Common Pitfalls When Using Java Streams: Avoid These Mistakes
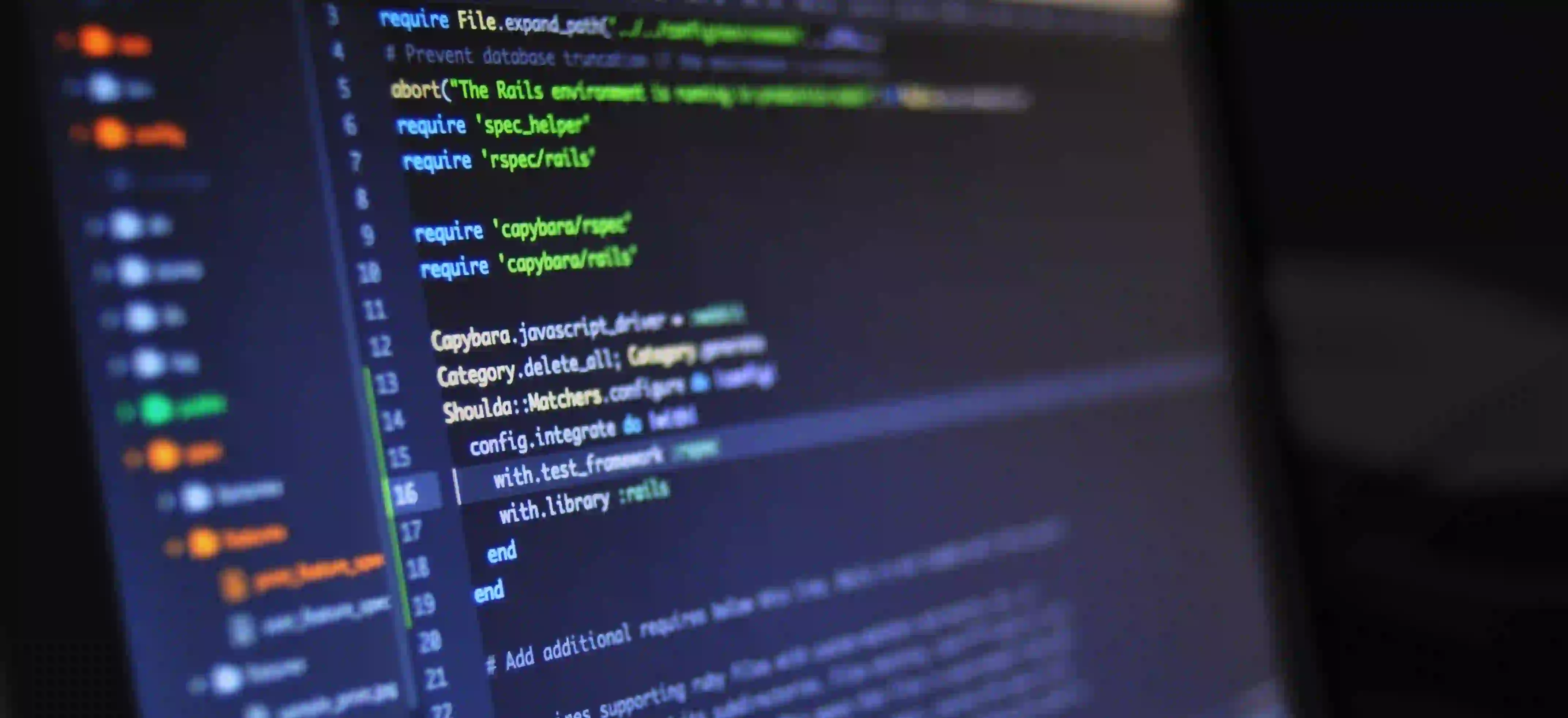
Common Pitfalls When Using Java Streams: Avoid These Mistakes
Java Streams, a powerful feature introduced in Java 8, provide a way to process sequences of elements in a functional style. While Stream API greatly enhances code readability and efficiency, it is easy to fall into common pitfalls if you're not careful. In this blog post, we'll discuss these mistakes, providing code snippets and best practices to help you navigate Java Streams effectively.
