Navigating the Confusion of Generics in Programming
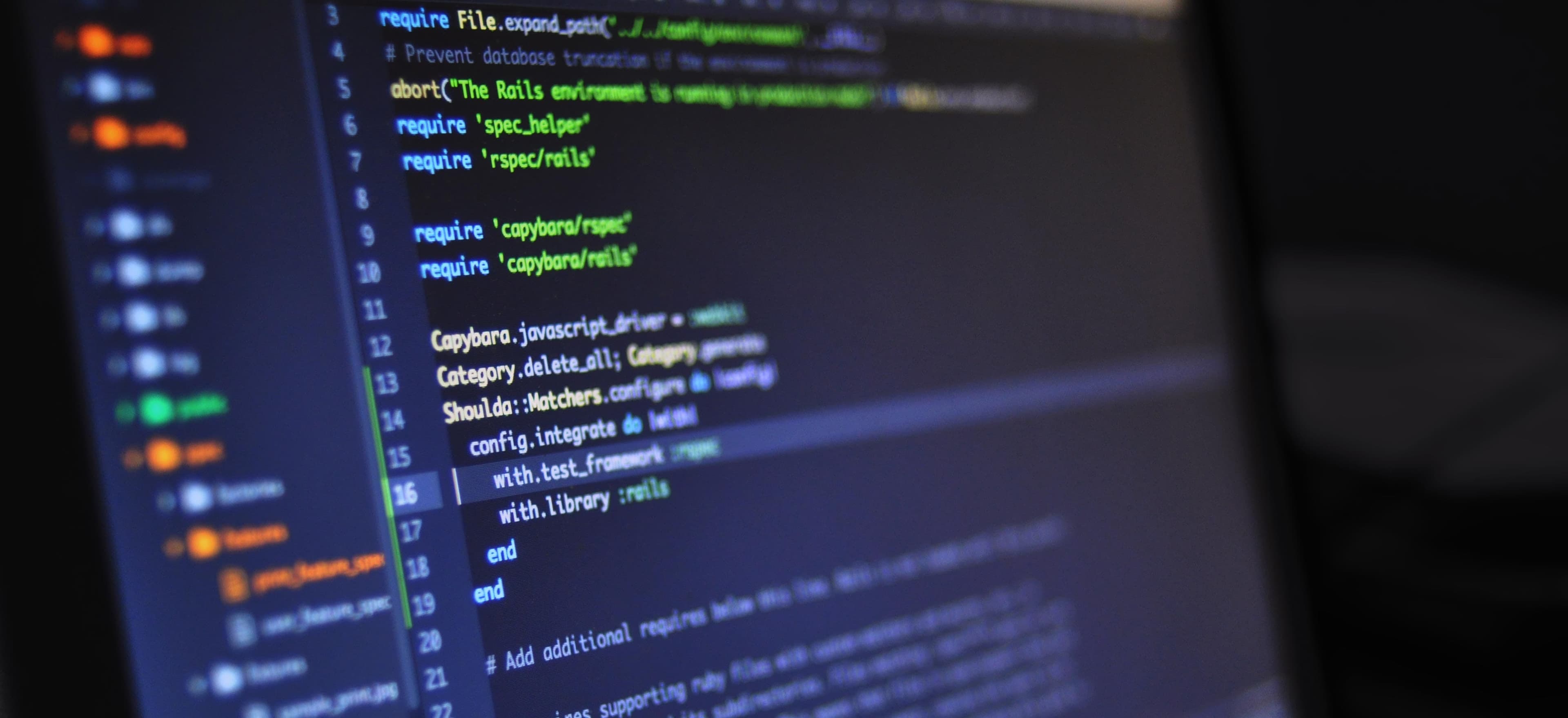
- Published on
Navigating the Confusion of Generics in Programming
Generics are a powerful feature of many programming languages, including Java. They allow developers to create classes, interfaces, and methods that operate on types specified by the user, increasing code reusability and type safety. However, generics can also introduce confusion, especially for those new to the concept. In this blog post, we'll unravel the complexities of generics in Java, provide useful examples, and explain why they matter.
