“Troubleshooting DynamoDBMapper Insert Errors”
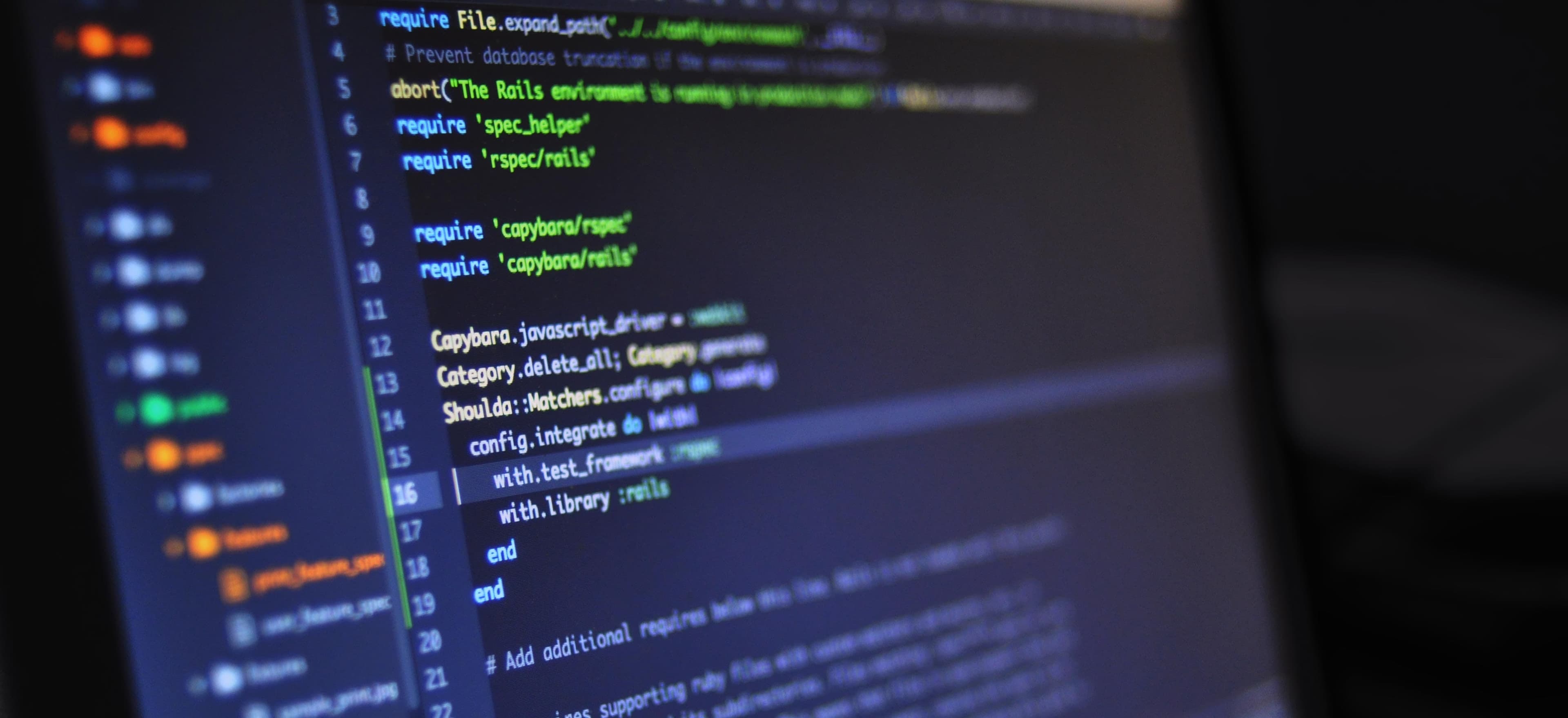
- Published on
Troubleshooting DynamoDBMapper Insert Errors
Amazon DynamoDB is a fully managed, serverless, key-value NoSQL database service designed to deliver single-digit millisecond performance at any scale. When working with the AWS SDK for Java, one commonly-used utility for interfacing with DynamoDB is the DynamoDBMapper
class. This powerful tool simplifies the interaction with DynamoDB by automatically handling conversions between your Java objects and DynamoDB items.
However, developers sometimes encounter insert errors while trying to save data to DynamoDB using DynamoDBMapper
. In this blog post, we'll discuss common errors and their troubleshooting steps, enriched with code snippets to illustrate each point.