What Are Java Streams?
Java Streams allow developers to work with sequences of data in a manner that supports complex operations like filtering, mapping, reducing, and more, often in a more readable form. They also promote a declarative approach, making your code more expressive and easier to understand.
Quick Example
Here’s a quick example of using Java Streams to filter a list of integers, keeping only even numbers:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers); // Outputs: [2, 4, 6]
}
}
In this example, we converted a list of integers into a stream, filtered out the odd numbers, and collected the results back into a list. Here, filter
and collect
are essential methods that we will dive deeper into.
1. Not Closing Resources
A common mistake developers make is forgetting to close resources when using streams. Streams themselves do not hold resources open, but if you’re using Files.lines()
or similar, failing to close the underlying resource can lead to memory leaks. Always use try-with-resources when working with such streams.
Example
import java.io.BufferedReader;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class StreamResourceExample {
public static void main(String[] args) {
Path path = Paths.get("data.txt");
try (BufferedReader br = Files.newBufferedReader(path)) {
br.lines().forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Using try-with-resources ensures that the BufferedReader
is closed automatically, thus preventing potential memory leaks.
2. Using Stateful Lambda Expressions
Stateful lambda expressions can lead to unpredictable results. For instance, modifying a variable outside of the lambda can produce undesired effects, such as incorrect filtering.
Wrong Approach
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
final List<Integer> evenNumbers = new ArrayList<>();
numbers.stream().filter(n -> {
if (n % 2 == 0) {
evenNumbers.add(n); // Modifying an outer variable
return true;
}
return false;
}).collect(Collectors.toList());
In the above code, the lambda modifies a variable from the outer scope, which can lead to concurrency issues if streams are run parallel.
Right Approach
Instead, aim for a stateless lambda:
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
This version clearly separates concerns and avoids side-effects.
3. Overusing Parallel Streams
Parallel streams can improve performance, but they are not a one-size-fits-all solution. They are beneficial when processing large data sets with independent tasks, but using them excessively can lead to overhead.
Cautionary Example
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> evenNumbers = numbers.parallelStream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
Using parallelStream()
here could introduce unnecessary complexity if your dataset isn't sufficiently large or if your processing logic is light.
Tip
Before opting for parallel streams, measure your processing time. Always consider the trade-off between readability and performance.
4. Ignoring Optional
Streams often return Optional
objects when retrieving single elements. One common pitfall is not handling the Optional
correctly, leading to NoSuchElementException
.
Example
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
String firstNameWithA = names.stream()
.filter(name -> name.startsWith("A"))
.findFirst()
.get(); // Risky usage
System.out.println(firstNameWithA);
Using .get()
without checking if a value is present can lead to runtime exceptions.
Correct Usage
String firstNameWithA = names.stream()
.filter(name -> name.startsWith("A"))
.findFirst()
.orElse("No names found");
System.out.println(firstNameWithA); // Outputs: Alice
Using orElse()
provides a safe default, thus avoiding potential exceptions.
5. Not Collecting Stream Results
Often, developers forget to collect their results, leaving streams unconsumed. An unconsumed stream will not yield any output and will simply traverse the data without saving or displaying any results.
Example
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.stream()
.filter(n -> n % 2 == 0);
// Result is not collected
The stream processes the numbers, but without a terminal operation like collect()
, forEach()
, or similar, no results are produced.
Right Way
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers); // Outputs: [2, 4]
Always remember to include a terminal operation to ensure your stream processing yields results.
Closing Remarks
Java Streams provide a modern approach for processing collections of data, making coding more efficient and readable. However, to harness their full potential, developers should be aware of the common pitfalls outlined in this blog post.
By avoiding the mistakes of neglecting to close resources, using stateful lambdas, overusing parallel streams, mishandling Optional
, and forgetting to collect results, you can significantly improve the reliability and performance of your Java applications.
For more insights, consider checking out the official Java documentation on streams, or dive deeper into functional programming concepts in Java to enhance your understanding.
Call to Action
Do you have more insights or questions about working with Java Streams? Share your thoughts and experiences in the comments below, or connect with us on social media!
Happy coding!