Common Mistakes with Java Annotations in Spring Framework
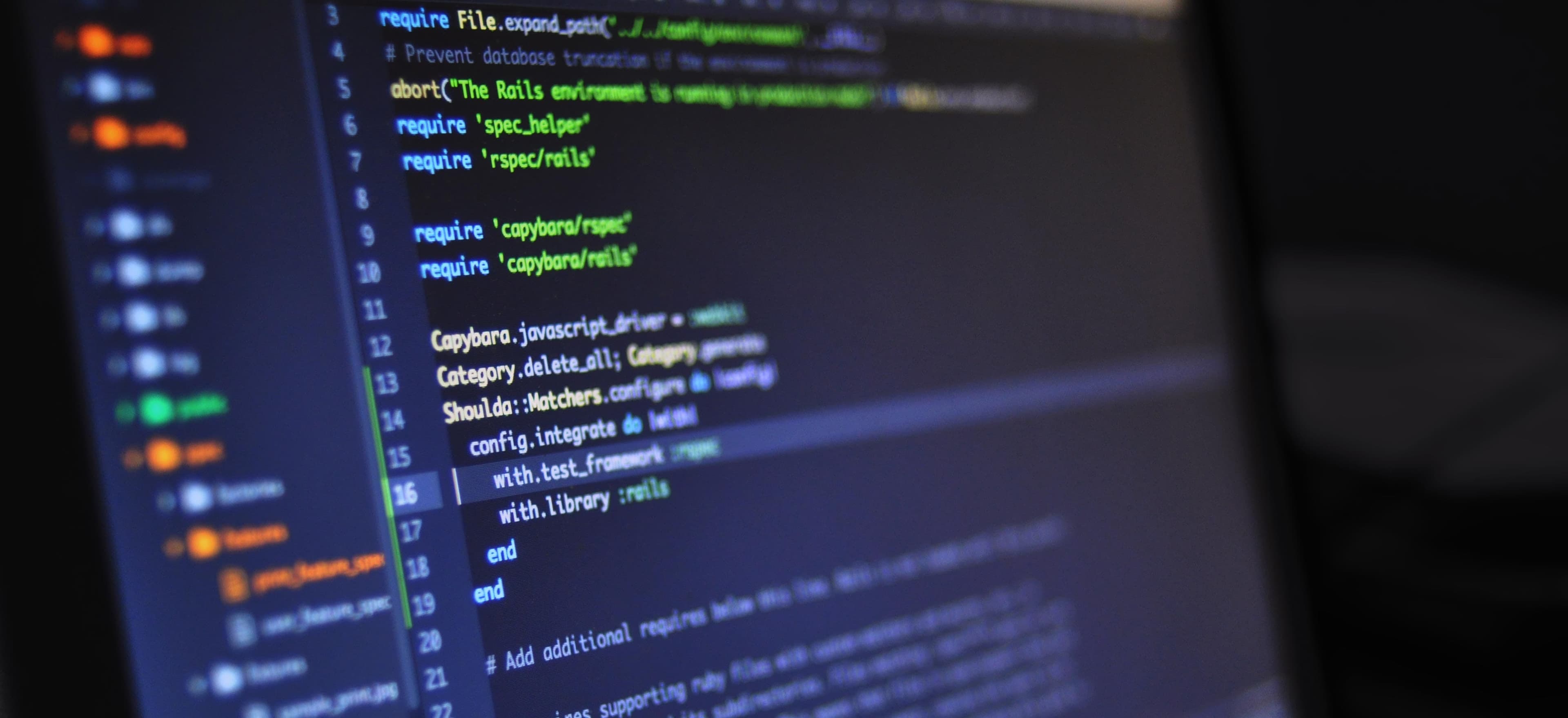
- Published on
Common Mistakes with Java Annotations in the Spring Framework
Java annotations provide a powerful mechanism to add metadata to your Java classes and methods. In the context of the Spring Framework, annotations greatly simplify the development process by reducing the amount of boilerplate code required to configure applications. However, misusing these annotations can lead to errors, performance issues, or unexpected behavior. In this blog post, we will explore some common mistakes developers make when using Java annotations in Spring and how to avoid them.
Understanding Java Annotations in Spring
Java annotations are essentially a form of metadata. They provide information about the program but are not part of the program itself. Spring uses annotations for various purposes, such as dependency injection, transaction management, and aspect-oriented programming. Here’s a simple example of how Java annotations are used in Spring:
import org.springframework.stereotype.Service;
@Service
public class MyService {
public String greet() {
return "Hello, World!";
}
}
In this case, the @Service
annotation indicates that this class is a service component in the Spring application context.
