Common Pitfalls in JSR 303 Parameter Validation with Spring
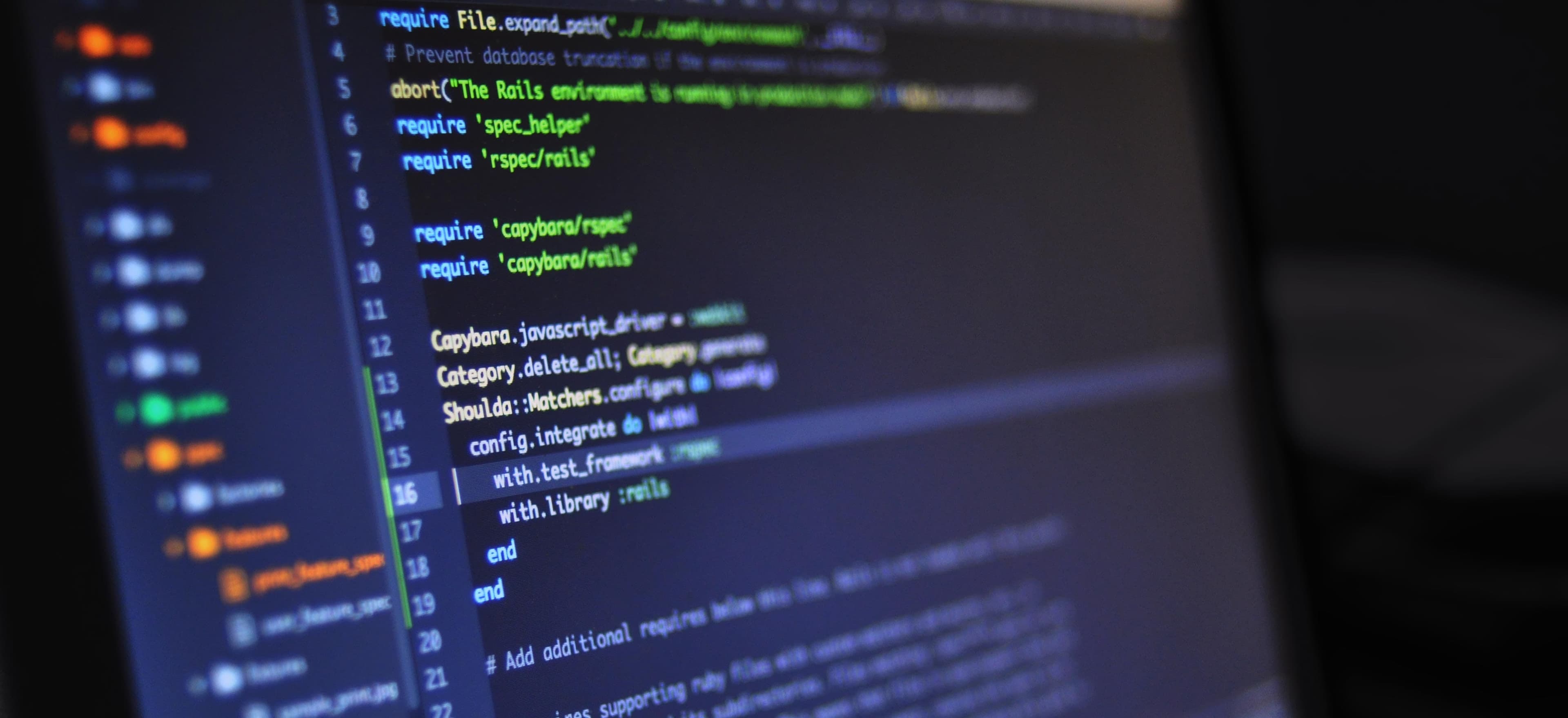
- Published on
Common Pitfalls in JSR 303 Parameter Validation with Spring
Java developers often grapple with the intricate world of parameter validation, especially when working with Spring Framework in conjunction with JSR 303 (Bean Validation). While these technologies provide powerful tools to ensure data consistency and integrity, developers can easily stumble into common traps that lead to unexpected behavior. This blog post aims to unpack these pitfalls and provide essential insights for efficient parameter validation in your Spring applications.
Understanding JSR 303 and Spring Validation
JSR 303, also known as Bean Validation, defines a specification for validating Java beans using annotations. Spring integrates seamlessly with this specification, allowing developers to apply validation annotations directly to model objects.
Here are some widely-used validation annotations defined in the JSR 303 specification:
@NotNull
: Ensures that a field is not null.@Size
: Specifies that the length of a string must fall within certain bounds.@Min
and@Max
: Constrains numeric values to a specified range.
