities, Spring provides a robust ecosystem for dependency injection and transaction management. Understanding how to configure these two frameworks together effectively is crucial for ensuring smooth database interactions in your applications. In this blog post, we will tackle some common configuration challenges when using jOOQ with Spring, providing code snippets and best practices along the way.
Table of Contents
- Introduction to jOOQ and Spring
- Setting Up Your Project
- Configuring jOOQ with Spring
- Transaction Management Challenges
- Common Configuration Issues and Solutions
- Conclusion
The Starting Line to jOOQ and Spring
jOOQ (Java Object Oriented Querying) is a popular library that allows developers to write SQL queries in a type-safe manner while leveraging the benefits of Java's compile-time checking. By translating SQL statements into Java code, jOOQ reduces the chances of runtime errors.
Spring, on the other hand, is a comprehensive framework providing various services, including dependency injection, aspect-oriented programming, and transaction management. Together, jOOQ and Spring can create a powerful combination for building applications with robust data access layers.
Setting Up Your Project
To begin, you'll need to set up a Spring project with jOOQ. Here’s a brief overview of how to do that using Maven.
Pom.xml Configuration
First, include the necessary dependencies in your pom.xml
. Here's an example:
<dependencies>
<!-- Spring dependencies -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- jOOQ dependencies -->
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq</artifactId>
</dependency>
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq-codegen</artifactId>
</dependency>
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq-meta</artifactId>
</dependency>
<!-- Database Driver -->
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
Project Structure
Ensure that your project structure follows common conventions. Here's a simple layout:
src
├── main
│ ├── java
│ │ └── com
│ │ └── example
│ │ ├── config
│ │ ├── service
│ │ └── controller
│ └── resources
│ └── application.properties
Configuring jOOQ with Spring
Properly configuring jOOQ with Spring requires attention to detail when setting up the DSLContext
, which serves as the main API for interacting with jOOQ’s SQL tools.
Example Configuration Class
Here is how you can configure DSLContext
in a Spring configuration class:
@Configuration
public class JooqConfig {
@Bean
public DSLContext dsl(DataSource dataSource) {
return DSL.using(dataSource, SQLDialect.POSTGRES);
}
@Bean
public DataSource dataSource() {
HikariDataSource ds = new HikariDataSource();
ds.setJdbcUrl("jdbc:postgresql://localhost:5432/your_db");
ds.setUsername("your_user");
ds.setPassword("your_password");
return ds;
}
}
Why Use HikariCP?
HikariCP is known for its performance and simplicity when managing connections and is the recommended datasource for Spring applications. By using it, you improve the efficiency of your application, especially under heavy loads.
Transaction Management Challenges
One of the key aspects of Spring is its robust transaction management capabilities. However, integrating this with jOOQ can pose challenges, particularly when using different transaction propagation settings or managing nested transactions.
Example Transactional Service
Here’s how to set up a transactional service utilizing jOOQ:
@Service
public class UserService {
private final DSLContext dsl;
@Autowired
public UserService(DSLContext dsl) {
this.dsl = dsl;
}
@Transactional
public void createUser(String username) {
dsl.insertInto(USERS)
.set(USERS.USERNAME, username)
.execute();
}
}
Key Takeaway: Propagation Behavior
When dealing with transactions, it is essential to understand the propagation behavior that best fits your use case. The default is REQUIRED
, which will join an existing transaction if present. This can lead to subtle bugs if not handled properly.
Common Configuration Issues and Solutions
Issue 1: DataSource Autowiring
Sometimes, you might encounter issues with the DataSource
not being autowired correctly. To resolve this, ensure that Spring's JDBC setup and your datasource are adequately configured:
@Bean
public DataSource dataSource() {
return DataSourceBuilder.create()
.driverClassName("org.postgresql.Driver")
.url("jdbc:postgresql://localhost:5432/your_db")
.username("your_user")
.password("your_password")
.build();
}
Issue 2: jOOQ Code Generation
When generating code with jOOQ, a common issue is misconfigured database schema settings. Ensure that your jOOQ generator is provided the correct database URL and credentials.
// Example jOOQ Generator Configuration
Generator generator = new Generator()
.setGeneratorStrategy(new DefaultGeneratorStrategy())
.setJdbc(new Jdbc()
.withDriver("org.postgresql.Driver")
.withUrl("jdbc:postgresql://localhost:5432/your_db")
.withUser("your_user")
.withPassword("your_password"))
.setTarget(new Target()
.withPackageName("com.example.jooq.model")
.withDirectory("src/main/java"));
Final Thoughts
Integrating jOOQ with Spring can significantly streamline your data access layer, but it does come with its challenges. By understanding how to configure the two frameworks, grasp the implications of transaction propagation, and be aware of common configuration hurdles, you can unleash the full potential of both libraries.
For developers seeking to build robust Java applications, the combination of jOOQ and Spring provides type-safe querying while maintaining the benefits of Spring's powerful dependency management and transaction capabilities.
For additional reading on configuring jOOQ with Spring and best practices, check out the jOOQ official documentation and the Spring framework documentation for an even deeper understanding of how to integrate these technologies seamlessly in your applications.