Understanding the Differences: Hashing vs. Encryption
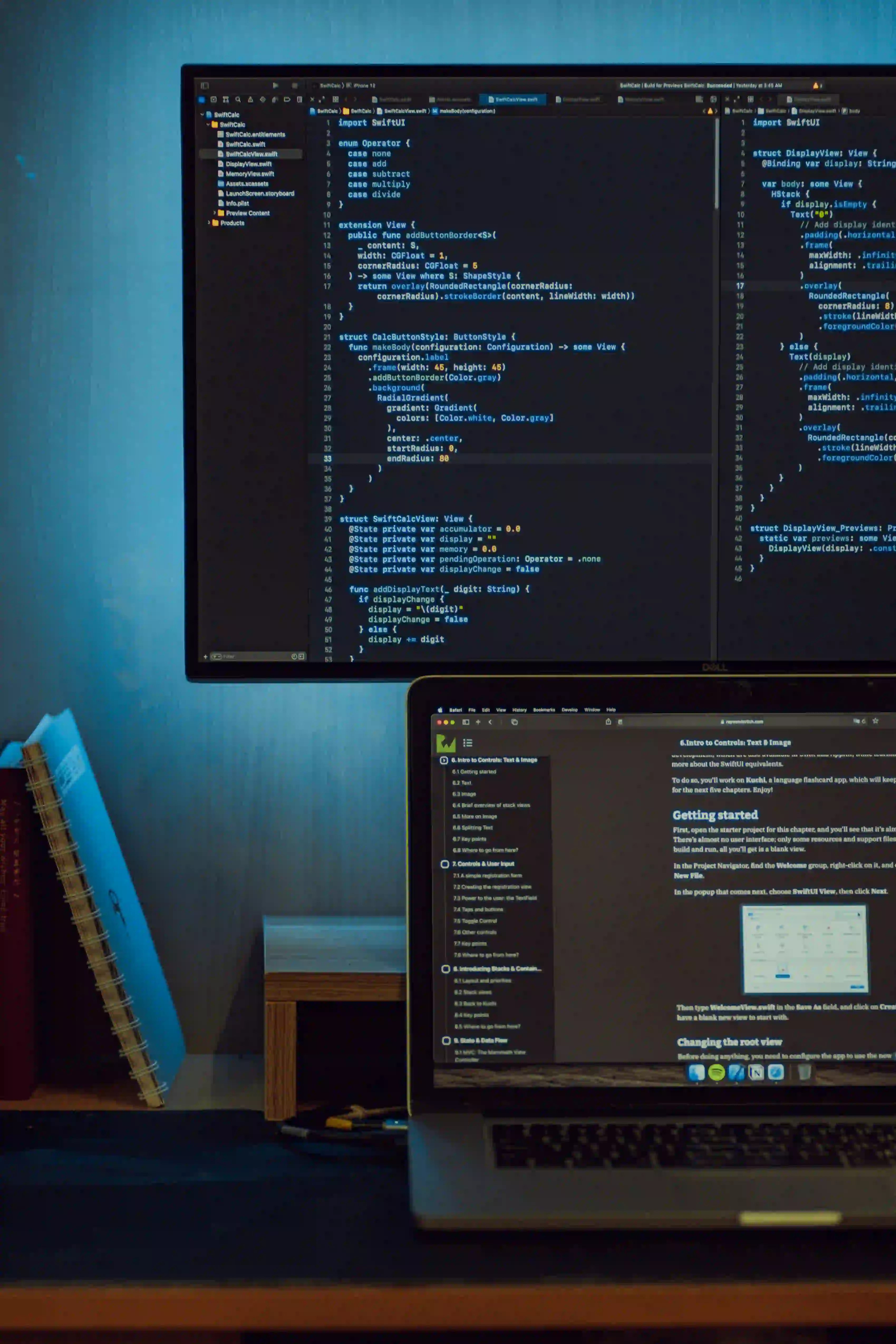
Understanding the Differences: Hashing vs. Encryption
In the world of data security, hashing and encryption are two fundamental concepts that often get conflated. Both methods serve the purpose of safeguarding sensitive information, but they do so in different ways. In this blog post, we’ll explore the differences between hashing and encryption, offering code snippets to enhance our understanding.
What is Hashing?
Hashing is a process that transforms input data into a fixed-length string of characters, which is typically a digest that represents the original data. One of the defining characteristics of hashing is that it is a one-way operation. This means that once data is hashed, it cannot be converted back to its original form. Hash functions are primarily used to ensure data integrity and to quickly look up data in databases.
Key Characteristics of Hashing
- Deterministic: The same input will always produce the same output (hash).
