Common J2Pay Integration Issues and How to Fix Them
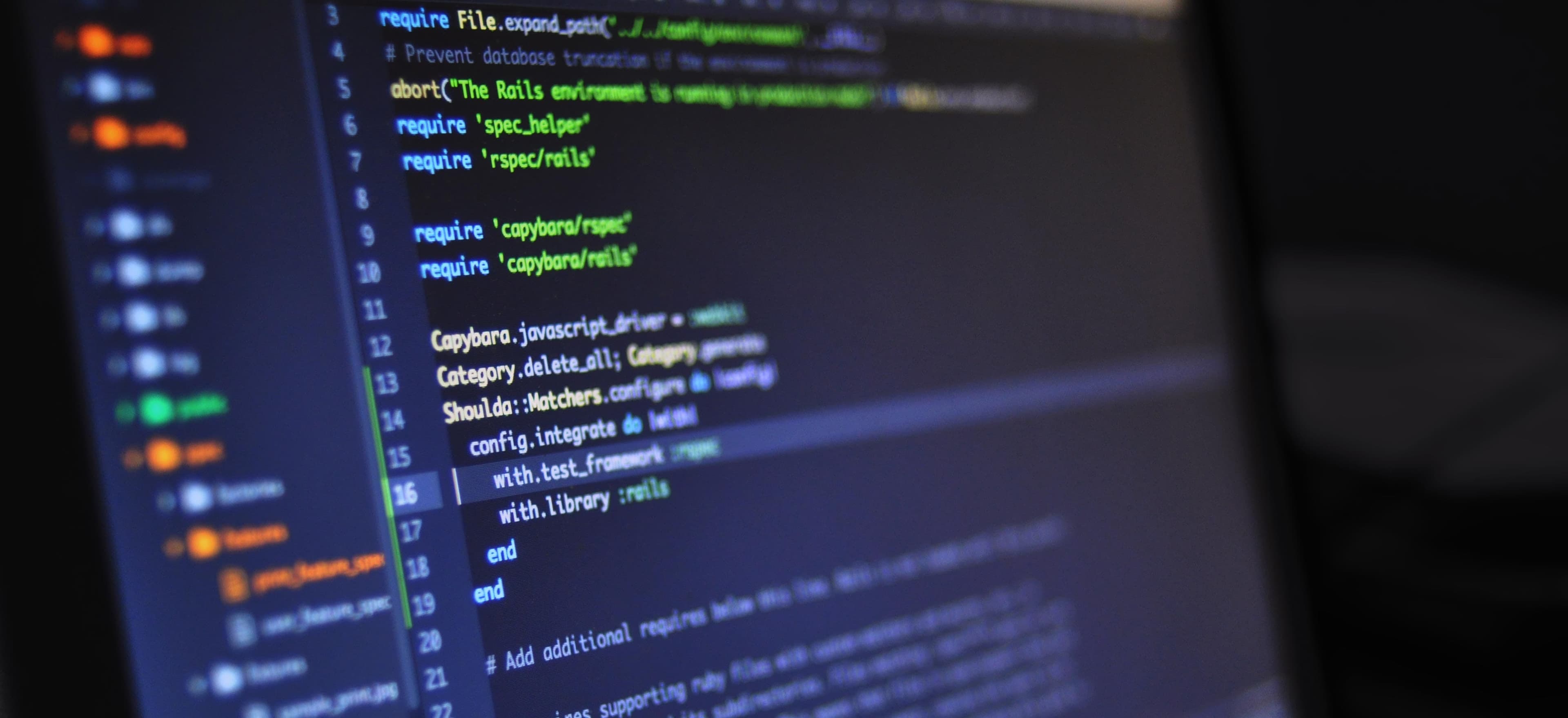
- Published on
Common J2Pay Integration Issues and How to Fix Them
Integrating J2Pay into your Java application can open the door to seamless transactions and a better user experience. However, developers often encounter common issues during the integration process. In this post, we will tackle these issues head-on and provide you with effective solutions to ensure a smooth implementation.
Table of Contents
- Understanding J2Pay
- Common Issues When Integrating J2Pay
- Incorrect API Keys
- Misconfigured Dependencies
- Network and Connectivity Errors
- Invalid Payment Data
- Troubleshooting Steps
- Best Practices for J2Pay Integration
- Conclusion
Understanding J2Pay
J2Pay is a widely-used payment processing gateway that enables businesses to handle online transactions securely. The platform provides a straightforward API, allowing developers to integrate its capabilities directly into their Java applications. To ensure you have a smooth experience, understanding the components involved in J2Pay integration is crucial.
