Running Into Classpath Conflicts with One Jar Deployments
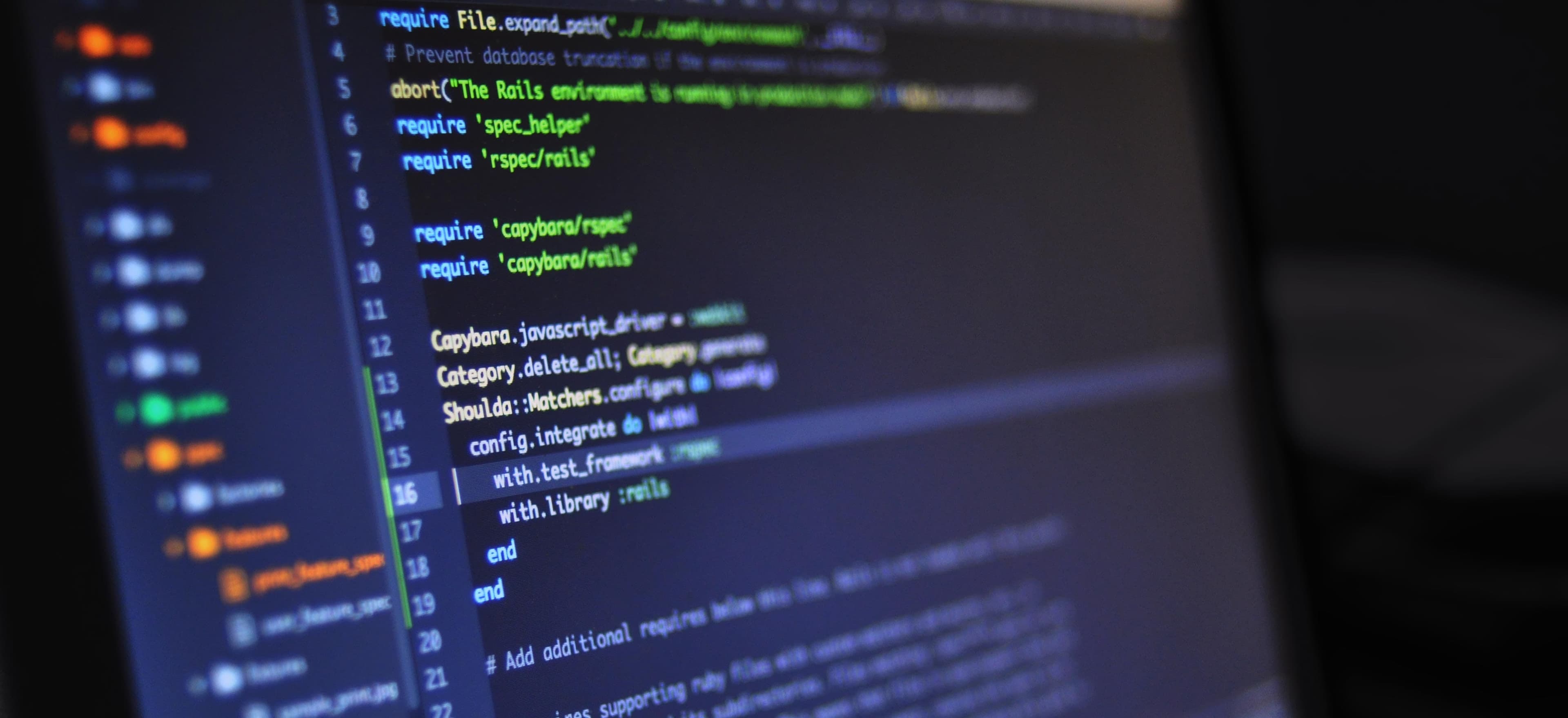
- Published on
Running Into Classpath Conflicts with One Jar Deployments
Java has fundamentally transformed the way we develop applications. One of its more attractive features is the ability to package all dependencies into a single JAR file. This approach is often referred to as "One Jar" or uber JAR deployment. However, as convenient as this method may be, it comes with its own set of challenges, particularly classpath conflicts. In this blog post, we will explore what classpath conflicts are, why they occur, and how to effectively solve them.
What is Classpath?
Before diving into classpath conflicts, let's clarify what a classpath is. In Java, the classpath is a parameter that tells the Java Virtual Machine (JVM) where to find user-defined classes and packages in Java programs. It can be set via the command line and plays a crucial role in how Java applications are executed.