- Fixed Length Output: Regardless of the input size, the output length is fixed.
- One-Way Function: Hashing cannot be reversed to retrieve the original data.
- Collision Resistant: It is difficult to find two different inputs that produce the same output.
When to Use Hashing?
Hashing is commonly used for:
- Password storage
- Data integrity verification (e.g., checksums)
- Digital signatures
Example of Hashing in Java
In Java, the MessageDigest
class can be used to perform hashing. Here’s an example that illustrates how to hash a password using SHA-256:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class HashExample {
public static void main(String[] args) {
String password = "mySecurePassword";
String hashedPassword = hashPassword(password);
System.out.println("Hashed Password: " + hashedPassword);
}
public static String hashPassword(String password) {
try {
// Create a MessageDigest instance for SHA-256
MessageDigest digest = MessageDigest.getInstance("SHA-256");
// Perform hashing
byte[] hashBytes = digest.digest(password.getBytes());
// Convert bytes to hexadecimal format
StringBuilder hexString = new StringBuilder();
for (byte b : hashBytes) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) hexString.append('0');
hexString.append(hex);
}
// Return the hexadecimal string
return hexString.toString();
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException("Error hashing password", e);
}
}
}
Why Use Hashing?
In the above code, we use SHA-256, a widely-used cryptographic hash function. We take a plaintext password, hash it, and print out the hashed version. This ensures that even if the password repository is breached, the attackers won't have access to the actual passwords.
What is Encryption?
Encryption, on the other hand, is a reversible process. It transforms readable data (plaintext) into unreadable data (ciphertext) using a specific algorithm and a key. The key is crucial, as it determines how the data is scrambled. Unlike hashing, encryption allows you to convert the ciphertext back to its original plaintext using the same key (symmetric encryption) or a different key (asymmetric encryption).
Key Characteristics of Encryption
- Reversible: Encrypted data can be decrypted back to its original form.
- Variable Length Output: Depending on the algorithm and input size, output can vary.
- Key-based: Requires a key for both encryption and decryption.
- Protects Confidentiality: Safeguards the data from unauthorized access.
When to Use Encryption?
Encryption is used primarily for:
- Protecting sensitive data (e.g., credit card information)
- Secure communications (e.g., HTTPS)
- File protection
Example of Encryption in Java
The following Java code demonstrates how to encrypt a string using AES (Advanced Encryption Standard):
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.util.Base64;
public class EncryptionExample {
public static void main(String[] args) throws Exception {
String originalText = "Sensitive Information";
SecretKey secretKey = generateKey();
String encryptedText = encrypt(originalText, secretKey);
String decryptedText = decrypt(encryptedText, secretKey);
System.out.println("Original: " + originalText);
System.out.println("Encrypted: " + encryptedText);
System.out.println("Decrypted: " + decryptedText);
}
public static SecretKey generateKey() throws Exception {
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(128); // Specify key size
return keyGen.generateKey();
}
public static String encrypt(String data, SecretKey secretKey) throws Exception {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedBytes = cipher.doFinal(data.getBytes());
return Base64.getEncoder().encodeToString(encryptedBytes);
}
public static String decrypt(String encryptedData, SecretKey secretKey) throws Exception {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(encryptedData));
return new String(decryptedBytes);
}
}
Why Use Encryption?
In this code, we initialize an AES cipher, generate a secret key, and demonstrate how to encrypt and decrypt a string. This ensures that only authorized parties who have the key can access the original information.
Key Differences Between Hashing and Encryption
- Purpose: Hashing is designed to ensure data integrity, while encryption is meant to protect confidentiality.
- Reversibility: Hashing is irreversible, whereas encryption is reversible.
- Key Usage: Hashing does not require a key, while encryption relies on one or more keys.
| Feature | Hashing | Encryption | |----------------------|----------------------------------|--------------------------------| | Purpose | Integrity Check | Data Protection | | Reversibility | Not reversible | Reversible | | Key Usage | No key needed | Requires key | | Output Length | Fixed length | Variable length | | Algorithms | MD5, SHA-1, SHA-256, etc. | AES, RSA, DES |
Lessons Learned
Understanding the differences between hashing and encryption is crucial for anyone dealing with data security. While both techniques serve to protect data, they do so in fundamentally different ways. Choosing the right approach for your needs is essential, whether you're storing passwords securely or transmitting sensitive information over the internet.
For more information, you can refer to the following resources:
With this grasp of hashing and encryption, you're now equipped to make informed decisions about how to secure your data effectively. Always consider your use case and the level of security required before implementing either technique. Happy coding!