The integration typically involves signing up for a merchant account, obtaining API keys, and setting up the necessary libraries and dependencies in your project. Detailed documentation can be found on the J2Pay Developer Site.
Common Issues When Integrating J2Pay
1. Incorrect API Keys
One of the most frequent hurdles developers face is using incorrect or outdated API keys. API keys serve as the credentials for your application to authenticate with the J2Pay services. When they are not configured correctly, transactions may fail or return error messages.
// Example: Using API keys in your J2Pay integration
String apiKey = "YOUR_API_KEY_HERE"; // Ensure this key is correct
PaymentProcessor paymentProcessor = new PaymentProcessor(apiKey);
Solution: Always double-check the API keys in your configuration file and ensure that you’ve copied them from the J2Pay dashboard without any extra spaces.
2. Misconfigured Dependencies
Java projects often use build systems like Maven or Gradle for dependency management. If the J2Pay library is not properly included, you may encounter issues during the compilation or runtime.
<!-- Example: Maven dependency for J2Pay -->
<dependency>
<groupId>com.j2pay</groupId>
<artifactId>j2pay-sdk</artifactId>
<version>1.0.0</version>
</dependency>
Solution: Verify that you've added the correct dependency version to your pom.xml
file (for Maven) or your build.gradle
file (for Gradle). Run a build to check for any unresolved dependencies.
3. Network and Connectivity Errors
Integration with any payment service requires reliable internet connectivity. Temporary outages or misconfigurations in your networking settings can lead to failures when trying to reach J2Pay servers.
Solution: Confirm that your application can reach the J2Pay URLs from your server or development environment. You can use simple command line tools like ping
or curl
to verify connectivity.
# Example: Check connectivity
curl https://api.j2pay.com/v1/transaction
If you receive a response, your connectivity is intact. If not, delve deeper into your network settings.
4. Invalid Payment Data
J2Pay requires that all payment transactions are sent with valid data. Missing fields, incorrect data types (e.g., a string instead of integer), or malformed requests can lead to payment submission failures.
// Example: Validating payment data before sending
if (amount <= 0) {
throw new IllegalArgumentException("Amount must be greater than zero.");
}
Solution: Always validate your payment data before sending requests to J2Pay. Doing so will minimize the chances of rejected transactions. Review the J2Pay API documentation to ensure that you meet the required specifications.
Troubleshooting Steps
When you face any of the issues mentioned, follow these troubleshooting steps:
-
Logs: Enable logging in your application to capture request and response data for J2Pay transactions. This will provide insights into what went wrong.
-
Read Error Messages: J2Pay typically returns error messages that can guide you in resolving integration issues. Pay attention to the status codes and error details.
-
Test Environment: Utilize the test environment provided by J2Pay for staging transactions before going live. This helps catch issues early in the development cycle.
-
Community and Support: If you're stuck, leverage online forums and J2Pay support. Chances are, someone has encountered and resolved the same issue.
Best Practices for J2Pay Integration
To ensure a successful integration process, consider following these best practices:
-
Maintain API Key Security: Do not hardcode your API keys within your code base. Use environment variables or secure vaults.
-
Version Management: Regularly update your dependencies and monitor updates from J2Pay for any API changes.
-
Error Handling: Implement robust error handling mechanisms to deal with possible failures gracefully. This way, users receive informative messages instead of cryptic errors.
-
Data Encryption: Ensure that sensitive data, such as credit card information, is transmitted securely using encryption standards to comply with PCI DSS guidelines.
-
Monitor Transactions: Set up monitoring or alerts to track your payment transactions for unusual activities.
Closing the Chapter
Integrating J2Pay into your Java application can come with its fair share of challenges. However, by being aware of common pitfalls such as API key errors, dependency issues, and invalid payment data, you can tackle them effectively. Following the best practices and troubleshooting steps outlined in this guide will not only help you fix issues as they arise but also flatline potential mistakes in future integrations.
Ready to begin your J2Pay integration journey? For more detailed information, visit the J2Pay official documentation.
Happy coding and happy transactions!