Understanding DynamoDBMapper
Before diving into troubleshooting, it's essential to understand the basics of DynamoDBMapper
. This class allows you to map Java objects to DynamoDB tables. Here’s a quick look:
import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBMapper;
public class DynamoDBExample {
private final DynamoDBMapper dynamoDBMapper;
public DynamoDBExample(DynamoDBMapper dynamoDBMapper) {
this.dynamoDBMapper = dynamoDBMapper;
}
}
In the snippet above, we create a constructor that accepts a DynamoDBMapper
instance. This instance will be used for performing various CRUD (Create, Read, Update, Delete) operations.
Common Insert Errors
Now, let’s look at some common errors and how to resolve them.
1. Conditional Check Failed
Error Message: ConditionalCheckFailedException
This exception arises when you use a conditional expression (like an attribute existence check) that fails during the insert.
Example
You might see this error when trying to insert an item with an attribute that must not exist.
MyItem item = new MyItem();
item.setId("123");
item.setName("Test");
// Attempt to save with a condition: item should not already exist
dynamoDBMapper.save(item, new DynamoDBSaveExpression()
.withConditionExpression("attribute_not_exists(id)"));
Troubleshooting Steps
- Confirm Existence: Ensure that the item with the same key does not already exist.
- Adjust Condition: Modify or remove the conditional expression if the existence check is not required.
2. Validation Errors
Error Message: AmazonDynamoDBException: ValidationException
Validation exceptions occur if the data being inserted does not conform to the defined schema or constraints in DynamoDB.
Example
Let’s say we have a string attribute defined to be between 1 and 10 characters long:
public class MyItem {
private String id; // Primary key
@DynamoDBAttribute
@Size(min=1, max=10) // Here is our constraint
private String name;
// Getters and Setters removed for brevity
}
If you attempt to save an item with a name longer than 10 characters, you'll encounter a validation error.
Troubleshooting Steps
- Check Constraints: Review the attributes and ensure they fit within the constraints established in your Java class using annotations (like
@Size
). - String Length: Validate string lengths before the save operation.
3. Mapper Configuration Errors
Error Message: AmazonDynamoDBException: Mapper configuration exception
Misconfigurations in your DynamoDBMapper
setup can lead to runtime issues. This often happens when specific mappings are incorrectly defined.
Example
If you define an incorrect primary key, your insert may fail:
@DynamoDBTable(tableName = "MyTable")
public class MyItem {
@DynamoDBHashKey
private String incorrectId; // Mistaken primary key
...
}
Troubleshooting Steps
- Correct Annotations: Ensure that your @DynamoDB annotations match your DynamoDB table’s structure. Check hash key and range key configurations.
- Verify Naming: Confirm that your table name is correctly spelled and matches the actual DynamoDB table.
4. Insufficient Permissions
Error Message: AccessDeniedException
This exception is triggered when your AWS IAM roles do not have the required permissions to perform the insert.
Example
Your IAM role should have the necessary permissions to access DynamoDB.
Troubleshooting Steps
- IAM Policy Review: Review the IAM policy attached to your role. Ensure it includes permissions for
dynamodb:PutItem
and any other relevant actions. - Permission Testing: Test the permissions using the IAM Policy Simulator.
5. Network and Configuration Issues
Error Message: AmazonDynamoDBException: Unable to connect
This type of error can occur due to network issues, endpoint misconfigurations, or incorrect AWS region settings.
Troubleshooting Steps
- Check Endpoint: If you're using a specific endpoint, ensure it points to the correct DynamoDB instance.
AWSClientBuilder.standard()
.withEndpointConfiguration(new AwsClientBuilder.EndpointConfiguration("http://localhost:8000", "us-west-2"));
- Internet Access: Confirm the application has internet access if you're using a public endpoint.
Final Thoughts
Insert errors when using DynamoDBMapper
can stem from various sources, including conditional checks, validation rules, configuration errors, insufficient permissions or network issues. By understanding the nature of these errors and applying the outlined troubleshooting steps, you can effectively minimize and resolve these issues.
For further reading, consider checking out the following resources:
By paying close attention to detail and employing best practices, you can turn DynamoDBMapper
into a powerful ally in your software development journey. Happy coding!
Checkout our other articles