Overcoming Complexity: Challenges in Mediator Pattern Usage
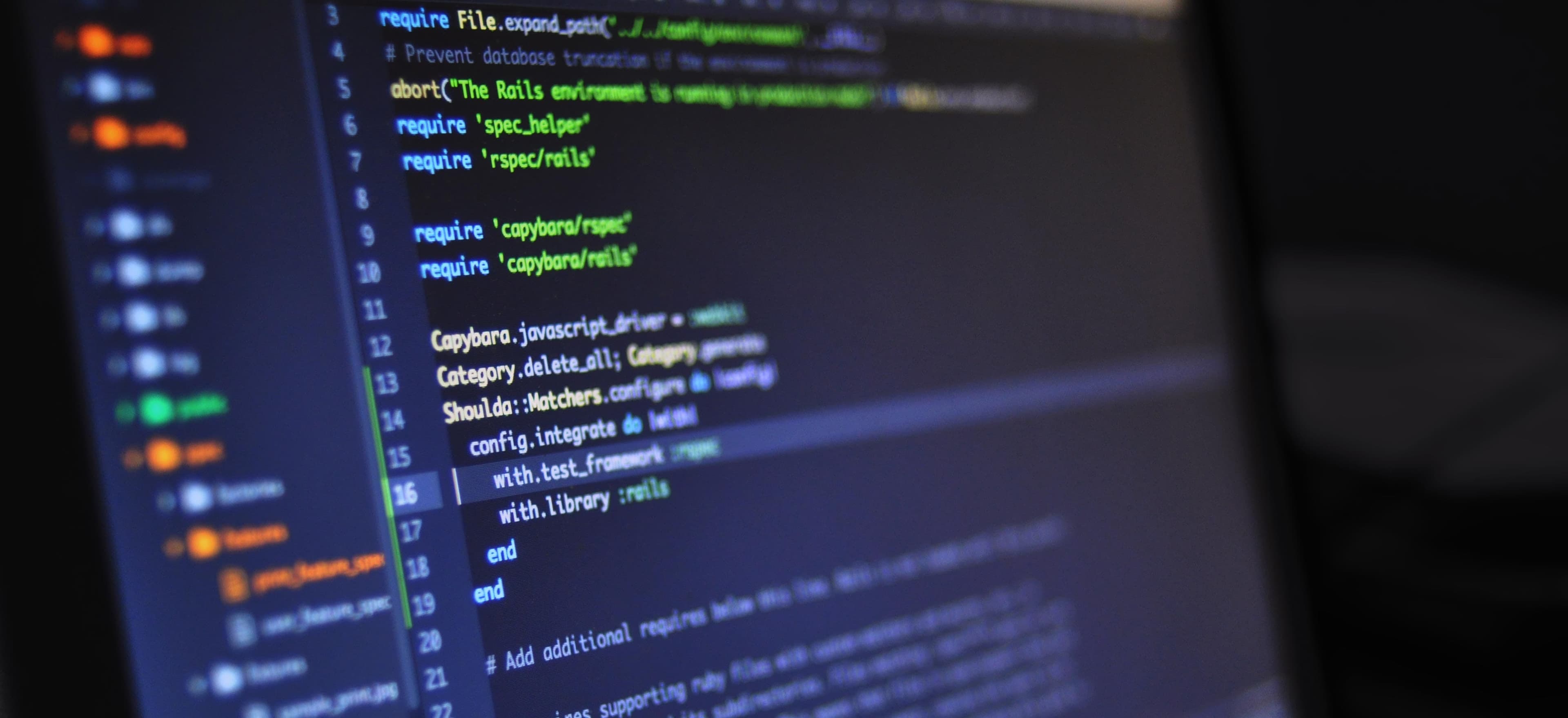
- Published on
Overcoming Complexity: Challenges in Mediator Pattern Usage
The Mediator Pattern is a behavioral design pattern that facilitates communication between multiple objects without requiring them to reference each other directly. This pattern promotes loose coupling between components, enhancing system modularity. However, when implemented in larger systems, developers might face complexities that can hinder the anticipated benefits. In this blog post, we'll explore these challenges and provide insight on how to effectively implement the Mediator Pattern in your Java applications.
Understanding the Mediator Pattern
Before diving into the challenges, let's grasp the fundamental workings of the Mediator Pattern. In essence, this pattern introduces a mediator class that encapsulates how different components interact. The components communicate with the mediator rather than directly with one another, which centralizes the interaction logic.
Key Components of the Mediator Pattern
- Mediator: The interface that defines the methods through which components communicate.
- Concrete Mediator: The implementation of the Mediator that orchestrates interactions between components.
