How Java 10's Features Impact Legacy Code Maintenance
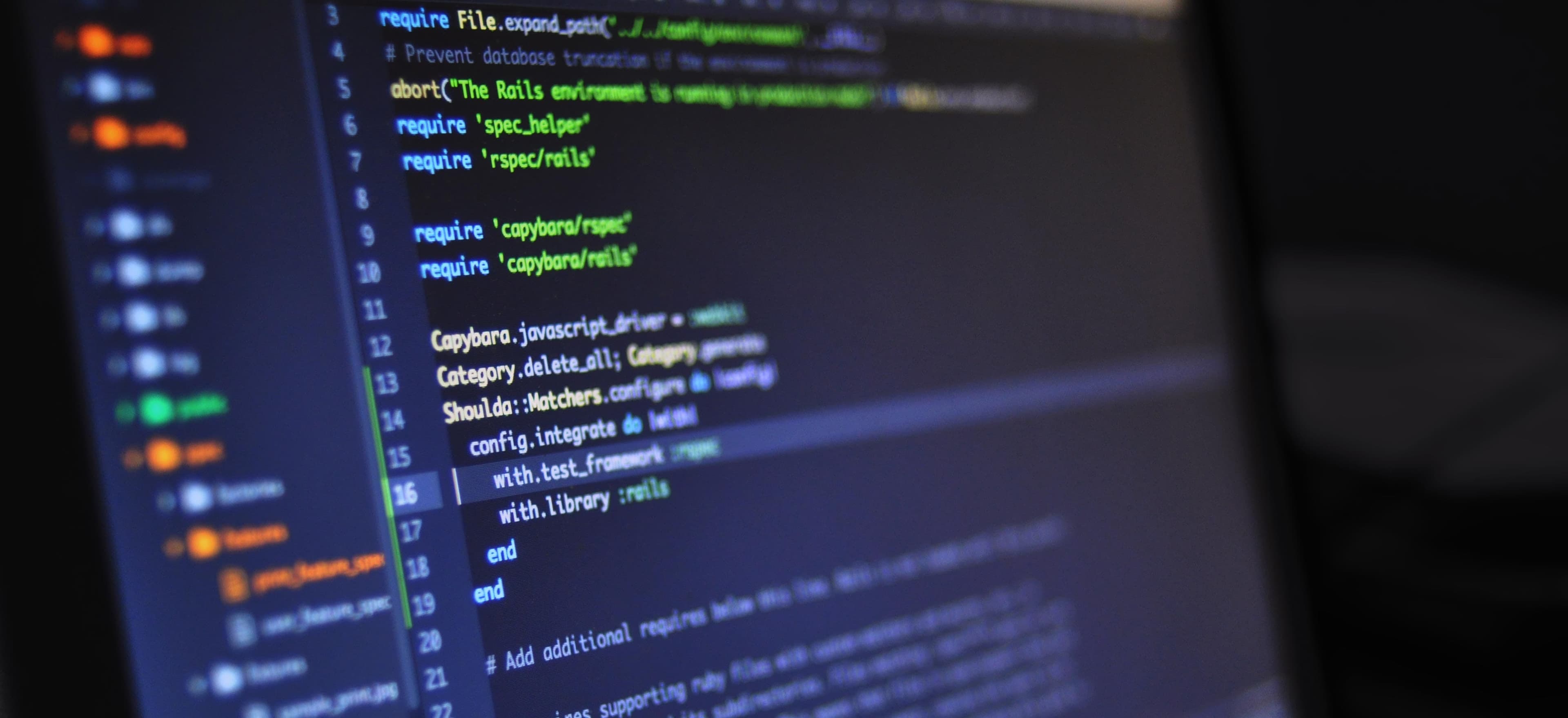
- Published on
How Java 10's Features Impact Legacy Code Maintenance
Java is a programming language that has been a staple in the software development world for over two decades. With its intrinsic design principles of portability, security, and performance, Java has established itself in a myriad of applications. However, a significant portion of the Java ecosystem consists of legacy code — older systems that often require maintenance and updates. With the advent of newer versions like Java 10, developers face the dual challenge of maintaining legacy code as well as adopting modern features. In this article, we will delve into how Java 10's features impact legacy code maintenance, enhancing its quality and performance without breaking the existing systems.
Overview of Java 10
Before we dive deep, let’s explore what Java 10 brought to the table. Released in March 2018, Java 10 introduced several features that primarily focused on performance enhancements, added syntactical sugar, and improved the way developers interact with Java's core libraries.
