Mastering Simple Types in Go: A Guide for Java Programmers
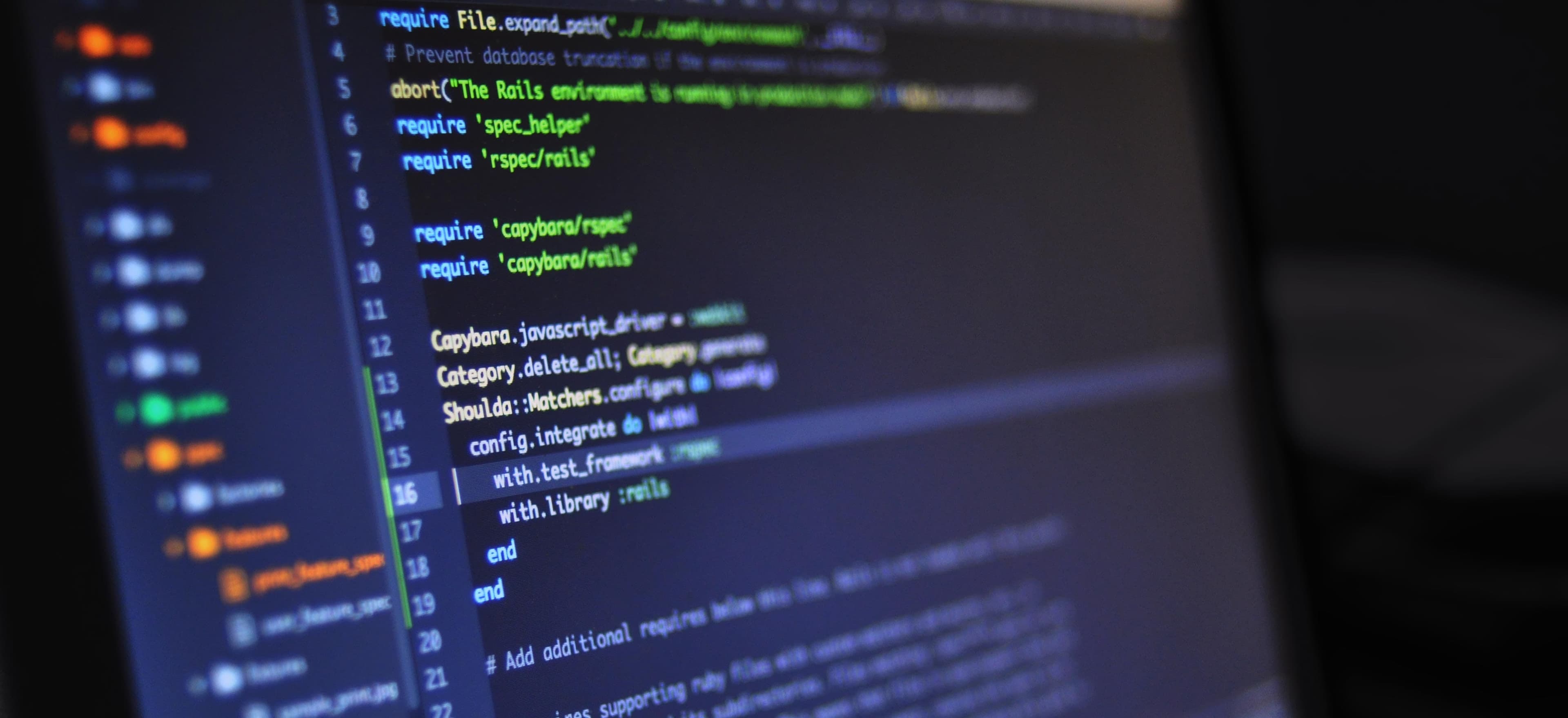
- Published on
Mastering Simple Types in Go: A Guide for Java Programmers
The Go programming language, known for its simplicity, performance, and strong typing, is gaining traction among developers. However, if you're coming from a Java background, you may find some differences in how types are used and declared in Go. This guide explores the simple types in Go, drawing parallels and contrasts with Java to help you transition smoothly.