Example Validation Annotation Usage
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
public class User {
@NotNull(message = "Username cannot be null")
@Size(min = 3, max = 20, message = "Username must be between 3 and 20 characters")
private String username;
// Getters and Setters omitted for brevity
}
In this snippet, the User
class uses annotations to specify constraints on the username
field. When User
instances are validated, a non-compliant username will trigger an error, which can be further processed in your application.
Common Pitfalls
1. Failing to Enable Validation
Before you can use JSR 303 validation effectively, it is crucial to enable validation in your Spring application. This is often overlooked and can cause validation rules to be ignored completely.
How to Enable Validation
In a Spring application, you need to annotate your controller methods with @Valid
. Here's how you do it:
import org.springframework.validation.annotation.Validated;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/users")
public class UserController {
@PostMapping
public ResponseEntity<Void> create(@Valid @RequestBody User user) {
// Logic to create user
return new ResponseEntity<>(HttpStatus.CREATED);
}
}
By using @Valid
, Spring ensures the User
object is validated before entering the method.
2. Missing Error Handling
When validation fails, it’s essential to capture and handle the error messages properly. Developers commonly forget to include the necessary handling, leading to confusion for users, who receive no feedback regarding validation failures.
Example of Proper Error Handling
import org.springframework.web.bind.MethodArgumentNotValidException;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ControllerAdvice;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(MethodArgumentNotValidException.class)
public ResponseEntity<String> handleValidationExceptions(MethodArgumentNotValidException ex) {
return ResponseEntity.badRequest()
.body(ex.getBindingResult().getAllErrors()
.get(0).getDefaultMessage());
}
}
In this example, we intercept any MethodArgumentNotValidException
and return the first validation error message back to the client, improving user experience.
3. Ignoring Group Validation
JSR 303 supports validation groups, which can be useful when different types of validation are needed for the same model object. Ignoring this feature can make your validation less flexible, forcing developers to create multiple classes instead.
Implementing Group Validation
public interface CreateGroup {}
public interface UpdateGroup {}
public class User {
@NotNull(groups = CreateGroup.class)
private String username;
@NotNull(groups = UpdateGroup.class)
private String email;
// Other fields and methods
}
Validation in the Controller
@PostMapping
public ResponseEntity<Void> create(@Validated(CreateGroup.class) @RequestBody User user) {
// Logic for creating a user
return new ResponseEntity<>(HttpStatus.CREATED);
}
@PutMapping
public ResponseEntity<Void> update(@Validated(UpdateGroup.class) @RequestBody User user) {
// Logic for updating a user
return new ResponseEntity<>(HttpStatus.OK);
}
This approach allows distinct validation logic based on the context in which your model object is being used.
4. Overusing Validation Annotations
While validation is vital, overdoing it can introduce complexity. Adding too many validation annotations can make your model classes hard to maintain and less readable.
Consider only applying validations that truly provide benefit. This also improves performance, as validating many fields can slow down processing speed unnecessarily.
5. Not Considering Performance Aspects
Invalidations can cause decreased performance when many entities undergo validation in high-load scenarios.
To mitigate this, consider the following best practices:
- Batch validation when dealing with collections
- Utilize “lazy validation” techniques by validating only before persisting entities to the database
6. Not Handling Cross-field Validation
While JSR 303 is excellent for single-property validations, it can fall short in scenarios where validation depends on other fields in your object.
Example of Cross-field Validation
To handle this capability, you may need to create custom validators:
import javax.validation.Constraint;
import javax.validation.Payload;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Constraint(validatedBy = UserValidator.class)
@Target({ ElementType.TYPE })
@Retention(RetentionPolicy.RUNTIME)
public @interface ValidUser {
String message() default "Invalid user details";
Class<?>[] groups() default {};
Class<? extends Payload>[] payload() default {};
}
Implementing the Validator Logic
import javax.validation.ConstraintValidator;
import javax.validation.ConstraintValidatorContext;
public class UserValidator implements ConstraintValidator<ValidUser, User> {
@Override
public boolean isValid(User user, ConstraintValidatorContext context) {
return user.getUsername() != null && user.getEmail() != null && user.getUsername().equals(user.getEmail());
}
}
In this hypothetical example, we've created a rule that states a user's username must match their email. By implementing such custom validators, you increase the robustness of your data validation strategy.
My Closing Thoughts on the Matter
Navigating through JSR 303 parameter validation with Spring requires awareness of its common pitfalls. Understanding these challenges and how to address them leads to cleaner code, better performance, and a more user-friendly application.
To delve deeper into validation and learn about its intricate details, consider exploring additional resources such as Spring's reference documentation and Bean Validation. Happy coding!