What are Generics?
Generics enable the definition of classes and methods with a placeholder for a type that will be specified later. This is particularly useful for collections, where the type of elements can vary. The primary benefit is stronger type checks at compile time, reducing the risk of ClassCastExceptions at runtime.
Example of a Generic Class
Consider the following generic class:
public class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
Why Use Generics?
- Type Safety: The compiler can detect type mismatches, leading to fewer runtime errors.
- Code Reusability: You can write a method or class once and reuse it for different types.
- Cleaner Code: Avoids the need for explicit casting, making the code clearer and easier to read.
How Generics Work
In the Box
class example, T
is a type parameter. When you create an instance of Box
, you specify the type you’d like to use.
Box<String> stringBox = new Box<>();
stringBox.setItem("Hello World");
String item = stringBox.getItem(); // No cast needed
The String
type replaces the type parameter T
, meaning stringBox
is now specifically a Box
of String
. If a programmer tries to set an Integer
in this box, the compiler will throw an error, safeguarding the type.
Understanding Bounded Type Parameters
Sometimes you want to restrict the types that can be used in a generic class or method. This is where bounded type parameters come in. You can use keywords such as extends
to limit the types to a specific class or its subclasses.
Example of Bounded Type Parameters
public class Box<T extends Number> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
In this modified version, T
can only be a subtype of Number
, which includes Integer
, Double
, etc.
Why Bounded Types are Useful
- Specific Functionality: By restricting types, you can call methods specific to the bounded type. For example, using
doubleValue()
on aNumber
type item. - Improve API Design: Improves API clarity by making expectations about generics clear.
Wildcards in Generics
The wildcard (?
) is another powerful tool in generics. Wildcards allow flexibility in code without sacrificing type safety. Wildcards can be useful in various scenarios, particularly when you want to allow multiple different types.
Example of Wildcards
public static void printBox(Box<?> box) {
System.out.println(box.getItem());
}
In this method, printBox
can accept a Box
containing any type of item.
Why Use Wildcards?
- More Flexibility: You can work with a variety of types without creating multiple overloaded methods.
- Maintain Type Safety: Wildcard usage is still type-safe, so you avoid issues with invalid type assignments.
Upper and Lower Bounded Wildcards
In addition to simple wildcards, Java provides upper and lower bounded wildcards:
- Upper Bounded Wildcards
Using extends
, we indicate that the type must be a specific type or its subtype.
public static void processBox(Box<? extends Number> box) {
// Only Number or its subclasses can be processed here
}
- Lower Bounded Wildcards
Using super
, we indicate something broader, allowing us to write to the box.
public static void addBoxItem(Box<? super Integer> box) {
box.setItem(10); // Can add Integer or its subclass
}
Why Upper and Lower Bounded Wildcards Matter
- Enhanced Flexibility: They allow precise definitions that align closely with the desired behavior.
- Code Safety: You can confidently manipulate objects knowing their bounds.
Common Pitfalls with Generics
Despite the benefits, generics can mislead if misunderstood. Here are some common pitfalls:
-
Raw Types: Using a generic class without specifying a type erases the genericity, losing type safety. Avoid this:
Box rawBox = new Box(); // Raw types lead to warnings and potential runtime errors
-
Type Erasure: Java uses type erasure for generics, meaning generic type information is not available at runtime. This can sidetrack debugging efforts.
-
Incompatibility with Primitive Types: Generics do not support primitive data types directly. You'll need to use their wrapper classes (
Integer
,Double
, etc.).
Final Considerations
Generics enhance Java's type system, allowing for greater reusability, safety, and clarity. Understanding their nuances—from basic generic types to bounded wildcards—empowers developers to write more robust code.
To further explore the concepts presented in this article, consider reading through the Java Generics documentation and Effective Java by Joshua Bloch, which offers deep insights into best practices.
By mastering generics, you'll not only write better Java code but also contribute to maintaining high-quality, scalable software development practices. Don't let confusion hold you back—embrace the power of generics today!