Some noteworthy features include:
- Local Variable Type Inference (var)
- Application Class-Data Sharing (AppCDS)
- Garbage-Collector Interface
- Thread-Local Handshakes
These features smoothly interlace with both new and legacy codebases.
1. Local Variable Type Inference (var)
One of the standout features of Java 10 is the introduction of local variable type inference using the var
keyword. Instead of explicitly declaring the type of a variable, developers can let the compiler infer the type, which can improve code readability.
Example Code Snippet
var numberList = new ArrayList<Integer>();
Why It Matters:
In legacy code, you might find overly verbose declarations, especially with generic classes, making the code appear cluttered. The var
keyword significantly reduces verbosity, enhancing clarity while keeping the type safety that Java offers.
Consideration:
While it reduces verbosity, developers must use var
judiciously. Clear and descriptive types should still be the standard for readability, especially in a legacy context where many developers may be involved in the project lifecycle.
2. Application Class-Data Sharing (AppCDS)
Java 10 introduced AppCDS, which allows for sharing class metadata across different Java applications. This feature is particularly advantageous in environments where multiple Java applications run on the same JVM.
Example Code Snippet
java -XX:SharedArchiveFile=hello.jsa -XX:+UseAppCDS -cp app.jar com.example.App
Why It Matters:
For legacy systems, performance can often be a bottleneck. AppCDS can reduce startup time by sharing data between processes. In environments with heavy traffic or high-load applications, this can prevent memory overhead and lead to performance improvements.
Consideration:
Legacy code often comes with extensive class libraries. AppCDS can help, but transitioning can require some initial setup and testing. Smaller incremental updates can mitigate risks associated with broader rewriting sessions.
3. Garbage-Collector Interface
Java 10 also introduced a more consistent interface for various garbage collectors. This allows developers to easily switch between different garbage collection options without having to delve into the specifics of each implementation.
Example Code Snippet
// Switch to G1 collector
java -XX:+UseG1GC YourJavaApp
Why It Matters:
Legacy applications running Java often suffer from performance issues due to inefficient garbage collection. The new GC interface allows developers to experiment and profile different garbage collection methods with ease.
Consideration:
However, as legacy applications might have unseen dependencies on older GC behaviors, it is critical to perform thorough testing before making such transitions.
4. Thread-Local Handshakes
Thread-Local Handshakes is a more fine-grained approach to safely stop and interact with Java threads. This feature can ease the implementation of safe stops, interrupt signals, or other interrupts, which are essential in long-running legacy applications.
Example Code Snippet
// Trigger a local handshake for a thread
Thread thread = new Thread(() -> {
// Perform operations
try {
Thread.sleep(100);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
thread.start();
Why It Matters:
Legacy systems often face challenges with interrupt safety during long-running operations. With the introduction of Thread-Local Handshakes, it becomes easier to manage thread state without jeopardizing the system integrity.
Consideration:
Migration can be tricky if the legacy codebase hasn't been structured with thread interruptions in mind. Therefore, it's essential to evaluate existing code patterns before making extensive changes.
Challenges and Considerations for Legacy Systems
Transitioning to Java 10 will have its overrides and hurdles. The following factors should be in mind during such transitions:
1. Compatibility
Java 10 retained a high level of backward compatibility; however, using modern features indiscriminately can lead to runtime issues. Legacy code often involves outdated dependencies, which may not support new features.
2. Resource Constraints
Legacy systems often have limited resources, both in terms of hardware and developer expertise. Upgrading requires thoughtful planning and execution — a 'big bang' approach can lead to downtime and performance degradation.
3. Documentation and Knowledge
You'll find that many legacy systems are poorly documented. Upgrading to newer Java features may require developers to unearth a significant amount of knowledge. Collaborating with domain experts can mitigate these gaps.
Closing the Chapter
Java 10's features present an exciting frontier for legacy code maintenance. The tools at its disposal offer significant improvements in productivity and performance while being adaptable to the existing systems. However, as exciting as the prospect of modernization might be, caution is warranted in transitioning.
By leveraging features such as Local Variable Type Inference, AppCDS, Garbage-Collector Interface, and Thread-Local Handshakes, developers can enhance their codebases while also navigating the complexity that legacy systems often present.
For further reading on how to effectively manage legacy code, consider visiting Martin Fowler's Refactoring or Wikipedia's Resource on Legacy Code.
By thoughtfully implementing Java 10's features, legacy code doesn't have to be a stumbling block; instead, it can pave the way for modern practices and enhanced application performance.
A Call to Action
Embrace the engage-and-adapt culture. Make your legacy Java applications cleaner and more maintainable, one feature at a time!
Checkout our other articles