1. Overusing Component Scanning
The Issue
Spring’s component scanning automatically detects classes marked with certain annotations like @Component
, @Service
, @Controller
, and @Repository
. However, one common mistake is to overuse component scanning across the entire project or in packages that contain unrelated classes.
The Fix
Be selective with the packages you include in component scanning. Define your scanning base package explicitly using either XML or Java-based configurations.
Here's an example of scanning a specific package using Java configuration:
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
@Configuration
@ComponentScan(basePackages = "com.example.services")
public class AppConfig {
}
Why It Matters
By limiting the scope of component scanning, you minimize the risk of loading unnecessary components and improve your application's performance.
2. Incorrect Usage of @Autowired
The Issue
The @Autowired
annotation is widely used for dependency injection. A common mistake is to forget to handle the required dependency when using @Autowired
. By default, if Spring cannot find a suitable bean for autowiring, it will throw an exception.
The Fix
To avoid application failure, use the required
attribute:
@Autowired(required = false)
private SomeService someService;
Why It Matters
Setting required = false
allows your application to run without that particular bean. However, you must be cautious, as it can hide issues related to missing dependencies.
3. Not Using @Primary
The Issue
When you have multiple beans of the same type, Spring does not know which one to inject. This can result in a runtime exception.
The Fix
When multiple beans of the same type are available, you can mark one of them as @Primary
.
import org.springframework.context.annotation.Primary;
@Primary
@Bean
public SomeService defaultService() {
return new DefaultSomeService();
}
@Bean
public SomeService anotherService() {
return new AnotherSomeService();
}
Why It Matters
Setting a primary bean helps Spring resolve ambiguities automatically, leading to cleaner and more manageable code.
4. Forgetting to Use @Transactional at Method Level
The Issue
The @Transactional
annotation is crucial for managing transactions in your application. A typical mistake is to overlook applying it at the method level, instead applying it only to the class. This won’t provide transaction management for methods that don't require the entire class's transactional boundaries.
The Fix
Apply @Transactional
on a per-method basis if certain methods require different transaction configurations.
@Transactional(readOnly = true)
public List<User> fetchAllUsers() {
return userRepository.findAll();
}
@Transactional
public void createUser(User user) {
userRepository.save(user);
}
Why It Matters
Fine-grained transaction management ensures that your application behaves predictably and reliably, reducing the risk of data inconsistencies.
5. Ignoring the Scope of Beans
The Issue
Beans can be defined at different scopes, including Singleton, Prototype, Request, Session, etc. A common mistake is to overlook the default Singleton scope, leading to unexpected shared state issues.
The Fix
Make sure to explicitly define the desired scope for your beans, especially in a web application.
import org.springframework.context.annotation.Scope;
@Scope("prototype")
@Bean
public SomeBean someBean() {
return new SomeBean();
}
Why It Matters
Properly managing bean scope prevents state leakage between requests or sessions in a web application, promoting better application design.
6. Not Understanding AOP with @Aspect
The Issue
Aspect-Oriented Programming (AOP) is a powerful feature in Spring that allows you to add cross-cutting concerns like logging, security, or transactions. However, improperly using or misunderstanding the @Aspect
annotation can lead to broken functionality.
The Fix
Always ensure that your pointcuts accurately define where your aspects should be applied using expressions.
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
@Aspect
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBeforeMethod() {
System.out.println("A method is being called.");
}
}
Why It Matters
Defining precise pointcuts allows for better control over the aspects' behavior and helps avoid unintended consequences.
7. Avoiding Context Configuration Errors
The Issue
Misconfigurations in your Spring context can lead to application startup failures. This often occurs when beans depend on one another, creating cyclic dependencies.
The Fix
- Always check bean dependencies.
- Use
@Lazy
and@PostConstruct
annotations as needed to break cycles.
Example:
@Lazy
@Autowired
private AnotherService anotherService;
Why It Matters
Properly managing bean configuration reduces configuration overhead and promotes a more robust application structure.
Final Thoughts
Java annotations in the Spring Framework can significantly enhance developer productivity, but it's crucial to use them wisely. By avoiding the common mistakes outlined above, developers can build more efficient, maintainable, and predictable applications.
For further reading on Java annotations and their usage in Spring, check out the following resources:
- Spring Framework Documentation
- Spring Annotations
- Effective Java: Annotations Guide
Remember, the power of annotations lies not just in their ability to add behavior but also in the clarity and simplicity they can bring to our code. Happy coding!