What is One Jar Deployment?
One Jar deployment refers to the practice of packaging your Java application and all its dependencies into a single JAR file. This can be achieved using tools like Maven's Shade Plugin or Gradle's Shadow Plugin. The primary advantage is reducing the complexity of managing multiple JAR files, making deployment simpler.
Sample Gradle Configuration for One Jar
plugins {
id 'java'
id 'com.github.johnrengelman.shadow' version '7.0.0'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.apache.commons:commons-lang3:3.12.0'
}
// Task to create the shaded jar
shadowJar {
archiveBaseName.set('myapp')
archiveVersion.set('1.0.0')
mergeServiceFiles()
}
In the code above, we configure the Shadow Plugin to create a single JAR file containing all the necessary dependencies. The mergeServiceFiles()
method ensures that services defined in multiple libraries are combined.
Understanding Classpath Conflicts
Classpath conflicts occur when multiple libraries (or dependencies) contain classes with the same name and package structure. This can lead to unpredictable behavior, as the Java classloader will resolve to one of those classes, potentially resulting in:
- Class Not Found Exceptions: The classloader fails to locate the necessary class.
- NoSuchMethodError or NoClassDefFoundError: The class exists but the required method or field does not.
Why Do Classpath Conflicts Occur?
-
Transitive Dependencies: When using dependency management systems like Maven or Gradle, it's common for libraries to pull in additional dependencies. If these transitive dependencies have overlapping packages, conflicts can arise.
-
Version Mismatches: Differences in versions can lead to different implementations of the same class. If your application includes two versions of the same dependency, your application may face runtime issues.
-
Classloader Behavior: The JVM classloader works hierarchically. If classes with the same name are loaded from different locations in the hierarchy, it may not load the expected version.
Diagnosing Classpath Conflicts
Identifying classpath conflicts can be tricky, but there are approaches and tools to assist:
-
Dependency Tree: Both Maven and Gradle provide commands to print out the dependency tree. This can help you visualize your dependencies and spot conflicts.
-
For Maven:
mvn dependency:tree
-
For Gradle:
./gradlew dependencies
-
-
Using
jar
Command: You can inspect the contents of individual JAR files using thejar -tvf
command. Listing classes in suspect JARs can help pinpoint conflicts. -
ClassNotFoundException: If you encounter a
ClassNotFoundException
, check which libraries are providing the class and their respective versions.
Resolving Classpath Conflicts
1. Exclude Conflicting Dependencies
In many cases, you can exclude problematic transitive dependencies.
For Gradle:
dependencies {
implementation('com.example:libA:1.0.0') {
exclude group: 'com.example', module: 'libB'
}
}
This configuration excludes libB
, which may be conflicting within the resolution process.
2. Shade/Relocate Dependencies
If you cannot avoid including two conflicting libraries, consider shading or relocating one of the libraries to change its package namespace.
For example, using the Shadow Plugin you can relocate:
shadowJar {
relocate 'com.example.lib', 'myapp.relocated.lib'
}
This effectively "hides" the original package, avoiding classloading issues.
3. Dependency Convergence
Strive for dependency convergence by managing versions consistently across your projects. If using Maven, you can use the <dependencyManagement>
tag to specify versions clearly.
4. Classpath Order
The order in which the classpath is set can define which classes are loaded first. Ensure that more specific dependencies come before more general ones.
Best Practices for Avoiding Classpath Conflicts
- Use Well-known Libraries: Favor established libraries that are known for compatibility.
- Maintain a Consistent Dependency Tree: Regularly analyze your dependency tree to catch conflicts early.
- Update Dependencies: Keep your dependencies up-to-date. This often leads to resolving issues that can arise from outdated libraries.
- Use Dependency Barriers: Where feasible, apply barriers to shield your application modules from one another in larger systems.
Closing Remarks
Classpath conflicts in One Jar deployments can be a source of frustration, but understanding their root causes and employing best practices can greatly minimize those issues. From diagnosing dependencies with tree explorations to configuring your build scripts effectively, there are several proactive solutions available. By following a structured approach and employing sound practices, you can ensure your Java applications run smoothly with all dependencies packaged together.
For further reading, consider exploring Maven Dependency Management or Gradle Dependency Management for deeper insights.
With proper awareness and strategies in place, classpath conflicts can be navigated effectively, paving the way for seamless Java application deployments. Happy coding!