Unlocking the Secrets of btrace for Java Developers
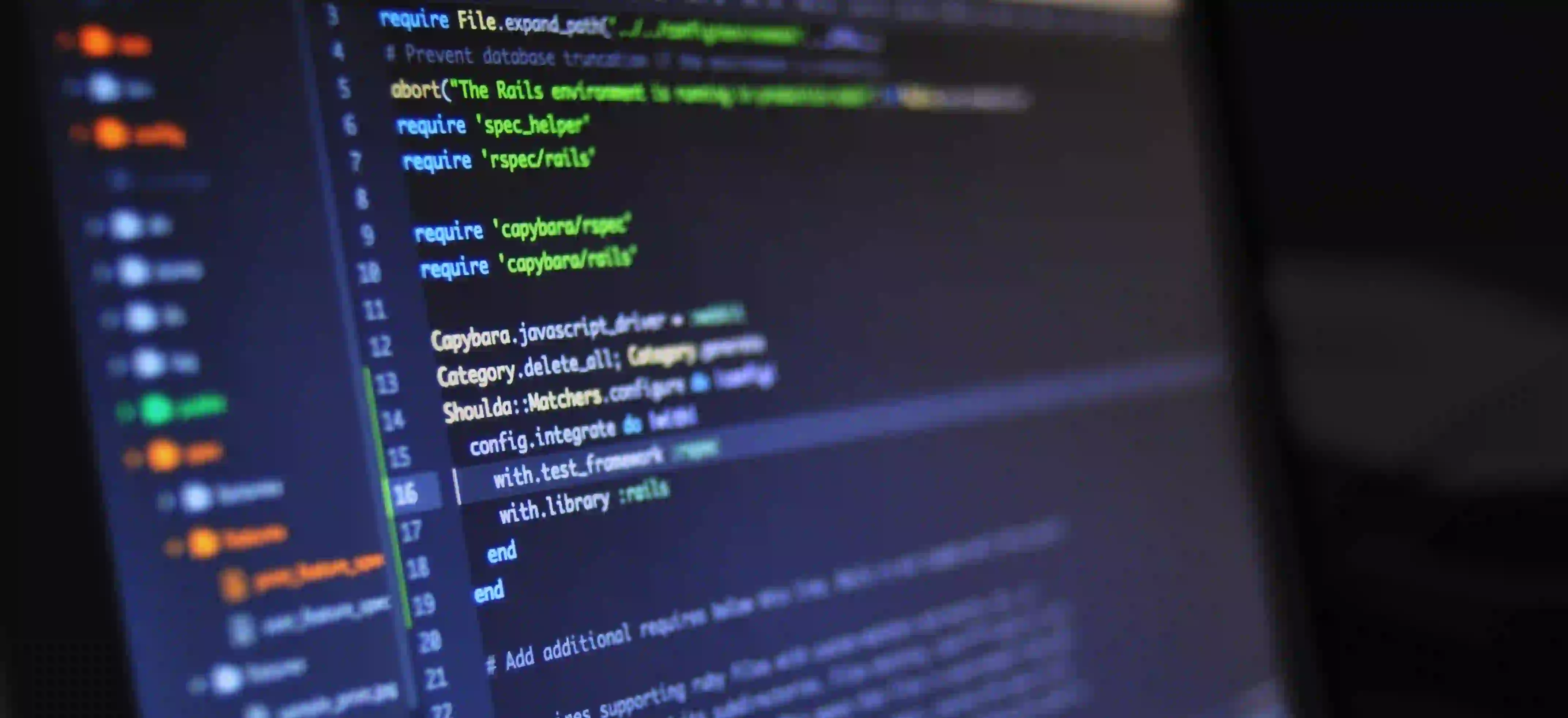
Unlocking the Secrets of btrace for Java Developers
Java developers are constantly looking for ways to debug and optimize their applications. Among the myriad of tools available, btrace stands out as a unique solution that allows developers to collect dynamic tracing information from their running Java applications without requiring any modifications to the code. In this blog post, we will delve into what btrace is, how it works, and showcase some practical examples to help you leverage this powerful tool in your Java development toolkit.
What is Btrace?
Btrace is a powerful and lightweight tool that allows developers to trace Java applications in real-time. It uses Java Instrumentation to inject Java code into a running application for monitoring and diagnostics purposes. This means that you can trace the behavior of your application without stopping it, making it invaluable in production environments where uptime is critical.