Understanding Basic Types in Go
In Go, basic types can be broadly categorized into several groups: numeric types, boolean types, and string types. Each type has its unique characteristics and uses.
Numeric Types
Go has various numeric types, including:
- Integers:
int
,int8
,int16
,int32
,int64
- Unsigned Integers:
uint
,uint8
,uint16
,uint32
,uint64
- Floating-point Numbers:
float32
,float64
- Complex Numbers:
complex64
,complex128
The key distinction here is the absence of wrapper classes, like Integer
or Double
in Java. Each type directly maps to a value, which can enhance performance.
Here’s a simple example:
package main
import "fmt"
func main() {
var num int = 42
var pi float64 = 3.14
var flag bool = true
var greet string = "Hello, Go!"
fmt.Println(num, pi, flag, greet)
}
In this snippet, each variable is declared with its specific type. The Go compiler infers the type, so you can also declare them using :=
, like so:
num := 42
pi := 3.14
flag := true
greet := "Hello, Go!"
Comparison with Java
In Java, you’d declare integers or doubles similarly:
int num = 42;
double pi = 3.14;
boolean flag = true;
String greet = "Hello, Java!";
One key difference is that Java uses a primitive vs. object distinction, while Go maintains a simpler type structure.
Why Simple Types Matter
Understanding how Go manages simple types is crucial because it directly impacts performance, memory efficiency, and code readability.
Type Size
An important decision to make when using numeric types is to understand their sizes. For instance, int
in Go can vary in size depending on the architecture (typically 32 or 64 bits). This is unlike Java’s int
, which always occupies 32 bits. Here’s how you can check the size of types in Go:
package main
import (
"fmt"
"unsafe"
)
func main() {
fmt.Println("Size of int:", unsafe.Sizeof(int(0)))
fmt.Println("Size of float64:", unsafe.Sizeof(float64(0)))
}
In this code, we utilize the unsafe
package, which is generally discouraged for regular use but is useful for understanding type sizes.
Strings in Go
Strings in Go are immutable sequences of bytes. When you work with strings, what you actually deal with is a read-only slice of bytes. This contrasts with Java's String
class, which internally uses a character array. Here’s an example of string manipulation in Go:
package main
import "fmt"
func main() {
str := "Hello, Go!"
fmt.Println("Length of string:", len(str))
fmt.Println("First character:", str[0])
// Converting string to a rune slice
runes := []rune(str)
fmt.Println("First character as rune:", runes[0])
}
The ability to treat strings as slices of bytes allows for various manipulation techniques. However, remember that if you're dealing with unicode characters, using rune
is essential.
Why Use Runes?
Runes provide a way to handle multi-byte characters correctly. It’s a crucial aspect of software development, especially in a globalized environment.
Booleans and Control Structures
In Go, the boolean type is straightforward, similar to Java. It can hold true
or false
.
Here's a simple control structure using booleans:
package main
import "fmt"
func main() {
flag := true
if flag {
fmt.Println("The flag is set!")
} else {
fmt.Println("The flag is not set!")
}
}
Go's control flow structures, like if
, for
, and switch
, are easy to follow. Unlike Java, Go doesn't have a separate while
statement, as for
can be used in multiple ways.
Why Simplicity Matters
Go's simplicity in control structures can lead to cleaner code. This approach decreases the cognitive load for developers transitioning from Java, allowing them to focus more on solving problems rather than navigating complex syntax.
Wrapping Up
Transitioning from Java to Go offers an exciting opportunity to master a new language's nuances. Understanding simple types—integers, floats, strings, and booleans—provides a solid foundation.
While Go may lack certain features like generic programming (as of now) compared to Java, its simplicity and efficiency are its greatest strengths. The type system encourages close attention to detail, enhancing performance across the board.
As you dive deeper into Go programming, you will discover more about struct types, interfaces, and concurrency, broadening your expertise.
If you want to learn more about Go types, communities like Golang.org and Go by Example provide excellent resources for building on this knowledge.
Now, go ahead and experiment with Go! Happy coding!
Checkout our other articles