- Colleagues: The classes that interact with the Mediator.
Example Code Snippet
// Mediator interface
public interface Mediator {
void registerColleague(Colleague colleague);
void relayMessage(Colleague sender, String message);
}
// Colleague interface
public abstract class Colleague {
protected Mediator mediator;
public Colleague(Mediator mediator) {
this.mediator = mediator;
mediator.registerColleague(this);
}
public abstract void send(String message);
public abstract void receive(String message);
}
In this code snippet:
- The
Mediator
interface declares methods to register colleagues and facilitate message relay. - The
Colleague
class acts as a base for other components that will interact through the mediator.
Implementation of a Concrete Mediator
import java.util.ArrayList;
import java.util.List;
public class ConcreteMediator implements Mediator {
private List<Colleague> colleagues = new ArrayList<>();
@Override
public void registerColleague(Colleague colleague) {
colleagues.add(colleague);
}
@Override
public void relayMessage(Colleague sender, String message) {
for (Colleague colleague : colleagues) {
// Ensuring the sender doesn't receive its own message
if (colleague != sender) {
colleague.receive(message);
}
}
}
}
Here:
- The
ConcreteMediator
manages a list ofColleague
objects. - It broadcasts messages from one colleague to others, exemplifying its central role in communication.
Common Challenges of Using the Mediator Pattern
Even though the Mediator Pattern brings benefits such as decoupling, it is not without its challenges. In fact, understanding these challenges is vital for effective implementation.
1. Complexity in Mediation Logic
As systems grow in size, the mediation logic can become increasingly complex. The ConcreteMediator
can start to embody many responsibilities, resembling a "God Object", which is best avoided.
Strategy to Combat Complexity
To manage this complexity, consider splitting responsibilities. Use smaller mediators specialized for different functionalities. This follows the Single Responsibility Principle (SRP) and keeps your code more manageable.
public class ChatMediator extends ConcreteMediator {
// Additional chat-specific methods can be added here.
}
public class NotificationMediator extends ConcreteMediator {
// Additional notification-specific methods can be added here.
}
2. Scalability Concerns
The more components you add, the more intricate the relationships can become. Each additional Colleague
not only increases the complexity of the ConcreteMediator
but also makes communication less efficient.
Solution
Limit the number of direct communications through the mediator. Introduce layers of abstraction by creating interfaces to minimize the coupling.
3. Debugging Challenges
Debugging a system using the Mediator Pattern can prove difficult due to indirect communication paths. When an issue arises, it can be challenging to trace the source of messages back to their origin.
Debugging Strategy
Implement comprehensive logging within the Mediator
methods. Log both the message sent and the sender details to facilitate tracking issues.
@Override
public void relayMessage(Colleague sender, String message) {
System.out.println("Message from " + sender.getClass().getSimpleName() + ": " + message);
for (Colleague colleague : colleagues) {
if (colleague != sender) {
colleague.receive(message);
}
}
}
4. Overhead in Message Passing
Relying on a mediator for all communications may add unnecessary overhead. This overhead can often lead to performance issues, especially in high-frequency messaging scenarios.
Refined Approach
Use direct communication between colleagues for critical performance paths when it is known that their interactions are stable and predictable. Only utilize the mediator for less frequent or more complex interactions.
5. Learning Curve for New Developers
New developers may struggle to understand the Mediator Pattern, particularly in large systems with extensive mediator logic. It can be overwhelming to grasp how components interrelate purely through the mediator.
Mitigating the Learning Curve
Provide thorough documentation and visual mapping of component interactions. Additionally, consider mentoring sessions to walk through existing implementations with new team members.
A Final Look
The Mediator Pattern is undeniably a useful design paradigm, promoting decoupling while streamlining communication. However, as we have seen, its implementation carries inherent complexities, especially in larger systems. Addressing these challenges requires careful planning, a balance between abstraction and performance, and adherence to best practices.
By taking a proactive approach to complexity, scalability, debugging, overhead, and learning curves, developers can reap the true benefits of the Mediator Pattern without falling prey to its pitfalls. As you embark on your journey to implement this pattern, remember that understanding these challenges is as critical as the technical acumen behind the actual implementation.
We encourage you to explore more about design patterns using reputable resources like Martin Fowler's Patterns of Enterprise Application Architecture or the Gang of Four's Design Patterns: Elements of Reusable Object-Oriented Software. As you navigate complex system designs, keep the principles of design patterns in mind, guiding you in creating robust, maintainable, and scalable applications.
For further reading on Java design patterns, check out the Java Design Patterns resource.
Happy coding!
Checkout our other articles