The primary goal of btrace is to provide developers with real-time insights into the application's runtime behavior. This can include method invocation counts, timing logic, catching exceptions, and tracking memory consumption, among other metrics.
Why Use Btrace?
-
No Code Changes: Btrace allows you to trace applications without altering the source code or redeploying.
-
Dynamic Analysis: You can add or remove trace points on-the-fly.
-
Low Overhead: Designed to have minimal impact on performance, btrace is suitable for production systems.
-
Flexible Scripting: It allows for dynamic scripting using Java syntax, enabling customized monitoring solutions tailored to specific needs.
-
Rich Output: Supports various types of output, such as logging to the console or writing to files.
Getting Started with Btrace
Installation
Ensure you have Java Development Kit (JDK) installed on your machine. You can install btrace by downloading it from the official btrace GitHub repository.
Once downloaded, follow these simple steps for setup:
- Unzip the btrace archive.
- Set the path to the btrace binary by adding it to your system's
PATH
variable. - Start a simple Java application to use as a target for tracing.
Example Java Application
Let's create a simple Java application to illustrate how btrace works. Below is a basic Java implementation simulating a service.
public class SimpleService {
public void processRequest(String request) {
try {
Thread.sleep(100); // Simulating processing delay
System.out.println("Processed request: " + request);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
SimpleService service = new SimpleService();
for (int i = 0; i < 10; i++) {
service.processRequest("Request " + i);
}
}
}
Writing Your First Btrace Script
Now that you have a sample application, let's create a btrace script to trace the processRequest
method. The script will log the method's entry and the input parameters.
Create a file named TraceScript.java
with the following content:
import com.sun.btrace.annotations.*;
import static com.sun.btrace.BTraceUtils.*;
@BTrace
public class TraceScript {
@OnMethod(
clazz = "SimpleService",
method = "processRequest",
location = @Location(Kind.ENTRY)
)
public static void onEntry(String request) {
println(strcat("Entering processRequest with request: ", request));
}
}
Explanation of the Btrace Script
- @BTrace: Indicates the class is a btrace script.
- @OnMethod: Specifies the target method to trace. Here, we are interested in the
processRequest
method in theSimpleService
class. - @Location(Kind.ENTRY): Specifies that we want to trigger the action when the method is entered.
- println: Logs output to the standard output, helping us understand what's happening at runtime.
Running the Btrace Script
- Compile your
TraceScript.java
:
javac -cp /path/to/btrace.jar TraceScript.java
- Start your SimpleService application:
java SimpleService
- Attach btrace to the running process:
btrace <pid_of_SimpleService> TraceScript.class
(replace <pid_of_SimpleService>
with the actual process ID).
Analyzing the Output
Once you run the above steps, you will see log entries in your console showing the inputs to the processRequest
method in real-time. This provides immediate feedback, allowing you to troubleshoot issues or monitor application behavior without the need to stop the service.
Advanced Btrace Features
Method Exit Tracing
You might want to know when a method exits along with its return value. You can achieve this by modifying the previous script to include an exit point as follows:
@OnMethod(
clazz = "SimpleService",
method = "processRequest",
location = @Location(Kind.EXIT)
)
public static void onExit(String request) {
println(strcat("Exiting processRequest with request: ", request));
}
This addition will log the exit point, giving you comprehensive insight into the method's lifecycle.
Exception Tracing
Another valuable feature is to capture exceptions that occur in your methods. You can enhance your script like so:
@OnMethod(
clazz = "SimpleService",
method = "processRequest",
location = @Location(Kind.THROW)
)
public static void onThrow(Throwable t) {
println(strcat("Exception thrown in processRequest: ", str(t)));
}
This will log any exceptions that bubble up during the execution, providing help in debugging errors.
Final Considerations
Btrace is an exceptional tool for Java developers looking to probe their applications' runtime behavior dynamically. The ability to execute tracing scripts without modifying existing code is invaluable, especially when you seek to troubleshoot issues in production.
In this guide, we've covered the basic steps to set up and run btrace, provided a starting example, created useful scripts, and explored advanced features. Embracing btrace can enhance your debugging capabilities and enrich your Java development experience.
For more detailed insights into Java Instrumentation, check out the Java Instrumentation API documentation. To further explore btrace capabilities, you can consult the official btrace GitHub repository for more advanced usage.
Start using btrace today, and unlock the secrets of your Java applications!